diff --git a/.gitignore b/.gitignore
index 259148f..26c9709 100644
--- a/.gitignore
+++ b/.gitignore
@@ -1,32 +1,18 @@
-# Prerequisites
-*.d
-
-# Compiled Object files
-*.slo
-*.lo
-*.o
-*.obj
-
-# Precompiled Headers
-*.gch
-*.pch
-
-# Compiled Dynamic libraries
-*.so
-*.dylib
-*.dll
-
-# Fortran module files
-*.mod
-*.smod
-
-# Compiled Static libraries
-*.lai
-*.la
-*.a
-*.lib
-
-# Executables
-*.exe
-*.out
-*.app
+*.bat
+*.log
+*.lnk
+**/msvc/Debug*
+**/msvc/Release*
+**/msvc/Tests
+**/msvc/*.sdf
+**/msvc/*.opensdf
+**/msvc/*.user
+**/msvc/*.suo
+**/msvc/*.db
+**/msvc/*.opendb
+**/msvc/*.txt
+**/msvc/*.amplxeproj
+**/msvc/.vs
+**/msvc/ipch
+**/msvc/PublishPath*.ini
+publish
diff --git a/README.md b/README.md
index 3f0b415..7cb4dd4 100644
--- a/README.md
+++ b/README.md
@@ -1,2 +1,52 @@
-# hitboxtracker
-Demonstrate position hitboxes calculated by server on client-side
+# Description
+Dev-tool that demonstrates on client-side true position of the hitboxes calculated by server
+
+# Installation
+* Install `hitboxtracker_mm.dll` on your HLDS as metamod plugin and put `delta.lst` to gamedir directory `cstrike/delta.lst`
+* Put `hitboxtracker.dll` and `cs.exe` to working directory of the game CS 1.6 client
+* Run `cs.exe`
+
+# Requirements
+* For `Client`: build 4554 and later
+* For `HLDS`: Metamod 1.20 and later
+
+# Configuring
+
+
+
+ r_drawentities |
+ Description |
+
+
+ 0 |
+ No entities |
+
+
+ 1 |
+ Default and draws entities normally |
+
+
+ 2 |
+ Entities draws as skeletons |
+
+
+ 3 |
+ Entities draws hitboxes |
+
+
+ 4 |
+ Entities draws with translucent hitboxes and the model beneath the hitboxes |
+
+
+ 5 |
+ Individual box for player and weapon |
+
+
+ 6 |
+ Same as 4 but draws true position of the hitboxes calculated by server :new: |
+
+
+
+
+# Preview
+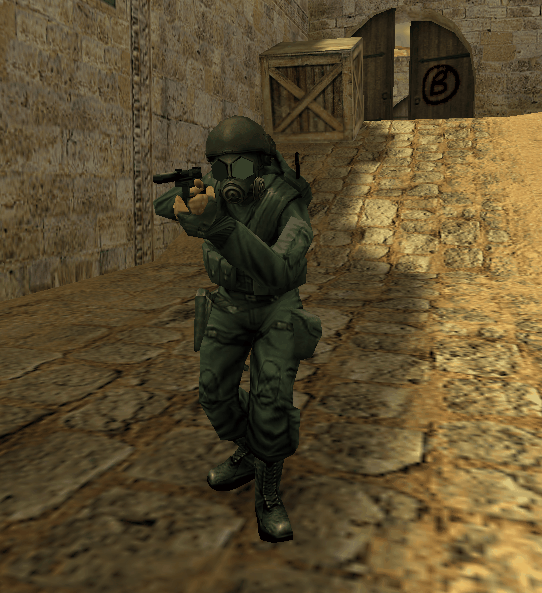
diff --git a/dep/ISysModule.h b/dep/ISysModule.h
new file mode 100644
index 0000000..f2ad44a
--- /dev/null
+++ b/dep/ISysModule.h
@@ -0,0 +1,37 @@
+#pragma once
+
+#define PSAPI_VERSION 1
+#include
+
+using module_handle_t = HINSTANCE;
+using byteptr_t = uint8_t *;
+
+enum OpCodes : int8_t
+{
+ OP_ANY = '\x2A',
+ OP_PUSH = '\x68',
+ OP_JUMP = '\xE9',
+ OP_CALL = '\xE8',
+};
+
+class ISysModule
+{
+public:
+ virtual ~ISysModule() {}
+ virtual bool Init(const char *szModuleName, const char *pszFile = nullptr) = 0;
+
+ virtual module_handle_t load(void *addr) = 0;
+ virtual module_handle_t load(const char *filename) = 0;
+
+ virtual void Printf(const char *fmt, ...) = 0;
+ virtual void TraceLog(const char *fmt, ...) = 0;
+
+ virtual byteptr_t getsym(const char *name) const = 0;
+ virtual module_handle_t gethandle() const = 0;
+ virtual byteptr_t getbase() const = 0;
+ virtual size_t getsize() const = 0;
+ virtual bool is_opened() const = 0;
+
+ virtual byteptr_t find_string(const char *string, int8_t opcode = OP_ANY) const = 0;
+ virtual byteptr_t find_pattern(byteptr_t pStart, size_t range, const char *pattern) const = 0;
+};
diff --git a/dep/hlsdk/common/BaseSystemModule.cpp b/dep/hlsdk/common/BaseSystemModule.cpp
new file mode 100644
index 0000000..3233b54
--- /dev/null
+++ b/dep/hlsdk/common/BaseSystemModule.cpp
@@ -0,0 +1,164 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#include "precompiled.h"
+
+BaseSystemModule::BaseSystemModule()
+{
+ m_System = nullptr;
+ m_Serial = 0;
+ m_SystemTime = 0;
+ m_State = MODULE_UNDEFINED;
+
+ Q_memset(m_Name, 0, sizeof(m_Name));
+}
+
+char *BaseSystemModule::GetName()
+{
+ return m_Name;
+}
+
+char *BaseSystemModule::GetType()
+{
+ return "GenericModule";
+}
+
+char *BaseSystemModule::GetStatusLine()
+{
+ return "No status available.\n";
+}
+
+void BaseSystemModule::ExecuteCommand(int commandID, char *commandLine)
+{
+ m_System->DPrintf("WARNING! Undeclared ExecuteCommand().\n");
+}
+
+extern int COM_BuildNumber();
+
+int BaseSystemModule::GetVersion()
+{
+ return COM_BuildNumber();
+}
+
+int BaseSystemModule::GetSerial()
+{
+ return m_Serial;
+}
+
+IBaseSystem *BaseSystemModule::GetSystem()
+{
+ return m_System;
+}
+
+bool BaseSystemModule::Init(IBaseSystem *system, int serial, char *name)
+{
+ if (!system)
+ return false;
+
+ m_State = MODULE_INITIALIZING;
+ m_System = system;
+ m_Serial = serial;
+ m_SystemTime = 0;
+
+ if (name) {
+ Q_strlcpy(m_Name, name);
+ }
+
+ return true;
+}
+
+void BaseSystemModule::RunFrame(double time)
+{
+ m_SystemTime = time;
+}
+
+void BaseSystemModule::ShutDown()
+{
+ if (m_State == MODULE_DISCONNECTED)
+ return;
+
+ m_Listener.Clear();
+ m_State = MODULE_DISCONNECTED;
+
+ if (!m_System->RemoveModule(this))
+ {
+ m_System->DPrintf("ERROR! BaseSystemModule::ShutDown: faild to remove module %s.\n", m_Name);
+ }
+}
+
+void BaseSystemModule::RegisterListener(ISystemModule *module)
+{
+ ISystemModule *listener = (ISystemModule *)m_Listener.GetFirst();
+ while (listener)
+ {
+ if (listener->GetSerial() == module->GetSerial())
+ {
+ m_System->DPrintf("WARNING! BaseSystemModule::RegisterListener: module %s already added.\n", module->GetName());
+ return;
+ }
+
+ listener = (ISystemModule *)m_Listener.GetNext();
+ }
+
+ m_Listener.Add(module);
+}
+
+void BaseSystemModule::RemoveListener(ISystemModule *module)
+{
+ ISystemModule *listener = (ISystemModule *)m_Listener.GetFirst();
+ while (listener)
+ {
+ if (listener->GetSerial() == module->GetSerial())
+ {
+ m_Listener.Remove(module);
+ return;
+ }
+
+ listener = (ISystemModule *)m_Listener.GetNext();
+ }
+}
+
+void BaseSystemModule::FireSignal(unsigned int signal, void *data)
+{
+ ISystemModule *listener = (ISystemModule *)m_Listener.GetFirst();
+ while (listener)
+ {
+ listener->ReceiveSignal(this, signal, data);
+ listener = (ISystemModule *)m_Listener.GetNext();
+ }
+}
+
+void BaseSystemModule::ReceiveSignal(ISystemModule *module, unsigned int signal, void *data)
+{
+ m_System->DPrintf("WARNING! Unhandled signal (%i) from module %s.\n", signal, module->GetName());
+}
+
+int BaseSystemModule::GetState()
+{
+ return m_State;
+}
diff --git a/dep/hlsdk/common/BaseSystemModule.h b/dep/hlsdk/common/BaseSystemModule.h
new file mode 100644
index 0000000..f4998b4
--- /dev/null
+++ b/dep/hlsdk/common/BaseSystemModule.h
@@ -0,0 +1,75 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "ObjectList.h"
+#include "IBaseSystem.h"
+
+// C4250 - 'class1' : inherits 'BaseSystemModule::member' via dominance
+#pragma warning(disable:4250)
+
+class BaseSystemModule: virtual public ISystemModule {
+public:
+ BaseSystemModule();
+ virtual ~BaseSystemModule() {}
+
+ EXT_FUNC virtual bool Init(IBaseSystem *system, int serial, char *name);
+ EXT_FUNC virtual void RunFrame(double time);
+ EXT_FUNC virtual void ReceiveSignal(ISystemModule *module, unsigned int signal, void *data);
+ EXT_FUNC virtual void ExecuteCommand(int commandID, char *commandLine);
+ EXT_FUNC virtual void RegisterListener(ISystemModule *module);
+ EXT_FUNC virtual void RemoveListener(ISystemModule *module);
+ EXT_FUNC virtual IBaseSystem *GetSystem();
+ EXT_FUNC virtual int GetSerial();
+ EXT_FUNC virtual char *GetStatusLine();
+ EXT_FUNC virtual char *GetType();
+ EXT_FUNC virtual char *GetName();
+
+ enum ModuleState {
+ MODULE_UNDEFINED = 0,
+ MODULE_INITIALIZING,
+ MODULE_CONNECTING,
+ MODULE_RUNNING,
+ MODULE_DISCONNECTED
+ };
+
+ EXT_FUNC virtual int GetState();
+ EXT_FUNC virtual int GetVersion();
+ EXT_FUNC virtual void ShutDown();
+ EXT_FUNC virtual char *GetBaseDir() { return ""; }
+ void FireSignal(unsigned int signal, void *data = nullptr);
+
+protected:
+ IBaseSystem *m_System;
+ ObjectList m_Listener;
+ char m_Name[255];
+ unsigned int m_State;
+ unsigned int m_Serial;
+ double m_SystemTime;
+};
diff --git a/dep/hlsdk/common/IAdminServer.h b/dep/hlsdk/common/IAdminServer.h
new file mode 100644
index 0000000..ab367ce
--- /dev/null
+++ b/dep/hlsdk/common/IAdminServer.h
@@ -0,0 +1,54 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "interface.h"
+
+// handle to a game window
+typedef unsigned int ManageServerUIHandle_t;
+class IManageServer;
+
+// Purpose: Interface to server administration functions
+class IAdminServer: public IBaseInterface
+{
+public:
+ // opens a manage server dialog for a local server
+ virtual ManageServerUIHandle_t OpenManageServerDialog(const char *serverName, const char *gameDir) = 0;
+
+ // opens a manage server dialog to a remote server
+ virtual ManageServerUIHandle_t OpenManageServerDialog(unsigned int gameIP, unsigned int gamePort, const char *password) = 0;
+
+ // forces the game info dialog closed
+ virtual void CloseManageServerDialog(ManageServerUIHandle_t gameDialog) = 0;
+
+ // Gets a handle to the interface
+ virtual IManageServer *GetManageServerInterface(ManageServerUIHandle_t handle) = 0;
+};
+
+#define ADMINSERVER_INTERFACE_VERSION "AdminServer002"
diff --git a/dep/hlsdk/common/IBaseSystem.h b/dep/hlsdk/common/IBaseSystem.h
new file mode 100644
index 0000000..9f50fe3
--- /dev/null
+++ b/dep/hlsdk/common/IBaseSystem.h
@@ -0,0 +1,91 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#if defined(_WIN32)
+ #define LIBRARY_PREFIX "dll"
+#elif defined(OSX)
+ #define LIBRARY_PREFIX "dylib"
+#else
+ #define LIBRARY_PREFIX "so"
+#endif
+
+#include "ISystemModule.h"
+#include "IVGuiModule.h"
+
+class Panel;
+class ObjectList;
+class IFileSystem;
+
+class IBaseSystem: virtual public ISystemModule {
+public:
+ virtual ~IBaseSystem() {}
+
+ virtual double GetTime() = 0;
+ virtual unsigned int GetTick() = 0;
+ virtual void SetFPS(float fps) = 0;
+
+ virtual void Printf(char *fmt, ...) = 0;
+ virtual void DPrintf(char *fmt, ...) = 0;
+
+ virtual void RedirectOutput(char *buffer = nullptr, int maxSize = 0) = 0;
+
+ virtual IFileSystem *GetFileSystem() = 0;
+ virtual unsigned char *LoadFile(const char *name, int *length = nullptr) = 0;
+ virtual void FreeFile(unsigned char *fileHandle) = 0;
+
+ virtual void SetTitle(char *text) = 0;
+ virtual void SetStatusLine(char *text) = 0;
+
+ virtual void ShowConsole(bool visible) = 0;
+ virtual void LogConsole(char *filename) = 0;
+
+ virtual bool InitVGUI(IVGuiModule *module) = 0;
+
+#ifdef _WIN32
+ virtual Panel *GetPanel() = 0;
+#endif // _WIN32
+
+ virtual bool RegisterCommand(char *name, ISystemModule *module, int commandID) = 0;
+ virtual void GetCommandMatches(char *string, ObjectList *pMatchList) = 0;
+ virtual void ExecuteString(char *commands) = 0;
+ virtual void ExecuteFile(char *filename) = 0;
+ virtual void Errorf(char *fmt, ...) = 0;
+
+ virtual char *CheckParam(char *param) = 0;
+
+ virtual bool AddModule(ISystemModule *module, char *name) = 0;
+ virtual ISystemModule *GetModule(char *interfacename, char *library, char *instancename = nullptr) = 0;
+ virtual bool RemoveModule(ISystemModule *module) = 0;
+
+ virtual void Stop() = 0;
+ virtual char *GetBaseDir() = 0;
+};
+
+#define BASESYSTEM_INTERFACE_VERSION "basesystem002"
diff --git a/dep/hlsdk/common/IDemoPlayer.h b/dep/hlsdk/common/IDemoPlayer.h
new file mode 100644
index 0000000..2431b54
--- /dev/null
+++ b/dep/hlsdk/common/IDemoPlayer.h
@@ -0,0 +1,93 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "ref_params.h"
+
+class IWorld;
+class IProxy;
+class DirectorCmd;
+class IBaseSystem;
+class ISystemModule;
+class IObjectContainer;
+
+class IDemoPlayer {
+public:
+ virtual ~IDemoPlayer() {}
+
+ virtual bool Init(IBaseSystem *system, int serial, char *name) = 0;
+ virtual void RunFrame(double time) = 0;
+ virtual void ReceiveSignal(ISystemModule *module, unsigned int signal, void *data) = 0;
+ virtual void ExecuteCommand(int commandID, char *commandLine) = 0;
+ virtual void RegisterListener(ISystemModule *module) = 0;
+ virtual void RemoveListener(ISystemModule *module) = 0;
+ virtual IBaseSystem *GetSystem() = 0;
+ virtual int GetSerial() = 0;
+ virtual char *GetStatusLine() = 0;
+ virtual char *GetType() = 0;
+ virtual char *GetName() = 0;
+ virtual int GetState() = 0;
+ virtual int GetVersion() = 0;
+ virtual void ShutDown() = 0;
+
+ virtual void NewGame(IWorld *world, IProxy *proxy = nullptr) = 0;
+ virtual char *GetModName() = 0;
+ virtual void WriteCommands(BitBuffer *stream, float startTime, float endTime) = 0;
+ virtual int AddCommand(DirectorCmd *cmd) = 0;
+ virtual bool RemoveCommand(int index) = 0;
+ virtual DirectorCmd *GetLastCommand() = 0;
+ virtual IObjectContainer *GetCommands() = 0;
+ virtual void SetWorldTime(double time, bool relative) = 0;
+ virtual void SetTimeScale(float scale) = 0;
+ virtual void SetPaused(bool state) = 0;
+ virtual void SetEditMode(bool state) = 0;
+ virtual void SetMasterMode(bool state) = 0;
+ virtual bool IsPaused() = 0;
+ virtual bool IsLoading() = 0;
+ virtual bool IsActive() = 0;
+ virtual bool IsEditMode() = 0;
+ virtual bool IsMasterMode() = 0;
+ virtual void RemoveFrames(double starttime, double endtime) = 0;
+ virtual void ExecuteDirectorCmd(DirectorCmd *cmd) = 0;
+ virtual double GetWorldTime() = 0;
+ virtual double GetStartTime() = 0;
+ virtual double GetEndTime() = 0;
+ virtual float GetTimeScale() = 0;
+ virtual IWorld *GetWorld() = 0;
+ virtual char *GetFileName() = 0;
+ virtual bool SaveGame(char *filename) = 0;
+ virtual bool LoadGame(char *filename) = 0;
+ virtual void Stop() = 0;
+ virtual void ForceHLTV(bool state) = 0;
+ virtual void GetDemoViewInfo(ref_params_t *rp, float *view, int *viewmodel) = 0;
+ virtual int ReadDemoMessage(unsigned char *buffer, int size) = 0;
+ virtual void ReadNetchanState(int *incoming_sequence, int *incoming_acknowledged, int *incoming_reliable_acknowledged, int *incoming_reliable_sequence, int *outgoing_sequence, int *reliable_sequence, int *last_reliable_sequence) = 0;
+};
+
+#define DEMOPLAYER_INTERFACE_VERSION "demoplayer001"
diff --git a/dep/hlsdk/common/IEngineWrapper.h b/dep/hlsdk/common/IEngineWrapper.h
new file mode 100644
index 0000000..0a58d4c
--- /dev/null
+++ b/dep/hlsdk/common/IEngineWrapper.h
@@ -0,0 +1,56 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "event_args.h"
+#include "vmodes.h"
+#include "cdll_int.h"
+
+class IBaseSystem;
+class ISystemModule;
+
+class IEngineWrapper: virtual public ISystemModule {
+public:
+ virtual ~IEngineWrapper() {}
+
+ virtual bool GetViewOrigin(float *origin) = 0;
+ virtual bool GetViewAngles(float *angles) = 0;
+ virtual int GetTraceEntity() = 0;
+ virtual float GetCvarFloat(char *szName) = 0;
+ virtual char *GetCvarString(char *szName) = 0;
+ virtual void SetCvar(char *szName, char *szValue) = 0;
+ virtual void Cbuf_AddText(char *text) = 0;
+ virtual void DemoUpdateClientData(client_data_t *cdat) = 0;
+ virtual void CL_QueueEvent(int flags, int index, float delay, event_args_t *pargs) = 0;
+ virtual void HudWeaponAnim(int iAnim, int body) = 0;
+ virtual void CL_DemoPlaySound(int channel, char *sample, float attenuation, float volume, int flags, int pitch) = 0;
+ virtual void ClientDLL_ReadDemoBuffer(int size, unsigned char *buffer) = 0;
+};
+
+#define ENGINEWRAPPER_INTERFACE_VERSION "enginewrapper001"
diff --git a/dep/hlsdk/common/IGameServerData.h b/dep/hlsdk/common/IGameServerData.h
new file mode 100644
index 0000000..d431f00
--- /dev/null
+++ b/dep/hlsdk/common/IGameServerData.h
@@ -0,0 +1,15 @@
+#pragma once
+
+#include "maintypes.h"
+#include "interface.h"
+
+class IGameServerData : public IBaseInterface {
+public:
+ virtual ~IGameServerData() { };
+
+ virtual void WriteDataRequest(const void *buffer, int bufferSize) = 0;
+
+ virtual int ReadDataResponse(void *data, int len) = 0;
+};
+
+#define GAMESERVERDATA_INTERFACE_VERSION "GameServerData001"
diff --git a/dep/hlsdk/common/IObjectContainer.h b/dep/hlsdk/common/IObjectContainer.h
new file mode 100644
index 0000000..333e9d0
--- /dev/null
+++ b/dep/hlsdk/common/IObjectContainer.h
@@ -0,0 +1,47 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class IObjectContainer {
+public:
+ virtual ~IObjectContainer() {}
+
+ virtual void Init() = 0;
+
+ virtual bool Add(void *newObject) = 0;
+ virtual bool Remove(void *object) = 0;
+ virtual void Clear(bool freeElementsMemory) = 0;
+
+ virtual void *GetFirst() = 0;
+ virtual void *GetNext() = 0;
+
+ virtual int CountElements() = 0;
+ virtual bool Contains(void *object) = 0;
+ virtual bool IsEmpty() = 0;
+};
diff --git a/dep/hlsdk/common/ISystemModule.h b/dep/hlsdk/common/ISystemModule.h
new file mode 100644
index 0000000..9004a95
--- /dev/null
+++ b/dep/hlsdk/common/ISystemModule.h
@@ -0,0 +1,57 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "interface.h"
+
+class IBaseSystem;
+class ISystemModule;
+
+class ISystemModule: public IBaseInterface {
+public:
+ virtual ~ISystemModule() {}
+ virtual bool Init(IBaseSystem *system, int serial, char *name) = 0;
+
+ virtual void RunFrame(double time) = 0;
+ virtual void ReceiveSignal(ISystemModule *module, unsigned int signal, void *data = nullptr) = 0;
+ virtual void ExecuteCommand(int commandID, char *commandLine) = 0;
+ virtual void RegisterListener(ISystemModule *module) = 0;
+ virtual void RemoveListener(ISystemModule *module) = 0;
+
+ virtual IBaseSystem *GetSystem() = 0;
+
+ virtual int GetSerial() = 0;
+ virtual char *GetStatusLine() = 0;
+ virtual char *GetType() = 0;
+ virtual char *GetName() = 0;
+
+ virtual int GetState() = 0;
+ virtual int GetVersion() = 0;
+ virtual void ShutDown() = 0;
+};
diff --git a/dep/hlsdk/common/IVGuiModule.h b/dep/hlsdk/common/IVGuiModule.h
new file mode 100644
index 0000000..e6864c8
--- /dev/null
+++ b/dep/hlsdk/common/IVGuiModule.h
@@ -0,0 +1,76 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include
+#include "interface.h"
+
+// Purpose: Standard interface to loading vgui modules
+class IVGuiModule: public IBaseInterface
+{
+public:
+ // called first to setup the module with the vgui
+ // returns true on success, false on failure
+ virtual bool Initialize(CreateInterfaceFn *vguiFactories, int factoryCount) = 0;
+
+ // called after all the modules have been initialized
+ // modules should use this time to link to all the other module interfaces
+ virtual bool PostInitialize(CreateInterfaceFn *modules = nullptr, int factoryCount = 0) = 0;
+
+ // called when the module is selected from the menu or otherwise activated
+ virtual bool Activate() = 0;
+
+ // returns true if the module is successfully initialized and available
+ virtual bool IsValid() = 0;
+
+ // requests that the UI is temporarily disabled and all data files saved
+ virtual void Deactivate() = 0;
+
+ // restart from a Deactivate()
+ virtual void Reactivate() = 0;
+
+ // called when the module is about to be shutdown
+ virtual void Shutdown() = 0;
+
+ // returns a handle to the main module panel
+ virtual vgui2::VPANEL GetPanel() = 0;
+
+ // sets the parent of the main module panel
+ virtual void SetParent(vgui2::VPANEL parent) = 0;
+
+ // messages sent through through the panel returned by GetPanel():
+ //
+ // "ConnectedToGame" "ip" "port" "gamedir"
+ // "DisconnectedFromGame"
+ // "ActiveGameName" "name"
+ // "LoadingStarted" "type" "name"
+ // "LoadingFinished" "type" "name"
+};
+
+#define VGUIMODULE_INTERFACE_VERSION "VGuiModuleAdminServer001"
diff --git a/dep/hlsdk/common/ObjectDictionary.cpp b/dep/hlsdk/common/ObjectDictionary.cpp
new file mode 100644
index 0000000..84a0f88
--- /dev/null
+++ b/dep/hlsdk/common/ObjectDictionary.cpp
@@ -0,0 +1,515 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#include "precompiled.h"
+
+ObjectDictionary::ObjectDictionary()
+{
+ m_currentEntry = 0;
+ m_findKey = 0;
+ m_entries = nullptr;
+
+ Q_memset(m_cache, 0, sizeof(m_cache));
+
+ m_cacheIndex = 0;
+ m_size = 0;
+ m_maxSize = 0;
+}
+
+ObjectDictionary::~ObjectDictionary()
+{
+ if (m_entries) {
+ Mem_Free(m_entries);
+ }
+}
+
+void ObjectDictionary::Clear(bool freeObjectssMemory)
+{
+ if (freeObjectssMemory)
+ {
+ for (int i = 0; i < m_size; i++)
+ {
+ void *obj = m_entries[i].object;
+ if (obj) {
+ Mem_Free(obj);
+ }
+ }
+ }
+
+ m_size = 0;
+ CheckSize();
+ ClearCache();
+}
+
+bool ObjectDictionary::Add(void *object, float key)
+{
+ if (m_size == m_maxSize && !CheckSize())
+ return false;
+
+ entry_t *p;
+ if (m_size && key < m_entries[m_size - 1].key)
+ {
+ p = &m_entries[FindClosestAsIndex(key)];
+
+ entry_t *e1 = &m_entries[m_size];
+ entry_t *e2 = &m_entries[m_size - 1];
+
+ while (p->key <= key) { p++; }
+ while (p != e1)
+ {
+ e1->object = e2->object;
+ e1->key = e2->key;
+
+ e1--;
+ e2--;
+ }
+ }
+ else
+ p = &m_entries[m_size];
+
+ p->key = key;
+ p->object = object;
+ m_size++;
+
+ ClearCache();
+ AddToCache(p);
+
+ return true;
+}
+
+int ObjectDictionary::FindClosestAsIndex(float key)
+{
+ if (m_size <= 0)
+ return -1;
+
+ if (key <= m_entries->key)
+ return 0;
+
+ int index = FindKeyInCache(key);
+ if (index >= 0) {
+ return index;
+ }
+
+ int middle;
+ int first = 0;
+ int last = m_size - 1;
+ float keyMiddle, keyNext;
+
+ if (key < m_entries[last].key)
+ {
+ while (true)
+ {
+ middle = (last + first) >> 1;
+ keyMiddle = m_entries[middle].key;
+
+ if (keyMiddle == key)
+ break;
+
+ if (keyMiddle < key)
+ {
+ if (m_entries[middle + 1].key >= key)
+ {
+ if (m_entries[middle + 1].key - key < key - keyMiddle)
+ ++middle;
+ break;
+ }
+
+ first = (last + first) >> 1;
+ }
+ else
+ {
+ last = (last + first) >> 1;
+ }
+ }
+ }
+ else
+ {
+ middle = last;
+ }
+
+ keyNext = m_entries[middle - 1].key;
+ while (keyNext == key) {
+ keyNext = m_entries[middle--].key;
+ }
+
+ AddToCache(&m_entries[middle], key);
+ return middle;
+}
+
+void ObjectDictionary::ClearCache()
+{
+ Q_memset(m_cache, 0, sizeof(m_cache));
+ m_cacheIndex = 0;
+}
+
+bool ObjectDictionary::RemoveIndex(int index, bool freeObjectMemory)
+{
+ if (index < 0 || index >= m_size)
+ return false;
+
+ entry_t *p = &m_entries[m_size - 1];
+ entry_t *e1 = &m_entries[index];
+ entry_t *e2 = &m_entries[index + 1];
+
+ if (freeObjectMemory && e1->object)
+ Mem_Free(e1->object);
+
+ while (p != e1)
+ {
+ e1->object = e2->object;
+ e1->key = e2->key;
+
+ e1++;
+ e2++;
+ }
+
+ p->object = nullptr;
+ p->key = 0;
+ m_size--;
+
+ CheckSize();
+ ClearCache();
+
+ return false;
+}
+
+bool ObjectDictionary::RemoveIndexRange(int minIndex, int maxIndex)
+{
+ if (minIndex > maxIndex)
+ {
+ if (maxIndex < 0)
+ maxIndex = 0;
+
+ if (minIndex >= m_size)
+ minIndex = m_size - 1;
+ }
+ else
+ {
+ if (minIndex < 0)
+ minIndex = 0;
+
+ if (maxIndex >= m_size)
+ maxIndex = m_size - 1;
+ }
+
+ int offset = minIndex + maxIndex - 1;
+ m_size -= offset;
+ CheckSize();
+ return true;
+}
+
+bool ObjectDictionary::Remove(void *object)
+{
+ bool found = false;
+ for (int i = 0; i < m_size; i++)
+ {
+ if (m_entries[i].object == object) {
+ RemoveIndex(i);
+ found = true;
+ }
+ }
+
+ return found ? true : false;
+}
+
+bool ObjectDictionary::RemoveSingle(void *object)
+{
+ for (int i = 0; i < m_size; i++)
+ {
+ if (m_entries[i].object == object) {
+ RemoveIndex(i);
+ return true;
+ }
+ }
+
+ return false;
+}
+
+bool ObjectDictionary::RemoveKey(float key)
+{
+ int i = FindClosestAsIndex(key);
+ if (m_entries[i].key == key)
+ {
+ int j = i;
+ do {
+ ++j;
+ }
+ while (key == m_entries[j + 1].key);
+
+ return RemoveIndexRange(i, j);
+ }
+
+ return false;
+}
+
+bool ObjectDictionary::CheckSize()
+{
+ int newSize = m_maxSize;
+ if (m_size == m_maxSize)
+ {
+ newSize = 1 - (int)(m_maxSize * -1.25f);
+ }
+ else if (m_maxSize * 0.5f > m_size)
+ {
+ newSize = (int)(m_maxSize * 0.75f);
+ }
+
+ if (newSize != m_maxSize)
+ {
+ entry_t *newEntries = (entry_t *)Mem_Malloc(sizeof(entry_t) * newSize);
+ if (!newEntries)
+ return false;
+
+ Q_memset(&newEntries[m_size], 0, sizeof(entry_t) * (newSize - m_size));
+
+ if (m_entries && m_size)
+ {
+ Q_memcpy(newEntries, m_entries, sizeof(entry_t) * m_size);
+ Mem_Free(m_entries);
+ }
+
+ m_entries = newEntries;
+ m_maxSize = newSize;
+ }
+
+ return true;
+}
+
+void ObjectDictionary::Init()
+{
+ m_size = 0;
+ m_maxSize = 0;
+ m_entries = nullptr;
+
+ CheckSize();
+ ClearCache();
+}
+
+void ObjectDictionary::Init(int baseSize)
+{
+ m_size = 0;
+ m_maxSize = 0;
+ m_entries = (entry_t *)Mem_ZeroMalloc(sizeof(entry_t) * baseSize);
+
+ if (m_entries) {
+ m_maxSize = baseSize;
+ }
+}
+
+bool ObjectDictionary::Add(void *object)
+{
+ return Add(object, 0);
+}
+
+int ObjectDictionary::CountElements()
+{
+ return m_size;
+}
+
+bool ObjectDictionary::IsEmpty()
+{
+ return (m_size == 0) ? true : false;
+}
+
+bool ObjectDictionary::Contains(void *object)
+{
+ if (FindObjectInCache(object) >= 0)
+ return true;
+
+ for (int i = 0; i < m_size; i++)
+ {
+ entry_t *e = &m_entries[i];
+ if (e->object == object) {
+ AddToCache(e);
+ return true;
+ }
+ }
+
+ return false;
+}
+
+void *ObjectDictionary::GetFirst()
+{
+ m_currentEntry = 0;
+ return GetNext();
+}
+
+void *ObjectDictionary::GetLast()
+{
+ return (m_size > 0) ? m_entries[m_size - 1].object : nullptr;
+}
+
+bool ObjectDictionary::ChangeKey(void *object, float newKey)
+{
+ int pos = FindObjectInCache(object);
+ if (pos < 0)
+ {
+ for (pos = 0; pos < m_size; pos++)
+ {
+ if (m_entries[pos].object == object) {
+ AddToCache(&m_entries[pos]);
+ break;
+ }
+ }
+
+ if (pos == m_size) {
+ return false;
+ }
+ }
+
+ entry_t *p, *e;
+
+ p = &m_entries[pos];
+ if (p->key == newKey)
+ return false;
+
+ int newpos = FindClosestAsIndex(newKey);
+ e = &m_entries[newpos];
+ if (pos < newpos)
+ {
+ if (e->key > newKey)
+ e--;
+
+ entry_t *e2 = &m_entries[pos + 1];
+ while (p < e)
+ {
+ p->object = e2->object;
+ p->key = e2->key;
+
+ p++;
+ e2++;
+ }
+ }
+ else if (pos > newpos)
+ {
+ if (e->key > newKey)
+ e++;
+
+ entry_t *e2 = &m_entries[pos - 1];
+ while (p > e)
+ {
+ p->object = e2->object;
+ p->key = e2->key;
+
+ p--;
+ e2--;
+ }
+ }
+
+ p->object = object;
+ p->key = newKey;
+ ClearCache();
+
+ return true;
+}
+
+bool ObjectDictionary::UnsafeChangeKey(void *object, float newKey)
+{
+ int pos = FindObjectInCache(object);
+ if (pos < 0)
+ {
+ for (pos = 0; pos < m_size; pos++)
+ {
+ if (m_entries[pos].object == object) {
+ break;
+ }
+ }
+
+ if (pos == m_size) {
+ return false;
+ }
+ }
+
+ m_entries[pos].key = newKey;
+ ClearCache();
+ return true;
+}
+
+void ObjectDictionary::AddToCache(entry_t *entry)
+{
+ int i = (m_cacheIndex % MAX_OBJECT_CACHE);
+
+ m_cache[i].object = entry;
+ m_cache[i].key = entry->key;
+ m_cacheIndex++;
+}
+
+void ObjectDictionary::AddToCache(entry_t *entry, float key)
+{
+ int i = (m_cacheIndex % MAX_OBJECT_CACHE);
+
+ m_cache[i].object = entry;
+ m_cache[i].key = key;
+ m_cacheIndex++;
+}
+
+int ObjectDictionary::FindKeyInCache(float key)
+{
+ for (auto& ch : m_cache)
+ {
+ if (ch.object && ch.key == key) {
+ return (entry_t *)ch.object - m_entries;
+ }
+ }
+
+ return -1;
+}
+
+int ObjectDictionary::FindObjectInCache(void *object)
+{
+ for (auto& ch : m_cache)
+ {
+ if (ch.object && ch.object == object) {
+ return (entry_t *)ch.object - m_entries;
+ }
+ }
+
+ return -1;
+}
+
+void *ObjectDictionary::FindClosestKey(float key)
+{
+ m_currentEntry = FindClosestAsIndex(key);
+ return GetNext();
+}
+
+void *ObjectDictionary::GetNext()
+{
+ if (m_currentEntry < 0 || m_currentEntry >= m_size)
+ return nullptr;
+
+ return m_entries[m_currentEntry++].object;
+}
+
+void *ObjectDictionary::FindExactKey(float key)
+{
+ if ((m_currentEntry = FindClosestAsIndex(key)) < 0)
+ return nullptr;
+
+ return (m_entries[m_currentEntry].key == key) ? GetNext() : nullptr;
+}
diff --git a/dep/hlsdk/common/ObjectDictionary.h b/dep/hlsdk/common/ObjectDictionary.h
new file mode 100644
index 0000000..bf1b77f
--- /dev/null
+++ b/dep/hlsdk/common/ObjectDictionary.h
@@ -0,0 +1,94 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "IObjectContainer.h"
+
+class ObjectDictionary: public IObjectContainer {
+public:
+ ObjectDictionary();
+ virtual ~ObjectDictionary();
+
+ void Init();
+ void Init(int baseSize);
+
+ bool Add(void *object);
+ bool Contains(void *object);
+ bool IsEmpty();
+ int CountElements();
+
+ void Clear(bool freeObjectssMemory = false);
+
+ bool Add(void *object, float key);
+ bool ChangeKey(void *object, float newKey);
+ bool UnsafeChangeKey(void *object, float newKey);
+
+ bool Remove(void *object);
+ bool RemoveSingle(void *object);
+ bool RemoveKey(float key);
+ bool RemoveRange(float startKey, float endKey);
+
+ void *FindClosestKey(float key);
+ void *FindExactKey(float key);
+
+ void *GetFirst();
+ void *GetLast();
+ void *GetNext();
+
+ int FindKeyInCache(float key);
+ int FindObjectInCache(void *object);
+
+ void ClearCache();
+ bool CheckSize();
+
+ typedef struct entry_s {
+ void *object;
+ float key;
+ } entry_t;
+
+ void AddToCache(entry_t *entry);
+ void AddToCache(entry_t *entry, float key);
+
+ bool RemoveIndex(int index, bool freeObjectMemory = false);
+ bool RemoveIndexRange(int minIndex, int maxIndex);
+ int FindClosestAsIndex(float key);
+
+protected:
+ int m_currentEntry;
+ float m_findKey;
+
+ enum { MAX_OBJECT_CACHE = 32 };
+
+ entry_t *m_entries;
+ entry_t m_cache[MAX_OBJECT_CACHE];
+
+ int m_cacheIndex;
+ int m_size;
+ int m_maxSize;
+};
diff --git a/dep/hlsdk/common/ObjectList.cpp b/dep/hlsdk/common/ObjectList.cpp
new file mode 100644
index 0000000..4518090
--- /dev/null
+++ b/dep/hlsdk/common/ObjectList.cpp
@@ -0,0 +1,259 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#include "precompiled.h"
+
+ObjectList::ObjectList()
+{
+ m_head = m_tail = m_current = nullptr;
+ m_number = 0;
+}
+
+ObjectList::~ObjectList()
+{
+ Clear(false);
+}
+
+bool ObjectList::AddHead(void *newObject)
+{
+ // create new element
+ element_t *newElement = (element_t *)Mem_ZeroMalloc(sizeof(element_t));
+
+ // out of memory
+ if (!newElement)
+ return false;
+
+ // insert element
+ newElement->object = newObject;
+
+ if (m_head)
+ {
+ newElement->next = m_head;
+ m_head->prev = newElement;
+ }
+
+ m_head = newElement;
+
+ // if list was empty set new m_tail
+ if (!m_tail)
+ m_tail = m_head;
+
+ m_number++;
+ return true;
+}
+
+void *ObjectList::RemoveHead()
+{
+ void *retObj;
+
+ // check m_head is present
+ if (m_head)
+ {
+ retObj = m_head->object;
+ element_t *newHead = m_head->next;
+ if (newHead)
+ newHead->prev = nullptr;
+
+ // if only one element is in list also update m_tail
+ // if we remove this prev element
+ if (m_tail == m_head)
+ m_tail = nullptr;
+
+ Mem_Free(m_head);
+ m_head = newHead;
+
+ m_number--;
+ }
+ else
+ retObj = nullptr;
+
+ return retObj;
+}
+
+bool ObjectList::AddTail(void *newObject)
+{
+ // create new element
+ element_t *newElement = (element_t *)Mem_ZeroMalloc(sizeof(element_t));
+
+ // out of memory
+ if (!newElement)
+ return false;
+
+ // insert element
+ newElement->object = newObject;
+
+ if (m_tail)
+ {
+ newElement->prev = m_tail;
+ m_tail->next = newElement;
+ }
+
+ m_tail = newElement;
+
+ // if list was empty set new m_tail
+ if (!m_head)
+ m_head = m_tail;
+
+ m_number++;
+ return true;
+}
+
+void *ObjectList::RemoveTail()
+{
+ void *retObj;
+
+ // check m_tail is present
+ if (m_tail)
+ {
+ retObj = m_tail->object;
+ element_t *newTail = m_tail->prev;
+ if (newTail)
+ newTail->next = nullptr;
+
+ // if only one element is in list also update m_tail
+ // if we remove this prev element
+ if (m_head == m_tail)
+ m_head = nullptr;
+
+ Mem_Free(m_tail);
+ m_tail = newTail;
+
+ m_number--;
+
+ }
+ else
+ retObj = nullptr;
+
+ return retObj;
+}
+
+bool ObjectList::IsEmpty()
+{
+ return (m_head == nullptr);
+}
+
+int ObjectList::CountElements()
+{
+ return m_number;
+}
+
+bool ObjectList::Contains(void *object)
+{
+ element_t *e = m_head;
+
+ while (e && e->object != object) { e = e->next; }
+
+ if (e)
+ {
+ m_current = e;
+ return true;
+ }
+ else
+ {
+ return false;
+ }
+}
+
+void ObjectList::Clear(bool freeElementsMemory)
+{
+ element_t *ne;
+ element_t *e = m_head;
+
+ while (e)
+ {
+ ne = e->next;
+
+ if (freeElementsMemory && e->object)
+ Mem_Free(e->object);
+
+ Mem_Free(e);
+ e = ne;
+ }
+
+ m_head = m_tail = m_current = nullptr;
+ m_number = 0;
+}
+
+bool ObjectList::Remove(void *object)
+{
+ element_t *e = m_head;
+
+ while (e && e->object != object) { e = e->next; }
+
+ if (e)
+ {
+ if (e->prev) e->prev->next = e->next;
+ if (e->next) e->next->prev = e->prev;
+
+ if (m_head == e) m_head = e->next;
+ if (m_tail == e) m_tail = e->prev;
+ if (m_current == e) m_current= e->next;
+
+ Mem_Free(e);
+ m_number--;
+ }
+
+ return (e != nullptr);
+}
+
+void ObjectList::Init()
+{
+ m_head = m_tail = m_current = nullptr;
+ m_number = 0;
+}
+
+void *ObjectList::GetFirst()
+{
+ if (m_head)
+ {
+ m_current = m_head->next;
+ return m_head->object;
+ }
+ else
+ {
+ m_current = nullptr;
+ return nullptr;
+ }
+}
+
+void *ObjectList::GetNext()
+{
+ void *retObj = nullptr;
+ if (m_current)
+ {
+ retObj = m_current->object;
+ m_current = m_current->next;
+ }
+
+ return retObj;
+}
+
+bool ObjectList::Add(void *newObject)
+{
+ return AddTail(newObject);
+}
diff --git a/dep/hlsdk/common/ObjectList.h b/dep/hlsdk/common/ObjectList.h
new file mode 100644
index 0000000..aa023f8
--- /dev/null
+++ b/dep/hlsdk/common/ObjectList.h
@@ -0,0 +1,65 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "IObjectContainer.h"
+
+class ObjectList: public IObjectContainer {
+public:
+ EXT_FUNC void Init();
+ EXT_FUNC bool Add(void *newObject);
+ EXT_FUNC void *GetFirst();
+ EXT_FUNC void *GetNext();
+
+ ObjectList();
+ virtual ~ObjectList();
+
+ EXT_FUNC void Clear(bool freeElementsMemory = false);
+ EXT_FUNC int CountElements();
+ void *RemoveTail();
+ void *RemoveHead();
+
+ bool AddTail(void *newObject);
+ bool AddHead(void *newObject);
+ EXT_FUNC bool Remove(void *object);
+ EXT_FUNC bool Contains(void *object);
+ EXT_FUNC bool IsEmpty();
+
+ typedef struct element_s {
+ struct element_s *prev; // pointer to the last element or NULL
+ struct element_s *next; // pointer to the next elemnet or NULL
+ void *object; // the element's object
+ } element_t;
+
+protected:
+ element_t *m_head; // first element in list
+ element_t *m_tail; // last element in list
+ element_t *m_current; // current element in list
+ int m_number;
+};
diff --git a/dep/hlsdk/common/Sequence.h b/dep/hlsdk/common/Sequence.h
new file mode 100644
index 0000000..8df553d
--- /dev/null
+++ b/dep/hlsdk/common/Sequence.h
@@ -0,0 +1,201 @@
+//---------------------------------------------------------------------------
+//
+// S c r i p t e d S e q u e n c e s
+//
+//---------------------------------------------------------------------------
+#ifndef _INCLUDE_SEQUENCE_H_
+#define _INCLUDE_SEQUENCE_H_
+
+
+#ifndef _DEF_BYTE_
+typedef unsigned char byte;
+#endif
+
+//---------------------------------------------------------------------------
+// client_textmessage_t
+//---------------------------------------------------------------------------
+typedef struct client_textmessage_s
+{
+ int effect;
+ byte r1, g1, b1, a1; // 2 colors for effects
+ byte r2, g2, b2, a2;
+ float x;
+ float y;
+ float fadein;
+ float fadeout;
+ float holdtime;
+ float fxtime;
+ const char *pName;
+ const char *pMessage;
+} client_textmessage_t;
+
+
+//--------------------------------------------------------------------------
+// sequenceDefaultBits_e
+//
+// Enumerated list of possible modifiers for a command. This enumeration
+// is used in a bitarray controlling what modifiers are specified for a command.
+//---------------------------------------------------------------------------
+enum sequenceModifierBits
+{
+ SEQUENCE_MODIFIER_EFFECT_BIT = (1 << 1),
+ SEQUENCE_MODIFIER_POSITION_BIT = (1 << 2),
+ SEQUENCE_MODIFIER_COLOR_BIT = (1 << 3),
+ SEQUENCE_MODIFIER_COLOR2_BIT = (1 << 4),
+ SEQUENCE_MODIFIER_FADEIN_BIT = (1 << 5),
+ SEQUENCE_MODIFIER_FADEOUT_BIT = (1 << 6),
+ SEQUENCE_MODIFIER_HOLDTIME_BIT = (1 << 7),
+ SEQUENCE_MODIFIER_FXTIME_BIT = (1 << 8),
+ SEQUENCE_MODIFIER_SPEAKER_BIT = (1 << 9),
+ SEQUENCE_MODIFIER_LISTENER_BIT = (1 << 10),
+ SEQUENCE_MODIFIER_TEXTCHANNEL_BIT = (1 << 11),
+};
+typedef enum sequenceModifierBits sequenceModifierBits_e ;
+
+
+//---------------------------------------------------------------------------
+// sequenceCommandEnum_e
+//
+// Enumerated sequence command types.
+//---------------------------------------------------------------------------
+enum sequenceCommandEnum_
+{
+ SEQUENCE_COMMAND_ERROR = -1,
+ SEQUENCE_COMMAND_PAUSE = 0,
+ SEQUENCE_COMMAND_FIRETARGETS,
+ SEQUENCE_COMMAND_KILLTARGETS,
+ SEQUENCE_COMMAND_TEXT,
+ SEQUENCE_COMMAND_SOUND,
+ SEQUENCE_COMMAND_GOSUB,
+ SEQUENCE_COMMAND_SENTENCE,
+ SEQUENCE_COMMAND_REPEAT,
+ SEQUENCE_COMMAND_SETDEFAULTS,
+ SEQUENCE_COMMAND_MODIFIER,
+ SEQUENCE_COMMAND_POSTMODIFIER,
+ SEQUENCE_COMMAND_NOOP,
+
+ SEQUENCE_MODIFIER_EFFECT,
+ SEQUENCE_MODIFIER_POSITION,
+ SEQUENCE_MODIFIER_COLOR,
+ SEQUENCE_MODIFIER_COLOR2,
+ SEQUENCE_MODIFIER_FADEIN,
+ SEQUENCE_MODIFIER_FADEOUT,
+ SEQUENCE_MODIFIER_HOLDTIME,
+ SEQUENCE_MODIFIER_FXTIME,
+ SEQUENCE_MODIFIER_SPEAKER,
+ SEQUENCE_MODIFIER_LISTENER,
+ SEQUENCE_MODIFIER_TEXTCHANNEL,
+};
+typedef enum sequenceCommandEnum_ sequenceCommandEnum_e;
+
+
+//---------------------------------------------------------------------------
+// sequenceCommandType_e
+//
+// Typeerated sequence command types.
+//---------------------------------------------------------------------------
+enum sequenceCommandType_
+{
+ SEQUENCE_TYPE_COMMAND,
+ SEQUENCE_TYPE_MODIFIER,
+};
+typedef enum sequenceCommandType_ sequenceCommandType_e;
+
+
+//---------------------------------------------------------------------------
+// sequenceCommandMapping_s
+//
+// A mapping of a command enumerated-value to its name.
+//---------------------------------------------------------------------------
+typedef struct sequenceCommandMapping_ sequenceCommandMapping_s;
+struct sequenceCommandMapping_
+{
+ sequenceCommandEnum_e commandEnum;
+ const char* commandName;
+ sequenceCommandType_e commandType;
+};
+
+
+//---------------------------------------------------------------------------
+// sequenceCommandLine_s
+//
+// Structure representing a single command (usually 1 line) from a
+// .SEQ file entry.
+//---------------------------------------------------------------------------
+typedef struct sequenceCommandLine_ sequenceCommandLine_s;
+struct sequenceCommandLine_
+{
+ int commandType; // Specifies the type of command
+ client_textmessage_t clientMessage; // Text HUD message struct
+ char* speakerName; // Targetname of speaking entity
+ char* listenerName; // Targetname of entity being spoken to
+ char* soundFileName; // Name of sound file to play
+ char* sentenceName; // Name of sentences.txt to play
+ char* fireTargetNames; // List of targetnames to fire
+ char* killTargetNames; // List of targetnames to remove
+ float delay; // Seconds 'till next command
+ int repeatCount; // If nonzero, reset execution pointer to top of block (N times, -1 = infinite)
+ int textChannel; // Display channel on which text message is sent
+ int modifierBitField; // Bit field to specify what clientmessage fields are valid
+ sequenceCommandLine_s* nextCommandLine; // Next command (linked list)
+};
+
+
+//---------------------------------------------------------------------------
+// sequenceEntry_s
+//
+// Structure representing a single command (usually 1 line) from a
+// .SEQ file entry.
+//---------------------------------------------------------------------------
+typedef struct sequenceEntry_ sequenceEntry_s;
+struct sequenceEntry_
+{
+ char* fileName; // Name of sequence file without .SEQ extension
+ char* entryName; // Name of entry label in file
+ sequenceCommandLine_s* firstCommand; // Linked list of commands in entry
+ sequenceEntry_s* nextEntry; // Next loaded entry
+ qboolean isGlobal; // Is entry retained over level transitions?
+};
+
+
+
+//---------------------------------------------------------------------------
+// sentenceEntry_s
+// Structure representing a single sentence of a group from a .SEQ
+// file entry. Sentences are identical to entries in sentences.txt, but
+// can be unique per level and are loaded/unloaded with the level.
+//---------------------------------------------------------------------------
+typedef struct sentenceEntry_ sentenceEntry_s;
+struct sentenceEntry_
+{
+ char* data; // sentence data (ie "We have hostiles" )
+ sentenceEntry_s* nextEntry; // Next loaded entry
+ qboolean isGlobal; // Is entry retained over level transitions?
+ unsigned int index; // this entry's position in the file.
+};
+
+//--------------------------------------------------------------------------
+// sentenceGroupEntry_s
+// Structure representing a group of sentences found in a .SEQ file.
+// A sentence group is defined by all sentences with the same name, ignoring
+// the number at the end of the sentence name. Groups enable a sentence
+// to be picked at random across a group.
+//--------------------------------------------------------------------------
+typedef struct sentenceGroupEntry_ sentenceGroupEntry_s;
+struct sentenceGroupEntry_
+{
+ char* groupName; // name of the group (ie CT_ALERT )
+ unsigned int numSentences; // number of sentences in group
+ sentenceEntry_s* firstSentence; // head of linked list of sentences in group
+ sentenceGroupEntry_s* nextEntry; // next loaded group
+};
+
+//---------------------------------------------------------------------------
+// Function declarations
+//---------------------------------------------------------------------------
+sequenceEntry_s* SequenceGet( const char* fileName, const char* entryName );
+void Sequence_ParseFile( const char* fileName, qboolean isGlobal );
+void Sequence_OnLevelLoad( const char* mapName );
+sentenceEntry_s* SequencePickSentence( const char *groupName, int pickMethod, int *picked );
+
+#endif // _INCLUDE_SEQUENCE_H_
diff --git a/dep/hlsdk/common/SteamAppStartUp.cpp b/dep/hlsdk/common/SteamAppStartUp.cpp
new file mode 100644
index 0000000..7f13f4b
--- /dev/null
+++ b/dep/hlsdk/common/SteamAppStartUp.cpp
@@ -0,0 +1,211 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#include "precompiled.h"
+
+#ifdef _WIN32
+#include "SteamAppStartup.h"
+
+#define WIN32_LEAN_AND_MEAN
+#include
+#include
+#include
+#include
+#include
+#include
+
+#define STEAM_PARM "-steam"
+
+bool FileExists(const char *fileName)
+{
+ struct _stat statbuf;
+ return (_stat(fileName, &statbuf) == 0);
+}
+
+// Handles launching the game indirectly via steam
+void LaunchSelfViaSteam(const char *params)
+{
+ // calculate the details of our launch
+ char appPath[MAX_PATH];
+ ::GetModuleFileName((HINSTANCE)GetModuleHandle(NULL), appPath, sizeof(appPath));
+
+ // strip out the exe name
+ char *slash = Q_strrchr(appPath, '\\');
+ if (slash)
+ {
+ *slash = '\0';
+ }
+
+ // save out our details to the registry
+ HKEY hKey;
+ if (ERROR_SUCCESS == RegOpenKey(HKEY_CURRENT_USER, "Software\\Valve\\Steam", &hKey))
+ {
+ DWORD dwType = REG_SZ;
+ DWORD dwSize = static_cast(Q_strlen(appPath) + 1);
+ RegSetValueEx(hKey, "TempAppPath", NULL, dwType, (LPBYTE)appPath, dwSize);
+ dwSize = static_cast(Q_strlen(params) + 1);
+ RegSetValueEx(hKey, "TempAppCmdLine", NULL, dwType, (LPBYTE)params, dwSize);
+ // clear out the appID (since we don't know it yet)
+ dwType = REG_DWORD;
+ int appID = -1;
+ RegSetValueEx(hKey, "TempAppID", NULL, dwType, (LPBYTE)&appID, sizeof(appID));
+ RegCloseKey(hKey);
+ }
+
+ // search for an active steam instance
+ HWND hwnd = ::FindWindow("Valve_SteamIPC_Class", "Hidden Window");
+ if (hwnd)
+ {
+ ::PostMessage(hwnd, WM_USER + 3, 0, 0);
+ }
+ else
+ {
+ // couldn't find steam, find and launch it
+
+ // first, search backwards through our current set of directories
+ char steamExe[MAX_PATH] = "";
+ char dir[MAX_PATH];
+
+ if (::GetCurrentDirectoryA(sizeof(dir), dir))
+ {
+ char *slash = Q_strrchr(dir, '\\');
+ while (slash)
+ {
+ // see if steam_dev.exe is in the directory first
+ slash[1] = '\0';
+ Q_strcat(slash, "steam_dev.exe");
+ FILE *f = fopen(dir, "rb");
+ if (f)
+ {
+ // found it
+ fclose(f);
+ Q_strcpy(steamExe, dir);
+ break;
+ }
+
+ // see if steam.exe is in the directory
+ slash[1] = '\0';
+ Q_strcat(slash, "steam.exe");
+ f = fopen(dir, "rb");
+ if (f)
+ {
+ // found it
+ fclose(f);
+ Q_strcpy(steamExe, dir);
+ break;
+ }
+
+ // kill the string at the slash
+ slash[0] = '\0';
+
+ // move to the previous slash
+ slash = Q_strrchr(dir, '\\');
+ }
+ }
+
+ if (!steamExe[0])
+ {
+ // still not found, use the one in the registry
+ HKEY hKey;
+ if (ERROR_SUCCESS == RegOpenKey(HKEY_CURRENT_USER, "Software\\Valve\\Steam", &hKey))
+ {
+ DWORD dwType;
+ DWORD dwSize = sizeof(steamExe);
+ RegQueryValueEx(hKey, "SteamExe", NULL, &dwType, (LPBYTE)steamExe, &dwSize);
+ RegCloseKey(hKey);
+ }
+ }
+
+ if (!steamExe[0])
+ {
+ // still no path, error
+ ::MessageBox(NULL, "Error running game: could not find steam.exe to launch", "Fatal Error", MB_OK | MB_ICONERROR);
+ return;
+ }
+
+ // fix any slashes
+ for (char *slash = steamExe; *slash; slash++)
+ {
+ if (*slash == '/')
+ {
+ *slash = '\\';
+ }
+ }
+
+ // change to the steam directory
+ Q_strcpy(dir, steamExe);
+ char *delimiter = Q_strrchr(dir, '\\');
+ if (delimiter)
+ {
+ *delimiter = '\0';
+ _chdir(dir);
+ }
+
+ // exec steam.exe, in silent mode, with the launch app param
+ char *args[4] = { steamExe, "-silent", "-applaunch", '\0' };
+ _spawnv(_P_NOWAIT, steamExe, args);
+ }
+}
+
+// Launches steam if necessary
+bool ShouldLaunchAppViaSteam(const char *lpCmdLine, const char *steamFilesystemDllName, const char *stdioFilesystemDllName)
+{
+ // see if steam is on the command line
+ const char *steamStr = Q_strstr(lpCmdLine, STEAM_PARM);
+
+ // check the character following it is a whitespace or null
+ if (steamStr)
+ {
+ const char *postChar = steamStr + Q_strlen(STEAM_PARM);
+ if (*postChar == 0 || isspace(*postChar))
+ {
+ // we're running under steam already, let the app continue
+ return false;
+ }
+ }
+
+ // we're not running under steam, see which filesystems are available
+ if (FileExists(stdioFilesystemDllName))
+ {
+ // we're being run with a stdio filesystem, so we can continue without steam
+ return false;
+ }
+
+ // make sure we have a steam filesystem available
+ if (!FileExists(steamFilesystemDllName))
+ {
+ return false;
+ }
+
+ // we have the steam filesystem, and no stdio filesystem, so we must need to be run under steam
+ // launch steam
+ LaunchSelfViaSteam(lpCmdLine);
+ return true;
+}
+
+#endif // _WIN32
diff --git a/dep/hlsdk/common/SteamAppStartUp.h b/dep/hlsdk/common/SteamAppStartUp.h
new file mode 100644
index 0000000..2b9fbe7
--- /dev/null
+++ b/dep/hlsdk/common/SteamAppStartUp.h
@@ -0,0 +1,37 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+// Call this first thing at startup
+// Works out if the app is a steam app that is being ran outside of steam,
+// and if so, launches steam and tells it to run us as a steam app
+//
+// if it returns true, then exit
+// if it ruturns false, then continue with normal startup
+bool ShouldLaunchAppViaSteam(const char *cmdLine, const char *steamFilesystemDllName, const char *stdioFilesystemDllName);
diff --git a/dep/hlsdk/common/SteamCommon.h b/dep/hlsdk/common/SteamCommon.h
new file mode 100644
index 0000000..c3fb1b0
--- /dev/null
+++ b/dep/hlsdk/common/SteamCommon.h
@@ -0,0 +1,707 @@
+
+//========= Copyright Valve Corporation, All rights reserved. ============//
+/*
+** The copyright to the contents herein is the property of Valve Corporation.
+** The contents may be used and/or copied only with the written permission of
+** Valve, or in accordance with the terms and conditions stipulated in
+** the agreement/contract under which the contents have been supplied.
+**
+*******************************************************************************
+**
+** Contents:
+**
+** Common types used in the Steam DLL interface.
+**
+** This file is distributed to Steam application developers.
+**
+**
+**
+*******************************************************************************/
+
+#ifndef INCLUDED_STEAM_COMMON_STEAMCOMMON_H
+#define INCLUDED_STEAM_COMMON_STEAMCOMMON_H
+
+#if defined(_MSC_VER) && (_MSC_VER > 1000)
+#pragma once
+#endif
+
+
+#ifdef __cplusplus
+extern "C"
+{
+#endif
+
+/* Applications should not define STEAM_EXPORTS. */
+
+#if defined ( _WIN32 )
+
+#ifdef STEAM_EXPORTS
+#define STEAM_API __declspec(dllexport) EXT_FUNC
+#else
+#define STEAM_API __declspec(dllimport)
+#endif
+
+#define STEAM_CALL __cdecl
+
+#else
+
+#ifdef STEAM_EXPORTS
+#define STEAM_API EXT_FUNC
+#else
+#define STEAM_API /* */
+#endif
+#define STEAM_CALL /* */
+
+#endif
+
+typedef void (STEAM_CALL *KeyValueIteratorCallback_t )(const char *Key, const char *Val, void *pvParam);
+
+
+/******************************************************************************
+**
+** Exported macros and constants
+**
+******************************************************************************/
+
+/* DEPRECATED -- these are ignored now, all API access is granted on SteamStartup */
+#define STEAM_USING_FILESYSTEM (0x00000001)
+#define STEAM_USING_LOGGING (0x00000002)
+#define STEAM_USING_USERID (0x00000004)
+#define STEAM_USING_ACCOUNT (0x00000008)
+#define STEAM_USING_ALL (0x0000000f)
+/* END DEPRECATED */
+
+#define STEAM_MAX_PATH (255)
+#define STEAM_QUESTION_MAXLEN (255)
+#define STEAM_SALT_SIZE (8)
+#define STEAM_PROGRESS_PERCENT_SCALE (0x00001000)
+
+/* These are maximum significant string lengths, excluding nul-terminator. */
+#define STEAM_CARD_NUMBER_SIZE (17)
+#define STEAM_CARD_HOLDERNAME_SIZE (100)
+#define STEAM_CARD_EXPYEAR_SIZE (4)
+#define STEAM_CARD_EXPMONTH_SIZE (2)
+#define STEAM_CARD_CVV2_SIZE (5)
+#define STEAM_BILLING_ADDRESS1_SIZE (128)
+#define STEAM_BILLING_ADDRESS2_SIZE (128)
+#define STEAM_BILLING_CITY_SIZE (50)
+#define STEAM_BILLING_ZIP_SIZE (16)
+#define STEAM_BILLING_STATE_SIZE (32)
+#define STEAM_BILLING_COUNTRY_SIZE (32)
+#define STEAM_BILLING_PHONE_SIZE (20)
+#define STEAM_BILLING_EMAIL_ADDRESS_SIZE (100)
+#define STEAM_TYPE_OF_PROOF_OF_PURCHASE_SIZE (20)
+#define STEAM_PROOF_OF_PURCHASE_TOKEN_SIZE (200)
+#define STEAM_EXTERNAL_ACCOUNTNAME_SIZE (100)
+#define STEAM_EXTERNAL_ACCOUNTPASSWORD_SIZE (80)
+#define STEAM_BILLING_CONFIRMATION_CODE_SIZE (22)
+#define STEAM_BILLING_CARD_APPROVAL_CODE_SIZE (100)
+#define STEAM_BILLING_TRANS_DATE_SIZE (9) // mm/dd/yy
+#define STEAM_BILLING_TRANS_TIME_SIZE (9) // hh:mm:ss
+
+/******************************************************************************
+**
+** Scalar type and enumerated type definitions.
+**
+******************************************************************************/
+
+
+typedef unsigned int SteamHandle_t;
+
+typedef void * SteamUserIDTicketValidationHandle_t;
+
+typedef unsigned int SteamCallHandle_t;
+
+#if defined(_MSC_VER)
+typedef unsigned __int64 SteamUnsigned64_t;
+#else
+typedef unsigned long long SteamUnsigned64_t;
+#endif
+
+typedef enum
+{
+ eSteamSeekMethodSet = 0,
+ eSteamSeekMethodCur = 1,
+ eSteamSeekMethodEnd = 2
+} ESteamSeekMethod;
+
+typedef enum
+{
+ eSteamBufferMethodFBF = 0,
+ eSteamBufferMethodNBF = 1
+} ESteamBufferMethod;
+
+typedef enum
+{
+ eSteamErrorNone = 0,
+ eSteamErrorUnknown = 1,
+ eSteamErrorLibraryNotInitialized = 2,
+ eSteamErrorLibraryAlreadyInitialized = 3,
+ eSteamErrorConfig = 4,
+ eSteamErrorContentServerConnect = 5,
+ eSteamErrorBadHandle = 6,
+ eSteamErrorHandlesExhausted = 7,
+ eSteamErrorBadArg = 8,
+ eSteamErrorNotFound = 9,
+ eSteamErrorRead = 10,
+ eSteamErrorEOF = 11,
+ eSteamErrorSeek = 12,
+ eSteamErrorCannotWriteNonUserConfigFile = 13,
+ eSteamErrorCacheOpen = 14,
+ eSteamErrorCacheRead = 15,
+ eSteamErrorCacheCorrupted = 16,
+ eSteamErrorCacheWrite = 17,
+ eSteamErrorCacheSession = 18,
+ eSteamErrorCacheInternal = 19,
+ eSteamErrorCacheBadApp = 20,
+ eSteamErrorCacheVersion = 21,
+ eSteamErrorCacheBadFingerPrint = 22,
+
+ eSteamErrorNotFinishedProcessing = 23,
+ eSteamErrorNothingToDo = 24,
+ eSteamErrorCorruptEncryptedUserIDTicket = 25,
+ eSteamErrorSocketLibraryNotInitialized = 26,
+ eSteamErrorFailedToConnectToUserIDTicketValidationServer = 27,
+ eSteamErrorBadProtocolVersion = 28,
+ eSteamErrorReplayedUserIDTicketFromClient = 29,
+ eSteamErrorReceiveResultBufferTooSmall = 30,
+ eSteamErrorSendFailed = 31,
+ eSteamErrorReceiveFailed = 32,
+ eSteamErrorReplayedReplyFromUserIDTicketValidationServer = 33,
+ eSteamErrorBadSignatureFromUserIDTicketValidationServer = 34,
+ eSteamErrorValidationStalledSoAborted = 35,
+ eSteamErrorInvalidUserIDTicket = 36,
+ eSteamErrorClientLoginRateTooHigh = 37,
+ eSteamErrorClientWasNeverValidated = 38,
+ eSteamErrorInternalSendBufferTooSmall = 39,
+ eSteamErrorInternalReceiveBufferTooSmall = 40,
+ eSteamErrorUserTicketExpired = 41,
+ eSteamErrorCDKeyAlreadyInUseOnAnotherClient = 42,
+
+ eSteamErrorNotLoggedIn = 101,
+ eSteamErrorAlreadyExists = 102,
+ eSteamErrorAlreadySubscribed = 103,
+ eSteamErrorNotSubscribed = 104,
+ eSteamErrorAccessDenied = 105,
+ eSteamErrorFailedToCreateCacheFile = 106,
+ eSteamErrorCallStalledSoAborted = 107,
+ eSteamErrorEngineNotRunning = 108,
+ eSteamErrorEngineConnectionLost = 109,
+ eSteamErrorLoginFailed = 110,
+ eSteamErrorAccountPending = 111,
+ eSteamErrorCacheWasMissingRetry = 112,
+ eSteamErrorLocalTimeIncorrect = 113,
+ eSteamErrorCacheNeedsDecryption = 114,
+ eSteamErrorAccountDisabled = 115,
+ eSteamErrorCacheNeedsRepair = 116,
+ eSteamErrorRebootRequired = 117,
+
+ eSteamErrorNetwork = 200,
+ eSteamErrorOffline = 201
+
+
+} ESteamError;
+
+
+typedef enum
+{
+ eNoDetailedErrorAvailable,
+ eStandardCerrno,
+ eWin32LastError,
+ eWinSockLastError,
+ eDetailedPlatformErrorCount
+} EDetailedPlatformErrorType;
+
+typedef enum /* Filter elements returned by SteamFind{First,Next} */
+{
+ eSteamFindLocalOnly, /* limit search to local filesystem */
+ eSteamFindRemoteOnly, /* limit search to remote repository */
+ eSteamFindAll /* do not limit search (duplicates allowed) */
+} ESteamFindFilter;
+
+
+/******************************************************************************
+**
+** Exported structure and complex type definitions.
+**
+******************************************************************************/
+
+
+typedef struct
+{
+ ESteamError eSteamError;
+ EDetailedPlatformErrorType eDetailedErrorType;
+ int nDetailedErrorCode;
+ char szDesc[STEAM_MAX_PATH];
+} TSteamError;
+
+
+
+typedef struct
+{
+ int bIsDir; /* If non-zero, element is a directory; if zero, element is a file */
+ unsigned int uSizeOrCount; /* If element is a file, this contains size of file in bytes */
+ int bIsLocal; /* If non-zero, reported item is a standalone element on local filesystem */
+ char cszName[STEAM_MAX_PATH]; /* Base element name (no path) */
+ long lLastAccessTime; /* Seconds since 1/1/1970 (like time_t) when element was last accessed */
+ long lLastModificationTime; /* Seconds since 1/1/1970 (like time_t) when element was last modified */
+ long lCreationTime; /* Seconds since 1/1/1970 (like time_t) when element was created */
+} TSteamElemInfo;
+
+
+typedef struct
+{
+ unsigned int uNumSubscriptions;
+ unsigned int uMaxNameChars;
+ unsigned int uMaxApps;
+
+} TSteamSubscriptionStats;
+
+
+typedef struct
+{
+ unsigned int uNumApps;
+ unsigned int uMaxNameChars;
+ unsigned int uMaxInstallDirNameChars;
+ unsigned int uMaxVersionLabelChars;
+ unsigned int uMaxLaunchOptions;
+ unsigned int uMaxLaunchOptionDescChars;
+ unsigned int uMaxLaunchOptionCmdLineChars;
+ unsigned int uMaxNumIcons;
+ unsigned int uMaxIconSize;
+ unsigned int uMaxDependencies;
+
+} TSteamAppStats;
+
+typedef struct
+{
+ char *szLabel;
+ unsigned int uMaxLabelChars;
+ unsigned int uVersionId;
+ int bIsNotAvailable;
+} TSteamAppVersion;
+
+typedef struct
+{
+ char *szDesc;
+ unsigned int uMaxDescChars;
+ char *szCmdLine;
+ unsigned int uMaxCmdLineChars;
+ unsigned int uIndex;
+ unsigned int uIconIndex;
+ int bNoDesktopShortcut;
+ int bNoStartMenuShortcut;
+ int bIsLongRunningUnattended;
+
+} TSteamAppLaunchOption;
+
+
+typedef struct
+{
+ char *szName;
+ unsigned int uMaxNameChars;
+ char *szLatestVersionLabel;
+ unsigned int uMaxLatestVersionLabelChars;
+ char *szCurrentVersionLabel;
+ unsigned int uMaxCurrentVersionLabelChars;
+ char *szInstallDirName;
+ unsigned int uMaxInstallDirNameChars;
+ unsigned int uId;
+ unsigned int uLatestVersionId;
+ unsigned int uCurrentVersionId;
+ unsigned int uMinCacheFileSizeMB;
+ unsigned int uMaxCacheFileSizeMB;
+ unsigned int uNumLaunchOptions;
+ unsigned int uNumIcons;
+ unsigned int uNumVersions;
+ unsigned int uNumDependencies;
+
+} TSteamApp;
+
+typedef enum
+{
+ eNoCost = 0,
+ eBillOnceOnly = 1,
+ eBillMonthly = 2,
+ eProofOfPrepurchaseOnly = 3,
+ eGuestPass = 4,
+ eHardwarePromo = 5,
+ eNumBillingTypes,
+} EBillingType;
+
+typedef struct
+{
+ char *szName;
+ unsigned int uMaxNameChars;
+ unsigned int *puAppIds;
+ unsigned int uMaxAppIds;
+ unsigned int uId;
+ unsigned int uNumApps;
+ EBillingType eBillingType;
+ unsigned int uCostInCents;
+ unsigned int uNumDiscounts;
+ int bIsPreorder;
+ int bRequiresShippingAddress;
+ unsigned int uDomesticShippingCostInCents;
+ unsigned int uInternationalShippingCostInCents;
+ bool bIsCyberCafeSubscription;
+ unsigned int uGameCode;
+ char szGameCodeDesc[STEAM_MAX_PATH];
+ bool bIsDisabled;
+ bool bRequiresCD;
+ unsigned int uTerritoryCode;
+ bool bIsSteam3Subscription;
+
+} TSteamSubscription;
+
+typedef struct
+{
+ char szName[STEAM_MAX_PATH];
+ unsigned int uDiscountInCents;
+ unsigned int uNumQualifiers;
+
+} TSteamSubscriptionDiscount;
+
+typedef struct
+{
+ char szName[STEAM_MAX_PATH];
+ unsigned int uRequiredSubscription;
+ int bIsDisqualifier;
+
+} TSteamDiscountQualifier;
+
+typedef struct TSteamProgress
+{
+ int bValid; /* non-zero if call provides progress info */
+ unsigned int uPercentDone; /* 0 to 100 * STEAM_PROGRESS_PERCENT_SCALE if bValid */
+ char szProgress[STEAM_MAX_PATH]; /* additional progress info */
+} TSteamProgress;
+
+typedef enum
+{
+ eSteamNotifyTicketsWillExpire,
+ eSteamNotifyAccountInfoChanged,
+ eSteamNotifyContentDescriptionChanged,
+ eSteamNotifyPleaseShutdown,
+ eSteamNotifyNewContentServer,
+ eSteamNotifySubscriptionStatusChanged,
+ eSteamNotifyContentServerConnectionLost,
+ eSteamNotifyCacheLoadingCompleted,
+ eSteamNotifyCacheNeedsDecryption,
+ eSteamNotifyCacheNeedsRepair
+} ESteamNotificationCallbackEvent;
+
+
+typedef void(*SteamNotificationCallback_t)(ESteamNotificationCallbackEvent eEvent, unsigned int nData);
+
+
+typedef char SteamPersonalQuestion_t[ STEAM_QUESTION_MAXLEN + 1 ];
+
+typedef struct
+{
+ unsigned char uchSalt[STEAM_SALT_SIZE];
+} SteamSalt_t;
+
+typedef enum
+{
+ eVisa = 1,
+ eMaster = 2,
+ eAmericanExpress = 3,
+ eDiscover = 4,
+ eDinnersClub = 5,
+ eJCB = 6
+} ESteamPaymentCardType;
+
+typedef struct
+{
+ ESteamPaymentCardType eCardType;
+ char szCardNumber[ STEAM_CARD_NUMBER_SIZE +1 ];
+ char szCardHolderName[ STEAM_CARD_HOLDERNAME_SIZE + 1];
+ char szCardExpYear[ STEAM_CARD_EXPYEAR_SIZE + 1 ];
+ char szCardExpMonth[ STEAM_CARD_EXPMONTH_SIZE+ 1 ];
+ char szCardCVV2[ STEAM_CARD_CVV2_SIZE + 1 ];
+ char szBillingAddress1[ STEAM_BILLING_ADDRESS1_SIZE + 1 ];
+ char szBillingAddress2[ STEAM_BILLING_ADDRESS2_SIZE + 1 ];
+ char szBillingCity[ STEAM_BILLING_CITY_SIZE + 1 ];
+ char szBillingZip[ STEAM_BILLING_ZIP_SIZE + 1 ];
+ char szBillingState[ STEAM_BILLING_STATE_SIZE + 1 ];
+ char szBillingCountry[ STEAM_BILLING_COUNTRY_SIZE + 1 ];
+ char szBillingPhone[ STEAM_BILLING_PHONE_SIZE + 1 ];
+ char szBillingEmailAddress[ STEAM_BILLING_EMAIL_ADDRESS_SIZE + 1 ];
+ unsigned int uExpectedCostInCents;
+ unsigned int uExpectedTaxInCents;
+ /* If the TSteamSubscription says that shipping info is required, */
+ /* then the following fields must be filled out. */
+ /* If szShippingName is empty, then assumes so are the rest. */
+ char szShippingName[ STEAM_CARD_HOLDERNAME_SIZE + 1];
+ char szShippingAddress1[ STEAM_BILLING_ADDRESS1_SIZE + 1 ];
+ char szShippingAddress2[ STEAM_BILLING_ADDRESS2_SIZE + 1 ];
+ char szShippingCity[ STEAM_BILLING_CITY_SIZE + 1 ];
+ char szShippingZip[ STEAM_BILLING_ZIP_SIZE + 1 ];
+ char szShippingState[ STEAM_BILLING_STATE_SIZE + 1 ];
+ char szShippingCountry[ STEAM_BILLING_COUNTRY_SIZE + 1 ];
+ char szShippingPhone[ STEAM_BILLING_PHONE_SIZE + 1 ];
+ unsigned int uExpectedShippingCostInCents;
+
+} TSteamPaymentCardInfo;
+
+typedef struct
+{
+ char szTypeOfProofOfPurchase[ STEAM_TYPE_OF_PROOF_OF_PURCHASE_SIZE + 1 ];
+
+ /* A ProofOfPurchase token is not necessarily a nul-terminated string; it may be binary data
+ (perhaps encrypted). Hence we need a length and an array of bytes. */
+ unsigned int uLengthOfBinaryProofOfPurchaseToken;
+ char cBinaryProofOfPurchaseToken[ STEAM_PROOF_OF_PURCHASE_TOKEN_SIZE + 1 ];
+} TSteamPrepurchaseInfo;
+
+typedef struct
+{
+ char szAccountName[ STEAM_EXTERNAL_ACCOUNTNAME_SIZE + 1 ];
+ char szPassword[ STEAM_EXTERNAL_ACCOUNTPASSWORD_SIZE + 1 ];
+} TSteamExternalBillingInfo;
+
+typedef enum
+{
+ ePaymentCardInfo = 1,
+ ePrepurchasedInfo = 2,
+ eAccountBillingInfo = 3,
+ eExternalBillingInfo = 4, /* indirect billing via ISP etc (not supported yet) */
+ ePaymentCardReceipt = 5,
+ ePrepurchaseReceipt = 6,
+ eEmptyReceipt = 7
+} ESteamSubscriptionBillingInfoType;
+
+typedef struct
+{
+ ESteamSubscriptionBillingInfoType eBillingInfoType;
+ union {
+ TSteamPaymentCardInfo PaymentCardInfo;
+ TSteamPrepurchaseInfo PrepurchaseInfo;
+ TSteamExternalBillingInfo ExternalBillingInfo;
+ char bUseAccountBillingInfo;
+ };
+
+} TSteamSubscriptionBillingInfo;
+
+typedef enum
+{
+ /* Subscribed */
+ eSteamSubscriptionOK = 0, /* Subscribed */
+ eSteamSubscriptionPending = 1, /* Awaiting transaction completion */
+ eSteamSubscriptionPreorder = 2, /* Is currently a pre-order */
+ eSteamSubscriptionPrepurchaseTransferred = 3, /* hop to this account */
+ /* Unusbscribed */
+ eSteamSubscriptionPrepurchaseInvalid = 4, /* Invalid cd-key */
+ eSteamSubscriptionPrepurchaseRejected = 5, /* hopped out / banned / etc */
+ eSteamSubscriptionPrepurchaseRevoked = 6, /* hop away from this account */
+ eSteamSubscriptionPaymentCardDeclined = 7, /* CC txn declined */
+ eSteamSubscriptionCancelledByUser = 8, /* Cancelled by client */
+ eSteamSubscriptionCancelledByVendor = 9, /* Cancelled by us */
+ eSteamSubscriptionPaymentCardUseLimit = 10, /* Card used too many times, potential fraud */
+ eSteamSubscriptionPaymentCardAlert = 11, /* Got a "pick up card" or the like from bank */
+ eSteamSubscriptionFailed = 12, /* Other Error in subscription data or transaction failed/lost */
+ eSteamSubscriptionPaymentCardAVSFailure = 13, /* Card failed Address Verification check */
+ eSteamSubscriptionPaymentCardInsufficientFunds = 14, /* Card failed due to insufficient funds */
+ eSteamSubscriptionRestrictedCountry = 15 /* The subscription is not available in the user's country */
+
+} ESteamSubscriptionStatus;
+
+typedef struct
+{
+ ESteamPaymentCardType eCardType;
+ char szCardLastFourDigits[ 4 + 1 ];
+ char szCardHolderName[ STEAM_CARD_HOLDERNAME_SIZE + 1];
+ char szBillingAddress1[ STEAM_BILLING_ADDRESS1_SIZE + 1 ];
+ char szBillingAddress2[ STEAM_BILLING_ADDRESS2_SIZE + 1 ];
+ char szBillingCity[ STEAM_BILLING_CITY_SIZE + 1 ];
+ char szBillingZip[ STEAM_BILLING_ZIP_SIZE + 1 ];
+ char szBillingState[ STEAM_BILLING_STATE_SIZE + 1 ];
+ char szBillingCountry[ STEAM_BILLING_COUNTRY_SIZE + 1 ];
+
+ // The following are only available after the subscription leaves "pending" status
+ char szCardApprovalCode[ STEAM_BILLING_CARD_APPROVAL_CODE_SIZE + 1];
+ char szTransDate[ STEAM_BILLING_TRANS_DATE_SIZE + 1]; /* mm/dd/yy */
+ char szTransTime[ STEAM_BILLING_TRANS_TIME_SIZE + 1]; /* hh:mm:ss */
+ unsigned int uPriceWithoutTax;
+ unsigned int uTaxAmount;
+ unsigned int uShippingCost;
+
+} TSteamPaymentCardReceiptInfo;
+
+typedef struct
+{
+ char szTypeOfProofOfPurchase[ STEAM_TYPE_OF_PROOF_OF_PURCHASE_SIZE + 1 ];
+} TSteamPrepurchaseReceiptInfo;
+
+typedef struct
+{
+ ESteamSubscriptionStatus eStatus;
+ ESteamSubscriptionStatus ePreviousStatus;
+ ESteamSubscriptionBillingInfoType eReceiptInfoType;
+ char szConfirmationCode[ STEAM_BILLING_CONFIRMATION_CODE_SIZE + 1];
+ union {
+ TSteamPaymentCardReceiptInfo PaymentCardReceiptInfo;
+ TSteamPrepurchaseReceiptInfo PrepurchaseReceiptInfo;
+ };
+
+} TSteamSubscriptionReceipt;
+
+typedef enum
+{
+ ePhysicalBytesReceivedThisSession = 1,
+ eAppReadyToLaunchStatus = 2,
+ eAppPreloadStatus = 3,
+ eAppEntireDepot = 4,
+ eCacheBytesPresent = 5
+} ESteamAppUpdateStatsQueryType;
+
+typedef struct
+{
+ SteamUnsigned64_t uBytesTotal;
+ SteamUnsigned64_t uBytesPresent;
+} TSteamUpdateStats;
+
+typedef enum
+{
+ eSteamUserAdministrator = 0x00000001, /* May subscribe, unsubscribe, etc */
+ eSteamUserDeveloper = 0x00000002, /* Steam or App developer */
+ eSteamUserCyberCafe = 0x00000004 /* CyberCafe, school, etc -- UI should ask for password */
+ /* before allowing logout, unsubscribe, etc */
+} ESteamUserTypeFlags;
+
+typedef enum
+{
+ eSteamAccountStatusDefault = 0x00000000,
+ eSteamAccountStatusEmailVerified = 0x00000001,
+ /* Note: Mask value 0x2 is reserved for future use. (Some, but not all, public accounts already have this set.) */
+ eSteamAccountDisabled = 0x00000004
+} ESteamAccountStatusBitFields ;
+
+
+typedef enum
+{
+ eSteamBootstrapperError = -1,
+ eSteamBootstrapperDontCheckForUpdate = 0,
+ eSteamBootstrapperCheckForUpdateAndRerun = 7
+
+} ESteamBootStrapperClientAppResult;
+
+typedef enum
+{
+ eSteamOnline = 0,
+ eSteamOffline = 1,
+ eSteamNoAuthMode = 2,
+ eSteamBillingOffline = 3
+} eSteamOfflineStatus;
+
+typedef struct
+{
+ int eOfflineNow;
+ int eOfflineNextSession;
+} TSteamOfflineStatus;
+
+typedef struct
+{
+ unsigned int uAppId;
+ int bIsSystemDefined;
+ char szMountPath[STEAM_MAX_PATH];
+} TSteamAppDependencyInfo;
+
+typedef enum
+{
+ eSteamOpenFileRegular = 0x0,
+ eSteamOpenFileIgnoreLocal = 0x1,
+ eSteamOpenFileChecksumReads = 0x2
+} ESteamOpenFileFlags;
+
+typedef enum
+{
+ eSteamValveCDKeyValidationServer = 0,
+ eSteamHalfLifeMasterServer = 1,
+ eSteamFriendsServer = 2,
+ eSteamCSERServer = 3,
+ eSteamHalfLife2MasterServer = 4,
+ eSteamRDKFMasterServer = 5,
+ eMaxServerTypes = 6
+} ESteamServerType;
+
+/******************************************************************************
+**
+** More exported constants
+**
+******************************************************************************/
+
+
+#ifdef __cplusplus
+
+const SteamHandle_t STEAM_INVALID_HANDLE = 0;
+const SteamCallHandle_t STEAM_INVALID_CALL_HANDLE = 0;
+const SteamUserIDTicketValidationHandle_t STEAM_INACTIVE_USERIDTICKET_VALIDATION_HANDLE = 0;
+const unsigned int STEAM_USE_LATEST_VERSION = 0xFFFFFFFF;
+
+#else
+
+#define STEAM_INVALID_HANDLE ((SteamHandle_t)(0))
+#define STEAM_INVALID_CALL_HANDLE ((SteamCallHandle_t)(0))
+#define STEAM_INACTIVE_USERIDTICKET_VALIDATION_HANDLE ((SteamUserIDTicketValidationHandle_t)(0))
+#define STEAM_USE_LATEST_VERSION (0xFFFFFFFFu);
+
+#endif
+
+
+/******************************************************************************
+** Each Steam instance (licensed Steam Service Provider) has a unique SteamInstanceID_t.
+**
+** Each Steam instance as its own DB of users.
+** Each user in the DB has a unique SteamLocalUserID_t (a serial number, with possible
+** rare gaps in the sequence).
+**
+******************************************************************************/
+
+typedef unsigned short SteamInstanceID_t; // MUST be 16 bits
+
+
+#if defined ( _WIN32 )
+typedef unsigned __int64 SteamLocalUserID_t; // MUST be 64 bits
+#else
+typedef unsigned long long SteamLocalUserID_t; // MUST be 64 bits
+#endif
+
+/******************************************************************************
+**
+** Applications need to be able to authenticate Steam users from ANY instance.
+** So a SteamIDTicket contains SteamGlobalUserID, which is a unique combination of
+** instance and user id.
+**
+** SteamLocalUserID is an unsigned 64-bit integer.
+** For platforms without 64-bit int support, we provide access via a union that splits it into
+** high and low unsigned 32-bit ints. Such platforms will only need to compare LocalUserIDs
+** for equivalence anyway - not perform arithmetic with them.
+**
+********************************************************************************/
+typedef struct
+{
+ unsigned int Low32bits;
+ unsigned int High32bits;
+} TSteamSplitLocalUserID;
+
+typedef struct
+{
+ SteamInstanceID_t m_SteamInstanceID;
+
+ union
+ {
+ SteamLocalUserID_t As64bits;
+ TSteamSplitLocalUserID Split;
+ } m_SteamLocalUserID;
+
+} TSteamGlobalUserID;
+
+
+#ifdef __cplusplus
+}
+#endif
+
+
+#endif
diff --git a/dep/hlsdk/common/TextConsoleUnix.cpp b/dep/hlsdk/common/TextConsoleUnix.cpp
new file mode 100644
index 0000000..32671ae
--- /dev/null
+++ b/dep/hlsdk/common/TextConsoleUnix.cpp
@@ -0,0 +1,324 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#include "precompiled.h"
+
+#if !defined(_WIN32)
+
+#include "TextConsoleUnix.h"
+#include "icommandline.h"
+
+#include
+#include
+#include
+#include
+#include
+#include
+#include
+
+CTextConsoleUnix console;
+
+CTextConsoleUnix::~CTextConsoleUnix()
+{
+ CTextConsoleUnix::ShutDown();
+}
+
+bool CTextConsoleUnix::Init(IBaseSystem *system)
+{
+ static struct termios termNew;
+ sigset_t block_ttou;
+
+ sigemptyset(&block_ttou);
+ sigaddset(&block_ttou, SIGTTOU);
+ sigprocmask(SIG_BLOCK, &block_ttou, NULL);
+
+ tty = stdout;
+
+ // this code is for echo-ing key presses to the connected tty
+ // (which is != STDOUT)
+ if (isatty(STDIN_FILENO))
+ {
+ tty = fopen(ctermid(NULL), "w+");
+ if (!tty)
+ {
+ printf("Unable to open tty(%s) for output\n", ctermid(NULL));
+ tty = stdout;
+ }
+ else
+ {
+ // turn buffering off
+ setbuf(tty, NULL);
+ }
+ }
+ else
+ {
+ tty = fopen("/dev/null", "w+");
+ if (!tty)
+ {
+ tty = stdout;
+ }
+ }
+
+ tcgetattr(STDIN_FILENO, &termStored);
+
+ Q_memcpy(&termNew, &termStored, sizeof(struct termios));
+
+ // Disable canonical mode, and set buffer size to 1 byte
+ termNew.c_lflag &= (~ICANON);
+ termNew.c_cc[ VMIN ] = 1;
+ termNew.c_cc[ VTIME ] = 0;
+
+ // disable echo
+ termNew.c_lflag &= (~ECHO);
+
+ tcsetattr(STDIN_FILENO, TCSANOW, &termNew);
+ sigprocmask(SIG_UNBLOCK, &block_ttou, NULL);
+
+ return CTextConsole::Init(system);
+}
+
+void CTextConsoleUnix::ShutDown()
+{
+ sigset_t block_ttou;
+
+ sigemptyset(&block_ttou);
+ sigaddset(&block_ttou, SIGTTOU);
+ sigprocmask(SIG_BLOCK, &block_ttou, NULL);
+ tcsetattr(STDIN_FILENO, TCSANOW, &termStored);
+ sigprocmask(SIG_UNBLOCK, &block_ttou, NULL);
+
+ CTextConsole::ShutDown();
+}
+
+// return 0 if the kb isn't hit
+int CTextConsoleUnix::kbhit()
+{
+ fd_set rfds;
+ struct timeval tv;
+
+ // Watch stdin (fd 0) to see when it has input.
+ FD_ZERO(&rfds);
+ FD_SET(STDIN_FILENO, &rfds);
+
+ // Return immediately.
+ tv.tv_sec = 0;
+ tv.tv_usec = 0;
+
+ // Must be in raw or cbreak mode for this to work correctly.
+ return select(STDIN_FILENO + 1, &rfds, NULL, NULL, &tv) != -1 && FD_ISSET(STDIN_FILENO, &rfds);
+}
+
+char *CTextConsoleUnix::GetLine()
+{
+ // early return for 99.999% case :)
+ if (!kbhit())
+ return NULL;
+
+ escape_sequence_t es;
+
+ es = ESCAPE_CLEAR;
+ sigset_t block_ttou;
+
+ sigemptyset(&block_ttou);
+ sigaddset(&block_ttou, SIGTTOU);
+ sigaddset(&block_ttou, SIGTTIN);
+ sigprocmask(SIG_BLOCK, &block_ttou, NULL);
+
+ while (true)
+ {
+ if (!kbhit())
+ break;
+
+ int nLen;
+ char ch = 0;
+ int numRead = read(STDIN_FILENO, &ch, 1);
+ if (!numRead)
+ break;
+
+ switch (ch)
+ {
+ case '\n': // Enter
+ es = ESCAPE_CLEAR;
+
+ nLen = ReceiveNewline();
+ if (nLen)
+ {
+ sigprocmask(SIG_UNBLOCK, &block_ttou, NULL);
+ return m_szConsoleText;
+ }
+ break;
+
+ case 127: // Backspace
+ case '\b': // Backspace
+ es = ESCAPE_CLEAR;
+ ReceiveBackspace();
+ break;
+
+ case '\t': // TAB
+ es = ESCAPE_CLEAR;
+ ReceiveTab();
+ break;
+
+ case 27: // Escape character
+ es = ESCAPE_RECEIVED;
+ break;
+
+ case '[': // 2nd part of escape sequence
+ case 'O':
+ case 'o':
+ switch (es)
+ {
+ case ESCAPE_CLEAR:
+ case ESCAPE_BRACKET_RECEIVED:
+ es = ESCAPE_CLEAR;
+ ReceiveStandardChar(ch);
+ break;
+
+ case ESCAPE_RECEIVED:
+ es = ESCAPE_BRACKET_RECEIVED;
+ break;
+ }
+ break;
+ case 'A':
+ if (es == ESCAPE_BRACKET_RECEIVED)
+ {
+ es = ESCAPE_CLEAR;
+ ReceiveUpArrow();
+ }
+ else
+ {
+ es = ESCAPE_CLEAR;
+ ReceiveStandardChar(ch);
+ }
+ break;
+ case 'B':
+ if (es == ESCAPE_BRACKET_RECEIVED)
+ {
+ es = ESCAPE_CLEAR;
+ ReceiveDownArrow();
+ }
+ else
+ {
+ es = ESCAPE_CLEAR;
+ ReceiveStandardChar(ch);
+ }
+ break;
+ case 'C':
+ if (es == ESCAPE_BRACKET_RECEIVED)
+ {
+ es = ESCAPE_CLEAR;
+ ReceiveRightArrow();
+ }
+ else
+ {
+ es = ESCAPE_CLEAR;
+ ReceiveStandardChar(ch);
+ }
+ break;
+ case 'D':
+ if (es == ESCAPE_BRACKET_RECEIVED)
+ {
+ es = ESCAPE_CLEAR;
+ ReceiveLeftArrow();
+ }
+ else
+ {
+ es = ESCAPE_CLEAR;
+ ReceiveStandardChar(ch);
+ }
+ break;
+ default:
+ // Just eat this char if it's an unsupported escape
+ if (es != ESCAPE_BRACKET_RECEIVED)
+ {
+ // dont' accept nonprintable chars
+ if ((ch >= ' ') && (ch <= '~'))
+ {
+ es = ESCAPE_CLEAR;
+ ReceiveStandardChar(ch);
+ }
+ }
+ break;
+ }
+
+ fflush(stdout);
+ }
+
+ sigprocmask(SIG_UNBLOCK, &block_ttou, NULL);
+ return NULL;
+}
+
+void CTextConsoleUnix::PrintRaw(char *pszMsg, int nChars)
+{
+ if (nChars == 0)
+ {
+ printf("%s", pszMsg);
+ }
+ else
+ {
+ for (int nCount = 0; nCount < nChars; nCount++)
+ {
+ putchar(pszMsg[ nCount ]);
+ }
+ }
+}
+
+void CTextConsoleUnix::Echo(char *pszMsg, int nChars)
+{
+ if (nChars == 0)
+ {
+ fputs(pszMsg, tty);
+ }
+ else
+ {
+ for (int nCount = 0; nCount < nChars; nCount++)
+ {
+ fputc(pszMsg[ nCount ], tty);
+ }
+ }
+}
+
+int CTextConsoleUnix::GetWidth()
+{
+ struct winsize ws;
+ int nWidth = 0;
+
+ if (ioctl(STDOUT_FILENO, TIOCGWINSZ, &ws) == 0)
+ {
+ nWidth = (int)ws.ws_col;
+ }
+
+ if (nWidth <= 1)
+ {
+ nWidth = 80;
+ }
+
+ return nWidth;
+}
+
+#endif // !defined(_WIN32)
diff --git a/dep/hlsdk/common/TextConsoleUnix.h b/dep/hlsdk/common/TextConsoleUnix.h
new file mode 100644
index 0000000..b40cbc4
--- /dev/null
+++ b/dep/hlsdk/common/TextConsoleUnix.h
@@ -0,0 +1,59 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include
+#include "textconsole.h"
+
+enum escape_sequence_t
+{
+ ESCAPE_CLEAR,
+ ESCAPE_RECEIVED,
+ ESCAPE_BRACKET_RECEIVED
+};
+
+class CTextConsoleUnix: public CTextConsole {
+public:
+ virtual ~CTextConsoleUnix();
+
+ bool Init(IBaseSystem *system = nullptr);
+ void ShutDown();
+ void PrintRaw(char *pszMsg, int nChars = 0);
+ void Echo(char *pszMsg, int nChars = 0);
+ char *GetLine();
+ int GetWidth();
+
+private:
+ int kbhit();
+
+ struct termios termStored;
+ FILE *tty;
+};
+
+extern CTextConsoleUnix console;
diff --git a/dep/hlsdk/common/TextConsoleWin32.cpp b/dep/hlsdk/common/TextConsoleWin32.cpp
new file mode 100644
index 0000000..aeaefb3
--- /dev/null
+++ b/dep/hlsdk/common/TextConsoleWin32.cpp
@@ -0,0 +1,282 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#include "precompiled.h"
+
+#if defined(_WIN32)
+
+CTextConsoleWin32 console;
+#pragma comment(lib, "user32.lib")
+
+BOOL WINAPI ConsoleHandlerRoutine(DWORD CtrlType)
+{
+ // TODO ?
+ /*if (CtrlType != CTRL_C_EVENT && CtrlType != CTRL_BREAK_EVENT)
+ {
+ // don't quit on break or ctrl+c
+ m_System->Stop();
+ }*/
+
+ return TRUE;
+}
+
+// GetConsoleHwnd() helper function from MSDN Knowledge Base Article Q124103
+// needed, because HWND GetConsoleWindow(VOID) is not avaliable under Win95/98/ME
+HWND GetConsoleHwnd()
+{
+ HWND hwndFound; // This is what is returned to the caller.
+ char pszNewWindowTitle[1024]; // Contains fabricated WindowTitle
+ char pszOldWindowTitle[1024]; // Contains original WindowTitle
+
+ // Fetch current window title.
+ GetConsoleTitle(pszOldWindowTitle, sizeof(pszOldWindowTitle));
+
+ // Format a "unique" NewWindowTitle.
+ wsprintf(pszNewWindowTitle, "%d/%d", GetTickCount(), GetCurrentProcessId());
+
+ // Change current window title.
+ SetConsoleTitle(pszNewWindowTitle);
+
+ // Ensure window title has been updated.
+ Sleep(40);
+
+ // Look for NewWindowTitle.
+ hwndFound = FindWindow(nullptr, pszNewWindowTitle);
+
+ // Restore original window title.
+ SetConsoleTitle(pszOldWindowTitle);
+
+ return hwndFound;
+}
+
+CTextConsoleWin32::~CTextConsoleWin32()
+{
+ CTextConsoleWin32::ShutDown();
+}
+
+bool CTextConsoleWin32::Init(IBaseSystem *system)
+{
+ if (!AllocConsole())
+ m_System = system;
+
+ SetTitle(m_System ? m_System->GetName() : "Console");
+
+ hinput = GetStdHandle(STD_INPUT_HANDLE);
+ houtput = GetStdHandle(STD_OUTPUT_HANDLE);
+
+ if (!SetConsoleCtrlHandler(&ConsoleHandlerRoutine, TRUE)) {
+ Print("WARNING! TextConsole::Init: Could not attach console hook.\n");
+ }
+
+ Attrib = FOREGROUND_GREEN | FOREGROUND_INTENSITY | BACKGROUND_INTENSITY;
+ SetWindowPos(GetConsoleHwnd(), HWND_TOP, 0, 0, 0, 0, SWP_NOSIZE | SWP_NOREPOSITION | SWP_SHOWWINDOW);
+
+ return CTextConsole::Init(system);
+}
+
+void CTextConsoleWin32::ShutDown()
+{
+ FreeConsole();
+ CTextConsole::ShutDown();
+}
+
+void CTextConsoleWin32::SetVisible(bool visible)
+{
+ ShowWindow(GetConsoleHwnd(), visible ? SW_SHOW : SW_HIDE);
+ m_ConsoleVisible = visible;
+}
+
+char *CTextConsoleWin32::GetLine()
+{
+ while (true)
+ {
+ INPUT_RECORD recs[1024];
+ unsigned long numread;
+ unsigned long numevents;
+
+ if (!GetNumberOfConsoleInputEvents(hinput, &numevents))
+ {
+ if (m_System) {
+ m_System->Errorf("CTextConsoleWin32::GetLine: !GetNumberOfConsoleInputEvents\n");
+ }
+
+ return nullptr;
+ }
+
+ if (numevents <= 0)
+ break;
+
+ if (!ReadConsoleInput(hinput, recs, ARRAYSIZE(recs), &numread))
+ {
+ if (m_System) {
+ m_System->Errorf("CTextConsoleWin32::GetLine: !ReadConsoleInput\n");
+ }
+
+ return nullptr;
+ }
+
+ if (numread == 0)
+ return nullptr;
+
+ for (int i = 0; i < (int)numread; i++)
+ {
+ INPUT_RECORD *pRec = &recs[i];
+ if (pRec->EventType != KEY_EVENT)
+ continue;
+
+ if (pRec->Event.KeyEvent.bKeyDown)
+ {
+ // check for cursor keys
+ if (pRec->Event.KeyEvent.wVirtualKeyCode == VK_UP)
+ {
+ ReceiveUpArrow();
+ }
+ else if (pRec->Event.KeyEvent.wVirtualKeyCode == VK_DOWN)
+ {
+ ReceiveDownArrow();
+ }
+ else if (pRec->Event.KeyEvent.wVirtualKeyCode == VK_LEFT)
+ {
+ ReceiveLeftArrow();
+ }
+ else if (pRec->Event.KeyEvent.wVirtualKeyCode == VK_RIGHT)
+ {
+ ReceiveRightArrow();
+ }
+ else
+ {
+ int nLen;
+ char ch = pRec->Event.KeyEvent.uChar.AsciiChar;
+ switch (ch)
+ {
+ case '\r': // Enter
+ nLen = ReceiveNewline();
+ if (nLen)
+ {
+ return m_szConsoleText;
+ }
+ break;
+ case '\b': // Backspace
+ ReceiveBackspace();
+ break;
+ case '\t': // TAB
+ ReceiveTab();
+ break;
+ default:
+ // dont' accept nonprintable chars
+ if ((ch >= ' ') && (ch <= '~'))
+ {
+ ReceiveStandardChar(ch);
+ }
+ break;
+ }
+ }
+ }
+ }
+ }
+
+ return nullptr;
+}
+
+void CTextConsoleWin32::PrintRaw(char *pszMsg, int nChars)
+{
+#ifdef LAUNCHER_FIXES
+ char outputStr[2048];
+ WCHAR unicodeStr[1024];
+
+ DWORD nSize = MultiByteToWideChar(CP_UTF8, 0, pszMsg, -1, NULL, 0);
+ if (nSize > sizeof(unicodeStr))
+ return;
+
+ MultiByteToWideChar(CP_UTF8, 0, pszMsg, -1, unicodeStr, nSize);
+ DWORD nLength = WideCharToMultiByte(CP_OEMCP, 0, unicodeStr, -1, 0, 0, NULL, NULL);
+ if (nLength > sizeof(outputStr))
+ return;
+
+ WideCharToMultiByte(CP_OEMCP, 0, unicodeStr, -1, outputStr, nLength, NULL, NULL);
+ WriteFile(houtput, outputStr, nChars ? nChars : Q_strlen(outputStr), NULL, NULL);
+#else
+ WriteFile(houtput, pszMsg, nChars ? nChars : Q_strlen(pszMsg), NULL, NULL);
+#endif
+}
+
+void CTextConsoleWin32::Echo(char *pszMsg, int nChars)
+{
+ PrintRaw(pszMsg, nChars);
+}
+
+int CTextConsoleWin32::GetWidth()
+{
+ CONSOLE_SCREEN_BUFFER_INFO csbi;
+ int nWidth = 0;
+
+ if (GetConsoleScreenBufferInfo(houtput, &csbi)) {
+ nWidth = csbi.dwSize.X;
+ }
+
+ if (nWidth <= 1)
+ nWidth = 80;
+
+ return nWidth;
+}
+
+void CTextConsoleWin32::SetStatusLine(char *pszStatus)
+{
+ Q_strncpy(statusline, pszStatus, sizeof(statusline) - 1);
+ statusline[sizeof(statusline) - 2] = '\0';
+ UpdateStatus();
+}
+
+void CTextConsoleWin32::UpdateStatus()
+{
+ COORD coord;
+ DWORD dwWritten = 0;
+ WORD wAttrib[ 80 ];
+
+ for (int i = 0; i < 80; i++)
+ {
+ wAttrib[i] = Attrib; // FOREGROUND_GREEN | FOREGROUND_INTENSITY | BACKGROUND_INTENSITY;
+ }
+
+ coord.X = coord.Y = 0;
+
+ WriteConsoleOutputAttribute(houtput, wAttrib, 80, coord, &dwWritten);
+ WriteConsoleOutputCharacter(houtput, statusline, 80, coord, &dwWritten);
+}
+
+void CTextConsoleWin32::SetTitle(char *pszTitle)
+{
+ SetConsoleTitle(pszTitle);
+}
+
+void CTextConsoleWin32::SetColor(WORD attrib)
+{
+ Attrib = attrib;
+}
+
+#endif // defined(_WIN32)
diff --git a/dep/hlsdk/common/TextConsoleWin32.h b/dep/hlsdk/common/TextConsoleWin32.h
new file mode 100644
index 0000000..656110e
--- /dev/null
+++ b/dep/hlsdk/common/TextConsoleWin32.h
@@ -0,0 +1,62 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#define WIN32_LEAN_AND_MEAN // Exclude rarely-used stuff from Windows headers
+#include
+#include "TextConsole.h"
+
+class CTextConsoleWin32: public CTextConsole {
+public:
+ virtual ~CTextConsoleWin32();
+
+ bool Init(IBaseSystem *system = nullptr);
+ void ShutDown();
+
+ void SetTitle(char *pszTitle);
+ void SetStatusLine(char *pszStatus);
+ void UpdateStatus();
+
+ void PrintRaw(char * pszMsz, int nChars = 0);
+ void Echo(char * pszMsz, int nChars = 0);
+ char *GetLine();
+ int GetWidth();
+
+ void SetVisible(bool visible);
+ void SetColor(WORD);
+
+private:
+ HANDLE hinput; // standard input handle
+ HANDLE houtput; // standard output handle
+ WORD Attrib; // attrib colours for status bar
+
+ char statusline[81]; // first line in console is status line
+};
+
+extern CTextConsoleWin32 console;
diff --git a/dep/hlsdk/common/TokenLine.cpp b/dep/hlsdk/common/TokenLine.cpp
new file mode 100644
index 0000000..7f88555
--- /dev/null
+++ b/dep/hlsdk/common/TokenLine.cpp
@@ -0,0 +1,132 @@
+#include "precompiled.h"
+
+TokenLine::TokenLine()
+{
+ Q_memset(m_token, 0, sizeof(m_token));
+ Q_memset(m_fullLine, 0, sizeof(m_fullLine));
+ Q_memset(m_tokenBuffer, 0, sizeof(m_tokenBuffer));
+
+ m_tokenNumber = 0;
+}
+
+TokenLine::TokenLine(char *string)
+{
+ SetLine(string);
+}
+
+TokenLine::~TokenLine()
+{
+
+}
+
+bool TokenLine::SetLine(const char *newLine)
+{
+ m_tokenNumber = 0;
+
+ if (!newLine || (Q_strlen(newLine) >= (MAX_LINE_CHARS - 1)))
+ {
+ Q_memset(m_fullLine, 0, sizeof(m_fullLine));
+ Q_memset(m_tokenBuffer, 0, sizeof(m_tokenBuffer));
+ return false;
+ }
+
+ Q_strlcpy(m_fullLine, newLine);
+ Q_strlcpy(m_tokenBuffer, newLine);
+
+ // parse tokens
+ char *charPointer = m_tokenBuffer;
+ while (*charPointer && (m_tokenNumber < MAX_LINE_TOKENS))
+ {
+ // skip nonprintable chars
+ while (*charPointer && ((*charPointer <= ' ') || (*charPointer > '~')))
+ charPointer++;
+
+ if (*charPointer)
+ {
+ m_token[m_tokenNumber] = charPointer;
+
+ // special treatment for quotes
+ if (*charPointer == '\"')
+ {
+ charPointer++;
+ m_token[m_tokenNumber] = charPointer;
+ while (*charPointer && (*charPointer != '\"'))
+ charPointer++;
+ }
+ else
+ {
+ m_token[m_tokenNumber] = charPointer;
+ while (*charPointer && ((*charPointer > 32) && (*charPointer <= 126)))
+ charPointer++;
+ }
+
+ m_tokenNumber++;
+
+ if (*charPointer)
+ {
+ *charPointer = '\0';
+ charPointer++;
+ }
+ }
+ }
+
+ return (m_tokenNumber != MAX_LINE_TOKENS);
+}
+
+char *TokenLine::GetLine()
+{
+ return m_fullLine;
+}
+
+char *TokenLine::GetToken(int i)
+{
+ if (i >= m_tokenNumber)
+ return NULL;
+
+ return m_token[i];
+}
+
+// if the given parm is not present return NULL
+// otherwise return the address of the following token, or an empty string
+char *TokenLine::CheckToken(char *parm)
+{
+ for (int i = 0; i < m_tokenNumber; i++)
+ {
+ if (!m_token[i])
+ continue;
+
+ if (!Q_strcmp(parm, m_token[i]))
+ {
+ char *ret = m_token[i + 1];
+
+ // if this token doesn't exist, since index i was the last
+ // return an empty string
+ if (m_tokenNumber == (i + 1))
+ ret = "";
+
+ return ret;
+ }
+ }
+
+ return NULL;
+}
+
+int TokenLine::CountToken()
+{
+ int c = 0;
+ for (int i = 0; i < m_tokenNumber; i++)
+ {
+ if (m_token[i])
+ c++;
+ }
+
+ return c;
+}
+
+char *TokenLine::GetRestOfLine(int i)
+{
+ if (i >= m_tokenNumber)
+ return NULL;
+
+ return m_fullLine + (m_token[i] - m_tokenBuffer);
+}
diff --git a/dep/hlsdk/common/TokenLine.h b/dep/hlsdk/common/TokenLine.h
new file mode 100644
index 0000000..0d16fb6
--- /dev/null
+++ b/dep/hlsdk/common/TokenLine.h
@@ -0,0 +1,51 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class TokenLine {
+public:
+ TokenLine();
+ TokenLine(char *string);
+ virtual ~TokenLine();
+
+ char *GetRestOfLine(int i); // returns all chars after token i
+ int CountToken(); // returns number of token
+ char *CheckToken(char *parm); // returns token after token parm or ""
+ char *GetToken(int i); // returns token i
+ char *GetLine(); // returns full line
+ bool SetLine(const char *newLine); // set new token line and parses it
+
+private:
+ enum { MAX_LINE_CHARS = 2048, MAX_LINE_TOKENS = 128 };
+
+ char m_tokenBuffer[MAX_LINE_CHARS];
+ char m_fullLine[MAX_LINE_CHARS];
+ char *m_token[MAX_LINE_TOKENS];
+ int m_tokenNumber;
+};
diff --git a/dep/hlsdk/common/beamdef.h b/dep/hlsdk/common/beamdef.h
new file mode 100644
index 0000000..fd77a76
--- /dev/null
+++ b/dep/hlsdk/common/beamdef.h
@@ -0,0 +1,62 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined ( BEAMDEFH )
+#define BEAMDEFH
+#ifdef _WIN32
+#pragma once
+#endif
+
+#define FBEAM_STARTENTITY 0x00000001
+#define FBEAM_ENDENTITY 0x00000002
+#define FBEAM_FADEIN 0x00000004
+#define FBEAM_FADEOUT 0x00000008
+#define FBEAM_SINENOISE 0x00000010
+#define FBEAM_SOLID 0x00000020
+#define FBEAM_SHADEIN 0x00000040
+#define FBEAM_SHADEOUT 0x00000080
+#define FBEAM_STARTVISIBLE 0x10000000 // Has this client actually seen this beam's start entity yet?
+#define FBEAM_ENDVISIBLE 0x20000000 // Has this client actually seen this beam's end entity yet?
+#define FBEAM_ISACTIVE 0x40000000
+#define FBEAM_FOREVER 0x80000000
+
+typedef struct beam_s BEAM;
+struct beam_s
+{
+ BEAM *next;
+ int type;
+ int flags;
+ vec3_t source;
+ vec3_t target;
+ vec3_t delta;
+ float t; // 0 .. 1 over lifetime of beam
+ float freq;
+ float die;
+ float width;
+ float amplitude;
+ float r, g, b;
+ float brightness;
+ float speed;
+ float frameRate;
+ float frame;
+ int segments;
+ int startEntity;
+ int endEntity;
+ int modelIndex;
+ int frameCount;
+ struct model_s *pFollowModel;
+ struct particle_s *particles;
+};
+
+#endif
diff --git a/dep/hlsdk/common/cl_entity.h b/dep/hlsdk/common/cl_entity.h
new file mode 100644
index 0000000..a7cd472
--- /dev/null
+++ b/dep/hlsdk/common/cl_entity.h
@@ -0,0 +1,115 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+// cl_entity.h
+#if !defined( CL_ENTITYH )
+#define CL_ENTITYH
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef struct efrag_s
+{
+ struct mleaf_s *leaf;
+ struct efrag_s *leafnext;
+ struct cl_entity_s *entity;
+ struct efrag_s *entnext;
+} efrag_t;
+
+typedef struct
+{
+ byte mouthopen; // 0 = mouth closed, 255 = mouth agape
+ byte sndcount; // counter for running average
+ int sndavg; // running average
+} mouth_t;
+
+typedef struct
+{
+ float prevanimtime;
+ float sequencetime;
+ byte prevseqblending[2];
+ vec3_t prevorigin;
+ vec3_t prevangles;
+
+ int prevsequence;
+ float prevframe;
+
+ byte prevcontroller[4];
+ byte prevblending[2];
+} latchedvars_t;
+
+typedef struct
+{
+ // Time stamp for this movement
+ float animtime;
+
+ vec3_t origin;
+ vec3_t angles;
+} position_history_t;
+
+typedef struct cl_entity_s cl_entity_t;
+
+#define HISTORY_MAX 64 // Must be power of 2
+#define HISTORY_MASK ( HISTORY_MAX - 1 )
+
+
+#if !defined( ENTITY_STATEH )
+#include "entity_state.h"
+#endif
+
+#if !defined( PROGS_H )
+#include "progs.h"
+#endif
+
+struct cl_entity_s
+{
+ int index; // Index into cl_entities ( should match actual slot, but not necessarily )
+
+ qboolean player; // True if this entity is a "player"
+
+ entity_state_t baseline; // The original state from which to delta during an uncompressed message
+ entity_state_t prevstate; // The state information from the penultimate message received from the server
+ entity_state_t curstate; // The state information from the last message received from server
+
+ int current_position; // Last received history update index
+ position_history_t ph[ HISTORY_MAX ]; // History of position and angle updates for this player
+
+ mouth_t mouth; // For synchronizing mouth movements.
+
+ latchedvars_t latched; // Variables used by studio model rendering routines
+
+ // Information based on interplocation, extrapolation, prediction, or just copied from last msg received.
+ //
+ float lastmove;
+
+ // Actual render position and angles
+ vec3_t origin;
+ vec3_t angles;
+
+ // Attachment points
+ vec3_t attachment[4];
+
+ // Other entity local information
+ int trivial_accept;
+
+ struct model_s *model; // cl.model_precache[ curstate.modelindes ]; all visible entities have a model
+ struct efrag_s *efrag; // linked list of efrags
+ struct mnode_s *topnode; // for bmodels, first world node that splits bmodel, or NULL if not split
+
+ float syncbase; // for client-side animations -- used by obsolete alias animation system, remove?
+ int visframe; // last frame this entity was found in an active leaf
+ colorVec cvFloorColor;
+};
+
+#endif // !CL_ENTITYH
diff --git a/dep/hlsdk/common/com_model.h b/dep/hlsdk/common/com_model.h
new file mode 100644
index 0000000..4e85d22
--- /dev/null
+++ b/dep/hlsdk/common/com_model.h
@@ -0,0 +1,340 @@
+//========= Copyright © 1996-2002, Valve LLC, All rights reserved. ============
+//
+// Purpose:
+//
+// $NoKeywords: $
+//=============================================================================
+
+// com_model.h
+#pragma once
+
+#define STUDIO_RENDER 1
+#define STUDIO_EVENTS 2
+
+//#define MAX_MODEL_NAME 64
+//#define MAX_MAP_HULLS 4
+//#define MIPLEVELS 4
+//#define NUM_AMBIENTS 4 // automatic ambient sounds
+//#define MAXLIGHTMAPS 4
+#define PLANE_ANYZ 5
+
+#define ALIAS_Z_CLIP_PLANE 5
+
+// flags in finalvert_t.flags
+#define ALIAS_LEFT_CLIP 0x0001
+#define ALIAS_TOP_CLIP 0x0002
+#define ALIAS_RIGHT_CLIP 0x0004
+#define ALIAS_BOTTOM_CLIP 0x0008
+#define ALIAS_Z_CLIP 0x0010
+#define ALIAS_ONSEAM 0x0020
+#define ALIAS_XY_CLIP_MASK 0x000F
+
+#define ZISCALE ((float)0x8000)
+
+#define CACHE_SIZE 32 // used to align key data structures
+
+//typedef enum
+//{
+// mod_brush,
+// mod_sprite,
+// mod_alias,
+// mod_studio
+//} modtype_t;
+
+// must match definition in modelgen.h
+//#ifndef SYNCTYPE_T
+//#define SYNCTYPE_T
+//
+//typedef enum
+//{
+// ST_SYNC=0,
+// ST_RAND
+//} synctype_t;
+//
+//#endif
+
+//typedef struct
+//{
+// float mins[3], maxs[3];
+// float origin[3];
+// int headnode[MAX_MAP_HULLS];
+// int visleafs; // not including the solid leaf 0
+// int firstface, numfaces;
+//} dmodel_t;
+
+// plane_t structure
+//typedef struct mplane_s
+//{
+// vec3_t normal; // surface normal
+// float dist; // closest appoach to origin
+// byte type; // for texture axis selection and fast side tests
+// byte signbits; // signx + signy<<1 + signz<<1
+// byte pad[2];
+//} mplane_t;
+
+//typedef struct
+//{
+// vec3_t position;
+//} mvertex_t;
+
+//typedef struct
+//{
+// unsigned short v[2];
+// unsigned int cachededgeoffset;
+//} medge_t;
+
+//typedef struct texture_s
+//{
+// char name[16];
+// unsigned width, height;
+// int anim_total; // total tenths in sequence ( 0 = no)
+// int anim_min, anim_max; // time for this frame min <=time< max
+// struct texture_s *anim_next; // in the animation sequence
+// struct texture_s *alternate_anims; // bmodels in frame 1 use these
+// unsigned offsets[MIPLEVELS]; // four mip maps stored
+// unsigned paloffset;
+//} texture_t;
+
+//typedef struct
+//{
+// float vecs[2][4]; // [s/t] unit vectors in world space.
+// // [i][3] is the s/t offset relative to the origin.
+// // s or t = dot(3Dpoint,vecs[i])+vecs[i][3]
+// float mipadjust; // ?? mipmap limits for very small surfaces
+// texture_t *texture;
+// int flags; // sky or slime, no lightmap or 256 subdivision
+//} mtexinfo_t;
+
+//typedef struct mnode_s
+//{
+// // common with leaf
+// int contents; // 0, to differentiate from leafs
+// int visframe; // node needs to be traversed if current
+//
+// short minmaxs[6]; // for bounding box culling
+//
+// struct mnode_s *parent;
+//
+// // node specific
+// mplane_t *plane;
+// struct mnode_s *children[2];
+//
+// unsigned short firstsurface;
+// unsigned short numsurfaces;
+//} mnode_t;
+
+//typedef struct msurface_s msurface_t;
+//typedef struct decal_s decal_t;
+
+// JAY: Compress this as much as possible
+//struct decal_s
+//{
+// decal_t *pnext; // linked list for each surface
+// msurface_t *psurface; // Surface id for persistence / unlinking
+// short dx; // Offsets into surface texture (in texture coordinates, so we don't need floats)
+// short dy;
+// short texture; // Decal texture
+// byte scale; // Pixel scale
+// byte flags; // Decal flags
+//
+// short entityIndex; // Entity this is attached to
+//};
+
+//typedef struct mleaf_s
+//{
+// // common with node
+// int contents; // wil be a negative contents number
+// int visframe; // node needs to be traversed if current
+//
+// short minmaxs[6]; // for bounding box culling
+//
+// struct mnode_s *parent;
+//
+// // leaf specific
+// byte *compressed_vis;
+// struct efrag_s *efrags;
+//
+// msurface_t **firstmarksurface;
+// int nummarksurfaces;
+// int key; // BSP sequence number for leaf's contents
+// byte ambient_sound_level[NUM_AMBIENTS];
+//} mleaf_t;
+
+//struct msurface_s
+//{
+// int visframe; // should be drawn when node is crossed
+//
+// int dlightframe; // last frame the surface was checked by an animated light
+// int dlightbits; // dynamically generated. Indicates if the surface illumination
+// // is modified by an animated light.
+//
+// mplane_t *plane; // pointer to shared plane
+// int flags; // see SURF_ #defines
+//
+// int firstedge; // look up in model->surfedges[], negative numbers
+// int numedges; // are backwards edges
+//
+// // surface generation data
+// struct surfcache_s *cachespots[MIPLEVELS];
+//
+// short texturemins[2]; // smallest s/t position on the surface.
+// short extents[2]; // ?? s/t texture size, 1..256 for all non-sky surfaces
+//
+// mtexinfo_t *texinfo;
+//
+// // lighting info
+// byte styles[MAXLIGHTMAPS]; // index into d_lightstylevalue[] for animated lights
+// // no one surface can be effected by more than 4
+// // animated lights.
+// color24 *samples;
+//
+// decal_t *pdecals;
+//};
+
+//typedef struct
+//{
+// int planenum;
+// short children[2]; // negative numbers are contents
+//} dclipnode_t;
+
+//typedef struct hull_s
+//{
+// dclipnode_t *clipnodes;
+// mplane_t *planes;
+// int firstclipnode;
+// int lastclipnode;
+// vec3_t clip_mins;
+// vec3_t clip_maxs;
+//} hull_t;
+
+typedef struct cache_user_s
+{
+ void *data;
+} cache_user_t;
+
+//typedef struct model_s
+//{
+// char name[ MAX_MODEL_NAME ];
+// qboolean needload; // bmodels and sprites don't cache normally
+//
+// modtype_t type;
+// int numframes;
+// synctype_t synctype;
+//
+// int flags;
+//
+// //
+// // volume occupied by the model
+// //
+// vec3_t mins, maxs;
+// float radius;
+//
+// //
+// // brush model
+// //
+// int firstmodelsurface, nummodelsurfaces;
+//
+// int numsubmodels;
+// dmodel_t *submodels;
+//
+// int numplanes;
+// mplane_t *planes;
+//
+// int numleafs; // number of visible leafs, not counting 0
+// struct mleaf_s *leafs;
+//
+// int numvertexes;
+// mvertex_t *vertexes;
+//
+// int numedges;
+// medge_t *edges;
+//
+// int numnodes;
+// mnode_t *nodes;
+//
+// int numtexinfo;
+// mtexinfo_t *texinfo;
+//
+// int numsurfaces;
+// msurface_t *surfaces;
+//
+// int numsurfedges;
+// int *surfedges;
+//
+// int numclipnodes;
+// dclipnode_t *clipnodes;
+//
+// int nummarksurfaces;
+// msurface_t **marksurfaces;
+//
+// hull_t hulls[MAX_MAP_HULLS];
+//
+// int numtextures;
+// texture_t **textures;
+//
+// byte *visdata;
+//
+// color24 *lightdata;
+//
+// char *entities;
+//
+// //
+// // additional model data
+// //
+// cache_user_t cache; // only access through Mod_Extradata
+//
+//} model_t;
+
+//typedef vec_t vec4_t[4];
+
+typedef struct alight_s
+{
+ int ambientlight; // clip at 128
+ int shadelight; // clip at 192 - ambientlight
+ vec3_t color;
+ float *plightvec;
+} alight_t;
+
+typedef struct auxvert_s
+{
+ float fv[3]; // viewspace x, y
+} auxvert_t;
+
+#include "custom.h"
+
+//#define MAX_SCOREBOARDNAME 32
+
+// Defined in client.h differently
+//typedef struct player_info_s
+//{
+// // User id on server
+// int userid;
+//
+// // User info string
+// char userinfo[ MAX_INFO_STRING ];
+//
+// // Name
+// char name[ MAX_SCOREBOARDNAME ];
+//
+// // Spectator or not, unused
+// int spectator;
+//
+// int ping;
+// int packet_loss;
+//
+// // skin information
+// char model[MAX_QPATH];
+// int topcolor;
+// int bottomcolor;
+//
+// // last frame rendered
+// int renderframe;
+//
+// // Gait frame estimation
+// int gaitsequence;
+// float gaitframe;
+// float gaityaw;
+// vec3_t prevgaitorigin;
+//
+// customization_t customdata;
+//} player_info_t;
diff --git a/dep/hlsdk/common/commandline.cpp b/dep/hlsdk/common/commandline.cpp
new file mode 100644
index 0000000..5efa7d8
--- /dev/null
+++ b/dep/hlsdk/common/commandline.cpp
@@ -0,0 +1,384 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#include "precompiled.h"
+
+class CCommandLine: public ICommandLine {
+public:
+ CCommandLine();
+ virtual ~CCommandLine();
+
+ void CreateCmdLine(const char *commandline);
+ void CreateCmdLine(int argc, const char *argv[]);
+ const char *GetCmdLine() const;
+
+ // Check whether a particular parameter exists
+ const char *CheckParm(const char *psz, char **ppszValue = nullptr) const;
+ void RemoveParm(const char *pszParm);
+ void AppendParm(const char *pszParm, const char *pszValues);
+
+ void SetParm(const char *pszParm, const char *pszValues);
+ void SetParm(const char *pszParm, int iValue);
+
+ // When the commandline contains @name, it reads the parameters from that file
+ void LoadParametersFromFile(const char *&pSrc, char *&pDst, int maxDestLen);
+
+private:
+ // Copy of actual command line
+ char *m_pszCmdLine;
+};
+
+CCommandLine g_CmdLine;
+ICommandLine *cmdline = &g_CmdLine;
+
+ICommandLine *CommandLine()
+{
+ return &g_CmdLine;
+}
+
+CCommandLine::CCommandLine()
+{
+ m_pszCmdLine = nullptr;
+}
+
+CCommandLine::~CCommandLine()
+{
+ if (m_pszCmdLine)
+ {
+ delete [] m_pszCmdLine;
+ m_pszCmdLine = nullptr;
+ }
+}
+
+char *CopyString(const char *src)
+{
+ if (!src)
+ return nullptr;
+
+ char *out = (char *)new char[Q_strlen(src) + 1];
+ Q_strcpy(out, src);
+ return out;
+}
+
+// Creates a command line from the arguments passed in
+void CCommandLine::CreateCmdLine(int argc, const char *argv[])
+{
+ char cmdline[4096] = "";
+ const int MAX_CHARS = sizeof(cmdline) - 1;
+
+ for (int i = 0; i < argc; ++i)
+ {
+ if (Q_strchr(argv[i], ' '))
+ {
+ Q_strlcat(cmdline, "\"");
+ Q_strlcat(cmdline, argv[i]);
+ Q_strlcat(cmdline, "\"");
+ }
+ else
+ {
+ Q_strlcat(cmdline, argv[i]);
+ }
+
+ Q_strlcat(cmdline, " ");
+ }
+
+ cmdline[Q_strlen(cmdline)] = '\0';
+ CreateCmdLine(cmdline);
+}
+
+void CCommandLine::LoadParametersFromFile(const char *&pSrc, char *&pDst, int maxDestLen)
+{
+ // Suck out the file name
+ char szFileName[ MAX_PATH ];
+ char *pOut;
+ char *pDestStart = pDst;
+
+ // Skip the @ sign
+ pSrc++;
+ pOut = szFileName;
+
+ while (*pSrc && *pSrc != ' ')
+ {
+ *pOut++ = *pSrc++;
+#if 0
+ if ((pOut - szFileName) >= (MAX_PATH - 1))
+ break;
+#endif
+ }
+
+ *pOut = '\0';
+
+ // Skip the space after the file name
+ if (*pSrc)
+ pSrc++;
+
+ // Now read in parameters from file
+ FILE *fp = fopen(szFileName, "r");
+ if (fp)
+ {
+ char c;
+ c = (char)fgetc(fp);
+ while (c != EOF)
+ {
+ // Turn return characters into spaces
+ if (c == '\n')
+ c = ' ';
+
+ *pDst++ = c;
+
+#if 0
+ // Don't go past the end, and allow for our terminating space character AND a terminating null character.
+ if ((pDst - pDestStart) >= (maxDestLen - 2))
+ break;
+#endif
+
+ // Get the next character, if there are more
+ c = (char)fgetc(fp);
+ }
+
+ // Add a terminating space character
+ *pDst++ = ' ';
+
+ fclose(fp);
+ }
+ else
+ {
+ printf("Parameter file '%s' not found, skipping...", szFileName);
+ }
+}
+
+// Purpose: Create a command line from the passed in string
+// Note that if you pass in a @filename, then the routine will read settings from a file instead of the command line
+void CCommandLine::CreateCmdLine(const char *commandline)
+{
+ if (m_pszCmdLine)
+ {
+ delete[] m_pszCmdLine;
+ m_pszCmdLine = nullptr;
+ }
+
+ char szFull[4096];
+
+ char *pDst = szFull;
+ const char *pSrc = commandline;
+
+ bool allowAtSign = true;
+
+ while (*pSrc)
+ {
+ if (*pSrc == '@')
+ {
+ if (allowAtSign)
+ {
+ LoadParametersFromFile(pSrc, pDst, sizeof(szFull) - (pDst - szFull));
+ continue;
+ }
+ }
+
+ allowAtSign = isspace(*pSrc) != 0;
+
+#if 0
+ // Don't go past the end.
+ if ((pDst - szFull) >= (sizeof(szFull) - 1))
+ break;
+#endif
+ *pDst++ = *pSrc++;
+ }
+
+ *pDst = '\0';
+
+ int len = Q_strlen(szFull) + 1;
+ m_pszCmdLine = new char[len];
+ Q_memcpy(m_pszCmdLine, szFull, len);
+}
+
+// Purpose: Remove specified string ( and any args attached to it ) from command line
+void CCommandLine::RemoveParm(const char *pszParm)
+{
+ if (!m_pszCmdLine)
+ return;
+
+ if (!pszParm || *pszParm == '\0')
+ return;
+
+ // Search for first occurrence of pszParm
+ char *p, *found;
+ char *pnextparam;
+ int n;
+ int curlen;
+
+ p = m_pszCmdLine;
+ while (*p)
+ {
+ curlen = Q_strlen(p);
+ found = Q_strstr(p, pszParm);
+
+ if (!found)
+ break;
+
+ pnextparam = found + 1;
+ while (pnextparam && *pnextparam && (*pnextparam != '-') && (*pnextparam != '+'))
+ pnextparam++;
+
+ if (pnextparam && *pnextparam)
+ {
+ // We are either at the end of the string, or at the next param. Just chop out the current param.
+ // # of characters after this param.
+ n = curlen - (pnextparam - p);
+
+ Q_memcpy(found, pnextparam, n);
+ found[n] = '\0';
+ }
+ else
+ {
+ // Clear out rest of string.
+ n = pnextparam - found;
+ Q_memset(found, 0, n);
+ }
+ }
+
+ // Strip and trailing ' ' characters left over.
+ while (1)
+ {
+ int curpos = Q_strlen(m_pszCmdLine);
+ if (curpos == 0 || m_pszCmdLine[ curpos - 1 ] != ' ')
+ break;
+
+ m_pszCmdLine[curpos - 1] = '\0';
+ }
+}
+
+// Purpose: Append parameter and argument values to command line
+void CCommandLine::AppendParm(const char *pszParm, const char *pszValues)
+{
+ int nNewLength = 0;
+ char *pCmdString;
+
+ // Parameter.
+ nNewLength = Q_strlen(pszParm);
+
+ // Values + leading space character.
+ if (pszValues)
+ nNewLength += Q_strlen(pszValues) + 1;
+
+ // Terminal 0;
+ nNewLength++;
+
+ if (!m_pszCmdLine)
+ {
+ m_pszCmdLine = new char[ nNewLength ];
+ Q_strcpy(m_pszCmdLine, pszParm);
+ if (pszValues)
+ {
+ Q_strcat(m_pszCmdLine, " ");
+ Q_strcat(m_pszCmdLine, pszValues);
+ }
+
+ return;
+ }
+
+ // Remove any remnants from the current Cmd Line.
+ RemoveParm(pszParm);
+
+ nNewLength += Q_strlen(m_pszCmdLine) + 1 + 1;
+
+ pCmdString = new char[ nNewLength ];
+ Q_memset(pCmdString, 0, nNewLength);
+
+ Q_strcpy(pCmdString, m_pszCmdLine); // Copy old command line.
+ Q_strcat(pCmdString, " "); // Put in a space
+ Q_strcat(pCmdString, pszParm);
+
+ if (pszValues)
+ {
+ Q_strcat(pCmdString, " ");
+ Q_strcat(pCmdString, pszValues);
+ }
+
+ // Kill off the old one
+ delete[] m_pszCmdLine;
+
+ // Point at the new command line.
+ m_pszCmdLine = pCmdString;
+}
+
+void CCommandLine::SetParm(const char *pszParm, const char *pszValues)
+{
+ RemoveParm(pszParm);
+ AppendParm(pszParm, pszValues);
+}
+
+void CCommandLine::SetParm(const char *pszParm, int iValue)
+{
+ char buf[64];
+ Q_snprintf(buf, sizeof(buf), "%d", iValue);
+ SetParm(pszParm, buf);
+}
+
+// Purpose: Search for the parameter in the current commandline
+const char *CCommandLine::CheckParm(const char *psz, char **ppszValue) const
+{
+ static char sz[128] = "";
+
+ if (!m_pszCmdLine)
+ return nullptr;
+
+ if (ppszValue)
+ *ppszValue = nullptr;
+
+ char *pret = Q_strstr(m_pszCmdLine, psz);
+ if (!pret || !ppszValue)
+ return pret;
+
+ // find the next whitespace
+ char *p1 = pret;
+ do {
+ ++p1;
+ } while (*p1 != ' ' && *p1);
+
+ int i = 0;
+ char *p2 = p1 + 1;
+
+ do {
+ if (p2[i] == '\0' || p2[i] == ' ')
+ break;
+
+ sz[i] = p2[i];
+ i++;
+ } while (i < sizeof(sz));
+
+ sz[i] = '\0';
+ *ppszValue = sz;
+
+ return pret;
+}
+
+const char *CCommandLine::GetCmdLine() const
+{
+ return m_pszCmdLine;
+}
diff --git a/dep/hlsdk/common/con_nprint.h b/dep/hlsdk/common/con_nprint.h
new file mode 100644
index 0000000..b2a1888
--- /dev/null
+++ b/dep/hlsdk/common/con_nprint.h
@@ -0,0 +1,38 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined( CON_NPRINTH )
+#define CON_NPRINTH
+#ifdef _WIN32
+#pragma once
+#endif
+
+#ifdef __cplusplus
+extern "C" {
+#endif
+
+typedef struct con_nprint_s
+{
+ int index; // Row #
+ float time_to_live; // # of seconds before it dissappears
+ float color[ 3 ]; // RGB colors ( 0.0 -> 1.0 scale )
+} con_nprint_t;
+
+void Con_NPrintf( int idx, const char *fmt, ... );
+void Con_NXPrintf( struct con_nprint_s *info, const char *fmt, ... );
+#ifdef __cplusplus
+}
+#endif
+
+#endif
diff --git a/dep/hlsdk/common/const.h b/dep/hlsdk/common/const.h
new file mode 100644
index 0000000..5d71a78
--- /dev/null
+++ b/dep/hlsdk/common/const.h
@@ -0,0 +1,805 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+
+#ifndef CONST_H
+#define CONST_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+// Max # of clients allowed in a server.
+#define MAX_CLIENTS 32
+
+// How many bits to use to encode an edict.
+#define MAX_EDICT_BITS 11 // # of bits needed to represent max edicts
+// Max # of edicts in a level (2048)
+#define MAX_EDICTS (1<flags
+#define FL_FLY (1<<0) // Changes the SV_Movestep() behavior to not need to be on ground
+#define FL_SWIM (1<<1) // Changes the SV_Movestep() behavior to not need to be on ground (but stay in water)
+#define FL_CONVEYOR (1<<2)
+#define FL_CLIENT (1<<3)
+#define FL_INWATER (1<<4)
+#define FL_MONSTER (1<<5)
+#define FL_GODMODE (1<<6)
+#define FL_NOTARGET (1<<7)
+#define FL_SKIPLOCALHOST (1<<8) // Don't send entity to local host, it's predicting this entity itself
+#define FL_ONGROUND (1<<9) // At rest / on the ground
+#define FL_PARTIALGROUND (1<<10) // not all corners are valid
+#define FL_WATERJUMP (1<<11) // player jumping out of water
+#define FL_FROZEN (1<<12) // Player is frozen for 3rd person camera
+#define FL_FAKECLIENT (1<<13) // JAC: fake client, simulated server side; don't send network messages to them
+#define FL_DUCKING (1<<14) // Player flag -- Player is fully crouched
+#define FL_FLOAT (1<<15) // Apply floating force to this entity when in water
+#define FL_GRAPHED (1<<16) // worldgraph has this ent listed as something that blocks a connection
+
+// UNDONE: Do we need these?
+#define FL_IMMUNE_WATER (1<<17)
+#define FL_IMMUNE_SLIME (1<<18)
+#define FL_IMMUNE_LAVA (1<<19)
+
+#define FL_PROXY (1<<20) // This is a spectator proxy
+#define FL_ALWAYSTHINK (1<<21) // Brush model flag -- call think every frame regardless of nextthink - ltime (for constantly changing velocity/path)
+#define FL_BASEVELOCITY (1<<22) // Base velocity has been applied this frame (used to convert base velocity into momentum)
+#define FL_MONSTERCLIP (1<<23) // Only collide in with monsters who have FL_MONSTERCLIP set
+#define FL_ONTRAIN (1<<24) // Player is _controlling_ a train, so movement commands should be ignored on client during prediction.
+#define FL_WORLDBRUSH (1<<25) // Not moveable/removeable brush entity (really part of the world, but represented as an entity for transparency or something)
+#define FL_SPECTATOR (1<<26) // This client is a spectator, don't run touch functions, etc.
+#define FL_CUSTOMENTITY (1<<29) // This is a custom entity
+#define FL_KILLME (1<<30) // This entity is marked for death -- This allows the engine to kill ents at the appropriate time
+#define FL_DORMANT (1<<31) // Entity is dormant, no updates to client
+
+// SV_EmitSound2 flags
+#define SND_EMIT2_NOPAS (1<<0) // never to do check PAS
+#define SND_EMIT2_INVOKER (1<<1) // do not send to the client invoker
+
+// Engine edict->spawnflags
+#define SF_NOTINDEATHMATCH 0x0800 // Do not spawn when deathmatch and loading entities from a file
+
+
+// Goes into globalvars_t.trace_flags
+#define FTRACE_SIMPLEBOX (1<<0) // Traceline with a simple box
+
+
+// walkmove modes
+#define WALKMOVE_NORMAL 0 // normal walkmove
+#define WALKMOVE_WORLDONLY 1 // doesn't hit ANY entities, no matter what the solid type
+#define WALKMOVE_CHECKONLY 2 // move, but don't touch triggers
+
+// edict->movetype values
+#define MOVETYPE_NONE 0 // never moves
+//#define MOVETYPE_ANGLENOCLIP 1
+//#define MOVETYPE_ANGLECLIP 2
+#define MOVETYPE_WALK 3 // Player only - moving on the ground
+#define MOVETYPE_STEP 4 // gravity, special edge handling -- monsters use this
+#define MOVETYPE_FLY 5 // No gravity, but still collides with stuff
+#define MOVETYPE_TOSS 6 // gravity/collisions
+#define MOVETYPE_PUSH 7 // no clip to world, push and crush
+#define MOVETYPE_NOCLIP 8 // No gravity, no collisions, still do velocity/avelocity
+#define MOVETYPE_FLYMISSILE 9 // extra size to monsters
+#define MOVETYPE_BOUNCE 10 // Just like Toss, but reflect velocity when contacting surfaces
+#define MOVETYPE_BOUNCEMISSILE 11 // bounce w/o gravity
+#define MOVETYPE_FOLLOW 12 // track movement of aiment
+#define MOVETYPE_PUSHSTEP 13 // BSP model that needs physics/world collisions (uses nearest hull for world collision)
+
+// edict->solid values
+// NOTE: Some movetypes will cause collisions independent of SOLID_NOT/SOLID_TRIGGER when the entity moves
+// SOLID only effects OTHER entities colliding with this one when they move - UGH!
+#define SOLID_NOT 0 // no interaction with other objects
+#define SOLID_TRIGGER 1 // touch on edge, but not blocking
+#define SOLID_BBOX 2 // touch on edge, block
+#define SOLID_SLIDEBOX 3 // touch on edge, but not an onground
+#define SOLID_BSP 4 // bsp clip, touch on edge, block
+
+// edict->deadflag values
+#define DEAD_NO 0 // alive
+#define DEAD_DYING 1 // playing death animation or still falling off of a ledge waiting to hit ground
+#define DEAD_DEAD 2 // dead. lying still.
+#define DEAD_RESPAWNABLE 3
+#define DEAD_DISCARDBODY 4
+
+#define DAMAGE_NO 0
+#define DAMAGE_YES 1
+#define DAMAGE_AIM 2
+
+// entity effects
+#define EF_BRIGHTFIELD 1 // swirling cloud of particles
+#define EF_MUZZLEFLASH 2 // single frame ELIGHT on entity attachment 0
+#define EF_BRIGHTLIGHT 4 // DLIGHT centered at entity origin
+#define EF_DIMLIGHT 8 // player flashlight
+#define EF_INVLIGHT 16 // get lighting from ceiling
+#define EF_NOINTERP 32 // don't interpolate the next frame
+#define EF_LIGHT 64 // rocket flare glow sprite
+#define EF_NODRAW 128 // don't draw entity
+#define EF_NIGHTVISION 256 // player nightvision
+#define EF_SNIPERLASER 512 // sniper laser effect
+#define EF_FIBERCAMERA 1024// fiber camera
+
+
+// entity flags
+#define EFLAG_SLERP 1 // do studio interpolation of this entity
+
+//
+// temp entity events
+//
+#define TE_BEAMPOINTS 0 // beam effect between two points
+// coord coord coord (start position)
+// coord coord coord (end position)
+// short (sprite index)
+// byte (starting frame)
+// byte (frame rate in 0.1's)
+// byte (life in 0.1's)
+// byte (line width in 0.1's)
+// byte (noise amplitude in 0.01's)
+// byte,byte,byte (color)
+// byte (brightness)
+// byte (scroll speed in 0.1's)
+
+#define TE_BEAMENTPOINT 1 // beam effect between point and entity
+// short (start entity)
+// coord coord coord (end position)
+// short (sprite index)
+// byte (starting frame)
+// byte (frame rate in 0.1's)
+// byte (life in 0.1's)
+// byte (line width in 0.1's)
+// byte (noise amplitude in 0.01's)
+// byte,byte,byte (color)
+// byte (brightness)
+// byte (scroll speed in 0.1's)
+
+#define TE_GUNSHOT 2 // particle effect plus ricochet sound
+// coord coord coord (position)
+
+#define TE_EXPLOSION 3 // additive sprite, 2 dynamic lights, flickering particles, explosion sound, move vertically 8 pps
+// coord coord coord (position)
+// short (sprite index)
+// byte (scale in 0.1's)
+// byte (framerate)
+// byte (flags)
+//
+// The Explosion effect has some flags to control performance/aesthetic features:
+#define TE_EXPLFLAG_NONE 0 // all flags clear makes default Half-Life explosion
+#define TE_EXPLFLAG_NOADDITIVE 1 // sprite will be drawn opaque (ensure that the sprite you send is a non-additive sprite)
+#define TE_EXPLFLAG_NODLIGHTS 2 // do not render dynamic lights
+#define TE_EXPLFLAG_NOSOUND 4 // do not play client explosion sound
+#define TE_EXPLFLAG_NOPARTICLES 8 // do not draw particles
+
+
+#define TE_TAREXPLOSION 4 // Quake1 "tarbaby" explosion with sound
+// coord coord coord (position)
+
+#define TE_SMOKE 5 // alphablend sprite, move vertically 30 pps
+// coord coord coord (position)
+// short (sprite index)
+// byte (scale in 0.1's)
+// byte (framerate)
+
+#define TE_TRACER 6 // tracer effect from point to point
+// coord, coord, coord (start)
+// coord, coord, coord (end)
+
+#define TE_LIGHTNING 7 // TE_BEAMPOINTS with simplified parameters
+// coord, coord, coord (start)
+// coord, coord, coord (end)
+// byte (life in 0.1's)
+// byte (width in 0.1's)
+// byte (amplitude in 0.01's)
+// short (sprite model index)
+
+#define TE_BEAMENTS 8
+// short (start entity)
+// short (end entity)
+// short (sprite index)
+// byte (starting frame)
+// byte (frame rate in 0.1's)
+// byte (life in 0.1's)
+// byte (line width in 0.1's)
+// byte (noise amplitude in 0.01's)
+// byte,byte,byte (color)
+// byte (brightness)
+// byte (scroll speed in 0.1's)
+
+#define TE_SPARKS 9 // 8 random tracers with gravity, ricochet sprite
+// coord coord coord (position)
+
+#define TE_LAVASPLASH 10 // Quake1 lava splash
+// coord coord coord (position)
+
+#define TE_TELEPORT 11 // Quake1 teleport splash
+// coord coord coord (position)
+
+#define TE_EXPLOSION2 12 // Quake1 colormaped (base palette) particle explosion with sound
+// coord coord coord (position)
+// byte (starting color)
+// byte (num colors)
+
+#define TE_BSPDECAL 13 // Decal from the .BSP file
+// coord, coord, coord (x,y,z), decal position (center of texture in world)
+// short (texture index of precached decal texture name)
+// short (entity index)
+// [optional - only included if previous short is non-zero (not the world)] short (index of model of above entity)
+
+#define TE_IMPLOSION 14 // tracers moving toward a point
+// coord, coord, coord (position)
+// byte (radius)
+// byte (count)
+// byte (life in 0.1's)
+
+#define TE_SPRITETRAIL 15 // line of moving glow sprites with gravity, fadeout, and collisions
+// coord, coord, coord (start)
+// coord, coord, coord (end)
+// short (sprite index)
+// byte (count)
+// byte (life in 0.1's)
+// byte (scale in 0.1's)
+// byte (velocity along vector in 10's)
+// byte (randomness of velocity in 10's)
+
+#define TE_BEAM 16 // obsolete
+
+#define TE_SPRITE 17 // additive sprite, plays 1 cycle
+// coord, coord, coord (position)
+// short (sprite index)
+// byte (scale in 0.1's)
+// byte (brightness)
+
+#define TE_BEAMSPRITE 18 // A beam with a sprite at the end
+// coord, coord, coord (start position)
+// coord, coord, coord (end position)
+// short (beam sprite index)
+// short (end sprite index)
+
+#define TE_BEAMTORUS 19 // screen aligned beam ring, expands to max radius over lifetime
+// coord coord coord (center position)
+// coord coord coord (axis and radius)
+// short (sprite index)
+// byte (starting frame)
+// byte (frame rate in 0.1's)
+// byte (life in 0.1's)
+// byte (line width in 0.1's)
+// byte (noise amplitude in 0.01's)
+// byte,byte,byte (color)
+// byte (brightness)
+// byte (scroll speed in 0.1's)
+
+#define TE_BEAMDISK 20 // disk that expands to max radius over lifetime
+// coord coord coord (center position)
+// coord coord coord (axis and radius)
+// short (sprite index)
+// byte (starting frame)
+// byte (frame rate in 0.1's)
+// byte (life in 0.1's)
+// byte (line width in 0.1's)
+// byte (noise amplitude in 0.01's)
+// byte,byte,byte (color)
+// byte (brightness)
+// byte (scroll speed in 0.1's)
+
+#define TE_BEAMCYLINDER 21 // cylinder that expands to max radius over lifetime
+// coord coord coord (center position)
+// coord coord coord (axis and radius)
+// short (sprite index)
+// byte (starting frame)
+// byte (frame rate in 0.1's)
+// byte (life in 0.1's)
+// byte (line width in 0.1's)
+// byte (noise amplitude in 0.01's)
+// byte,byte,byte (color)
+// byte (brightness)
+// byte (scroll speed in 0.1's)
+
+#define TE_BEAMFOLLOW 22 // create a line of decaying beam segments until entity stops moving
+// short (entity:attachment to follow)
+// short (sprite index)
+// byte (life in 0.1's)
+// byte (line width in 0.1's)
+// byte,byte,byte (color)
+// byte (brightness)
+
+#define TE_GLOWSPRITE 23
+// coord, coord, coord (pos) short (model index) byte (scale / 10)
+
+#define TE_BEAMRING 24 // connect a beam ring to two entities
+// short (start entity)
+// short (end entity)
+// short (sprite index)
+// byte (starting frame)
+// byte (frame rate in 0.1's)
+// byte (life in 0.1's)
+// byte (line width in 0.1's)
+// byte (noise amplitude in 0.01's)
+// byte,byte,byte (color)
+// byte (brightness)
+// byte (scroll speed in 0.1's)
+
+#define TE_STREAK_SPLASH 25 // oriented shower of tracers
+// coord coord coord (start position)
+// coord coord coord (direction vector)
+// byte (color)
+// short (count)
+// short (base speed)
+// short (ramdon velocity)
+
+#define TE_BEAMHOSE 26 // obsolete
+
+#define TE_DLIGHT 27 // dynamic light, effect world, minor entity effect
+// coord, coord, coord (pos)
+// byte (radius in 10's)
+// byte byte byte (color)
+// byte (brightness)
+// byte (life in 10's)
+// byte (decay rate in 10's)
+
+#define TE_ELIGHT 28 // point entity light, no world effect
+// short (entity:attachment to follow)
+// coord coord coord (initial position)
+// coord (radius)
+// byte byte byte (color)
+// byte (life in 0.1's)
+// coord (decay rate)
+
+#define TE_TEXTMESSAGE 29
+// short 1.2.13 x (-1 = center)
+// short 1.2.13 y (-1 = center)
+// byte Effect 0 = fade in/fade out
+ // 1 is flickery credits
+ // 2 is write out (training room)
+
+// 4 bytes r,g,b,a color1 (text color)
+// 4 bytes r,g,b,a color2 (effect color)
+// ushort 8.8 fadein time
+// ushort 8.8 fadeout time
+// ushort 8.8 hold time
+// optional ushort 8.8 fxtime (time the highlight lags behing the leading text in effect 2)
+// string text message (512 chars max sz string)
+#define TE_LINE 30
+// coord, coord, coord startpos
+// coord, coord, coord endpos
+// short life in 0.1 s
+// 3 bytes r, g, b
+
+#define TE_BOX 31
+// coord, coord, coord boxmins
+// coord, coord, coord boxmaxs
+// short life in 0.1 s
+// 3 bytes r, g, b
+
+#define TE_KILLBEAM 99 // kill all beams attached to entity
+// short (entity)
+
+#define TE_LARGEFUNNEL 100
+// coord coord coord (funnel position)
+// short (sprite index)
+// short (flags)
+
+#define TE_BLOODSTREAM 101 // particle spray
+// coord coord coord (start position)
+// coord coord coord (spray vector)
+// byte (color)
+// byte (speed)
+
+#define TE_SHOWLINE 102 // line of particles every 5 units, dies in 30 seconds
+// coord coord coord (start position)
+// coord coord coord (end position)
+
+#define TE_BLOOD 103 // particle spray
+// coord coord coord (start position)
+// coord coord coord (spray vector)
+// byte (color)
+// byte (speed)
+
+#define TE_DECAL 104 // Decal applied to a brush entity (not the world)
+// coord, coord, coord (x,y,z), decal position (center of texture in world)
+// byte (texture index of precached decal texture name)
+// short (entity index)
+
+#define TE_FIZZ 105 // create alpha sprites inside of entity, float upwards
+// short (entity)
+// short (sprite index)
+// byte (density)
+
+#define TE_MODEL 106 // create a moving model that bounces and makes a sound when it hits
+// coord, coord, coord (position)
+// coord, coord, coord (velocity)
+// angle (initial yaw)
+// short (model index)
+// byte (bounce sound type)
+// byte (life in 0.1's)
+
+#define TE_EXPLODEMODEL 107 // spherical shower of models, picks from set
+// coord, coord, coord (origin)
+// coord (velocity)
+// short (model index)
+// short (count)
+// byte (life in 0.1's)
+
+#define TE_BREAKMODEL 108 // box of models or sprites
+// coord, coord, coord (position)
+// coord, coord, coord (size)
+// coord, coord, coord (velocity)
+// byte (random velocity in 10's)
+// short (sprite or model index)
+// byte (count)
+// byte (life in 0.1 secs)
+// byte (flags)
+
+#define TE_GUNSHOTDECAL 109 // decal and ricochet sound
+// coord, coord, coord (position)
+// short (entity index???)
+// byte (decal???)
+
+#define TE_SPRITE_SPRAY 110 // spay of alpha sprites
+// coord, coord, coord (position)
+// coord, coord, coord (velocity)
+// short (sprite index)
+// byte (count)
+// byte (speed)
+// byte (noise)
+
+#define TE_ARMOR_RICOCHET 111 // quick spark sprite, client ricochet sound.
+// coord, coord, coord (position)
+// byte (scale in 0.1's)
+
+#define TE_PLAYERDECAL 112 // ???
+// byte (playerindex)
+// coord, coord, coord (position)
+// short (entity???)
+// byte (decal number???)
+// [optional] short (model index???)
+
+#define TE_BUBBLES 113 // create alpha sprites inside of box, float upwards
+// coord, coord, coord (min start position)
+// coord, coord, coord (max start position)
+// coord (float height)
+// short (model index)
+// byte (count)
+// coord (speed)
+
+#define TE_BUBBLETRAIL 114 // create alpha sprites along a line, float upwards
+// coord, coord, coord (min start position)
+// coord, coord, coord (max start position)
+// coord (float height)
+// short (model index)
+// byte (count)
+// coord (speed)
+
+#define TE_BLOODSPRITE 115 // spray of opaque sprite1's that fall, single sprite2 for 1..2 secs (this is a high-priority tent)
+// coord, coord, coord (position)
+// short (sprite1 index)
+// short (sprite2 index)
+// byte (color)
+// byte (scale)
+
+#define TE_WORLDDECAL 116 // Decal applied to the world brush
+// coord, coord, coord (x,y,z), decal position (center of texture in world)
+// byte (texture index of precached decal texture name)
+
+#define TE_WORLDDECALHIGH 117 // Decal (with texture index > 256) applied to world brush
+// coord, coord, coord (x,y,z), decal position (center of texture in world)
+// byte (texture index of precached decal texture name - 256)
+
+#define TE_DECALHIGH 118 // Same as TE_DECAL, but the texture index was greater than 256
+// coord, coord, coord (x,y,z), decal position (center of texture in world)
+// byte (texture index of precached decal texture name - 256)
+// short (entity index)
+
+#define TE_PROJECTILE 119 // Makes a projectile (like a nail) (this is a high-priority tent)
+// coord, coord, coord (position)
+// coord, coord, coord (velocity)
+// short (modelindex)
+// byte (life)
+// byte (owner) projectile won't collide with owner (if owner == 0, projectile will hit any client).
+
+#define TE_SPRAY 120 // Throws a shower of sprites or models
+// coord, coord, coord (position)
+// coord, coord, coord (direction)
+// short (modelindex)
+// byte (count)
+// byte (speed)
+// byte (noise)
+// byte (rendermode)
+
+#define TE_PLAYERSPRITES 121 // sprites emit from a player's bounding box (ONLY use for players!)
+// byte (playernum)
+// short (sprite modelindex)
+// byte (count)
+// byte (variance) (0 = no variance in size) (10 = 10% variance in size)
+
+#define TE_PARTICLEBURST 122 // very similar to lavasplash.
+// coord (origin)
+// short (radius)
+// byte (particle color)
+// byte (duration * 10) (will be randomized a bit)
+
+#define TE_FIREFIELD 123 // makes a field of fire.
+// coord (origin)
+// short (radius) (fire is made in a square around origin. -radius, -radius to radius, radius)
+// short (modelindex)
+// byte (count)
+// byte (flags)
+// byte (duration (in seconds) * 10) (will be randomized a bit)
+//
+// to keep network traffic low, this message has associated flags that fit into a byte:
+#define TEFIRE_FLAG_ALLFLOAT 1 // all sprites will drift upwards as they animate
+#define TEFIRE_FLAG_SOMEFLOAT 2 // some of the sprites will drift upwards. (50% chance)
+#define TEFIRE_FLAG_LOOP 4 // if set, sprite plays at 15 fps, otherwise plays at whatever rate stretches the animation over the sprite's duration.
+#define TEFIRE_FLAG_ALPHA 8 // if set, sprite is rendered alpha blended at 50% else, opaque
+#define TEFIRE_FLAG_PLANAR 16 // if set, all fire sprites have same initial Z instead of randomly filling a cube.
+#define TEFIRE_FLAG_ADDITIVE 32 // if set, sprite is rendered non-opaque with additive
+
+#define TE_PLAYERATTACHMENT 124 // attaches a TENT to a player (this is a high-priority tent)
+// byte (entity index of player)
+// coord (vertical offset) ( attachment origin.z = player origin.z + vertical offset )
+// short (model index)
+// short (life * 10 );
+
+#define TE_KILLPLAYERATTACHMENTS 125 // will expire all TENTS attached to a player.
+// byte (entity index of player)
+
+#define TE_MULTIGUNSHOT 126 // much more compact shotgun message
+// This message is used to make a client approximate a 'spray' of gunfire.
+// Any weapon that fires more than one bullet per frame and fires in a bit of a spread is
+// a good candidate for MULTIGUNSHOT use. (shotguns)
+//
+// NOTE: This effect makes the client do traces for each bullet, these client traces ignore
+// entities that have studio models.Traces are 4096 long.
+//
+// coord (origin)
+// coord (origin)
+// coord (origin)
+// coord (direction)
+// coord (direction)
+// coord (direction)
+// coord (x noise * 100)
+// coord (y noise * 100)
+// byte (count)
+// byte (bullethole decal texture index)
+
+#define TE_USERTRACER 127 // larger message than the standard tracer, but allows some customization.
+// coord (origin)
+// coord (origin)
+// coord (origin)
+// coord (velocity)
+// coord (velocity)
+// coord (velocity)
+// byte ( life * 10 )
+// byte ( color ) this is an index into an array of color vectors in the engine. (0 - )
+// byte ( length * 10 )
+
+
+
+#define MSG_BROADCAST 0 // unreliable to all
+#define MSG_ONE 1 // reliable to one (msg_entity)
+#define MSG_ALL 2 // reliable to all
+#define MSG_INIT 3 // write to the init string
+#define MSG_PVS 4 // Ents in PVS of org
+#define MSG_PAS 5 // Ents in PAS of org
+#define MSG_PVS_R 6 // Reliable to PVS
+#define MSG_PAS_R 7 // Reliable to PAS
+#define MSG_ONE_UNRELIABLE 8 // Send to one client, but don't put in reliable stream, put in unreliable datagram ( could be dropped )
+#define MSG_SPEC 9 // Sends to all spectator proxies
+
+// contents of a spot in the world
+#define CONTENTS_EMPTY -1
+#define CONTENTS_SOLID -2
+#define CONTENTS_WATER -3
+#define CONTENTS_SLIME -4
+#define CONTENTS_LAVA -5
+#define CONTENTS_SKY -6
+/* These additional contents constants are defined in bspfile.h
+#define CONTENTS_ORIGIN -7 // removed at csg time
+#define CONTENTS_CLIP -8 // changed to contents_solid
+#define CONTENTS_CURRENT_0 -9
+#define CONTENTS_CURRENT_90 -10
+#define CONTENTS_CURRENT_180 -11
+#define CONTENTS_CURRENT_270 -12
+#define CONTENTS_CURRENT_UP -13
+#define CONTENTS_CURRENT_DOWN -14
+
+#define CONTENTS_TRANSLUCENT -15
+*/
+#define CONTENTS_LADDER -16
+
+#define CONTENT_FLYFIELD -17
+#define CONTENT_GRAVITY_FLYFIELD -18
+#define CONTENT_FOG -19
+
+#define CONTENT_EMPTY -1
+#define CONTENT_SOLID -2
+#define CONTENT_WATER -3
+#define CONTENT_SLIME -4
+#define CONTENT_LAVA -5
+#define CONTENT_SKY -6
+
+// channels
+#define CHAN_AUTO 0
+#define CHAN_WEAPON 1
+#define CHAN_VOICE 2
+#define CHAN_ITEM 3
+#define CHAN_BODY 4
+#define CHAN_STREAM 5 // allocate stream channel from the static or dynamic area
+#define CHAN_STATIC 6 // allocate channel from the static area
+#define CHAN_NETWORKVOICE_BASE 7 // voice data coming across the network
+#define CHAN_NETWORKVOICE_END 500 // network voice data reserves slots (CHAN_NETWORKVOICE_BASE through CHAN_NETWORKVOICE_END).
+#define CHAN_BOT 501 // channel used for bot chatter.
+
+// attenuation values
+#define ATTN_NONE 0
+#define ATTN_NORM (float)0.8
+#define ATTN_IDLE (float)2
+#define ATTN_STATIC (float)1.25
+
+// pitch values
+#define PITCH_NORM 100 // non-pitch shifted
+#define PITCH_LOW 95 // other values are possible - 0-255, where 255 is very high
+#define PITCH_HIGH 120
+
+// volume values
+#define VOL_NORM 1.0
+
+// plats
+#define PLAT_LOW_TRIGGER 1
+
+// Trains
+#define SF_TRAIN_WAIT_RETRIGGER 1
+#define SF_TRAIN_START_ON 4 // Train is initially moving
+#define SF_TRAIN_PASSABLE 8 // Train is not solid -- used to make water trains
+
+// buttons
+#ifndef IN_BUTTONS_H
+#include "in_buttons.h"
+#endif
+
+// Break Model Defines
+
+#define BREAK_TYPEMASK 0x4F
+#define BREAK_GLASS 0x01
+#define BREAK_METAL 0x02
+#define BREAK_FLESH 0x04
+#define BREAK_WOOD 0x08
+
+#define BREAK_SMOKE 0x10
+#define BREAK_TRANS 0x20
+#define BREAK_CONCRETE 0x40
+#define BREAK_2 0x80
+
+// Colliding temp entity sounds
+
+#define BOUNCE_GLASS BREAK_GLASS
+#define BOUNCE_METAL BREAK_METAL
+#define BOUNCE_FLESH BREAK_FLESH
+#define BOUNCE_WOOD BREAK_WOOD
+#define BOUNCE_SHRAP 0x10
+#define BOUNCE_SHELL 0x20
+#define BOUNCE_CONCRETE BREAK_CONCRETE
+#define BOUNCE_SHOTSHELL 0x80
+
+// Temp entity bounce sound types
+#define TE_BOUNCE_NULL 0
+#define TE_BOUNCE_SHELL 1
+#define TE_BOUNCE_SHOTSHELL 2
+
+// Rendering constants
+enum
+{
+ kRenderNormal, // src
+ kRenderTransColor, // c*a+dest*(1-a)
+ kRenderTransTexture, // src*a+dest*(1-a)
+ kRenderGlow, // src*a+dest -- No Z buffer checks
+ kRenderTransAlpha, // src*srca+dest*(1-srca)
+ kRenderTransAdd, // src*a+dest
+};
+
+enum
+{
+ kRenderFxNone = 0,
+ kRenderFxPulseSlow,
+ kRenderFxPulseFast,
+ kRenderFxPulseSlowWide,
+ kRenderFxPulseFastWide,
+ kRenderFxFadeSlow,
+ kRenderFxFadeFast,
+ kRenderFxSolidSlow,
+ kRenderFxSolidFast,
+ kRenderFxStrobeSlow,
+ kRenderFxStrobeFast,
+ kRenderFxStrobeFaster,
+ kRenderFxFlickerSlow,
+ kRenderFxFlickerFast,
+ kRenderFxNoDissipation,
+ kRenderFxDistort, // Distort/scale/translate flicker
+ kRenderFxHologram, // kRenderFxDistort + distance fade
+ kRenderFxDeadPlayer, // kRenderAmt is the player index
+ kRenderFxExplode, // Scale up really big!
+ kRenderFxGlowShell, // Glowing Shell
+ kRenderFxClampMinScale, // Keep this sprite from getting very small (SPRITES only!)
+ kRenderFxLightMultiplier, //CTM !!!CZERO added to tell the studiorender that the value in iuser2 is a lightmultiplier
+};
+
+
+typedef unsigned int func_t;
+
+typedef unsigned char byte;
+typedef unsigned short word;
+#define _DEF_BYTE_
+
+#undef true
+#undef false
+
+#ifndef __cplusplus
+typedef enum {false, true} qboolean;
+#else
+typedef int qboolean;
+#endif
+
+typedef struct
+{
+ byte r, g, b;
+} color24;
+
+typedef struct
+{
+ unsigned r, g, b, a;
+} colorVec;
+
+#ifdef _WIN32
+#pragma pack(push,2)
+#endif
+
+typedef struct
+{
+ unsigned short r, g, b, a;
+} PackedColorVec;
+
+#ifdef _WIN32
+#pragma pack(pop)
+#endif
+typedef struct link_s
+{
+ struct link_s *prev, *next;
+} link_t;
+
+typedef struct edict_s edict_t;
+
+typedef struct
+{
+ vec3_t normal;
+ float dist;
+} plane_t;
+
+typedef struct
+{
+ qboolean allsolid; // if true, plane is not valid
+ qboolean startsolid; // if true, the initial point was in a solid area
+ qboolean inopen, inwater;
+ float fraction; // time completed, 1.0 = didn't hit anything
+ vec3_t endpos; // final position
+ plane_t plane; // surface normal at impact
+ edict_t * ent; // entity the surface is on
+ int hitgroup; // 0 == generic, non zero is specific body part
+} trace_t;
+
+#endif // CONST_H
diff --git a/dep/hlsdk/common/crc.h b/dep/hlsdk/common/crc.h
new file mode 100644
index 0000000..f7c6bb2
--- /dev/null
+++ b/dep/hlsdk/common/crc.h
@@ -0,0 +1,38 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+/* crc.h */
+#pragma once
+
+#include "quakedef.h"
+
+typedef unsigned int CRC32_t;
+
+#ifdef __cplusplus
+extern "C"
+{
+#endif
+
+void CRC32_Init(CRC32_t *pulCRC);
+CRC32_t CRC32_Final(CRC32_t pulCRC);
+void CRC32_ProcessByte(CRC32_t *pulCRC, unsigned char ch);
+void CRC32_ProcessBuffer(CRC32_t *pulCRC, void *pBuffer, int nBuffer);
+BOOL CRC_File(CRC32_t *crcvalue, char *pszFileName);
+
+#ifdef __cplusplus
+}
+#endif
+
+byte COM_BlockSequenceCRCByte(byte *base, int length, int sequence);
+int CRC_MapFile(CRC32_t *crcvalue, char *pszFileName);
diff --git a/dep/hlsdk/common/cvardef.h b/dep/hlsdk/common/cvardef.h
new file mode 100644
index 0000000..7bba22b
--- /dev/null
+++ b/dep/hlsdk/common/cvardef.h
@@ -0,0 +1,50 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+
+#ifndef CVARDEF_H
+#define CVARDEF_H
+
+#define FCVAR_ARCHIVE (1<<0) // set to cause it to be saved to vars.rc
+#define FCVAR_USERINFO (1<<1) // changes the client's info string
+#define FCVAR_SERVER (1<<2) // notifies players when changed
+#define FCVAR_EXTDLL (1<<3) // defined by external DLL
+#define FCVAR_CLIENTDLL (1<<4) // defined by the client dll
+#define FCVAR_PROTECTED (1<<5) // It's a server cvar, but we don't send the data since it's a password, etc. Sends 1 if it's not bland/zero, 0 otherwise as value
+#define FCVAR_SPONLY (1<<6) // This cvar cannot be changed by clients connected to a multiplayer server.
+#define FCVAR_PRINTABLEONLY (1<<7) // This cvar's string cannot contain unprintable characters ( e.g., used for player name etc ).
+#define FCVAR_UNLOGGED (1<<8) // If this is a FCVAR_SERVER, don't log changes to the log file / console if we are creating a log
+#define FCVAR_NOEXTRAWHITEPACE (1<<9) // strip trailing/leading white space from this cvar
+
+typedef struct cvar_s
+{
+ const char *name;
+ char *string;
+ int flags;
+ float value;
+ struct cvar_s *next;
+} cvar_t;
+
+using cvar_callback_t = void (*)(const char *pszNewValue);
+
+struct cvar_listener_t
+{
+ cvar_listener_t(const char *var_name, cvar_callback_t handler) :
+ func(handler), name(var_name) {}
+
+ cvar_callback_t func;
+ const char *name;
+};
+
+#endif // CVARDEF_H
diff --git a/dep/hlsdk/common/demo_api.h b/dep/hlsdk/common/demo_api.h
new file mode 100644
index 0000000..8284a81
--- /dev/null
+++ b/dep/hlsdk/common/demo_api.h
@@ -0,0 +1,31 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined ( DEMO_APIH )
+#define DEMO_APIH
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef struct demo_api_s
+{
+ int ( *IsRecording ) ( void );
+ int ( *IsPlayingback ) ( void );
+ int ( *IsTimeDemo ) ( void );
+ void ( *WriteBuffer ) ( int size, unsigned char *buffer );
+} demo_api_t;
+
+extern demo_api_t demoapi;
+
+#endif
diff --git a/dep/hlsdk/common/director_cmds.h b/dep/hlsdk/common/director_cmds.h
new file mode 100644
index 0000000..4c8fdd5
--- /dev/null
+++ b/dep/hlsdk/common/director_cmds.h
@@ -0,0 +1,38 @@
+//========= Copyright © 1996-2002, Valve LLC, All rights reserved. ============
+//
+// Purpose:
+//
+// $NoKeywords: $
+//=============================================================================
+
+// director_cmds.h
+// sub commands for svc_director
+
+#define DRC_ACTIVE 0 // tells client that he's an spectator and will get director command
+#define DRC_STATUS 1 // send status infos about proxy
+#define DRC_CAMERA 2 // set the actual director camera position
+#define DRC_EVENT 3 // informs the dircetor about ann important game event
+
+
+#define DRC_FLAG_PRIO_MASK 0x0F // priorities between 0 and 15 (15 most important)
+#define DRC_FLAG_SIDE (1<<4)
+#define DRC_FLAG_DRAMATIC (1<<5)
+
+
+
+// commands of the director API function CallDirectorProc(...)
+
+#define DRCAPI_NOP 0 // no operation
+#define DRCAPI_ACTIVE 1 // de/acivates director mode in engine
+#define DRCAPI_STATUS 2 // request proxy information
+#define DRCAPI_SETCAM 3 // set camera n to given position and angle
+#define DRCAPI_GETCAM 4 // request camera n position and angle
+#define DRCAPI_DIRPLAY 5 // set director time and play with normal speed
+#define DRCAPI_DIRFREEZE 6 // freeze directo at this time
+#define DRCAPI_SETVIEWMODE 7 // overview or 4 cameras
+#define DRCAPI_SETOVERVIEWPARAMS 8 // sets parameter for overview mode
+#define DRCAPI_SETFOCUS 9 // set the camera which has the input focus
+#define DRCAPI_GETTARGETS 10 // queries engine for player list
+#define DRCAPI_SETVIEWPOINTS 11 // gives engine all waypoints
+
+
diff --git a/dep/hlsdk/common/dlight.h b/dep/hlsdk/common/dlight.h
new file mode 100644
index 0000000..f869c98
--- /dev/null
+++ b/dep/hlsdk/common/dlight.h
@@ -0,0 +1,33 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined ( DLIGHTH )
+#define DLIGHTH
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef struct dlight_s
+{
+ vec3_t origin;
+ float radius;
+ color24 color;
+ float die; // stop lighting after this time
+ float decay; // drop this each second
+ float minlight; // don't add when contributing less
+ int key;
+ qboolean dark; // subtracts light instead of adding
+} dlight_t;
+
+#endif
diff --git a/dep/hlsdk/common/dll_state.h b/dep/hlsdk/common/dll_state.h
new file mode 100644
index 0000000..ad16296
--- /dev/null
+++ b/dep/hlsdk/common/dll_state.h
@@ -0,0 +1,23 @@
+//========= Copyright © 1996-2002, Valve LLC, All rights reserved. ============
+//
+// Purpose:
+//
+// $NoKeywords: $
+//=============================================================================
+
+//DLL State Flags
+
+#define DLL_INACTIVE 0 // no dll
+#define DLL_ACTIVE 1 // dll is running
+#define DLL_PAUSED 2 // dll is paused
+#define DLL_CLOSE 3 // closing down dll
+#define DLL_TRANS 4 // Level Transition
+
+// DLL Pause reasons
+
+#define DLL_NORMAL 0 // User hit Esc or something.
+#define DLL_QUIT 4 // Quit now
+#define DLL_RESTART 5 // Switch to launcher for linux, does a quit but returns 1
+
+// DLL Substate info ( not relevant )
+#define ENG_NORMAL (1<<0)
diff --git a/dep/hlsdk/common/entity_state.h b/dep/hlsdk/common/entity_state.h
new file mode 100644
index 0000000..bdfd19e
--- /dev/null
+++ b/dep/hlsdk/common/entity_state.h
@@ -0,0 +1,198 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+
+#ifndef ENTITY_STATE_H
+#define ENTITY_STATE_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+#include "const.h"
+
+
+// For entityType below
+#define ENTITY_NORMAL (1<<0)
+#define ENTITY_BEAM (1<<1)
+#define ENTITY_UNINITIALIZED (1<<30)
+
+// Entity state is used for the baseline and for delta compression of a packet of
+// entities that is sent to a client.
+typedef struct entity_state_s entity_state_t;
+
+struct entity_state_s
+{
+// Fields which are filled in by routines outside of delta compression
+ int entityType;
+ // Index into cl_entities array for this entity.
+ int number;
+ float msg_time;
+
+ // Message number last time the player/entity state was updated.
+ int messagenum;
+
+ // Fields which can be transitted and reconstructed over the network stream
+ vec3_t origin;
+ vec3_t angles;
+
+ int modelindex;
+ int sequence;
+ float frame;
+ int colormap;
+ short skin;
+ short solid;
+ int effects;
+ float scale;
+
+ byte eflags;
+
+ // Render information
+ int rendermode;
+ int renderamt;
+ color24 rendercolor;
+ int renderfx;
+
+ int movetype;
+ float animtime;
+ float framerate;
+ int body;
+ byte controller[4];
+ byte blending[4];
+ vec3_t velocity;
+
+ // Send bbox down to client for use during prediction.
+ vec3_t mins;
+ vec3_t maxs;
+
+ int aiment;
+ // If owned by a player, the index of that player ( for projectiles ).
+ int owner;
+
+ // Friction, for prediction.
+ float friction;
+ // Gravity multiplier
+ float gravity;
+
+// PLAYER SPECIFIC
+ int team;
+ int playerclass;
+ int health;
+ qboolean spectator;
+ int weaponmodel;
+ int gaitsequence;
+ // If standing on conveyor, e.g.
+ vec3_t basevelocity;
+ // Use the crouched hull, or the regular player hull.
+ int usehull;
+ // Latched buttons last time state updated.
+ int oldbuttons;
+ // -1 = in air, else pmove entity number
+ int onground;
+ int iStepLeft;
+ // How fast we are falling
+ float flFallVelocity;
+
+ float fov;
+ int weaponanim;
+
+ // Parametric movement overrides
+ vec3_t startpos;
+ vec3_t endpos;
+ float impacttime;
+ float starttime;
+
+ // For mods
+ int iuser1;
+ int iuser2;
+ int iuser3;
+ int iuser4;
+ float fuser1;
+ float fuser2;
+ float fuser3;
+ float fuser4;
+ vec3_t vuser1;
+ vec3_t vuser2;
+ vec3_t vuser3;
+ vec3_t vuser4;
+};
+
+#include "pm_info.h"
+
+typedef struct clientdata_s
+{
+ vec3_t origin;
+ vec3_t velocity;
+
+ int viewmodel;
+ vec3_t punchangle;
+ int flags;
+ int waterlevel;
+ int watertype;
+ vec3_t view_ofs;
+ float health;
+
+ int bInDuck;
+
+ int weapons; // remove?
+
+ int flTimeStepSound;
+ int flDuckTime;
+ int flSwimTime;
+ int waterjumptime;
+
+ float maxspeed;
+
+ float fov;
+ int weaponanim;
+
+ int m_iId;
+ int ammo_shells;
+ int ammo_nails;
+ int ammo_cells;
+ int ammo_rockets;
+ float m_flNextAttack;
+
+ int tfstate;
+
+ int pushmsec;
+
+ int deadflag;
+
+ char physinfo[ MAX_PHYSINFO_STRING ];
+
+ // For mods
+ int iuser1;
+ int iuser2;
+ int iuser3;
+ int iuser4;
+ float fuser1;
+ float fuser2;
+ float fuser3;
+ float fuser4;
+ vec3_t vuser1;
+ vec3_t vuser2;
+ vec3_t vuser3;
+ vec3_t vuser4;
+} clientdata_t;
+
+#include "weaponinfo.h"
+
+typedef struct local_state_s
+{
+ entity_state_t playerstate;
+ clientdata_t client;
+ weapon_data_t weapondata[ 64 ];
+} local_state_t;
+
+#endif // ENTITY_STATE_H
diff --git a/dep/hlsdk/common/entity_types.h b/dep/hlsdk/common/entity_types.h
new file mode 100644
index 0000000..ff783df
--- /dev/null
+++ b/dep/hlsdk/common/entity_types.h
@@ -0,0 +1,26 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+// entity_types.h
+#if !defined( ENTITY_TYPESH )
+#define ENTITY_TYPESH
+
+#define ET_NORMAL 0
+#define ET_PLAYER 1
+#define ET_TEMPENTITY 2
+#define ET_BEAM 3
+// BMODEL or SPRITE that was split across BSP nodes
+#define ET_FRAGMENTED 4
+
+#endif // !ENTITY_TYPESH
diff --git a/dep/hlsdk/common/enums.h b/dep/hlsdk/common/enums.h
new file mode 100644
index 0000000..ad8bfe0
--- /dev/null
+++ b/dep/hlsdk/common/enums.h
@@ -0,0 +1,28 @@
+/***
+ *
+ * Copyright (c) 2009, Valve LLC. All rights reserved.
+ *
+ * This product contains software technology licensed from Id
+ * Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+ * All Rights Reserved.
+ *
+ * Use, distribution, and modification of this source code and/or resulting
+ * object code is restricted to non-commercial enhancements to products from
+ * Valve LLC. All other use, distribution, or modification is prohibited
+ * without written permission from Valve LLC.
+ *
+ ****/
+
+#ifndef ENUMS_H
+#define ENUMS_H
+
+// Used as array indexer
+typedef enum netsrc_s
+{
+ NS_CLIENT = 0,
+ NS_SERVER,
+ NS_MULTICAST, // xxxMO
+ NS_MAX
+} netsrc_t;
+
+#endif
diff --git a/dep/hlsdk/common/event_api.h b/dep/hlsdk/common/event_api.h
new file mode 100644
index 0000000..722dfe2
--- /dev/null
+++ b/dep/hlsdk/common/event_api.h
@@ -0,0 +1,51 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined ( EVENT_APIH )
+#define EVENT_APIH
+#ifdef _WIN32
+#pragma once
+#endif
+
+#define EVENT_API_VERSION 1
+
+typedef struct event_api_s
+{
+ int version;
+ void ( *EV_PlaySound ) ( int ent, float *origin, int channel, const char *sample, float volume, float attenuation, int fFlags, int pitch );
+ void ( *EV_StopSound ) ( int ent, int channel, const char *sample );
+ int ( *EV_FindModelIndex )( const char *pmodel );
+ int ( *EV_IsLocal ) ( int playernum );
+ int ( *EV_LocalPlayerDucking ) ( void );
+ void ( *EV_LocalPlayerViewheight ) ( float * );
+ void ( *EV_LocalPlayerBounds ) ( int hull, float *mins, float *maxs );
+ int ( *EV_IndexFromTrace) ( struct pmtrace_s *pTrace );
+ struct physent_s *( *EV_GetPhysent ) ( int idx );
+ void ( *EV_SetUpPlayerPrediction ) ( int dopred, int bIncludeLocalClient );
+ void ( *EV_PushPMStates ) ( void );
+ void ( *EV_PopPMStates ) ( void );
+ void ( *EV_SetSolidPlayers ) (int playernum);
+ void ( *EV_SetTraceHull ) ( int hull );
+ void ( *EV_PlayerTrace ) ( float *start, float *end, int traceFlags, int ignore_pe, struct pmtrace_s *tr );
+ void ( *EV_WeaponAnimation ) ( int sequence, int body );
+ unsigned short ( *EV_PrecacheEvent ) ( int type, const char* psz );
+ void ( *EV_PlaybackEvent ) ( int flags, const struct edict_s *pInvoker, unsigned short eventindex, float delay, float *origin, float *angles, float fparam1, float fparam2, int iparam1, int iparam2, int bparam1, int bparam2 );
+ const char *( *EV_TraceTexture ) ( int ground, float *vstart, float *vend );
+ void ( *EV_StopAllSounds ) ( int entnum, int entchannel );
+ void ( *EV_KillEvents ) ( int entnum, const char *eventname );
+} event_api_t;
+
+extern event_api_t eventapi;
+
+#endif
diff --git a/dep/hlsdk/common/event_args.h b/dep/hlsdk/common/event_args.h
new file mode 100644
index 0000000..99dd49a
--- /dev/null
+++ b/dep/hlsdk/common/event_args.h
@@ -0,0 +1,50 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined( EVENT_ARGSH )
+#define EVENT_ARGSH
+#ifdef _WIN32
+#pragma once
+#endif
+
+// Event was invoked with stated origin
+#define FEVENT_ORIGIN ( 1<<0 )
+
+// Event was invoked with stated angles
+#define FEVENT_ANGLES ( 1<<1 )
+
+typedef struct event_args_s
+{
+ int flags;
+
+ // Transmitted
+ int entindex;
+
+ float origin[3];
+ float angles[3];
+ float velocity[3];
+
+ int ducking;
+
+ float fparam1;
+ float fparam2;
+
+ int iparam1;
+ int iparam2;
+
+ int bparam1;
+ int bparam2;
+} event_args_t;
+
+#endif
diff --git a/dep/hlsdk/common/event_flags.h b/dep/hlsdk/common/event_flags.h
new file mode 100644
index 0000000..43f804f
--- /dev/null
+++ b/dep/hlsdk/common/event_flags.h
@@ -0,0 +1,47 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined( EVENT_FLAGSH )
+#define EVENT_FLAGSH
+#ifdef _WIN32
+#pragma once
+#endif
+
+// Skip local host for event send.
+#define FEV_NOTHOST (1<<0)
+
+// Send the event reliably. You must specify the origin and angles and use
+// PLAYBACK_EVENT_FULL for this to work correctly on the server for anything
+// that depends on the event origin/angles. I.e., the origin/angles are not
+// taken from the invoking edict for reliable events.
+#define FEV_RELIABLE (1<<1)
+
+// Don't restrict to PAS/PVS, send this event to _everybody_ on the server ( useful for stopping CHAN_STATIC
+// sounds started by client event when client is not in PVS anymore ( hwguy in TFC e.g. ).
+#define FEV_GLOBAL (1<<2)
+
+// If this client already has one of these events in its queue, just update the event instead of sending it as a duplicate
+//
+#define FEV_UPDATE (1<<3)
+
+// Only send to entity specified as the invoker
+#define FEV_HOSTONLY (1<<4)
+
+// Only send if the event was created on the server.
+#define FEV_SERVER (1<<5)
+
+// Only issue event client side ( from shared code )
+#define FEV_CLIENT (1<<6)
+
+#endif
diff --git a/dep/hlsdk/common/hltv.h b/dep/hlsdk/common/hltv.h
new file mode 100644
index 0000000..ea420a3
--- /dev/null
+++ b/dep/hlsdk/common/hltv.h
@@ -0,0 +1,60 @@
+//========= Copyright © 1996-2002, Valve LLC, All rights reserved. ============
+//
+// Purpose:
+//
+// $NoKeywords: $
+//=============================================================================
+
+// hltv.h
+// all shared consts between server, clients and proxy
+
+#ifndef HLTV_H
+#define HLTV_H
+
+#define TYPE_CLIENT 0 // client is a normal HL client (default)
+#define TYPE_PROXY 1 // client is another proxy
+#define TYPE_DIRECTOR 2
+#define TYPE_COMMENTATOR 3 // client is a commentator
+#define TYPE_DEMO 4 // client is a demo file
+
+// sub commands of svc_hltv:
+#define HLTV_ACTIVE 0 // tells client that he's an spectator and will get director commands
+#define HLTV_STATUS 1 // send status infos about proxy
+#define HLTV_LISTEN 2 // tell client to listen to a multicast stream
+
+// director command types:
+#define DRC_CMD_NONE 0 // NULL director command
+#define DRC_CMD_START 1 // start director mode
+#define DRC_CMD_EVENT 2 // informs about director command
+#define DRC_CMD_MODE 3 // switches camera modes
+#define DRC_CMD_CAMERA 4 // set fixed camera
+#define DRC_CMD_TIMESCALE 5 // sets time scale
+#define DRC_CMD_MESSAGE 6 // send HUD centerprint
+#define DRC_CMD_SOUND 7 // plays a particular sound
+#define DRC_CMD_STATUS 8 // HLTV broadcast status
+#define DRC_CMD_BANNER 9 // set GUI banner
+#define DRC_CMD_STUFFTEXT 10 // like the normal svc_stufftext but as director command
+#define DRC_CMD_CHASE 11 // chase a certain player
+#define DRC_CMD_INEYE 12 // view player through own eyes
+#define DRC_CMD_MAP 13 // show overview map
+#define DRC_CMD_CAMPATH 14 // define camera waypoint
+#define DRC_CMD_WAYPOINTS 15 // start moving camera, inetranl message
+
+#define DRC_CMD_LAST 15
+
+// DRC_CMD_EVENT event flags
+#define DRC_FLAG_PRIO_MASK 0x0F // priorities between 0 and 15 (15 most important)
+#define DRC_FLAG_SIDE (1<<4) //
+#define DRC_FLAG_DRAMATIC (1<<5) // is a dramatic scene
+#define DRC_FLAG_SLOWMOTION (1<<6) // would look good in SloMo
+#define DRC_FLAG_FACEPLAYER (1<<7) // player is doning something (reload/defuse bomb etc)
+#define DRC_FLAG_INTRO (1<<8) // is a introduction scene
+#define DRC_FLAG_FINAL (1<<9) // is a final scene
+#define DRC_FLAG_NO_RANDOM (1<<10) // don't randomize event data
+
+// DRC_CMD_WAYPOINT flags
+#define DRC_FLAG_STARTPATH 1 // end with speed 0.0
+#define DRC_FLAG_SLOWSTART 2 // start with speed 0.0
+#define DRC_FLAG_SLOWEND 4 // end with speed 0.0
+
+#endif // HLTV_H
diff --git a/dep/hlsdk/common/in_buttons.h b/dep/hlsdk/common/in_buttons.h
new file mode 100644
index 0000000..4196a2d
--- /dev/null
+++ b/dep/hlsdk/common/in_buttons.h
@@ -0,0 +1,38 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#ifndef IN_BUTTONS_H
+#define IN_BUTTONS_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+#define IN_ATTACK (1 << 0)
+#define IN_JUMP (1 << 1)
+#define IN_DUCK (1 << 2)
+#define IN_FORWARD (1 << 3)
+#define IN_BACK (1 << 4)
+#define IN_USE (1 << 5)
+#define IN_CANCEL (1 << 6)
+#define IN_LEFT (1 << 7)
+#define IN_RIGHT (1 << 8)
+#define IN_MOVELEFT (1 << 9)
+#define IN_MOVERIGHT (1 << 10)
+#define IN_ATTACK2 (1 << 11)
+#define IN_RUN (1 << 12)
+#define IN_RELOAD (1 << 13)
+#define IN_ALT1 (1 << 14)
+#define IN_SCORE (1 << 15) // Used by client.dll for when scoreboard is held down
+
+#endif // IN_BUTTONS_H
diff --git a/dep/hlsdk/common/ivoicetweak.h b/dep/hlsdk/common/ivoicetweak.h
new file mode 100644
index 0000000..da568c5
--- /dev/null
+++ b/dep/hlsdk/common/ivoicetweak.h
@@ -0,0 +1,38 @@
+//========= Copyright © 1996-2002, Valve LLC, All rights reserved. ============
+//
+// Purpose:
+//
+// $NoKeywords: $
+//=============================================================================
+
+#ifndef IVOICETWEAK_H
+#define IVOICETWEAK_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+// These provide access to the voice controls.
+typedef enum
+{
+ MicrophoneVolume=0, // values 0-1.
+ OtherSpeakerScale, // values 0-1. Scales how loud other players are.
+ MicBoost, // 20 db gain to voice input
+} VoiceTweakControl;
+
+
+typedef struct IVoiceTweak_s
+{
+ // These turn voice tweak mode on and off. While in voice tweak mode, the user's voice is echoed back
+ // without sending to the server.
+ int (*StartVoiceTweakMode)(); // Returns 0 on error.
+ void (*EndVoiceTweakMode)();
+
+ // Get/set control values.
+ void (*SetControlFloat)(VoiceTweakControl iControl, float value);
+ float (*GetControlFloat)(VoiceTweakControl iControl);
+
+ int (*GetSpeakingVolume)();
+} IVoiceTweak;
+
+
+#endif // IVOICETWEAK_H
diff --git a/dep/hlsdk/common/kbutton.h b/dep/hlsdk/common/kbutton.h
new file mode 100644
index 0000000..5607568
--- /dev/null
+++ b/dep/hlsdk/common/kbutton.h
@@ -0,0 +1,41 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#ifndef KBUTTON_H
+#define KBUTTON_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef struct kbutton_s
+{
+ int down[2];
+ int state;
+} kbutton_t;
+
+#endif // KBUTTON_H
diff --git a/dep/hlsdk/common/mathlib.h b/dep/hlsdk/common/mathlib.h
new file mode 100644
index 0000000..757a7f4
--- /dev/null
+++ b/dep/hlsdk/common/mathlib.h
@@ -0,0 +1,176 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#ifndef MATHLIB_H
+#define MATHLIB_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef float vec_t;
+
+#if !defined DID_VEC3_T_DEFINE && !defined vec3_t
+#define DID_VEC3_T_DEFINE
+typedef vec_t vec3_t[3];
+#endif
+
+typedef vec_t vec4_t[4];
+typedef int fixed16_t;
+
+typedef union DLONG_u
+{
+ int i[2];
+ double d;
+ float f;
+} DLONG;
+
+#define M_PI 3.14159265358979323846
+
+#ifdef __cplusplus
+#ifdef min
+#undef min
+#endif
+
+#ifdef max
+#undef max
+#endif
+
+#ifdef clamp
+#undef clamp
+#endif
+
+template
+inline T min(T a, T b)
+{
+ return (a < b) ? a : b;
+}
+
+template
+inline T max(T a, T b)
+{
+ return (a < b) ? b : a;
+}
+
+template
+inline T clamp(T a, T min, T max)
+{
+ return (a > max) ? max : (a < min) ? min : a;
+}
+
+template
+inline T bswap(T s)
+{
+ switch (sizeof(T))
+ {
+ case 2: {auto res = __builtin_bswap16(*(uint16 *)&s); return *(T *)&res; }
+ case 4: {auto res = __builtin_bswap32(*(uint32 *)&s); return *(T *)&res; }
+ case 8: {auto res = __builtin_bswap64(*(uint64 *)&s); return *(T *)&res; }
+ default: return s;
+ }
+}
+#else // __cplusplus
+#ifndef max
+#define max(a,b) (((a) > (b)) ? (a) : (b))
+#endif
+
+#ifndef min
+#define min(a,b) (((a) < (b)) ? (a) : (b))
+#endif
+
+#define clamp(val, min, max) (((val) > (max)) ? (max) : (((val) < (min)) ? (min) : (val)))
+#endif // __cplusplus
+
+inline void VectorAdd(const vec_t *veca, const vec_t *vecb, vec_t *out)
+{
+ out[0] = veca[0] + vecb[0];
+ out[1] = veca[1] + vecb[1];
+ out[2] = veca[2] + vecb[2];
+}
+
+inline void VectorSubtract(const vec_t *veca, const vec_t *vecb, vec_t *out)
+{
+ out[0] = veca[0] - vecb[0];
+ out[1] = veca[1] - vecb[1];
+ out[2] = veca[2] - vecb[2];
+}
+
+inline void VectorMA(const vec_t *veca, float scale, const vec_t *vecm, vec_t *out)
+{
+ out[0] = scale * vecm[0] + veca[0];
+ out[1] = scale * vecm[1] + veca[1];
+ out[2] = scale * vecm[2] + veca[2];
+}
+
+inline void VectorScale(const vec_t *in, float scale, vec_t *out)
+{
+ out[0] = scale * in[0];
+ out[1] = scale * in[1];
+ out[2] = scale * in[2];
+}
+
+inline void VectorClear(vec_t *in)
+{
+ in[0] = 0.0f;
+ in[1] = 0.0f;
+ in[2] = 0.0f;
+}
+
+inline void VectorCopy(const vec_t *in, vec_t *out)
+{
+ out[0] = in[0];
+ out[1] = in[1];
+ out[2] = in[2];
+}
+
+inline void VectorNegate(const vec_t *in, vec_t *out)
+{
+ out[0] = -in[0];
+ out[1] = -in[1];
+ out[2] = -in[2];
+}
+
+inline void VectorInverse(vec_t *in)
+{
+ in[0] = -in[0];
+ in[1] = -in[1];
+ in[2] = -in[2];
+}
+
+inline void VectorAverage(const vec_t *veca, const vec_t *vecb, vec_t *out)
+{
+ out[0] = (veca[0] + vecb[0]) * 0.5f;
+ out[1] = (veca[1] + vecb[1]) * 0.5f;
+ out[2] = (veca[2] + vecb[2]) * 0.5f;
+}
+
+inline bool VectorIsZero(const vec_t *in)
+{
+ return (in[0] == 0.0f && in[1] == 0.0f && in[2] == 0.0f);
+}
+
+#endif // MATHLIB_H
diff --git a/dep/hlsdk/common/md5.h b/dep/hlsdk/common/md5.h
new file mode 100644
index 0000000..637ba0f
--- /dev/null
+++ b/dep/hlsdk/common/md5.h
@@ -0,0 +1,34 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+
+#pragma once
+
+#include
+
+// MD5 Hash
+typedef struct
+{
+ unsigned int buf[4];
+ unsigned int bits[2];
+ unsigned char in[64];
+} MD5Context_t;
+
+void MD5Init(MD5Context_t *ctx);
+void MD5Update(MD5Context_t *ctx, const unsigned char *buf, unsigned int len);
+void MD5Final(unsigned char digest[16], MD5Context_t *ctx);
+void MD5Transform(unsigned int buf[4], const unsigned int in[16]);
+
+BOOL MD5_Hash_File(unsigned char digest[16], char *pszFileName, BOOL bUsefopen, BOOL bSeed, unsigned int seed[4]);
+char *MD5_Print(unsigned char hash[16]);
diff --git a/dep/hlsdk/common/net_api.h b/dep/hlsdk/common/net_api.h
new file mode 100644
index 0000000..9551d18
--- /dev/null
+++ b/dep/hlsdk/common/net_api.h
@@ -0,0 +1,99 @@
+//========= Copyright © 1996-2002, Valve LLC, All rights reserved. ============
+//
+// Purpose:
+//
+// $NoKeywords: $
+//=============================================================================
+
+#if !defined( NET_APIH )
+#define NET_APIH
+#ifdef _WIN32
+#pragma once
+#endif
+
+#if !defined ( NETADRH )
+#include "netadr.h"
+#endif
+
+#define NETAPI_REQUEST_SERVERLIST ( 0 ) // Doesn't need a remote address
+#define NETAPI_REQUEST_PING ( 1 )
+#define NETAPI_REQUEST_RULES ( 2 )
+#define NETAPI_REQUEST_PLAYERS ( 3 )
+#define NETAPI_REQUEST_DETAILS ( 4 )
+
+// Set this flag for things like broadcast requests, etc. where the engine should not
+// kill the request hook after receiving the first response
+#define FNETAPI_MULTIPLE_RESPONSE ( 1<<0 )
+
+typedef void ( *net_api_response_func_t ) ( struct net_response_s *response );
+
+#define NET_SUCCESS ( 0 )
+#define NET_ERROR_TIMEOUT ( 1<<0 )
+#define NET_ERROR_PROTO_UNSUPPORTED ( 1<<1 )
+#define NET_ERROR_UNDEFINED ( 1<<2 )
+
+typedef struct net_adrlist_s
+{
+ struct net_adrlist_s *next;
+ netadr_t remote_address;
+} net_adrlist_t;
+
+typedef struct net_response_s
+{
+ // NET_SUCCESS or an error code
+ int error;
+
+ // Context ID
+ int context;
+ // Type
+ int type;
+
+ // Server that is responding to the request
+ netadr_t remote_address;
+
+ // Response RTT ping time
+ double ping;
+ // Key/Value pair string ( separated by backlash \ characters )
+ // WARNING: You must copy this buffer in the callback function, because it is freed
+ // by the engine right after the call!!!!
+ // ALSO: For NETAPI_REQUEST_SERVERLIST requests, this will be a pointer to a linked list of net_adrlist_t's
+ void *response;
+} net_response_t;
+
+typedef struct net_status_s
+{
+ // Connected to remote server? 1 == yes, 0 otherwise
+ int connected;
+ // Client's IP address
+ netadr_t local_address;
+ // Address of remote server
+ netadr_t remote_address;
+ // Packet Loss ( as a percentage )
+ int packet_loss;
+ // Latency, in seconds ( multiply by 1000.0 to get milliseconds )
+ double latency;
+ // Connection time, in seconds
+ double connection_time;
+ // Rate setting ( for incoming data )
+ double rate;
+} net_status_t;
+
+typedef struct net_api_s
+{
+ // APIs
+ void ( *InitNetworking )( void );
+ void ( *Status ) ( struct net_status_s *status );
+ void ( *SendRequest) ( int context, int request, int flags, double timeout, struct netadr_s *remote_address, net_api_response_func_t response );
+ void ( *CancelRequest ) ( int context );
+ void ( *CancelAllRequests ) ( void );
+ char *( *AdrToString ) ( struct netadr_s *a );
+ int ( *CompareAdr ) ( struct netadr_s *a, struct netadr_s *b );
+ int ( *StringToAdr ) ( char *s, struct netadr_s *a );
+ const char *( *ValueForKey ) ( const char *s, const char *key );
+ void ( *RemoveKey ) ( char *s, const char *key );
+ void ( *SetValueForKey ) (char *s, const char *key, const char *value, int maxsize );
+} net_api_t;
+
+extern net_api_t netapi;
+
+#endif // NET_APIH
diff --git a/dep/hlsdk/common/netadr.h b/dep/hlsdk/common/netadr.h
new file mode 100644
index 0000000..0a468d2
--- /dev/null
+++ b/dep/hlsdk/common/netadr.h
@@ -0,0 +1,40 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+
+#ifndef NETADR_H
+#define NETADR_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef enum
+{
+ NA_UNUSED,
+ NA_LOOPBACK,
+ NA_BROADCAST,
+ NA_IP,
+ NA_IPX,
+ NA_BROADCAST_IPX,
+} netadrtype_t;
+
+typedef struct netadr_s
+{
+ netadrtype_t type;
+ unsigned char ip[4];
+ unsigned char ipx[10];
+ unsigned short port;
+} netadr_t;
+
+#endif // NETADR_H
diff --git a/dep/hlsdk/common/netapi.cpp b/dep/hlsdk/common/netapi.cpp
new file mode 100644
index 0000000..7c69449
--- /dev/null
+++ b/dep/hlsdk/common/netapi.cpp
@@ -0,0 +1,208 @@
+#include
+#include
+
+#ifdef _WIN32
+ #include "winsock.h"
+#else
+ #include
+ #include
+ #include
+ #include
+ #include
+ #include
+#endif // _WIN32
+
+#include "netapi.h"
+
+class CNetAPI: public INetAPI {
+public:
+ virtual void NetAdrToSockAddr(netadr_t *a, struct sockaddr *s);
+ virtual void SockAddrToNetAdr(struct sockaddr *s, netadr_t *a);
+
+ virtual char *AdrToString(netadr_t *a);
+ virtual bool StringToAdr(const char *s, netadr_t *a);
+
+ virtual void GetSocketAddress(int socket, netadr_t *a);
+ virtual bool CompareAdr(netadr_t *a, netadr_t *b);
+ virtual void GetLocalIP(netadr_t *a);
+};
+
+// Expose interface
+CNetAPI g_NetAPI;
+INetAPI *net = (INetAPI *)&g_NetAPI;
+
+void CNetAPI::NetAdrToSockAddr(netadr_t *a, struct sockaddr *s)
+{
+ memset(s, 0, sizeof(*s));
+
+ if (a->type == NA_BROADCAST)
+ {
+ ((struct sockaddr_in *)s)->sin_family = AF_INET;
+ ((struct sockaddr_in *)s)->sin_port = a->port;
+ ((struct sockaddr_in *)s)->sin_addr.s_addr = INADDR_BROADCAST;
+ }
+ else if (a->type == NA_IP)
+ {
+ ((struct sockaddr_in *)s)->sin_family = AF_INET;
+ ((struct sockaddr_in *)s)->sin_addr.s_addr = *(int *)&a->ip;
+ ((struct sockaddr_in *)s)->sin_port = a->port;
+ }
+}
+
+void CNetAPI::SockAddrToNetAdr(struct sockaddr *s, netadr_t *a)
+{
+ if (s->sa_family == AF_INET)
+ {
+ a->type = NA_IP;
+ *(int *)&a->ip = ((struct sockaddr_in *)s)->sin_addr.s_addr;
+ a->port = ((struct sockaddr_in *)s)->sin_port;
+ }
+}
+
+char *CNetAPI::AdrToString(netadr_t *a)
+{
+ static char s[64];
+ memset(s, 0, sizeof(s));
+
+ if (a)
+ {
+ if (a->type == NA_LOOPBACK)
+ {
+ sprintf(s, "loopback");
+ }
+ else if (a->type == NA_IP)
+ {
+ sprintf(s, "%i.%i.%i.%i:%i", a->ip[0], a->ip[1], a->ip[2], a->ip[3], ntohs(a->port));
+ }
+ }
+
+ return s;
+}
+
+bool StringToSockaddr(const char *s, struct sockaddr *sadr)
+{
+ struct hostent *h;
+ char *colon;
+ char copy[128];
+ struct sockaddr_in *p;
+
+ memset(sadr, 0, sizeof(*sadr));
+
+ p = (struct sockaddr_in *)sadr;
+ p->sin_family = AF_INET;
+ p->sin_port = 0;
+
+ strcpy(copy, s);
+
+ // strip off a trailing :port if present
+ for (colon = copy ; *colon ; colon++)
+ {
+ if (*colon == ':')
+ {
+ // terminate
+ *colon = '\0';
+
+ // Start at next character
+ p->sin_port = htons((short)atoi(colon + 1));
+ }
+ }
+
+ // Numeric IP, no DNS
+ if (copy[0] >= '0' && copy[0] <= '9' && strstr(copy, "."))
+ {
+ *(int *)&p->sin_addr = inet_addr(copy);
+ }
+ else
+ {
+ // DNS it
+ if (!(h = gethostbyname(copy)))
+ {
+ return false;
+ }
+
+ // Use first result
+ *(int *)&p->sin_addr = *(int *)h->h_addr_list[0];
+ }
+
+ return true;
+}
+
+bool CNetAPI::StringToAdr(const char *s, netadr_t *a)
+{
+ struct sockaddr sadr;
+ if (!strcmp(s, "localhost"))
+ {
+ memset(a, 0, sizeof(*a));
+ a->type = NA_LOOPBACK;
+ return true;
+ }
+
+ if (!StringToSockaddr(s, &sadr))
+ {
+ return false;
+ }
+
+ SockAddrToNetAdr(&sadr, a);
+ return true;
+}
+
+// Lookup the IP address for the specified IP socket
+void CNetAPI::GetSocketAddress(int socket, netadr_t *a)
+{
+ char buff[512];
+ struct sockaddr_in address;
+ int namelen;
+
+ memset(a, 0, sizeof(*a));
+ gethostname(buff, sizeof(buff));
+
+ // Ensure that it doesn't overrun the buffer
+ buff[sizeof buff - 1] = '\0';
+ StringToAdr(buff, a);
+
+ namelen = sizeof(address);
+ if (getsockname(socket, (struct sockaddr *)&address, (int *)&namelen) == 0)
+ {
+ a->port = address.sin_port;
+ }
+}
+
+bool CNetAPI::CompareAdr(netadr_t *a, netadr_t *b)
+{
+ if (a->type != b->type)
+ {
+ return false;
+ }
+
+ if (a->type == NA_LOOPBACK)
+ {
+ return true;
+ }
+
+ if (a->type == NA_IP &&
+ a->ip[0] == b->ip[0] &&
+ a->ip[1] == b->ip[1] &&
+ a->ip[2] == b->ip[2] &&
+ a->ip[3] == b->ip[3] &&
+ a->port == b->port)
+ {
+ return true;
+ }
+
+ return false;
+}
+
+void CNetAPI::GetLocalIP(netadr_t *a)
+{
+ char s[64];
+ if(!::gethostname(s, 64))
+ {
+ struct hostent *localip = ::gethostbyname(s);
+ if(localip)
+ {
+ a->type = NA_IP;
+ a->port = 0;
+ memcpy(a->ip, localip->h_addr_list[0], 4);
+ }
+ }
+}
diff --git a/dep/hlsdk/common/netapi.h b/dep/hlsdk/common/netapi.h
new file mode 100644
index 0000000..8401c92
--- /dev/null
+++ b/dep/hlsdk/common/netapi.h
@@ -0,0 +1,25 @@
+#ifndef NETAPI_H
+#define NETAPI_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+#include "netadr.h"
+
+class INetAPI {
+public:
+ virtual void NetAdrToSockAddr(netadr_t *a, struct sockaddr *s) = 0; // Convert a netadr_t to sockaddr
+ virtual void SockAddrToNetAdr(struct sockaddr *s, netadr_t *a) = 0; // Convert a sockaddr to netadr_t
+
+ virtual char *AdrToString(netadr_t *a) = 0; // Convert a netadr_t to a string
+ virtual bool StringToAdr(const char *s, netadr_t *a) = 0; // Convert a string address to a netadr_t, doing DNS if needed
+ virtual void GetSocketAddress(int socket, netadr_t *a) = 0; // Look up IP address for socket
+ virtual bool CompareAdr(netadr_t *a, netadr_t *b) = 0;
+
+ // return the IP of the local host
+ virtual void GetLocalIP(netadr_t *a) = 0;
+};
+
+extern INetAPI *net;
+
+#endif // NETAPI_H
diff --git a/dep/hlsdk/common/nowin.h b/dep/hlsdk/common/nowin.h
new file mode 100644
index 0000000..0b1f577
--- /dev/null
+++ b/dep/hlsdk/common/nowin.h
@@ -0,0 +1,16 @@
+//========= Copyright © 1996-2002, Valve LLC, All rights reserved. ============
+//
+// Purpose:
+//
+// $NoKeywords: $
+//=============================================================================
+
+#ifndef INC_NOWIN_H
+#define INC_NOWIN_H
+#ifndef _WIN32
+
+#include
+#include
+
+#endif //!_WIN32
+#endif //INC_NOWIN_H
diff --git a/dep/hlsdk/common/parsemsg.cpp b/dep/hlsdk/common/parsemsg.cpp
new file mode 100644
index 0000000..3d121d6
--- /dev/null
+++ b/dep/hlsdk/common/parsemsg.cpp
@@ -0,0 +1,258 @@
+/***
+*
+* Copyright (c) 1999, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+//
+// parsemsg.cpp
+//
+//--------------------------------------------------------------------------------------------------------------
+#include "precompiled.h"
+
+typedef unsigned char byte;
+#define true 1
+
+static byte *gpBuf;
+static int giSize;
+static int giRead;
+static int giBadRead;
+
+int READ_OK( void )
+{
+ return !giBadRead;
+}
+
+void BEGIN_READ( void *buf, int size )
+{
+ giRead = 0;
+ giBadRead = 0;
+ giSize = size;
+ gpBuf = (byte*)buf;
+}
+
+
+int READ_CHAR( void )
+{
+ int c;
+
+ if (giRead + 1 > giSize)
+ {
+ giBadRead = true;
+ return -1;
+ }
+
+ c = (signed char)gpBuf[giRead];
+ giRead++;
+
+ return c;
+}
+
+int READ_BYTE( void )
+{
+ int c;
+
+ if (giRead+1 > giSize)
+ {
+ giBadRead = true;
+ return -1;
+ }
+
+ c = (unsigned char)gpBuf[giRead];
+ giRead++;
+
+ return c;
+}
+
+int READ_SHORT( void )
+{
+ int c;
+
+ if (giRead+2 > giSize)
+ {
+ giBadRead = true;
+ return -1;
+ }
+
+ c = (short)( gpBuf[giRead] + ( gpBuf[giRead+1] << 8 ) );
+
+ giRead += 2;
+
+ return c;
+}
+
+int READ_WORD( void )
+{
+ return READ_SHORT();
+}
+
+
+int READ_LONG( void )
+{
+ int c;
+
+ if (giRead+4 > giSize)
+ {
+ giBadRead = true;
+ return -1;
+ }
+
+ c = gpBuf[giRead] + (gpBuf[giRead + 1] << 8) + (gpBuf[giRead + 2] << 16) + (gpBuf[giRead + 3] << 24);
+
+ giRead += 4;
+
+ return c;
+}
+
+float READ_FLOAT( void )
+{
+ union
+ {
+ byte b[4];
+ float f;
+ int l;
+ } dat;
+
+ dat.b[0] = gpBuf[giRead];
+ dat.b[1] = gpBuf[giRead+1];
+ dat.b[2] = gpBuf[giRead+2];
+ dat.b[3] = gpBuf[giRead+3];
+ giRead += 4;
+
+// dat.l = LittleLong (dat.l);
+
+ return dat.f;
+}
+
+char* READ_STRING( void )
+{
+ static char string[2048];
+ int l,c;
+
+ string[0] = 0;
+
+ l = 0;
+ do
+ {
+ if ( giRead+1 > giSize )
+ break; // no more characters
+
+ c = READ_CHAR();
+ if (c == -1 || c == 0)
+ break;
+ string[l] = c;
+ l++;
+ } while (l < sizeof(string)-1);
+
+ string[l] = 0;
+
+ return string;
+}
+
+float READ_COORD( void )
+{
+ return (float)(READ_SHORT() * (1.0/8));
+}
+
+float READ_ANGLE( void )
+{
+ return (float)(READ_CHAR() * (360.0/256));
+}
+
+float READ_HIRESANGLE( void )
+{
+ return (float)(READ_SHORT() * (360.0/65536));
+}
+
+//--------------------------------------------------------------------------------------------------------------
+BufferWriter::BufferWriter()
+{
+ Init( NULL, 0 );
+}
+
+//--------------------------------------------------------------------------------------------------------------
+BufferWriter::BufferWriter( unsigned char *buffer, int bufferLen )
+{
+ Init( buffer, bufferLen );
+}
+
+//--------------------------------------------------------------------------------------------------------------
+void BufferWriter::Init( unsigned char *buffer, int bufferLen )
+{
+ m_overflow = false;
+ m_buffer = buffer;
+ m_remaining = bufferLen;
+ m_overallLength = bufferLen;
+}
+
+//--------------------------------------------------------------------------------------------------------------
+void BufferWriter::WriteByte( unsigned char data )
+{
+ if (!m_buffer || !m_remaining)
+ {
+ m_overflow = true;
+ return;
+ }
+
+ *m_buffer = data;
+ ++m_buffer;
+ --m_remaining;
+}
+
+//--------------------------------------------------------------------------------------------------------------
+void BufferWriter::WriteLong( int data )
+{
+ if (!m_buffer || m_remaining < 4)
+ {
+ m_overflow = true;
+ return;
+ }
+
+ m_buffer[0] = data&0xff;
+ m_buffer[1] = (data>>8)&0xff;
+ m_buffer[2] = (data>>16)&0xff;
+ m_buffer[3] = data>>24;
+ m_buffer += 4;
+ m_remaining -= 4;
+}
+
+//--------------------------------------------------------------------------------------------------------------
+void BufferWriter::WriteString( const char *str )
+{
+ if (!m_buffer || !m_remaining)
+ {
+ m_overflow = true;
+ return;
+ }
+
+ if (!str)
+ str = "";
+
+ int len = strlen(str)+1;
+ if ( len > m_remaining )
+ {
+ m_overflow = true;
+ str = "";
+ len = 1;
+ }
+
+ strcpy((char *)m_buffer, str);
+ m_remaining -= len;
+ m_buffer += len;
+}
+
+//--------------------------------------------------------------------------------------------------------------
+int BufferWriter::GetSpaceUsed()
+{
+ return m_overallLength - m_remaining;
+}
+
+//--------------------------------------------------------------------------------------------------------------
diff --git a/dep/hlsdk/common/parsemsg.h b/dep/hlsdk/common/parsemsg.h
new file mode 100644
index 0000000..be7affc
--- /dev/null
+++ b/dep/hlsdk/common/parsemsg.h
@@ -0,0 +1,66 @@
+/***
+*
+* Copyright (c) 1999, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+//
+// parsemsg.h
+// MDC - copying from cstrike\cl_dll so career-mode stuff can catch messages
+// in this dll. (and C++ifying it)
+//
+
+#ifndef PARSEMSG_H
+#define PARSEMSG_H
+
+#define ASSERT( x )
+//--------------------------------------------------------------------------------------------------------------
+void BEGIN_READ( void *buf, int size );
+int READ_CHAR( void );
+int READ_BYTE( void );
+int READ_SHORT( void );
+int READ_WORD( void );
+int READ_LONG( void );
+float READ_FLOAT( void );
+char* READ_STRING( void );
+float READ_COORD( void );
+float READ_ANGLE( void );
+float READ_HIRESANGLE( void );
+int READ_OK( void );
+
+//--------------------------------------------------------------------------------------------------------------
+class BufferWriter
+{
+public:
+ BufferWriter();
+ BufferWriter( unsigned char *buffer, int bufferLen );
+ void Init( unsigned char *buffer, int bufferLen );
+
+ void WriteByte( unsigned char data );
+ void WriteLong( int data );
+ void WriteString( const char *str );
+
+ bool HasOverflowed();
+ int GetSpaceUsed();
+
+protected:
+ unsigned char *m_buffer;
+ int m_remaining;
+ bool m_overflow;
+ int m_overallLength;
+};
+
+//--------------------------------------------------------------------------------------------------------------
+
+#endif // PARSEMSG_H
+
+
+
diff --git a/dep/hlsdk/common/particledef.h b/dep/hlsdk/common/particledef.h
new file mode 100644
index 0000000..7e4043a
--- /dev/null
+++ b/dep/hlsdk/common/particledef.h
@@ -0,0 +1,57 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined( PARTICLEDEFH )
+#define PARTICLEDEFH
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef enum {
+ pt_static,
+ pt_grav,
+ pt_slowgrav,
+ pt_fire,
+ pt_explode,
+ pt_explode2,
+ pt_blob,
+ pt_blob2,
+ pt_vox_slowgrav,
+ pt_vox_grav,
+ pt_clientcustom // Must have callback function specified
+} ptype_t;
+
+// !!! if this is changed, it must be changed in d_ifacea.h too !!!
+typedef struct particle_s
+{
+// driver-usable fields
+ vec3_t org;
+ short color;
+ short packedColor;
+// drivers never touch the following fields
+ struct particle_s *next;
+ vec3_t vel;
+ float ramp;
+ float die;
+ ptype_t type;
+ void (*deathfunc)( struct particle_s *particle );
+
+ // for pt_clientcusttom, we'll call this function each frame
+ void (*callback)( struct particle_s *particle, float frametime );
+
+ // For deathfunc, etc.
+ unsigned char context;
+} particle_t;
+
+#endif
diff --git a/dep/hlsdk/common/pmtrace.h b/dep/hlsdk/common/pmtrace.h
new file mode 100644
index 0000000..1784e9c
--- /dev/null
+++ b/dep/hlsdk/common/pmtrace.h
@@ -0,0 +1,43 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined( PMTRACEH )
+#define PMTRACEH
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef struct
+{
+ vec3_t normal;
+ float dist;
+} pmplane_t;
+
+typedef struct pmtrace_s pmtrace_t;
+
+struct pmtrace_s
+{
+ qboolean allsolid; // if true, plane is not valid
+ qboolean startsolid; // if true, the initial point was in a solid area
+ qboolean inopen, inwater; // End point is in empty space or in water
+ float fraction; // time completed, 1.0 = didn't hit anything
+ vec3_t endpos; // final position
+ pmplane_t plane; // surface normal at impact
+ int ent; // entity at impact
+ vec3_t deltavelocity; // Change in player's velocity caused by impact.
+ // Only run on server.
+ int hitgroup;
+};
+
+#endif
diff --git a/dep/hlsdk/common/port.h b/dep/hlsdk/common/port.h
new file mode 100644
index 0000000..a0838a7
--- /dev/null
+++ b/dep/hlsdk/common/port.h
@@ -0,0 +1,119 @@
+// port.h: portability helper
+//
+//////////////////////////////////////////////////////////////////////
+
+#pragma once
+
+#include "archtypes.h" // DAL
+
+#ifdef _WIN32
+
+ // Insert your headers here
+ #define WIN32_LEAN_AND_MEAN // Exclude rarely-used stuff from Windows headers
+ #define WIN32_EXTRA_LEAN
+
+ #include "winsani_in.h"
+ #include
+ #include "winsani_out.h"
+
+ #include
+ #include
+ #include
+
+#else // _WIN32
+
+ #include
+ #include
+ #include // exit()
+ #include // strncpy()
+ #include // tolower()
+ #include
+ #include
+ #include
+ #include
+
+ typedef unsigned char BYTE;
+
+ typedef int32 LONG;
+ //typedef uint32 ULONG;
+
+ #ifndef ARCHTYPES_H
+ typedef uint32 ULONG;
+ #endif
+
+ typedef void *HANDLE;
+
+ #ifndef HMODULE
+ typedef void *HMODULE;
+ #endif
+
+ typedef char * LPSTR;
+
+ #define __cdecl
+
+
+ #ifdef __linux__
+ typedef struct POINT_s
+ {
+ int x;
+ int y;
+ } POINT;
+ typedef void *HINSTANCE;
+ typedef void *HWND;
+ typedef void *HDC;
+ typedef void *HGLRC;
+
+ typedef struct RECT_s
+ {
+ int left;
+ int right;
+ int top;
+ int bottom;
+ } RECT;
+ #endif
+
+
+ #ifdef __cplusplus
+
+ //#undef FALSE
+ //#undef TRUE
+
+ #ifdef OSX
+ //#else
+ //const bool FALSE = false;
+ //const bool TRUE = true;
+ #endif
+ #endif
+
+ #ifndef NULL
+ #ifdef __cplusplus
+ #define NULL 0
+ #else
+ #define NULL ((void *)0)
+ #endif
+ #endif
+
+ #ifdef __cplusplus
+ inline int ioctlsocket( int d, int cmd, uint32 *argp ) { return ioctl( d, cmd, argp ); }
+ inline int closesocket( int fd ) { return close( fd ); }
+ inline char * GetCurrentDirectory( size_t size, char * buf ) { return getcwd( buf, size ); }
+ inline int WSAGetLastError() { return errno; }
+
+ inline void DebugBreak( void ) { exit( 1 ); }
+ #endif
+
+ extern char g_szEXEName[ 4096 ];
+
+ #define _snprintf snprintf
+
+ #if defined(OSX)
+ #define SO_ARCH_SUFFIX ".dylib"
+ #else
+ #if defined ( __x86_64__ )
+ #define SO_ARCH_SUFFIX "_amd64.so"
+ #else
+ #define SO_ARCH_SUFFIX ".so"
+ #endif
+ #endif
+#endif
+
diff --git a/dep/hlsdk/common/qfont.h b/dep/hlsdk/common/qfont.h
new file mode 100644
index 0000000..2fc8129
--- /dev/null
+++ b/dep/hlsdk/common/qfont.h
@@ -0,0 +1,41 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined( QFONTH )
+#define QFONTH
+#ifdef _WIN32
+#pragma once
+#endif
+
+// Font stuff
+
+#define NUM_GLYPHS 256
+// does not exist: // #include "basetypes.h"
+
+typedef struct
+{
+ short startoffset;
+ short charwidth;
+} charinfo;
+
+typedef struct qfont_s
+{
+ int width, height;
+ int rowcount;
+ int rowheight;
+ charinfo fontinfo[ NUM_GLYPHS ];
+ unsigned char data[4];
+} qfont_t;
+
+#endif // qfont.h
diff --git a/dep/hlsdk/common/qlimits.h b/dep/hlsdk/common/qlimits.h
new file mode 100644
index 0000000..1a67bd5
--- /dev/null
+++ b/dep/hlsdk/common/qlimits.h
@@ -0,0 +1,44 @@
+//========= Copyright (c) 1996-2002, Valve LLC, All rights reserved. ==========
+//
+// Purpose:
+//
+// $NoKeywords: $
+//=============================================================================
+
+#ifndef QLIMITS_H
+#define QLIMITS_H
+
+#if defined( _WIN32 )
+#pragma once
+#endif
+
+// DATA STRUCTURE INFO
+
+#define MAX_NUM_ARGVS 50
+
+// SYSTEM INFO
+#define MAX_QPATH 64 // max length of a game pathname
+#define MAX_OSPATH 260 // max length of a filesystem pathname
+
+#define ON_EPSILON 0.1 // point on plane side epsilon
+
+#define MAX_LIGHTSTYLE_INDEX_BITS 6
+#define MAX_LIGHTSTYLES (1<
+
+#if !defined(_WIN32)
+void NORETURN Sys_Error(const char *error, ...);
+
+// This file adds the necessary compatibility tricks to avoid symbols with
+// version GLIBCXX_3.4.16 and bigger, keeping binary compatibility with libstdc++ 4.6.1.
+namespace std
+{
+ // We shouldn't be throwing exceptions at all, but it sadly turns out we call STL (inline) functions that do.
+ void __throw_out_of_range_fmt(const char *fmt, ...)
+ {
+ va_list ap;
+ char buf[1024]; // That should be big enough.
+
+ va_start(ap, fmt);
+ vsnprintf(buf, sizeof(buf), fmt, ap);
+ buf[sizeof(buf) - 1] = '\0';
+ va_end(ap);
+
+ Sys_Error(buf);
+ }
+}; // namespace std
+
+// Technically, this symbol is not in GLIBCXX_3.4.20, but in CXXABI_1.3.8,
+// but that's equivalent, version-wise. Those calls are added by the compiler
+// itself on `new Class[n]` calls.
+extern "C"
+void __cxa_throw_bad_array_new_length()
+{
+ Sys_Error("Bad array new length.");
+}
+#endif // !defined(_WIN32)
diff --git a/dep/hlsdk/common/studio_event.h b/dep/hlsdk/common/studio_event.h
new file mode 100644
index 0000000..c79c210
--- /dev/null
+++ b/dep/hlsdk/common/studio_event.h
@@ -0,0 +1,29 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined( STUDIO_EVENTH )
+#define STUDIO_EVENTH
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef struct mstudioevent_s
+{
+ int frame;
+ int event;
+ int type;
+ char options[64];
+} mstudioevent_t;
+
+#endif // STUDIO_EVENTH
diff --git a/dep/hlsdk/common/textconsole.cpp b/dep/hlsdk/common/textconsole.cpp
new file mode 100644
index 0000000..45d13d7
--- /dev/null
+++ b/dep/hlsdk/common/textconsole.cpp
@@ -0,0 +1,392 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#include "precompiled.h"
+
+bool CTextConsole::Init(IBaseSystem *system)
+{
+ // NULL or a valid base system interface
+ m_System = system;
+
+ Q_memset(m_szConsoleText, 0, sizeof(m_szConsoleText));
+ m_nConsoleTextLen = 0;
+ m_nCursorPosition = 0;
+
+ Q_memset(m_szSavedConsoleText, 0, sizeof(m_szSavedConsoleText));
+ m_nSavedConsoleTextLen = 0;
+
+ Q_memset(m_aszLineBuffer, 0, sizeof(m_aszLineBuffer));
+ m_nTotalLines = 0;
+ m_nInputLine = 0;
+ m_nBrowseLine = 0;
+
+ // these are log messages, not related to console
+ Sys_Printf("\n");
+ Sys_Printf("Console initialized.\n");
+
+ m_ConsoleVisible = true;
+
+ return true;
+}
+
+void CTextConsole::InitSystem(IBaseSystem *system)
+{
+ m_System = system;
+}
+
+void CTextConsole::SetVisible(bool visible)
+{
+ m_ConsoleVisible = visible;
+}
+
+bool CTextConsole::IsVisible()
+{
+ return m_ConsoleVisible;
+}
+
+void CTextConsole::ShutDown()
+{
+ ;
+}
+
+void CTextConsole::Print(char *pszMsg)
+{
+ if (m_nConsoleTextLen)
+ {
+ int nLen = m_nConsoleTextLen;
+ while (nLen--)
+ {
+ PrintRaw("\b \b");
+ }
+ }
+
+ PrintRaw(pszMsg);
+
+ if (m_nConsoleTextLen)
+ {
+ PrintRaw(m_szConsoleText, m_nConsoleTextLen);
+ }
+
+ UpdateStatus();
+}
+
+int CTextConsole::ReceiveNewline()
+{
+ int nLen = 0;
+
+ Echo("\n");
+
+ if (m_nConsoleTextLen)
+ {
+ nLen = m_nConsoleTextLen;
+
+ m_szConsoleText[ m_nConsoleTextLen ] = '\0';
+ m_nConsoleTextLen = 0;
+ m_nCursorPosition = 0;
+
+ // cache line in buffer, but only if it's not a duplicate of the previous line
+ if ((m_nInputLine == 0) || (Q_strcmp(m_aszLineBuffer[ m_nInputLine - 1 ], m_szConsoleText)))
+ {
+ Q_strncpy(m_aszLineBuffer[ m_nInputLine ], m_szConsoleText, MAX_CONSOLE_TEXTLEN);
+ m_nInputLine++;
+
+ if (m_nInputLine > m_nTotalLines)
+ m_nTotalLines = m_nInputLine;
+
+ if (m_nInputLine >= MAX_BUFFER_LINES)
+ m_nInputLine = 0;
+
+ }
+
+ m_nBrowseLine = m_nInputLine;
+ }
+
+ return nLen;
+}
+
+void CTextConsole::ReceiveBackspace()
+{
+ int nCount;
+
+ if (m_nCursorPosition == 0)
+ {
+ return;
+ }
+
+ m_nConsoleTextLen--;
+ m_nCursorPosition--;
+
+ Echo("\b");
+
+ for (nCount = m_nCursorPosition; nCount < m_nConsoleTextLen; ++nCount)
+ {
+ m_szConsoleText[ nCount ] = m_szConsoleText[ nCount + 1 ];
+ Echo(m_szConsoleText + nCount, 1);
+ }
+
+ Echo(" ");
+
+ nCount = m_nConsoleTextLen;
+ while (nCount >= m_nCursorPosition)
+ {
+ Echo("\b");
+ nCount--;
+ }
+
+ m_nBrowseLine = m_nInputLine;
+}
+
+void CTextConsole::ReceiveTab()
+{
+ if (!m_System)
+ return;
+
+ ObjectList matches;
+ m_szConsoleText[ m_nConsoleTextLen ] = '\0';
+ m_System->GetCommandMatches(m_szConsoleText, &matches);
+
+ if (matches.IsEmpty())
+ return;
+
+ if (matches.CountElements() == 1)
+ {
+ char *pszCmdName = (char *)matches.GetFirst();
+ char *pszRest = pszCmdName + Q_strlen(m_szConsoleText);
+
+ if (pszRest)
+ {
+ Echo(pszRest);
+ Q_strlcat(m_szConsoleText, pszRest);
+ m_nConsoleTextLen += Q_strlen(pszRest);
+
+ Echo(" ");
+ Q_strlcat(m_szConsoleText, " ");
+ m_nConsoleTextLen++;
+ }
+ }
+ else
+ {
+ int nLongestCmd = 0;
+ int nSmallestCmd = 0;
+ int nCurrentColumn;
+ int nTotalColumns;
+ char szCommonCmd[256]; // Should be enough.
+ char szFormatCmd[256];
+ char *pszSmallestCmd;
+ char *pszCurrentCmd = (char *)matches.GetFirst();
+ nSmallestCmd = Q_strlen(pszCurrentCmd);
+ pszSmallestCmd = pszCurrentCmd;
+ while (pszCurrentCmd)
+ {
+ if ((int)Q_strlen(pszCurrentCmd) > nLongestCmd)
+ {
+ nLongestCmd = Q_strlen(pszCurrentCmd);
+ }
+ if ((int)Q_strlen(pszCurrentCmd) < nSmallestCmd)
+ {
+ nSmallestCmd = Q_strlen(pszCurrentCmd);
+ pszSmallestCmd = pszCurrentCmd;
+ }
+ pszCurrentCmd = (char *)matches.GetNext();
+ }
+
+ nTotalColumns = (GetWidth() - 1) / (nLongestCmd + 1);
+ nCurrentColumn = 0;
+
+ Echo("\n");
+ Q_strcpy(szCommonCmd, pszSmallestCmd);
+
+ // Would be nice if these were sorted, but not that big a deal
+ pszCurrentCmd = (char *)matches.GetFirst();
+ while (pszCurrentCmd)
+ {
+ if (++nCurrentColumn > nTotalColumns)
+ {
+ Echo("\n");
+ nCurrentColumn = 1;
+ }
+
+ Q_snprintf(szFormatCmd, sizeof(szFormatCmd), "%-*s ", nLongestCmd, pszCurrentCmd);
+ Echo(szFormatCmd);
+ for (char *pCur = pszCurrentCmd, *pCommon = szCommonCmd; (*pCur && *pCommon); pCur++, pCommon++)
+ {
+ if (*pCur != *pCommon)
+ {
+ *pCommon = 0;
+ break;
+ }
+ }
+
+ pszCurrentCmd = (char *)matches.GetNext();
+ }
+
+ Echo("\n");
+ if (Q_strcmp(szCommonCmd, m_szConsoleText))
+ {
+ Q_strcpy(m_szConsoleText, szCommonCmd);
+ m_nConsoleTextLen = Q_strlen(szCommonCmd);
+ }
+
+ Echo(m_szConsoleText);
+ }
+
+ m_nCursorPosition = m_nConsoleTextLen;
+ m_nBrowseLine = m_nInputLine;
+}
+
+void CTextConsole::ReceiveStandardChar(const char ch)
+{
+ int nCount;
+
+ // If the line buffer is maxed out, ignore this char
+ if (m_nConsoleTextLen >= (sizeof(m_szConsoleText) - 2))
+ {
+ return;
+ }
+
+ nCount = m_nConsoleTextLen;
+ while (nCount > m_nCursorPosition)
+ {
+ m_szConsoleText[ nCount ] = m_szConsoleText[ nCount - 1 ];
+ nCount--;
+ }
+
+ m_szConsoleText[ m_nCursorPosition ] = ch;
+
+ Echo(m_szConsoleText + m_nCursorPosition, m_nConsoleTextLen - m_nCursorPosition + 1);
+
+ m_nConsoleTextLen++;
+ m_nCursorPosition++;
+
+ nCount = m_nConsoleTextLen;
+ while (nCount > m_nCursorPosition)
+ {
+ Echo("\b");
+ nCount--;
+ }
+
+ m_nBrowseLine = m_nInputLine;
+}
+
+void CTextConsole::ReceiveUpArrow()
+{
+ int nLastCommandInHistory = m_nInputLine + 1;
+ if (nLastCommandInHistory > m_nTotalLines)
+ nLastCommandInHistory = 0;
+
+ if (m_nBrowseLine == nLastCommandInHistory)
+ return;
+
+ if (m_nBrowseLine == m_nInputLine)
+ {
+ if (m_nConsoleTextLen > 0)
+ {
+ // Save off current text
+ Q_strncpy(m_szSavedConsoleText, m_szConsoleText, m_nConsoleTextLen);
+ // No terminator, it's a raw buffer we always know the length of
+ }
+
+ m_nSavedConsoleTextLen = m_nConsoleTextLen;
+ }
+
+ m_nBrowseLine--;
+ if (m_nBrowseLine < 0)
+ {
+ m_nBrowseLine = m_nTotalLines - 1;
+ }
+
+ // delete old line
+ while (m_nConsoleTextLen--)
+ {
+ Echo("\b \b");
+ }
+
+ // copy buffered line
+ Echo(m_aszLineBuffer[ m_nBrowseLine ]);
+
+ Q_strncpy(m_szConsoleText, m_aszLineBuffer[ m_nBrowseLine ], MAX_CONSOLE_TEXTLEN);
+
+ m_nConsoleTextLen = Q_strlen(m_aszLineBuffer[ m_nBrowseLine ]);
+ m_nCursorPosition = m_nConsoleTextLen;
+}
+
+void CTextConsole::ReceiveDownArrow()
+{
+ if (m_nBrowseLine == m_nInputLine)
+ return;
+
+ if (++m_nBrowseLine > m_nTotalLines)
+ m_nBrowseLine = 0;
+
+ // delete old line
+ while (m_nConsoleTextLen--)
+ {
+ Echo("\b \b");
+ }
+
+ if (m_nBrowseLine == m_nInputLine)
+ {
+ if (m_nSavedConsoleTextLen > 0)
+ {
+ // Restore current text
+ Q_strncpy(m_szConsoleText, m_szSavedConsoleText, m_nSavedConsoleTextLen);
+ // No terminator, it's a raw buffer we always know the length of
+
+ Echo(m_szConsoleText, m_nSavedConsoleTextLen);
+ }
+
+ m_nConsoleTextLen = m_nSavedConsoleTextLen;
+ }
+ else
+ {
+ // copy buffered line
+ Echo(m_aszLineBuffer[ m_nBrowseLine ]);
+ Q_strncpy(m_szConsoleText, m_aszLineBuffer[ m_nBrowseLine ], MAX_CONSOLE_TEXTLEN);
+ m_nConsoleTextLen = Q_strlen(m_aszLineBuffer[ m_nBrowseLine ]);
+ }
+
+ m_nCursorPosition = m_nConsoleTextLen;
+}
+
+void CTextConsole::ReceiveLeftArrow()
+{
+ if (m_nCursorPosition == 0)
+ return;
+
+ Echo("\b");
+ m_nCursorPosition--;
+}
+
+void CTextConsole::ReceiveRightArrow()
+{
+ if (m_nCursorPosition == m_nConsoleTextLen)
+ return;
+
+ Echo(m_szConsoleText + m_nCursorPosition, 1);
+ m_nCursorPosition++;
+}
diff --git a/dep/hlsdk/common/textconsole.h b/dep/hlsdk/common/textconsole.h
new file mode 100644
index 0000000..9d1b67e
--- /dev/null
+++ b/dep/hlsdk/common/textconsole.h
@@ -0,0 +1,95 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "IBaseSystem.h"
+
+#define MAX_CONSOLE_TEXTLEN 256
+#define MAX_BUFFER_LINES 30
+
+class CTextConsole {
+public:
+ virtual ~CTextConsole() {}
+
+ virtual bool Init(IBaseSystem *system = nullptr);
+ virtual void ShutDown();
+ virtual void Print(char *pszMsg);
+
+ virtual void SetTitle(char *pszTitle) {}
+ virtual void SetStatusLine(char *pszStatus) {}
+ virtual void UpdateStatus() {}
+
+ // Must be provided by children
+ virtual void PrintRaw(char *pszMsg, int nChars = 0) = 0;
+ virtual void Echo(char *pszMsg, int nChars = 0) = 0;
+ virtual char *GetLine() = 0;
+ virtual int GetWidth() = 0;
+
+ virtual void SetVisible(bool visible);
+ virtual bool IsVisible();
+
+ void InitSystem(IBaseSystem *system);
+
+protected:
+ char m_szConsoleText[MAX_CONSOLE_TEXTLEN]; // console text buffer
+ int m_nConsoleTextLen; // console textbuffer length
+ int m_nCursorPosition; // position in the current input line
+
+ // Saved input data when scrolling back through command history
+ char m_szSavedConsoleText[MAX_CONSOLE_TEXTLEN]; // console text buffer
+ int m_nSavedConsoleTextLen; // console textbuffer length
+
+ char m_aszLineBuffer[MAX_BUFFER_LINES][MAX_CONSOLE_TEXTLEN]; // command buffer last MAX_BUFFER_LINES commands
+ int m_nInputLine; // Current line being entered
+ int m_nBrowseLine; // current buffer line for up/down arrow
+ int m_nTotalLines; // # of nonempty lines in the buffer
+
+ bool m_ConsoleVisible;
+
+ IBaseSystem *m_System;
+
+ int ReceiveNewline();
+ void ReceiveBackspace();
+ void ReceiveTab();
+ void ReceiveStandardChar(const char ch);
+ void ReceiveUpArrow();
+ void ReceiveDownArrow();
+ void ReceiveLeftArrow();
+ void ReceiveRightArrow();
+};
+
+#include "SteamAppStartUp.h"
+
+#if defined(_WIN32)
+ #include "TextConsoleWin32.h"
+#else
+ #include "TextConsoleUnix.h"
+#endif // defined(_WIN32)
+
+void Sys_Printf(char *fmt, ...);
diff --git a/dep/hlsdk/common/triangleapi.h b/dep/hlsdk/common/triangleapi.h
new file mode 100644
index 0000000..c1d6bd0
--- /dev/null
+++ b/dep/hlsdk/common/triangleapi.h
@@ -0,0 +1,64 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#if !defined( TRIANGLEAPIH )
+#define TRIANGLEAPIH
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef enum
+{
+ TRI_FRONT = 0,
+ TRI_NONE = 1,
+} TRICULLSTYLE;
+
+#define TRI_API_VERSION 1
+
+#define TRI_TRIANGLES 0
+#define TRI_TRIANGLE_FAN 1
+#define TRI_QUADS 2
+#define TRI_POLYGON 3
+#define TRI_LINES 4
+#define TRI_TRIANGLE_STRIP 5
+#define TRI_QUAD_STRIP 6
+
+typedef struct triangleapi_s
+{
+ int version;
+
+ void ( *RenderMode )( int mode );
+ void ( *Begin )( int primitiveCode );
+ void ( *End ) ( void );
+
+ void ( *Color4f ) ( float r, float g, float b, float a );
+ void ( *Color4ub ) ( unsigned char r, unsigned char g, unsigned char b, unsigned char a );
+ void ( *TexCoord2f ) ( float u, float v );
+ void ( *Vertex3fv ) ( float *worldPnt );
+ void ( *Vertex3f ) ( float x, float y, float z );
+ void ( *Brightness ) ( float brightness );
+ void ( *CullFace ) ( TRICULLSTYLE style );
+ int ( *SpriteTexture ) ( struct model_s *pSpriteModel, int frame );
+ int ( *WorldToScreen ) ( float *world, float *screen ); // Returns 1 if it's z clipped
+ void ( *Fog ) ( float flFogColor[3], float flStart, float flEnd, int bOn ); // Works just like GL_FOG, flFogColor is r/g/b.
+ void ( *ScreenToWorld ) ( float *screen, float *world );
+ void ( *GetMatrix ) ( const int pname, float *matrix );
+ int ( *BoxInPVS ) ( float *mins, float *maxs );
+ void ( *LightAtPoint ) ( float *pos, float *value );
+ void ( *Color4fRendermode ) ( float r, float g, float b, float a, int rendermode );
+ void ( *FogParams ) ( float flDensity, int iFogSkybox ); // Used with Fog()...sets fog density and whether the fog should be applied to the skybox
+
+} triangleapi_t;
+
+#endif // !TRIANGLEAPIH
diff --git a/dep/hlsdk/common/usercmd.h b/dep/hlsdk/common/usercmd.h
new file mode 100644
index 0000000..3411769
--- /dev/null
+++ b/dep/hlsdk/common/usercmd.h
@@ -0,0 +1,41 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+#ifndef USERCMD_H
+#define USERCMD_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+typedef struct usercmd_s
+{
+ short lerp_msec; // Interpolation time on client
+ byte msec; // Duration in ms of command
+ vec3_t viewangles; // Command view angles.
+
+// intended velocities
+ float forwardmove; // Forward velocity.
+ float sidemove; // Sideways velocity.
+ float upmove; // Upward velocity.
+ byte lightlevel; // Light level at spot where we are standing.
+ unsigned short buttons; // Attack buttons
+ byte impulse; // Impulse command issued.
+ byte weaponselect; // Current weapon id
+
+// Experimental player impact stuff.
+ int impact_index;
+ vec3_t impact_position;
+} usercmd_t;
+
+#endif // USERCMD_H
diff --git a/dep/hlsdk/common/vmodes.h b/dep/hlsdk/common/vmodes.h
new file mode 100644
index 0000000..9765bca
--- /dev/null
+++ b/dep/hlsdk/common/vmodes.h
@@ -0,0 +1,33 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+
+typedef struct rect_s
+{
+ int left, right, top, bottom;
+} wrect_t;
diff --git a/dep/hlsdk/common/weaponinfo.h b/dep/hlsdk/common/weaponinfo.h
new file mode 100644
index 0000000..5abd38b
--- /dev/null
+++ b/dep/hlsdk/common/weaponinfo.h
@@ -0,0 +1,53 @@
+/***
+*
+* Copyright (c) 1996-2002, Valve LLC. All rights reserved.
+*
+* This product contains software technology licensed from Id
+* Software, Inc. ("Id Technology"). Id Technology (c) 1996 Id Software, Inc.
+* All Rights Reserved.
+*
+* Use, distribution, and modification of this source code and/or resulting
+* object code is restricted to non-commercial enhancements to products from
+* Valve LLC. All other use, distribution, or modification is prohibited
+* without written permission from Valve LLC.
+*
+****/
+
+#ifndef WEAPONINFO_H
+#define WEAPONINFO_H
+#ifdef _WIN32
+#pragma once
+#endif
+
+// Info about weapons player might have in his/her possession
+typedef struct weapon_data_s
+{
+ int m_iId;
+ int m_iClip;
+
+ float m_flNextPrimaryAttack;
+ float m_flNextSecondaryAttack;
+ float m_flTimeWeaponIdle;
+
+ int m_fInReload;
+ int m_fInSpecialReload;
+ float m_flNextReload;
+ float m_flPumpTime;
+ float m_fReloadTime;
+
+ float m_fAimedDamage;
+ float m_fNextAimBonus;
+ int m_fInZoom;
+ int m_iWeaponState;
+
+ int iuser1;
+ int iuser2;
+ int iuser3;
+ int iuser4;
+ float fuser1;
+ float fuser2;
+ float fuser3;
+ float fuser4;
+} weapon_data_t;
+
+#endif // WEAPONINFO_H
diff --git a/dep/hlsdk/common/winsani_in.h b/dep/hlsdk/common/winsani_in.h
new file mode 100644
index 0000000..d8c8527
--- /dev/null
+++ b/dep/hlsdk/common/winsani_in.h
@@ -0,0 +1,7 @@
+#if _MSC_VER >= 1500 // MSVC++ 9.0 (Visual Studio 2008)
+#pragma push_macro("ARRAYSIZE")
+#ifdef ARRAYSIZE
+#undef ARRAYSIZE
+#endif
+#define HSPRITE WINDOWS_HSPRITE
+#endif
diff --git a/dep/hlsdk/common/winsani_out.h b/dep/hlsdk/common/winsani_out.h
new file mode 100644
index 0000000..2726950
--- /dev/null
+++ b/dep/hlsdk/common/winsani_out.h
@@ -0,0 +1,4 @@
+#if _MSC_VER >= 1500 // MSVC++ 9.0 (Visual Studio 2008)
+#undef HSPRITE
+#pragma pop_macro("ARRAYSIZE")
+#endif
diff --git a/dep/hlsdk/dlls/activity.h b/dep/hlsdk/dlls/activity.h
new file mode 100644
index 0000000..d854e9a
--- /dev/null
+++ b/dep/hlsdk/dlls/activity.h
@@ -0,0 +1,146 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+typedef enum Activity_s
+{
+ ACT_INVALID = -1,
+
+ ACT_RESET = 0, // Set m_Activity to this invalid value to force a reset to m_IdealActivity
+ ACT_IDLE,
+ ACT_GUARD,
+ ACT_WALK,
+ ACT_RUN,
+ ACT_FLY, // Fly (and flap if appropriate)
+ ACT_SWIM,
+ ACT_HOP, // vertical jump
+ ACT_LEAP, // long forward jump
+ ACT_FALL,
+ ACT_LAND,
+ ACT_STRAFE_LEFT,
+ ACT_STRAFE_RIGHT,
+ ACT_ROLL_LEFT, // tuck and roll, left
+ ACT_ROLL_RIGHT, // tuck and roll, right
+ ACT_TURN_LEFT, // turn quickly left (stationary)
+ ACT_TURN_RIGHT, // turn quickly right (stationary)
+ ACT_CROUCH, // the act of crouching down from a standing position
+ ACT_CROUCHIDLE, // holding body in crouched position (loops)
+ ACT_STAND, // the act of standing from a crouched position
+ ACT_USE,
+ ACT_SIGNAL1,
+ ACT_SIGNAL2,
+ ACT_SIGNAL3,
+ ACT_TWITCH,
+ ACT_COWER,
+ ACT_SMALL_FLINCH,
+ ACT_BIG_FLINCH,
+ ACT_RANGE_ATTACK1,
+ ACT_RANGE_ATTACK2,
+ ACT_MELEE_ATTACK1,
+ ACT_MELEE_ATTACK2,
+ ACT_RELOAD,
+ ACT_ARM, // pull out gun, for instance
+ ACT_DISARM, // reholster gun
+ ACT_EAT, // monster chowing on a large food item (loop)
+ ACT_DIESIMPLE,
+ ACT_DIEBACKWARD,
+ ACT_DIEFORWARD,
+ ACT_DIEVIOLENT,
+ ACT_BARNACLE_HIT, // barnacle tongue hits a monster
+ ACT_BARNACLE_PULL, // barnacle is lifting the monster ( loop )
+ ACT_BARNACLE_CHOMP, // barnacle latches on to the monster
+ ACT_BARNACLE_CHEW, // barnacle is holding the monster in its mouth ( loop )
+ ACT_SLEEP,
+ ACT_INSPECT_FLOOR, // for active idles, look at something on or near the floor
+ ACT_INSPECT_WALL, // for active idles, look at something directly ahead of you ( doesn't HAVE to be a wall or on a wall )
+ ACT_IDLE_ANGRY, // alternate idle animation in which the monster is clearly agitated. (loop)
+ ACT_WALK_HURT, // limp (loop)
+ ACT_RUN_HURT, // limp (loop)
+ ACT_HOVER, // Idle while in flight
+ ACT_GLIDE, // Fly (don't flap)
+ ACT_FLY_LEFT, // Turn left in flight
+ ACT_FLY_RIGHT, // Turn right in flight
+ ACT_DETECT_SCENT, // this means the monster smells a scent carried by the air
+ ACT_SNIFF, // this is the act of actually sniffing an item in front of the monster
+ ACT_BITE, // some large monsters can eat small things in one bite. This plays one time, EAT loops.
+ ACT_THREAT_DISPLAY, // without attacking, monster demonstrates that it is angry. (Yell, stick out chest, etc )
+ ACT_FEAR_DISPLAY, // monster just saw something that it is afraid of
+ ACT_EXCITED, // for some reason, monster is excited. Sees something he really likes to eat, or whatever.
+ ACT_SPECIAL_ATTACK1, // very monster specific special attacks.
+ ACT_SPECIAL_ATTACK2,
+ ACT_COMBAT_IDLE, // agitated idle.
+ ACT_WALK_SCARED,
+ ACT_RUN_SCARED,
+ ACT_VICTORY_DANCE, // killed a player, do a victory dance.
+ ACT_DIE_HEADSHOT, // die, hit in head.
+ ACT_DIE_CHESTSHOT, // die, hit in chest
+ ACT_DIE_GUTSHOT, // die, hit in gut
+ ACT_DIE_BACKSHOT, // die, hit in back
+ ACT_FLINCH_HEAD,
+ ACT_FLINCH_CHEST,
+ ACT_FLINCH_STOMACH,
+ ACT_FLINCH_LEFTARM,
+ ACT_FLINCH_RIGHTARM,
+ ACT_FLINCH_LEFTLEG,
+ ACT_FLINCH_RIGHTLEG,
+ ACT_FLINCH,
+ ACT_LARGE_FLINCH,
+ ACT_HOLDBOMB,
+ ACT_IDLE_FIDGET,
+ ACT_IDLE_SCARED,
+ ACT_IDLE_SCARED_FIDGET,
+ ACT_FOLLOW_IDLE,
+ ACT_FOLLOW_IDLE_FIDGET,
+ ACT_FOLLOW_IDLE_SCARED,
+ ACT_FOLLOW_IDLE_SCARED_FIDGET,
+ ACT_CROUCH_IDLE,
+ ACT_CROUCH_IDLE_FIDGET,
+ ACT_CROUCH_IDLE_SCARED,
+ ACT_CROUCH_IDLE_SCARED_FIDGET,
+ ACT_CROUCH_WALK,
+ ACT_CROUCH_WALK_SCARED,
+ ACT_CROUCH_DIE,
+ ACT_WALK_BACK,
+ ACT_IDLE_SNEAKY,
+ ACT_IDLE_SNEAKY_FIDGET,
+ ACT_WALK_SNEAKY,
+ ACT_WAVE,
+ ACT_YES,
+ ACT_NO,
+
+} Activity;
+
+typedef struct
+{
+ int type;
+ char *name;
+
+} activity_map_t;
+
+extern activity_map_t activity_map[];
diff --git a/dep/hlsdk/dlls/activitymap.h b/dep/hlsdk/dlls/activitymap.h
new file mode 100644
index 0000000..17e5be2
--- /dev/null
+++ b/dep/hlsdk/dlls/activitymap.h
@@ -0,0 +1,111 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#define _A(a)\
+ { a, #a }
+
+activity_map_t activity_map[] =
+{
+ _A(ACT_IDLE),
+ _A(ACT_GUARD),
+ _A(ACT_WALK),
+ _A(ACT_RUN),
+ _A(ACT_FLY),
+ _A(ACT_SWIM),
+ _A(ACT_HOP),
+ _A(ACT_LEAP),
+ _A(ACT_FALL),
+ _A(ACT_LAND),
+ _A(ACT_STRAFE_LEFT),
+ _A(ACT_STRAFE_RIGHT),
+ _A(ACT_ROLL_LEFT),
+ _A(ACT_ROLL_RIGHT),
+ _A(ACT_TURN_LEFT),
+ _A(ACT_TURN_RIGHT),
+ _A(ACT_CROUCH),
+ _A(ACT_CROUCHIDLE),
+ _A(ACT_STAND),
+ _A(ACT_USE),
+ _A(ACT_SIGNAL1),
+ _A(ACT_SIGNAL2),
+ _A(ACT_SIGNAL3),
+ _A(ACT_TWITCH),
+ _A(ACT_COWER),
+ _A(ACT_SMALL_FLINCH),
+ _A(ACT_BIG_FLINCH),
+ _A(ACT_RANGE_ATTACK1),
+ _A(ACT_RANGE_ATTACK2),
+ _A(ACT_MELEE_ATTACK1),
+ _A(ACT_MELEE_ATTACK2),
+ _A(ACT_RELOAD),
+ _A(ACT_ARM),
+ _A(ACT_DISARM),
+ _A(ACT_EAT),
+ _A(ACT_DIESIMPLE),
+ _A(ACT_DIEBACKWARD),
+ _A(ACT_DIEFORWARD),
+ _A(ACT_DIEVIOLENT),
+ _A(ACT_BARNACLE_HIT),
+ _A(ACT_BARNACLE_PULL),
+ _A(ACT_BARNACLE_CHOMP),
+ _A(ACT_BARNACLE_CHEW),
+ _A(ACT_SLEEP),
+ _A(ACT_INSPECT_FLOOR),
+ _A(ACT_INSPECT_WALL),
+ _A(ACT_IDLE_ANGRY),
+ _A(ACT_WALK_HURT),
+ _A(ACT_RUN_HURT),
+ _A(ACT_HOVER),
+ _A(ACT_GLIDE),
+ _A(ACT_FLY_LEFT),
+ _A(ACT_FLY_RIGHT),
+ _A(ACT_DETECT_SCENT),
+ _A(ACT_SNIFF),
+ _A(ACT_BITE),
+ _A(ACT_THREAT_DISPLAY),
+ _A(ACT_FEAR_DISPLAY),
+ _A(ACT_EXCITED),
+ _A(ACT_SPECIAL_ATTACK1),
+ _A(ACT_SPECIAL_ATTACK2),
+ _A(ACT_COMBAT_IDLE),
+ _A(ACT_WALK_SCARED),
+ _A(ACT_RUN_SCARED),
+ _A(ACT_VICTORY_DANCE),
+ _A(ACT_DIE_HEADSHOT),
+ _A(ACT_DIE_CHESTSHOT),
+ _A(ACT_DIE_GUTSHOT),
+ _A(ACT_DIE_BACKSHOT),
+ _A(ACT_FLINCH_HEAD),
+ _A(ACT_FLINCH_CHEST),
+ _A(ACT_FLINCH_STOMACH),
+ _A(ACT_FLINCH_LEFTARM),
+ _A(ACT_FLINCH_RIGHTARM),
+ _A(ACT_FLINCH_LEFTLEG),
+ _A(ACT_FLINCH_RIGHTLEG),
+ 0, nullptr
+};
diff --git a/dep/hlsdk/dlls/airtank.h b/dep/hlsdk/dlls/airtank.h
new file mode 100644
index 0000000..90de064
--- /dev/null
+++ b/dep/hlsdk/dlls/airtank.h
@@ -0,0 +1,43 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class CAirtank: public CGrenade {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Killed(entvars_t *pevAttacker, int iGib) = 0;
+ virtual int BloodColor() = 0;
+
+ int GetState() const { return m_state; }
+private:
+ int m_state;
+};
diff --git a/dep/hlsdk/dlls/ammo.h b/dep/hlsdk/dlls/ammo.h
new file mode 100644
index 0000000..8c41ff6
--- /dev/null
+++ b/dep/hlsdk/dlls/ammo.h
@@ -0,0 +1,99 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class C9MMAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
+
+class CBuckShotAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
+
+class C556NatoAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
+
+class C556NatoBoxAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
+
+class C762NatoAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
+
+class C45ACPAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
+
+class C50AEAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
+
+class C338MagnumAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
+
+class C57MMAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
+
+class C357SIGAmmo: public CBasePlayerAmmo {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL AddAmmo(CBaseEntity *pOther) = 0;
+};
diff --git a/dep/hlsdk/dlls/basemonster.h b/dep/hlsdk/dlls/basemonster.h
new file mode 100644
index 0000000..c26746e
--- /dev/null
+++ b/dep/hlsdk/dlls/basemonster.h
@@ -0,0 +1,118 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "gib.h"
+#include "activity.h"
+
+enum
+{
+ ITBD_PARALLYZE = 0,
+ ITBD_NERVE_GAS,
+ ITBD_POISON,
+ ITBD_RADIATION,
+ ITBD_DROWN_RECOVER,
+ ITBD_ACID,
+ ITBD_SLOW_BURN,
+ ITBD_SLOW_FREEZE,
+ ITBD_END
+};
+
+enum MONSTERSTATE
+{
+ MONSTERSTATE_NONE = 0,
+ MONSTERSTATE_IDLE,
+ MONSTERSTATE_COMBAT,
+ MONSTERSTATE_ALERT,
+ MONSTERSTATE_HUNT,
+ MONSTERSTATE_PRONE,
+ MONSTERSTATE_SCRIPT,
+ MONSTERSTATE_PLAYDEAD,
+ MONSTERSTATE_DEAD
+};
+
+class CBaseToggle;
+class CBaseMonster: public CBaseToggle {
+public:
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual void TraceAttack(entvars_t *pevAttacker, float flDamage, Vector vecDir, TraceResult *ptr, int bitsDamageType) = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+ virtual BOOL TakeHealth(float flHealth, int bitsDamageType) = 0;
+ virtual void Killed(entvars_t *pevAttacker, int iGib) = 0;
+ virtual int BloodColor() = 0;
+ virtual BOOL IsAlive() = 0;
+ virtual float ChangeYaw(int speed) = 0;
+ virtual BOOL HasHumanGibs() = 0;
+ virtual BOOL HasAlienGibs() = 0;
+ virtual void FadeMonster() = 0;
+ virtual void GibMonster() = 0;
+ virtual Activity GetDeathActivity() = 0;
+ virtual void BecomeDead() = 0;
+ virtual BOOL ShouldFadeOnDeath() = 0;
+ virtual int IRelationship(CBaseEntity *pTarget) = 0;
+ virtual void PainSound() = 0;
+ virtual void ResetMaxSpeed() = 0;
+ virtual void ReportAIState() = 0;
+ virtual void MonsterInitDead() = 0;
+ virtual void Look(int iDistance) = 0;
+ virtual CBaseEntity *BestVisibleEnemy() = 0;
+ virtual BOOL FInViewCone(CBaseEntity *pEntity) = 0;
+ virtual BOOL FInViewCone(const Vector *pOrigin) = 0;
+public:
+ void SetConditions(int iConditions) { m_afConditions |= iConditions; }
+ void ClearConditions(int iConditions) { m_afConditions &= ~iConditions; }
+ BOOL HasConditions(int iConditions) { return (m_afConditions & iConditions) ? TRUE : FALSE; }
+ BOOL HasAllConditions(int iConditions) { return ((m_afConditions & iConditions) == iConditions) ? TRUE : FALSE; }
+
+ void Remember(int iMemory) { m_afMemory |= iMemory; }
+ void Forget(int iMemory) { m_afMemory &= ~iMemory; }
+ BOOL HasMemory(int iMemory) { return (m_afMemory & iMemory) ? TRUE : FALSE; }
+ BOOL HasAllMemories(int iMemory) { return ((m_afMemory & iMemory) == iMemory) ? TRUE : FALSE; }
+
+ void StopAnimation() { pev->framerate = 0.0f; }
+public:
+ Activity m_Activity; // what the monster is doing (animation)
+ Activity m_IdealActivity; // monster should switch to this activity
+ int m_LastHitGroup; // the last body region that took damage
+ int m_bitsDamageType; // what types of damage has monster (player) taken
+ byte m_rgbTimeBasedDamage[ITBD_END];
+
+ MONSTERSTATE m_MonsterState; // monster's current state
+ MONSTERSTATE m_IdealMonsterState; // monster should change to this state
+ int m_afConditions;
+ int m_afMemory;
+
+ float m_flNextAttack; // cannot attack again until this time
+ EHANDLE m_hEnemy; // the entity that the monster is fighting.
+ EHANDLE m_hTargetEnt; // the entity that the monster is trying to reach
+ float m_flFieldOfView; // width of monster's field of view (dot product)
+ int m_bloodColor; // color of blood particless
+ Vector m_HackedGunPos; // HACK until we can query end of gun
+ Vector m_vecEnemyLKP; // last known position of enemy. (enemy's origin)
+};
diff --git a/dep/hlsdk/dlls/bmodels.h b/dep/hlsdk/dlls/bmodels.h
new file mode 100644
index 0000000..cc24fa6
--- /dev/null
+++ b/dep/hlsdk/dlls/bmodels.h
@@ -0,0 +1,149 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+// covering cheesy noise1, noise2, & noise3 fields so they make more sense (for rotating fans)
+#define noiseStart noise1
+#define noiseStop noise2
+#define noiseRunning noise3
+
+// This is just a solid wall if not inhibited
+class CFuncWall: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+
+ // Bmodels don't go across transitions
+ virtual int ObjectCaps() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+};
+
+#define SF_WALL_TOOGLE_START_OFF BIT(0)
+#define SF_WALL_TOOGLE_NOTSOLID BIT(3)
+
+class CFuncWallToggle: public CFuncWall {
+public:
+ virtual void Spawn() = 0;
+ virtual void Restart() = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+};
+
+#define SF_CONVEYOR_VISUAL BIT(0)
+#define SF_CONVEYOR_NOTSOLID BIT(1)
+
+class CFuncConveyor: public CFuncWall {
+public:
+ virtual void Spawn() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+};
+
+// A simple entity that looks solid but lets you walk through it.
+class CFuncIllusionary: public CBaseToggle {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int ObjectCaps() = 0;
+};
+
+// Monster only clip brush
+//
+// This brush will be solid for any entity who has the FL_MONSTERCLIP flag set
+// in pev->flags
+//
+// otherwise it will be invisible and not solid. This can be used to keep
+// specific monsters out of certain areas
+class CFuncMonsterClip: public CFuncWall {
+public:
+ virtual void Spawn() = 0;
+
+ // Clear out func_wall's use function
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+};
+
+#define SF_BRUSH_ROTATE_START_ON BIT(0)
+#define SF_BRUSH_ROTATE_BACKWARDS BIT(1)
+#define SF_BRUSH_ROTATE_Z_AXIS BIT(2)
+#define SF_BRUSH_ROTATE_X_AXIS BIT(3)
+#define SF_BRUSH_ACCDCC BIT(4) // Brush should accelerate and decelerate when toggled
+#define SF_BRUSH_HURT BIT(5) // Rotating brush that inflicts pain based on rotation speed
+#define SF_BRUSH_ROTATE_NOT_SOLID BIT(6) // Some special rotating objects are not solid.
+#define SF_BRUSH_ROTATE_SMALLRADIUS BIT(7)
+#define SF_BRUSH_ROTATE_MEDIUMRADIUS BIT(8)
+#define SF_BRUSH_ROTATE_LARGERADIUS BIT(9)
+
+const int MAX_FANPITCH = 100;
+const int MIN_FANPITCH = 30;
+
+class CFuncRotating: public CBaseEntity {
+public:
+ // basic functions
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Restart() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Blocked(CBaseEntity *pOther) = 0;
+public:
+
+ float m_flFanFriction;
+ float m_flAttenuation;
+ float m_flVolume;
+ float m_pitch;
+ int m_sounds;
+
+ Vector m_angles;
+};
+
+#define SF_PENDULUM_START_ON BIT(0)
+#define SF_PENDULUM_SWING BIT(1) // Spawnflag that makes a pendulum a rope swing
+#define SF_PENDULUM_PASSABLE BIT(3)
+#define SF_PENDULUM_AUTO_RETURN BIT(4)
+
+class CPendulum: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Touch(CBaseEntity *pOther) = 0;
+ virtual void Blocked(CBaseEntity *pOther) = 0;
+public:
+ float m_accel; // Acceleration
+ float m_distance;
+ float m_time;
+ float m_damp;
+ float m_maxSpeed;
+ float m_dampSpeed;
+
+ Vector m_center;
+ Vector m_start;
+};
diff --git a/dep/hlsdk/dlls/bot/cs_bot.h b/dep/hlsdk/dlls/bot/cs_bot.h
new file mode 100644
index 0000000..28c4d86
--- /dev/null
+++ b/dep/hlsdk/dlls/bot/cs_bot.h
@@ -0,0 +1,639 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "bot/cs_gamestate.h"
+#include "bot/cs_bot_manager.h"
+#include "bot/cs_bot_chatter.h"
+
+const int MAX_BUY_WEAPON_PRIMARY = 13;
+const int MAX_BUY_WEAPON_SECONDARY = 3;
+
+enum
+{
+ BOT_PROGGRESS_DRAW = 0, // draw status bar progress
+ BOT_PROGGRESS_START, // init status bar progress
+ BOT_PROGGRESS_HIDE, // hide status bar progress
+};
+
+extern int _navAreaCount;
+extern int _currentIndex;
+
+extern struct BuyInfo primaryWeaponBuyInfoCT[MAX_BUY_WEAPON_PRIMARY];
+extern struct BuyInfo secondaryWeaponBuyInfoCT[MAX_BUY_WEAPON_SECONDARY];
+
+extern struct BuyInfo primaryWeaponBuyInfoT[MAX_BUY_WEAPON_PRIMARY];
+extern struct BuyInfo secondaryWeaponBuyInfoT[MAX_BUY_WEAPON_SECONDARY];
+
+class CCSBot;
+class BotChatterInterface;
+
+class BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const = 0;
+};
+
+class IdleState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual const char *GetName() const { return "Idle"; }
+};
+
+class HuntState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "Hunt"; }
+public:
+ CNavArea *m_huntArea;
+};
+
+class AttackState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "Attack"; }
+public:
+ enum DodgeStateType
+ {
+ STEADY_ON,
+ SLIDE_LEFT,
+ SLIDE_RIGHT,
+ JUMP,
+ NUM_ATTACK_STATES
+ } m_dodgeState;
+
+ float m_nextDodgeStateTimestamp;
+ CountdownTimer m_repathTimer;
+ float m_scopeTimestamp;
+ bool m_haveSeenEnemy;
+ bool m_isEnemyHidden;
+ float m_reacquireTimestamp;
+ float m_shieldToggleTimestamp;
+ bool m_shieldForceOpen;
+ float m_pinnedDownTimestamp;
+ bool m_crouchAndHold;
+ bool m_didAmbushCheck;
+ bool m_dodge;
+ bool m_firstDodge;
+ bool m_isCoward;
+ CountdownTimer m_retreatTimer;
+};
+
+class InvestigateNoiseState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "InvestigateNoise"; }
+private:
+ void AttendCurrentNoise(CCSBot *me);
+ Vector m_checkNoisePosition;
+};
+
+class BuyState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "Buy"; }
+public:
+ bool m_isInitialDelay;
+ int m_prefRetries;
+ int m_prefIndex;
+ int m_retries;
+ bool m_doneBuying;
+ bool m_buyDefuseKit;
+ bool m_buyGrenade;
+ bool m_buyShield;
+ bool m_buyPistol;
+};
+
+class MoveToState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "MoveTo"; }
+
+ void SetGoalPosition(const Vector &pos) { m_goalPosition = pos; }
+ void SetRouteType(RouteType route) { m_routeType = route; }
+
+private:
+ Vector m_goalPosition;
+ RouteType m_routeType;
+ bool m_radioedPlan;
+ bool m_askedForCover;
+};
+
+class FetchBombState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual const char *GetName() const { return "FetchBomb"; }
+};
+
+class PlantBombState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "PlantBomb"; }
+};
+
+class DefuseBombState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "DefuseBomb"; }
+};
+
+class HideState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "Hide"; }
+
+public:
+ void SetHidingSpot(const Vector &pos) { m_hidingSpot = pos; }
+ const Vector &GetHidingSpot() const { return m_hidingSpot; }
+
+ void SetSearchArea(CNavArea *area) { m_searchFromArea = area; }
+ void SetSearchRange(float range) { m_range = range; }
+
+ void SetDuration(float time) { m_duration = time; }
+ void SetHoldPosition(bool hold) { m_isHoldingPosition = hold; }
+
+ bool IsAtSpot() const { return m_isAtSpot; }
+
+public:
+ CNavArea *m_searchFromArea;
+ float m_range;
+
+ Vector m_hidingSpot;
+ bool m_isAtSpot;
+ float m_duration;
+ bool m_isHoldingPosition;
+ float m_holdPositionTime;
+ bool m_heardEnemy;
+
+ float m_firstHeardEnemyTime;
+ int m_retry;
+ Vector m_leaderAnchorPos;
+};
+
+class EscapeFromBombState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "EscapeFromBomb"; }
+};
+
+class FollowState: public BotState
+{
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "Follow"; }
+
+ void SetLeader(CBasePlayer *leader) { m_leader = leader; }
+
+public:
+ EntityHandle m_leader;
+ Vector m_lastLeaderPos;
+ bool m_isStopped;
+ float m_stoppedTimestamp;
+
+ enum LeaderMotionStateType
+ {
+ INVALID,
+ STOPPED,
+ WALKING,
+ RUNNING
+
+ } m_leaderMotionState;
+
+ IntervalTimer m_leaderMotionStateTime;
+
+ bool m_isSneaking;
+ float m_lastSawLeaderTime;
+ CountdownTimer m_repathInterval;
+
+ IntervalTimer m_walkTime;
+ bool m_isAtWalkSpeed;
+
+ float m_waitTime;
+ CountdownTimer m_idleTimer;
+};
+
+class UseEntityState: public BotState {
+public:
+ virtual void OnEnter(CCSBot *me) {}
+ virtual void OnUpdate(CCSBot *me) {}
+ virtual void OnExit(CCSBot *me) {}
+ virtual const char *GetName() const { return "UseEntity"; }
+
+ void SetEntity(CBaseEntity *entity) { m_entity = entity; }
+
+private:
+ EntityHandle m_entity;
+};
+
+// The Counter-strike Bot
+class CCSBot: public CBot {
+public:
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0; // invoked when injured by something (EXTEND) - returns the amount of damage inflicted
+ virtual void Killed(entvars_t *pevAttacker, int iGib) = 0; // invoked when killed (EXTEND)
+ virtual void RoundRespawn() = 0;
+ virtual void Blind(float duration, float holdTime, float fadeTime, int alpha = 255) = 0; // player blinded by a flashbang
+ virtual void OnTouchingWeapon(CWeaponBox *box) = 0; // invoked when in contact with a CWeaponBox
+
+ virtual bool Initialize(const BotProfile *profile) = 0; // (EXTEND) prepare bot for action
+ virtual void SpawnBot() = 0; // (EXTEND) spawn the bot into the game
+
+ virtual void Upkeep() = 0; // lightweight maintenance, invoked frequently
+ virtual void Update() = 0; // heavyweight algorithms, invoked less often
+
+ virtual void Walk() = 0;
+ virtual bool Jump(bool mustJump = false) = 0; // returns true if jump was started
+
+ virtual void OnEvent(GameEventType event, CBaseEntity *entity = NULL, CBaseEntity *other = NULL) = 0; // invoked when event occurs in the game (some events have NULL entity)
+
+ #define CHECK_FOV true
+ virtual bool IsVisible(const Vector *pos, bool testFOV = false) const = 0; // return true if we can see the point
+ virtual bool IsVisible(CBasePlayer *player, bool testFOV = false, unsigned char *visParts = NULL) const = 0; // return true if we can see any part of the player
+
+ virtual bool IsEnemyPartVisible(VisiblePartType part) const = 0; // if enemy is visible, return the part we see for our current enemy
+
+
+public:
+ const Vector &GetEyePosition() const
+ {
+ m_eyePos = pev->origin + pev->view_ofs;
+ return m_eyePos;
+ }
+public:
+ friend class CCSBotManager;
+
+ // TODO: Get rid of these
+ friend class AttackState;
+ friend class BuyState;
+
+ char m_name[64]; // copied from STRING(pev->netname) for debugging
+
+ // behavior properties
+ float m_combatRange; // desired distance between us and them during gunplay
+ mutable bool m_isRogue; // if true, the bot is a "rogue" and listens to no-one
+ mutable CountdownTimer m_rogueTimer;
+
+ enum MoraleType
+ {
+ TERRIBLE = -3,
+ BAD = -2,
+ NEGATIVE = -1,
+ NEUTRAL = 0,
+ POSITIVE = 1,
+ GOOD = 2,
+ EXCELLENT = 3,
+ };
+
+ MoraleType m_morale; // our current morale, based on our win/loss history
+ bool m_diedLastRound; // true if we died last round
+ float m_safeTime; // duration at the beginning of the round where we feel "safe"
+ bool m_wasSafe; // true if we were in the safe time last update
+ NavRelativeDirType m_blindMoveDir; // which way to move when we're blind
+ bool m_blindFire; // if true, fire weapon while blinded
+
+ // TODO: implement through CountdownTimer
+ float m_surpriseDelay; // when we were surprised
+ float m_surpriseTimestamp;
+
+ bool m_isFollowing; // true if we are following someone
+ EHANDLE m_leader; // the ID of who we are following
+ float m_followTimestamp; // when we started following
+ float m_allowAutoFollowTime; // time when we can auto follow
+
+ CountdownTimer m_hurryTimer; // if valid, bot is in a hurry
+
+ // instances of each possible behavior state, to avoid dynamic memory allocation during runtime
+ IdleState m_idleState;
+ HuntState m_huntState;
+ AttackState m_attackState;
+ InvestigateNoiseState m_investigateNoiseState;
+ BuyState m_buyState;
+ MoveToState m_moveToState;
+ FetchBombState m_fetchBombState;
+ PlantBombState m_plantBombState;
+ DefuseBombState m_defuseBombState;
+ HideState m_hideState;
+ EscapeFromBombState m_escapeFromBombState;
+ FollowState m_followState;
+ UseEntityState m_useEntityState;
+
+ // TODO: Allow multiple simultaneous state machines (look around, etc)
+ BotState *m_state; // current behavior state
+ float m_stateTimestamp; // time state was entered
+ bool m_isAttacking; // if true, special Attack state is overriding the state machine
+
+ // high-level tasks
+ enum TaskType
+ {
+ SEEK_AND_DESTROY,
+ PLANT_BOMB,
+ FIND_TICKING_BOMB,
+ DEFUSE_BOMB,
+ GUARD_TICKING_BOMB,
+ GUARD_BOMB_DEFUSER,
+ GUARD_LOOSE_BOMB,
+ GUARD_BOMB_ZONE,
+ ESCAPE_FROM_BOMB,
+ HOLD_POSITION,
+ FOLLOW,
+ VIP_ESCAPE,
+ GUARD_VIP_ESCAPE_ZONE,
+ COLLECT_HOSTAGES,
+ RESCUE_HOSTAGES,
+ GUARD_HOSTAGES,
+ GUARD_HOSTAGE_RESCUE_ZONE,
+ MOVE_TO_LAST_KNOWN_ENEMY_POSITION,
+ MOVE_TO_SNIPER_SPOT,
+ SNIPING,
+
+ NUM_TASKS
+ };
+ TaskType m_task; // our current task
+ EntityHandle m_taskEntity; // an entity used for our task
+
+ // navigation
+ Vector m_goalPosition;
+ EHandle m_goalEntity;
+
+ CNavArea *m_currentArea; // the nav area we are standing on
+ CNavArea *m_lastKnownArea; // the last area we were in
+ EntityHandle m_avoid; // higher priority player we need to make way for
+ float m_avoidTimestamp;
+ bool m_isJumpCrouching;
+ bool m_isJumpCrouched;
+ float m_jumpCrouchTimestamp;
+
+ // path navigation data
+ enum { _MAX_PATH_LENGTH = 256 };
+ struct ConnectInfo
+ {
+ CNavArea *area; // the area along the path
+ NavTraverseType how; // how to enter this area from the previous one
+ Vector pos; // our movement goal position at this point in the path
+ const CNavLadder *ladder; // if "how" refers to a ladder, this is it
+ }
+ m_path[_MAX_PATH_LENGTH];
+ int m_pathLength;
+ int m_pathIndex;
+ float m_areaEnteredTimestamp;
+
+ CountdownTimer m_repathTimer; // must have elapsed before bot can pathfind again
+
+ mutable CountdownTimer m_avoidFriendTimer; // used to throttle how often we check for friends in our path
+ mutable bool m_isFriendInTheWay; // true if a friend is blocking our path
+ CountdownTimer m_politeTimer; // we'll wait for friend to move until this runs out
+ bool m_isWaitingBehindFriend; // true if we are waiting for a friend to move
+
+ enum LadderNavState
+ {
+ APPROACH_ASCENDING_LADDER, // prepare to scale a ladder
+ APPROACH_DESCENDING_LADDER, // prepare to go down ladder
+ FACE_ASCENDING_LADDER,
+ FACE_DESCENDING_LADDER,
+ MOUNT_ASCENDING_LADDER, // move toward ladder until "on" it
+ MOUNT_DESCENDING_LADDER, // move toward ladder until "on" it
+ ASCEND_LADDER, // go up the ladder
+ DESCEND_LADDER, // go down the ladder
+ DISMOUNT_ASCENDING_LADDER, // get off of the ladder
+ DISMOUNT_DESCENDING_LADDER, // get off of the ladder
+ MOVE_TO_DESTINATION, // dismount ladder and move to destination area
+ }
+ m_pathLadderState;
+ bool m_pathLadderFaceIn; // if true, face towards ladder, otherwise face away
+ const CNavLadder *m_pathLadder; // the ladder we need to use to reach the next area
+ NavRelativeDirType m_pathLadderDismountDir; // which way to dismount
+ float m_pathLadderDismountTimestamp; // time when dismount started
+ float m_pathLadderEnd; // if ascending, z of top, if descending z of bottom
+ float m_pathLadderTimestamp; // time when we started using ladder - for timeout check
+
+ CountdownTimer m_mustRunTimer; // if nonzero, bot cannot walk
+
+ // game scenario mechanisms
+ CSGameState m_gameState;
+
+ // hostages mechanism
+ byte m_hostageEscortCount;
+ float m_hostageEscortCountTimestamp;
+ bool m_isWaitingForHostage;
+ CountdownTimer m_inhibitWaitingForHostageTimer;
+ CountdownTimer m_waitForHostageTimer;
+
+ // listening mechanism
+ Vector m_noisePosition; // position we last heard non-friendly noise
+ float m_noiseTimestamp; // when we heard it (can get zeroed)
+ CNavArea *m_noiseArea; // the nav area containing the noise
+ float m_noiseCheckTimestamp;
+ PriorityType m_noisePriority; // priority of currently heard noise
+ bool m_isNoiseTravelRangeChecked;
+
+ // "looking around" mechanism
+ float m_lookAroundStateTimestamp; // time of next state change
+ float m_lookAheadAngle; // our desired forward look angle
+ float m_forwardAngle; // our current forward facing direction
+ float m_inhibitLookAroundTimestamp; // time when we can look around again
+
+ enum LookAtSpotState
+ {
+ NOT_LOOKING_AT_SPOT, // not currently looking at a point in space
+ LOOK_TOWARDS_SPOT, // in the process of aiming at m_lookAtSpot
+ LOOK_AT_SPOT, // looking at m_lookAtSpot
+ NUM_LOOK_AT_SPOT_STATES
+ }
+ m_lookAtSpotState;
+ Vector m_lookAtSpot; // the spot we're currently looking at
+ PriorityType m_lookAtSpotPriority;
+ float m_lookAtSpotDuration; // how long we need to look at the spot
+ float m_lookAtSpotTimestamp; // when we actually began looking at the spot
+ float m_lookAtSpotAngleTolerance; // how exactly we must look at the spot
+ bool m_lookAtSpotClearIfClose; // if true, the look at spot is cleared if it gets close to us
+ const char *m_lookAtDesc; // for debugging
+ float m_peripheralTimestamp;
+
+ enum { MAX_APPROACH_POINTS = 16 };
+ Vector m_approachPoint[MAX_APPROACH_POINTS];
+ unsigned char m_approachPointCount;
+ Vector m_approachPointViewPosition; // the position used when computing current approachPoint set
+ bool m_isWaitingToTossGrenade; // lining up throw
+ CountdownTimer m_tossGrenadeTimer; // timeout timer for grenade tossing
+
+ SpotEncounter *m_spotEncounter; // the spots we will encounter as we move thru our current area
+ float m_spotCheckTimestamp; // when to check next encounter spot
+
+ // TODO: Add timestamp for each possible client to hiding spots
+ enum { MAX_CHECKED_SPOTS = 64 };
+ struct HidingSpotCheckInfo
+ {
+ HidingSpot *spot;
+ float timestamp;
+ }
+ m_checkedHidingSpot[MAX_CHECKED_SPOTS];
+ int m_checkedHidingSpotCount;
+
+ // view angle mechanism
+ float m_lookPitch; // our desired look pitch angle
+ float m_lookPitchVel;
+ float m_lookYaw; // our desired look yaw angle
+ float m_lookYawVel;
+
+ // aim angle mechanism
+ mutable Vector m_eyePos;
+ Vector m_aimOffset; // current error added to victim's position to get actual aim spot
+ Vector m_aimOffsetGoal; // desired aim offset
+ float m_aimOffsetTimestamp; // time of next offset adjustment
+ float m_aimSpreadTimestamp; // time used to determine max spread as it begins to tighten up
+ Vector m_aimSpot; // the spot we are currently aiming to fire at
+
+ // attack state data
+ // behavior modifiers
+ enum DispositionType
+ {
+ ENGAGE_AND_INVESTIGATE, // engage enemies on sight and investigate enemy noises
+ OPPORTUNITY_FIRE, // engage enemies on sight, but only look towards enemy noises, dont investigate
+ SELF_DEFENSE, // only engage if fired on, or very close to enemy
+ IGNORE_ENEMIES, // ignore all enemies - useful for ducking around corners, running away, etc
+
+ NUM_DISPOSITIONS
+ };
+ DispositionType m_disposition; // how we will react to enemies
+ CountdownTimer m_ignoreEnemiesTimer; // how long will we ignore enemies
+ mutable EntityHandle m_enemy; // our current enemy
+ bool m_isEnemyVisible; // result of last visibility test on enemy
+ unsigned char m_visibleEnemyParts; // which parts of the visible enemy do we see
+ Vector m_lastEnemyPosition; // last place we saw the enemy
+ float m_lastSawEnemyTimestamp;
+ float m_firstSawEnemyTimestamp;
+ float m_currentEnemyAcquireTimestamp;
+ float m_enemyDeathTimestamp; // if m_enemy is dead, this is when he died
+ bool m_isLastEnemyDead; // true if we killed or saw our last enemy die
+ int m_nearbyEnemyCount; // max number of enemies we've seen recently
+ unsigned int m_enemyPlace; // the location where we saw most of our enemies
+
+ struct WatchInfo
+ {
+ float timestamp;
+ bool isEnemy;
+ }
+ m_watchInfo[MAX_CLIENTS];
+ mutable EntityHandle m_bomber; // points to bomber if we can see him
+
+ int m_nearbyFriendCount; // number of nearby teammates
+ mutable EntityHandle m_closestVisibleFriend; // the closest friend we can see
+ mutable EntityHandle m_closestVisibleHumanFriend; // the closest human friend we can see
+
+ CBasePlayer *m_attacker; // last enemy that hurt us (may not be same as m_enemy)
+ float m_attackedTimestamp; // when we were hurt by the m_attacker
+
+ int m_lastVictimID; // the entindex of the last victim we killed, or zero
+ bool m_isAimingAtEnemy; // if true, we are trying to aim at our enemy
+ bool m_isRapidFiring; // if true, RunUpkeep() will toggle our primary attack as fast as it can
+ IntervalTimer m_equipTimer; // how long have we had our current weapon equipped
+ float m_fireWeaponTimestamp;
+
+ // reaction time system
+ enum { MAX_ENEMY_QUEUE = 20 };
+ struct ReactionState
+ {
+ // NOTE: player position & orientation is not currently stored separately
+ EntityHandle player;
+ bool isReloading;
+ bool isProtectedByShield;
+ }
+ m_enemyQueue[MAX_ENEMY_QUEUE]; // round-robin queue for simulating reaction times
+
+ byte m_enemyQueueIndex;
+ byte m_enemyQueueCount;
+ byte m_enemyQueueAttendIndex; // index of the timeframe we are "conscious" of
+
+ // stuck detection
+ bool m_isStuck;
+ float m_stuckTimestamp; // time when we got stuck
+ Vector m_stuckSpot; // the location where we became stuck
+ NavRelativeDirType m_wiggleDirection;
+ float m_wiggleTimestamp;
+ float m_stuckJumpTimestamp; // time for next jump when stuck
+
+ enum { MAX_VEL_SAMPLES = 5 };
+ float m_avgVel[MAX_VEL_SAMPLES];
+ int m_avgVelIndex;
+ int m_avgVelCount;
+ Vector m_lastOrigin;
+
+ // chatter mechanism
+ GameEventType m_lastRadioCommand; // last radio command we recieved
+
+ float m_lastRadioRecievedTimestamp; // time we recieved a radio message
+ float m_lastRadioSentTimestamp; // time when we send a radio message
+ EntityHandle m_radioSubject; // who issued the radio message
+ Vector m_radioPosition; // position referred to in radio message
+ float m_voiceFeedbackStartTimestamp;
+ float m_voiceFeedbackEndTimestamp; // new-style "voice" chatter gets voice feedback
+ BotChatterInterface m_chatter;
+
+ // learn map mechanism
+ const CNavNode *m_navNodeList;
+ CNavNode *m_currentNode;
+ NavDirType m_generationDir;
+ NavAreaList::iterator m_analyzeIter;
+
+ enum ProcessType
+ {
+ PROCESS_NORMAL,
+ PROCESS_LEARN,
+ PROCESS_ANALYZE_ALPHA,
+ PROCESS_ANALYZE_BETA,
+ PROCESS_SAVE,
+ }
+ m_processMode;
+ CountdownTimer m_mumbleTimer;
+ CountdownTimer m_booTimer;
+ CountdownTimer m_relocateTimer;
+};
diff --git a/dep/hlsdk/dlls/bot/cs_bot_chatter.h b/dep/hlsdk/dlls/bot/cs_bot_chatter.h
new file mode 100644
index 0000000..2e62a33
--- /dev/null
+++ b/dep/hlsdk/dlls/bot/cs_bot_chatter.h
@@ -0,0 +1,339 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+
+#define UNDEFINED_COUNT 0xFFFF
+#define MAX_PLACES_PER_MAP 64
+#define UNDEFINED_SUBJECT (-1)
+#define COUNT_MANY 4 // equal to or greater than this is "many"
+
+class CCSBot;
+class BotChatterInterface;
+
+typedef unsigned int PlaceCriteria;
+typedef unsigned int CountCriteria;
+
+// A meme is a unit information that bots use to
+// transmit information to each other via the radio
+class BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const = 0; // cause the given bot to act on this meme
+};
+
+class BotAllHostagesGoneMeme: public BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const; // cause the given bot to act on this meme
+};
+
+class BotHostageBeingTakenMeme: public BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const; // cause the given bot to act on this meme
+};
+
+class BotHelpMeme: public BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const; // cause the given bot to act on this meme
+
+public:
+ Place m_place;
+};
+
+class BotBombsiteStatusMeme: public BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const; // cause the given bot to act on this meme
+
+public:
+ enum StatusType { CLEAR, PLANTED };
+ int m_zoneIndex; // the bombsite
+ StatusType m_status; // whether it is cleared or the bomb is there (planted)
+};
+
+class BotBombStatusMeme: public BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const; // cause the given bot to act on this meme
+
+public:
+ CSGameState::BombState m_state;
+ Vector m_pos;
+};
+
+class BotFollowMeme: public BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const; // cause the given bot to act on this meme
+};
+
+class BotDefendHereMeme: public BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const; // cause the given bot to act on this meme
+
+public:
+ Vector m_pos;
+};
+
+class BotWhereBombMeme: public BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const; // cause the given bot to act on this meme
+};
+
+class BotRequestReportMeme: public BotMeme {
+public:
+ virtual void Interpret(CCSBot *sender, CCSBot *receiver) const; // cause the given bot to act on this meme
+};
+
+enum BotStatementType
+{
+ REPORT_VISIBLE_ENEMIES,
+ REPORT_ENEMY_ACTION,
+ REPORT_MY_CURRENT_TASK,
+ REPORT_MY_INTENTION,
+ REPORT_CRITICAL_EVENT,
+ REPORT_REQUEST_HELP,
+ REPORT_REQUEST_INFORMATION,
+ REPORT_ROUND_END,
+ REPORT_MY_PLAN,
+ REPORT_INFORMATION,
+ REPORT_EMOTE,
+ REPORT_ACKNOWLEDGE, // affirmative or negative
+ REPORT_ENEMIES_REMAINING,
+ REPORT_FRIENDLY_FIRE,
+ REPORT_KILLED_FRIEND,
+ //REPORT_ENEMY_LOST
+
+ NUM_BOT_STATEMENT_TYPES,
+};
+
+// BotSpeakables are the smallest unit of bot chatter.
+// They represent a specific wav file of a phrase, and the criteria for which it is useful
+class BotSpeakable {
+public:
+ char *m_phrase;
+ float m_duration;
+ PlaceCriteria m_place;
+ CountCriteria m_count;
+};
+
+typedef std::vector BotSpeakableVector;
+typedef std::vector BotVoiceBankVector;
+
+// The BotPhrase class is a collection of Speakables associated with a name, ID, and criteria
+class BotPhrase {
+public:
+ const char *GetName() const { return m_name; }
+ Place GetID() const { return m_id; }
+ GameEventType GetRadioEquivalent() const { return m_radioEvent; }
+ bool IsImportant() const { return m_isImportant; } // return true if this phrase is part of an important statement
+ bool IsPlace() const { return m_isPlace; }
+
+public:
+ friend class BotPhraseManager;
+ char *m_name;
+ Place m_id;
+ bool m_isPlace; // true if this is a Place phrase
+ GameEventType m_radioEvent;
+ bool m_isImportant; // mission-critical statement
+
+ mutable BotVoiceBankVector m_voiceBank; // array of voice banks (arrays of speakables)
+ std::vector m_count; // number of speakables
+ mutable std::vector< int > m_index; // index of next speakable to return
+ int m_numVoiceBanks; // number of voice banks that have been initialized
+
+ mutable PlaceCriteria m_placeCriteria;
+ mutable CountCriteria m_countCriteria;
+};
+
+typedef std::list BotPhraseList;
+
+// The BotPhraseManager is a singleton that provides an interface to all BotPhrase collections
+class BotPhraseManager {
+public:
+ const BotPhraseList *GetPlaceList() const { return &m_placeList; }
+
+ // return time last statement of given type was emitted by a teammate for the given place
+ float GetPlaceStatementInterval(Place place) const;
+
+ // set time of last statement of given type was emitted by a teammate for the given place
+ void ResetPlaceStatementInterval(Place place) const;
+
+public:
+ int FindPlaceIndex(Place where) const;
+
+ // master list of all phrase collections
+ BotPhraseList m_list;
+
+ // master list of all Place phrases
+ BotPhraseList m_placeList;
+
+ struct PlaceTimeInfo
+ {
+ Place placeID;
+ IntervalTimer timer;
+ };
+
+ mutable PlaceTimeInfo m_placeStatementHistory[MAX_PLACES_PER_MAP];
+ mutable int m_placeCount;
+};
+
+inline int BotPhraseManager::FindPlaceIndex(Place where) const
+{
+ for (int i = 0; i < m_placeCount; ++i)
+ {
+ if (m_placeStatementHistory[i].placeID == where)
+ return i;
+ }
+
+ if (m_placeCount < MAX_PLACES_PER_MAP)
+ {
+ m_placeStatementHistory[++m_placeCount].placeID = where;
+ m_placeStatementHistory[++m_placeCount].timer.Invalidate();
+ return m_placeCount - 1;
+ }
+
+ return -1;
+}
+
+inline float BotPhraseManager::GetPlaceStatementInterval(Place place) const
+{
+ int index = FindPlaceIndex(place);
+
+ if (index < 0)
+ return 999999.9f;
+
+ if (index >= m_placeCount)
+ return 999999.9f;
+
+ return m_placeStatementHistory[index].timer.GetElapsedTime();
+}
+
+inline void BotPhraseManager::ResetPlaceStatementInterval(Place place) const
+{
+ int index = FindPlaceIndex(place);
+
+ if (index < 0)
+ return;
+
+ if (index >= m_placeCount)
+ return;
+
+ m_placeStatementHistory[index].timer.Reset();
+}
+
+// Statements are meaningful collections of phrases
+class BotStatement {
+public:
+ BotChatterInterface *GetChatter() const { return m_chatter; }
+ BotStatementType GetType() const { return m_type; } // return the type of statement this is
+ bool HasSubject() const { return (m_subject != UNDEFINED_SUBJECT); }
+ void SetSubject(int playerID) { m_subject = playerID; } // who this statement is about
+ int GetSubject() const { return m_subject; } // who this statement is about
+ void SetPlace(Place where) { m_place = where; } // explicitly set place
+
+ void SetStartTime(float timestamp) { m_startTime = timestamp; } // define the earliest time this statement can be spoken
+ float GetStartTime() const { return m_startTime; }
+ bool IsSpeaking() const { return m_isSpeaking; } // return true if this statement is currently being spoken
+ float GetTimestamp() const { return m_timestamp; } // get time statement was created (but not necessarily started talking)
+
+public:
+ friend class BotChatterInterface;
+
+ BotChatterInterface *m_chatter; // the chatter system this statement is part of
+ BotStatement *m_next, *m_prev; // linked list hooks
+ BotStatementType m_type; // what kind of statement this is
+ int m_subject; // who this subject is about
+ Place m_place; // explicit place - note some phrases have implicit places as well
+ BotMeme *m_meme; // a statement can only have a single meme for now
+
+ float m_timestamp; // time when message was created
+ float m_startTime; // the earliest time this statement can be spoken
+ float m_expireTime; // time when this statement is no longer valid
+ float m_speakTimestamp; // time when message began being spoken
+ bool m_isSpeaking; // true if this statement is current being spoken
+
+ float m_nextTime; // time for next phrase to begin
+
+ enum { MAX_BOT_PHRASES = 4 };
+ enum ContextType
+ {
+ CURRENT_ENEMY_COUNT,
+ REMAINING_ENEMY_COUNT,
+ SHORT_DELAY,
+ LONG_DELAY,
+ ACCUMULATE_ENEMIES_DELAY,
+ };
+ struct
+ {
+ bool isPhrase;
+ union
+ {
+ const BotPhrase *phrase;
+ ContextType context;
+ };
+
+ }
+ m_statement[MAX_BOT_PHRASES];
+
+ enum { MAX_BOT_CONDITIONS = 4 };
+ enum ConditionType
+ {
+ IS_IN_COMBAT,
+ RADIO_SILENCE,
+ ENEMIES_REMAINING,
+ NUM_CONDITIONS,
+ };
+
+ ConditionType m_condition[MAX_BOT_CONDITIONS]; // conditions that must be true for the statement to be said
+ int m_conditionCount;
+
+ int m_index; // m_index refers to the phrase currently being spoken, or -1 if we havent started yet
+ int m_count;
+};
+
+// This class defines the interface to the bot radio chatter system
+class BotChatterInterface {
+public:
+ CCSBot *GetOwner() const { return m_me; }
+ int GetPitch() const { return m_pitch; }
+ bool SeesAtLeastOneEnemy() const { return m_seeAtLeastOneEnemy; }
+
+public:
+ BotStatement *m_statementList; // list of all active/pending messages for this bot
+ CCSBot *m_me; // the bot this chatter is for
+ bool m_seeAtLeastOneEnemy;
+ float m_timeWhenSawFirstEnemy;
+ bool m_reportedEnemies;
+ bool m_requestedBombLocation; // true if we already asked where the bomb has been planted
+ int m_pitch;
+ IntervalTimer m_needBackupInterval;
+ IntervalTimer m_spottedBomberInterval;
+ IntervalTimer m_scaredInterval;
+ IntervalTimer m_planInterval;
+ CountdownTimer m_spottedLooseBombTimer;
+ CountdownTimer m_heardNoiseTimer;
+ CountdownTimer m_escortingHostageTimer;
+};
+
+extern BotPhraseManager *TheBotPhrases;
diff --git a/dep/hlsdk/dlls/bot/cs_bot_manager.h b/dep/hlsdk/dlls/bot/cs_bot_manager.h
new file mode 100644
index 0000000..38f3d60
--- /dev/null
+++ b/dep/hlsdk/dlls/bot/cs_bot_manager.h
@@ -0,0 +1,145 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+
+extern CBotManager *TheBots;
+
+// The manager for Counter-Strike specific bots
+class CCSBotManager: public CBotManager {
+public:
+ virtual void ClientDisconnect(CBasePlayer *pPlayer) = 0;
+ virtual BOOL ClientCommand(CBasePlayer *pPlayer, const char *pcmd) = 0;
+
+ virtual void ServerActivate() = 0;
+ virtual void ServerDeactivate() = 0;
+
+ virtual void ServerCommand(const char *pcmd) = 0;
+ virtual void AddServerCommand(const char *cmd) = 0;
+ virtual void AddServerCommands() = 0;
+
+ virtual void RestartRound() = 0; // (EXTEND) invoked when a new round begins
+ virtual void StartFrame() = 0; // (EXTEND) called each frame
+
+ virtual void OnEvent(GameEventType event, CBaseEntity *entity = NULL, CBaseEntity *other = NULL) = 0;
+ virtual unsigned int GetPlayerPriority(CBasePlayer *player) const = 0; // return priority of player (0 = max pri)
+ virtual bool IsImportantPlayer(CBasePlayer *player) const = 0; // return true if player is important to scenario (VIP, bomb carrier, etc)
+
+public:
+ // the supported game scenarios
+ enum GameScenarioType
+ {
+ SCENARIO_DEATHMATCH,
+ SCENARIO_DEFUSE_BOMB,
+ SCENARIO_RESCUE_HOSTAGES,
+ SCENARIO_ESCORT_VIP
+ };
+ GameScenarioType GetScenario() const { return m_gameScenario; }
+
+ // "zones"
+ // depending on the game mode, these are bomb zones, rescue zones, etc.
+ enum { MAX_ZONES = 4 }; // max # of zones in a map
+ enum { MAX_ZONE_NAV_AREAS = 16 }; // max # of nav areas in a zone
+ struct Zone
+ {
+ CBaseEntity *m_entity; // the map entity
+ CNavArea *m_area[MAX_ZONE_NAV_AREAS]; // nav areas that overlap this zone
+ int m_areaCount;
+ Vector m_center;
+ bool m_isLegacy; // if true, use pev->origin and 256 unit radius as zone
+ int m_index;
+ Extent m_extent;
+ };
+
+ const Zone *GetZone(int i) const { return &m_zone[i]; }
+ int GetZoneCount() const { return m_zoneCount; }
+
+ // pick a zone at random and return it
+ const Zone *GetRandomZone() const
+ {
+ if (!m_zoneCount)
+ return NULL;
+
+ return &m_zone[RANDOM_LONG(0, m_zoneCount - 1)];
+ }
+
+ bool IsBombPlanted() const { return m_isBombPlanted; } // returns true if bomb has been planted
+ float GetBombPlantTimestamp() const { return m_bombPlantTimestamp; } // return time bomb was planted
+ bool IsTimeToPlantBomb() const { return (gpGlobals->time >= m_earliestBombPlantTimestamp); } // return true if it's ok to try to plant bomb
+ CBasePlayer *GetBombDefuser() const { return m_bombDefuser; } // return the player currently defusing the bomb, or NULL
+ CBaseEntity *GetLooseBomb() { return m_looseBomb; } // return the bomb if it is loose on the ground
+ CNavArea *GetLooseBombArea() const { return m_looseBombArea; } // return area that bomb is in/near
+
+ float GetLastSeenEnemyTimestamp() const { return m_lastSeenEnemyTimestamp; } // return the last time anyone has seen an enemy
+ void SetLastSeenEnemyTimestamp() { m_lastSeenEnemyTimestamp = gpGlobals->time; }
+
+ float GetRoundStartTime() const { return m_roundStartTimestamp; }
+ float GetElapsedRoundTime() const { return gpGlobals->time - m_roundStartTimestamp; } // return the elapsed time since the current round began
+
+ bool IsDefenseRushing() const { return m_isDefenseRushing; } // returns true if defense team has "decided" to rush this round
+ bool IsRoundOver() const { return m_isRoundOver; } // return true if the round has ended
+
+ unsigned int GetNavPlace() const { return m_navPlace; }
+ void SetNavPlace(unsigned int place) { m_navPlace = place; }
+
+public:
+ GameScenarioType m_gameScenario; // what kind of game are we playing
+
+ Zone m_zone[MAX_ZONES];
+ int m_zoneCount;
+
+ bool m_isBombPlanted; // true if bomb has been planted
+ float m_bombPlantTimestamp; // time bomb was planted
+ float m_earliestBombPlantTimestamp; // don't allow planting until after this time has elapsed
+ CBasePlayer *m_bombDefuser; // the player currently defusing a bomb
+ EHANDLE m_looseBomb; // will be non-NULL if bomb is loose on the ground
+ CNavArea *m_looseBombArea; // area that bomb is is/near
+
+ bool m_isRoundOver; // true if the round has ended
+ float m_radioMsgTimestamp[24][2];
+
+ float m_lastSeenEnemyTimestamp;
+ float m_roundStartTimestamp; // the time when the current round began
+
+ bool m_isDefenseRushing; // whether defensive team is rushing this round or not
+
+ unsigned int m_navPlace;
+ CountdownTimer m_respawnTimer;
+ bool m_isRespawnStarted;
+ bool m_canRespawn;
+ bool m_bServerActive;
+};
+
+inline int OtherTeam(int team)
+{
+ return (team == TERRORIST) ? CT : TERRORIST;
+}
+
+inline CCSBotManager *TheCSBots()
+{
+ return reinterpret_cast(TheBots);
+}
diff --git a/dep/hlsdk/dlls/bot/cs_gamestate.h b/dep/hlsdk/dlls/bot/cs_gamestate.h
new file mode 100644
index 0000000..769575f
--- /dev/null
+++ b/dep/hlsdk/dlls/bot/cs_gamestate.h
@@ -0,0 +1,90 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+
+class CCSBot;
+
+// This class represents the game state as known by a particular bot
+class CSGameState {
+public:
+ // bomb defuse scenario
+ enum BombState
+ {
+ MOVING, // being carried by a Terrorist
+ LOOSE, // loose on the ground somewhere
+ PLANTED, // planted and ticking
+ DEFUSED, // the bomb has been defused
+ EXPLODED, // the bomb has exploded
+ };
+
+ bool IsBombMoving() const { return (m_bombState == MOVING); }
+ bool IsBombLoose() const { return (m_bombState == LOOSE); }
+ bool IsBombPlanted() const { return (m_bombState == PLANTED); }
+ bool IsBombDefused() const { return (m_bombState == DEFUSED); }
+ bool IsBombExploded() const { return (m_bombState == EXPLODED); }
+
+public:
+ CCSBot *m_owner; // who owns this gamestate
+ bool m_isRoundOver; // true if round is over, but no yet reset
+
+ // bomb defuse scenario
+ BombState GetBombState() { return m_bombState; }
+ BombState m_bombState; // what we think the bomb is doing
+
+ IntervalTimer m_lastSawBomber;
+ Vector m_bomberPos;
+
+ IntervalTimer m_lastSawLooseBomb;
+ Vector m_looseBombPos;
+
+ bool m_isBombsiteClear[4]; // corresponds to zone indices in CCSBotManager
+ int m_bombsiteSearchOrder[4]; // randomized order of bombsites to search
+ int m_bombsiteCount;
+ int m_bombsiteSearchIndex; // the next step in the search
+
+ int m_plantedBombsite; // zone index of the bombsite where the planted bomb is
+
+ bool m_isPlantedBombPosKnown; // if true, we know the exact location of the bomb
+ Vector m_plantedBombPos;
+
+ // hostage rescue scenario
+ struct HostageInfo
+ {
+ CHostage *hostage;
+ Vector knownPos;
+ bool isValid;
+ bool isAlive;
+ bool isFree; // not being escorted by a CT
+ }
+ m_hostage[MAX_HOSTAGES];
+ int m_hostageCount; // number of hostages left in map
+ CountdownTimer m_validateInterval;
+
+ bool m_allHostagesRescued; // if true, so every hostages been is rescued
+ bool m_haveSomeHostagesBeenTaken; // true if a hostage has been moved by a CT (and we've seen it)
+};
diff --git a/dep/hlsdk/dlls/buttons.h b/dep/hlsdk/dlls/buttons.h
new file mode 100644
index 0000000..65a72b8
--- /dev/null
+++ b/dep/hlsdk/dlls/buttons.h
@@ -0,0 +1,105 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#define SF_GLOBAL_SET BIT(0) // Set global state to initial state on spawn
+
+class CEnvGlobal: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ string_t m_globalstate;
+ int m_triggermode;
+ int m_initialstate;
+};
+
+#define SF_ROTBUTTON_NOTSOLID BIT(0)
+#define SF_ROTBUTTON_BACKWARDS BIT(1)
+
+class CRotButton: public CBaseButton {
+public:
+ virtual void Spawn() = 0;
+ virtual void Restart() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+public:
+ Vector m_vecSpawn;
+};
+
+// Make this button behave like a door (HACKHACK)
+// This will disable use and make the button solid
+// rotating buttons were made SOLID_NOT by default since their were some
+// collision problems with them...
+#define SF_MOMENTARY_DOOR BIT(0)
+
+class CMomentaryRotButton: public CBaseToggle {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ int m_lastUsed;
+ int m_direction;
+ float m_returnSpeed;
+ Vector m_start;
+ Vector m_end;
+ int m_sounds;
+};
+
+#define SF_SPARK_TOOGLE BIT(5)
+#define SF_SPARK_IF_OFF BIT(6)
+
+class CEnvSpark: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+public:
+ float m_flDelay;
+};
+
+#define SF_BTARGET_USE BIT(0)
+#define SF_BTARGET_ON BIT(1)
+
+class CButtonTarget: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual int ObjectCaps() = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+};
diff --git a/dep/hlsdk/dlls/cbase.h b/dep/hlsdk/dlls/cbase.h
new file mode 100644
index 0000000..a156a34
--- /dev/null
+++ b/dep/hlsdk/dlls/cbase.h
@@ -0,0 +1,485 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "util.h"
+#include "schedule.h"
+#include "saverestore.h"
+#include "scriptevent.h"
+#include "monsterevent.h"
+
+class CSave;
+class CRestore;
+class CBasePlayer;
+class CBaseEntity;
+class CBaseMonster;
+class CBasePlayerItem;
+class CSquadMonster;
+class CCSEntity;
+
+class CBaseEntity {
+public:
+ // Constructor. Set engine to use C/C++ callback functions
+ // pointers to engine data
+ entvars_t *pev; // Don't need to save/restore this pointer, the engine resets it
+
+ // path corners
+ CBaseEntity *m_pGoalEnt; // path corner we are heading towards
+ CBaseEntity *m_pLink; // used for temporary link-list operations.
+
+ // initialization functions
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Restart() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Activate() = 0;
+
+ // Setup the object->object collision box (pev->mins / pev->maxs is the object->world collision box)
+ virtual void SetObjectCollisionBox() = 0;
+
+ // Classify - returns the type of group (i.e, "houndeye", or "human military" so that monsters with different classnames
+ // still realize that they are teammates. (overridden for monsters that form groups)
+ virtual int Classify() = 0;
+ virtual void DeathNotice(entvars_t *pevChild) = 0;
+
+ virtual void TraceAttack(entvars_t *pevAttacker, float flDamage, Vector vecDir, TraceResult *ptr, int bitsDamageType) = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+ virtual BOOL TakeHealth(float flHealth, int bitsDamageType) = 0;
+ virtual void Killed(entvars_t *pevAttacker, int iGib) = 0;
+ virtual int BloodColor() = 0;
+ virtual void TraceBleed(float flDamage, Vector vecDir, TraceResult *ptr, int bitsDamageType) = 0;
+ virtual BOOL IsTriggered(CBaseEntity *pActivator) = 0;
+ virtual CBaseMonster *MyMonsterPointer() = 0;
+ virtual CSquadMonster *MySquadMonsterPointer() = 0;
+ virtual int GetToggleState() = 0;
+ virtual void AddPoints(int score, BOOL bAllowNegativeScore) = 0;
+ virtual void AddPointsToTeam(int score, BOOL bAllowNegativeScore) = 0;
+ virtual BOOL AddPlayerItem(CBasePlayerItem *pItem) = 0;
+ virtual BOOL RemovePlayerItem(CBasePlayerItem *pItem) = 0;
+ virtual int GiveAmmo(int iAmount, const char *szName, int iMax = -1) = 0;
+ virtual float GetDelay() = 0;
+ virtual int IsMoving() = 0;
+ virtual void OverrideReset() = 0;
+ virtual int DamageDecal(int bitsDamageType) = 0;
+
+ // This is ONLY used by the node graph to test movement through a door
+ virtual void SetToggleState(int state) = 0;
+ virtual void StartSneaking() = 0;
+ virtual void UpdateOnRemove() = 0;
+ virtual BOOL OnControls(entvars_t *onpev) = 0;
+ virtual BOOL IsSneaking() = 0;
+ virtual BOOL IsAlive() = 0;
+ virtual BOOL IsBSPModel() = 0;
+ virtual BOOL ReflectGauss() = 0;
+ virtual BOOL HasTarget(string_t targetname) = 0;
+ virtual BOOL IsInWorld() = 0;
+ virtual BOOL IsPlayer() = 0;
+ virtual BOOL IsNetClient() = 0;
+ virtual const char *TeamID() = 0;
+
+ virtual CBaseEntity *GetNextTarget() = 0;
+
+ // fundamental callbacks
+ void (CBaseEntity::*m_pfnThink)();
+ void (CBaseEntity::*m_pfnTouch)(CBaseEntity *pOther);
+ void (CBaseEntity::*m_pfnUse)(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value);
+ void (CBaseEntity::*m_pfnBlocked)(CBaseEntity *pOther);
+
+ void EXT_FUNC DLLEXPORT SUB_Think();
+ void EXT_FUNC DLLEXPORT SUB_Touch(CBaseEntity *pOther);
+ void EXT_FUNC DLLEXPORT SUB_Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value);
+ void EXT_FUNC DLLEXPORT SUB_Blocked(CBaseEntity *pOther);
+
+ using thinkfn_t = decltype(m_pfnThink);
+ template
+ void SetThink(void (T::*pfn)());
+ void SetThink(std::nullptr_t);
+
+ using touchfn_t = decltype(m_pfnTouch);
+ template
+ void SetTouch(void (T::*pfn)(CBaseEntity *pOther));
+ void SetTouch(std::nullptr_t);
+
+ using usefn_t = decltype(m_pfnUse);
+ template
+ void SetUse(void (T::*pfn)(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value));
+ void SetUse(std::nullptr_t);
+
+ using blockedfn_t = decltype(m_pfnBlocked);
+ template
+ void SetBlocked(void (T::*pfn)(CBaseEntity *pOther));
+ void SetBlocked(std::nullptr_t);
+
+ virtual void Think() = 0;
+ virtual void Touch(CBaseEntity *pOther) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType = USE_OFF, float value = 0.0f) = 0;
+ virtual void Blocked(CBaseEntity *pOther) = 0;
+
+ virtual CBaseEntity *Respawn() = 0;
+ virtual void UpdateOwner() = 0;
+ virtual BOOL FBecomeProne() = 0;
+
+ virtual Vector Center() = 0; // center point of entity
+ virtual Vector EyePosition() = 0; // position of eyes
+ virtual Vector EarPosition() = 0; // position of ears
+ virtual Vector BodyTarget(const Vector &posSrc) = 0; // position to shoot at
+
+ virtual int Illumination() = 0;
+ virtual BOOL FVisible(CBaseEntity *pEntity) = 0;
+ virtual BOOL FVisible(const Vector &vecOrigin) = 0;
+public:
+ static CBaseEntity *Instance(edict_t *pent) { return (CBaseEntity *)GET_PRIVATE(pent ? pent : ENT(0)); }
+ static CBaseEntity *Instance(entvars_t *pev) { return Instance(ENT(pev)); }
+ static CBaseEntity *Instance(int offset) { return Instance(ENT(offset)); }
+
+ edict_t *edict();
+ EOFFSET eoffset();
+ int entindex();
+ int IsDormant();
+
+ bool Intersects(CBaseEntity *pOther);
+ bool Intersects(const Vector &mins, const Vector &maxs);
+
+ // Exports func's, useful method's for SetThink
+ void EXPORT SUB_CallUseToggle()
+ {
+ Use(this, this, USE_TOGGLE, 0);
+ }
+
+ void EXPORT SUB_Remove()
+ {
+ if (pev->health > 0)
+ {
+ // this situation can screw up monsters who can't tell their entity pointers are invalid.
+ pev->health = 0;
+ ALERT(at_aiconsole, "SUB_Remove called on entity with health > 0\n");
+ }
+
+ REMOVE_ENTITY(ENT(pev));
+ }
+
+public:
+ // NOTE: it was replaced on member "int *current_ammo" because it is useless.
+ CCSEntity *m_pEntity;
+
+ // We use this variables to store each ammo count.
+ float currentammo;
+ int maxammo_buckshot;
+ int ammo_buckshot;
+ int maxammo_9mm;
+ int ammo_9mm;
+ int maxammo_556nato;
+ int ammo_556nato;
+ int maxammo_556natobox;
+ int ammo_556natobox;
+ int maxammo_762nato;
+ int ammo_762nato;
+ int maxammo_45acp;
+ int ammo_45acp;
+ int maxammo_50ae;
+ int ammo_50ae;
+ int maxammo_338mag;
+ int ammo_338mag;
+ int maxammo_57mm;
+ int ammo_57mm;
+ int maxammo_357sig;
+ int ammo_357sig;
+
+ // Special stuff for grenades and knife.
+ float m_flStartThrow;
+ float m_flReleaseThrow;
+ int m_iSwing;
+
+ // client has left the game
+ bool has_disconnected;
+};
+
+#include "ehandle.h"
+
+// Inlines
+inline BOOL FNullEnt(CBaseEntity *ent) { return (ent == NULL || FNullEnt(ent->edict())); }
+
+template
+inline void CBaseEntity::SetThink(void (T::*pfn)())
+{
+ m_pfnThink = static_cast(pfn);
+}
+
+inline void CBaseEntity::SetThink(std::nullptr_t)
+{
+ m_pfnThink = nullptr;
+}
+
+template
+inline void CBaseEntity::SetTouch(void (T::*pfn)(CBaseEntity *pOther))
+{
+ m_pfnTouch = static_cast(pfn);
+}
+
+inline void CBaseEntity::SetTouch(std::nullptr_t)
+{
+ m_pfnTouch = nullptr;
+}
+
+template
+inline void CBaseEntity::SetUse(void (T::*pfn)(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value))
+{
+ m_pfnUse = static_cast(pfn);
+}
+
+inline void CBaseEntity::SetUse(std::nullptr_t)
+{
+ m_pfnUse = nullptr;
+}
+
+template
+inline void CBaseEntity::SetBlocked(void (T::*pfn)(CBaseEntity *pOther))
+{
+ m_pfnBlocked = static_cast(pfn);
+}
+
+inline void CBaseEntity::SetBlocked(std::nullptr_t)
+{
+ m_pfnBlocked = nullptr;
+}
+
+class CPointEntity: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual int ObjectCaps() = 0;
+};
+
+// generic Delay entity
+class CBaseDelay: public CBaseEntity {
+public:
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+public:
+ float m_flDelay;
+ string_t m_iszKillTarget;
+};
+
+class CBaseAnimating: public CBaseDelay {
+public:
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void HandleAnimEvent(MonsterEvent_t *pEvent) = 0;
+public:
+ // animation needs
+ float m_flFrameRate; // computed FPS for current sequence
+ float m_flGroundSpeed; // computed linear movement rate for current sequence
+ float m_flLastEventCheck; // last time the event list was checked
+ BOOL m_fSequenceFinished; // flag set when StudioAdvanceFrame moves across a frame boundry
+ BOOL m_fSequenceLoops; // true if the sequence loops
+};
+
+// generic Toggle entity.
+class CBaseToggle: public CBaseAnimating {
+public:
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int GetToggleState() = 0;
+ virtual float GetDelay() = 0;
+
+ void EXT_FUNC DLLEXPORT SUB_MoveDone();
+public:
+ TOGGLE_STATE m_toggle_state;
+ float m_flActivateFinished; // like attack_finished, but for doors
+ float m_flMoveDistance; // how far a door should slide or rotate
+ float m_flWait;
+ float m_flLip;
+ float m_flTWidth; // for plats
+ float m_flTLength; // for plats
+
+ Vector m_vecPosition1;
+ Vector m_vecPosition2;
+ Vector m_vecAngle1;
+ Vector m_vecAngle2;
+
+ int m_cTriggersLeft; // trigger_counter only, # of activations remaining
+ float m_flHeight;
+ EHANDLE m_hActivator;
+ void (CBaseToggle::*m_pfnCallWhenMoveDone)();
+
+ using movedonefn_t = decltype(m_pfnCallWhenMoveDone);
+ template
+ void SetMoveDone(void (T::*pfn)());
+ void SetMoveDone(std::nullptr_t);
+
+ Vector m_vecFinalDest;
+ Vector m_vecFinalAngle;
+
+ int m_bitsDamageInflict; // DMG_ damage type that the door or tigger does
+
+ string_t m_sMaster; // If this button has a master switch, this is the targetname.
+ // A master switch must be of the multisource type. If all
+ // of the switches in the multisource have been triggered, then
+ // the button will be allowed to operate. Otherwise, it will be
+ // deactivated.
+};
+
+template
+inline void CBaseToggle::SetMoveDone(void (T::*pfn)())
+{
+ m_pfnCallWhenMoveDone = static_cast(pfn);
+}
+
+inline void CBaseToggle::SetMoveDone(std::nullptr_t)
+{
+ m_pfnCallWhenMoveDone = nullptr;
+}
+
+#include "world.h"
+#include "basemonster.h"
+#include "player.h"
+
+#define SF_BUTTON_DONTMOVE BIT(0)
+#define SF_BUTTON_TOGGLE BIT(5) // button stays pushed until reactivated
+#define SF_BUTTON_SPARK_IF_OFF BIT(6) // button sparks in OFF state
+#define SF_BUTTON_TOUCH_ONLY BIT(8) // button only fires as a result of USE key.
+
+// Generic Button
+class CBaseButton: public CBaseToggle {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Restart() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0; // Buttons that don't take damage can be IMPULSE used
+public:
+ BOOL m_fStayPushed; // button stays pushed in until touched again?
+ BOOL m_fRotating; // a rotating button? default is a sliding button.
+
+ string_t m_strChangeTarget; // if this field is not null, this is an index into the engine string array.
+ // when this button is touched, it's target entity's TARGET field will be set
+ // to the button's ChangeTarget. This allows you to make a func_train switch paths, etc.
+
+ locksound_t m_ls; // door lock sounds
+
+ byte m_bLockedSound; // ordinals from entity selection
+ byte m_bLockedSentence;
+ byte m_bUnlockedSound;
+ byte m_bUnlockedSentence;
+ int m_sounds;
+};
+
+// MultiSouce
+#define MAX_MS_TARGETS 32 // maximum number of targets a single multisource entity may be assigned.
+#define SF_MULTI_INIT BIT(0)
+
+class CMultiSource: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Restart() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual BOOL IsTriggered(CBaseEntity *pActivator) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ EHANDLE m_rgEntities[MAX_MS_TARGETS];
+ int m_rgTriggered[MAX_MS_TARGETS];
+
+ int m_iTotal;
+ string_t m_globalstate;
+};
+
+// Converts a entvars_t * to a class pointer
+// It will allocate the class and entity if necessary
+template
+T *GetClassPtr(T *a)
+{
+ entvars_t *pev = (entvars_t *)a;
+
+ // allocate entity if necessary
+ if (pev == nullptr)
+ pev = VARS(CREATE_ENTITY());
+
+ // get the private data
+ a = (T *)GET_PRIVATE(ENT(pev));
+
+ if (a == nullptr)
+ {
+ // allocate private data
+ a = new(pev) T;
+ a->pev = pev;
+ }
+
+ return a;
+}
+
+// Inlines
+inline edict_t *CBaseEntity::edict()
+{
+ return ENT(pev);
+}
+
+inline EOFFSET CBaseEntity::eoffset()
+{
+ return OFFSET(pev);
+}
+
+inline int CBaseEntity::entindex()
+{
+ return ENTINDEX(edict());
+}
+
+inline int CBaseEntity::IsDormant()
+{
+ return (pev->flags & FL_DORMANT) == FL_DORMANT;
+}
+
+inline bool CBaseEntity::Intersects(CBaseEntity *pOther)
+{
+ return Intersects(pOther->pev->absmin, pOther->pev->absmax);
+}
+
+inline bool CBaseEntity::Intersects(const Vector &mins, const Vector &maxs)
+{
+ if (mins.x > pev->absmax.x
+ || mins.y > pev->absmax.y
+ || mins.z > pev->absmax.z
+ || maxs.x < pev->absmin.x
+ || maxs.y < pev->absmin.y
+ || maxs.z < pev->absmin.z)
+ {
+ return false;
+ }
+
+ return true;
+}
diff --git a/dep/hlsdk/dlls/cdll_dll.h b/dep/hlsdk/dlls/cdll_dll.h
new file mode 100644
index 0000000..91fc662
--- /dev/null
+++ b/dep/hlsdk/dlls/cdll_dll.h
@@ -0,0 +1,195 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+const int MAX_WEAPON_SLOTS = 5; // hud item selection slots
+const int MAX_ITEM_TYPES = 6; // hud item selection slots
+const int MAX_AMMO_SLOTS = 32; // not really slots
+const int MAX_ITEMS = 4; // hard coded item types
+
+const int DEFAULT_FOV = 90; // the default field of view
+
+#define HIDEHUD_WEAPONS BIT(0)
+#define HIDEHUD_FLASHLIGHT BIT(1)
+#define HIDEHUD_ALL BIT(2)
+#define HIDEHUD_HEALTH BIT(3)
+#define HIDEHUD_TIMER BIT(4)
+#define HIDEHUD_MONEY BIT(5)
+#define HIDEHUD_CROSSHAIR BIT(6)
+#define HIDEHUD_OBSERVER_CROSSHAIR BIT(7)
+
+#define STATUSICON_HIDE 0
+#define STATUSICON_SHOW 1
+#define STATUSICON_FLASH 2
+
+#define HUD_PRINTNOTIFY 1
+#define HUD_PRINTCONSOLE 2
+#define HUD_PRINTTALK 3
+#define HUD_PRINTCENTER 4
+#define HUD_PRINTRADIO 5
+
+#define TEAM_UNASSIGNED 0
+#define TEAM_TERRORIST 1
+#define TEAM_CT 2
+#define TEAM_SPECTATOR 3
+
+#define STATUS_NIGHTVISION_ON 1
+#define STATUS_NIGHTVISION_OFF 0
+
+#define ITEM_STATUS_NIGHTVISION BIT(0)
+#define ITEM_STATUS_DEFUSER BIT(1)
+
+#define SCORE_STATUS_DEAD BIT(0)
+#define SCORE_STATUS_BOMB BIT(1)
+#define SCORE_STATUS_VIP BIT(2)
+
+// player data iuser3
+#define PLAYER_CAN_SHOOT BIT(0)
+#define PLAYER_FREEZE_TIME_OVER BIT(1)
+#define PLAYER_IN_BOMB_ZONE BIT(2)
+#define PLAYER_HOLDING_SHIELD BIT(3)
+
+#define MENU_KEY_1 BIT(0)
+#define MENU_KEY_2 BIT(1)
+#define MENU_KEY_3 BIT(2)
+#define MENU_KEY_4 BIT(3)
+#define MENU_KEY_5 BIT(4)
+#define MENU_KEY_6 BIT(5)
+#define MENU_KEY_7 BIT(6)
+#define MENU_KEY_8 BIT(7)
+#define MENU_KEY_9 BIT(8)
+#define MENU_KEY_0 BIT(9)
+
+#define CS_NUM_SKIN 4
+#define CZ_NUM_SKIN 5
+
+#define FIELD_ORIGIN0 0
+#define FIELD_ORIGIN1 1
+#define FIELD_ORIGIN2 2
+
+#define FIELD_ANGLES0 3
+#define FIELD_ANGLES1 4
+#define FIELD_ANGLES2 5
+
+#define CUSTOMFIELD_ORIGIN0 0
+#define CUSTOMFIELD_ORIGIN1 1
+#define CUSTOMFIELD_ORIGIN2 2
+
+#define CUSTOMFIELD_ANGLES0 3
+#define CUSTOMFIELD_ANGLES1 4
+#define CUSTOMFIELD_ANGLES2 5
+
+#define CUSTOMFIELD_SKIN 6
+#define CUSTOMFIELD_SEQUENCE 7
+#define CUSTOMFIELD_ANIMTIME 8
+
+#define HUD_PRINTNOTIFY 1
+#define HUD_PRINTCONSOLE 2
+#define HUD_PRINTTALK 3
+#define HUD_PRINTCENTER 4
+
+#define WEAPON_SUIT 31
+#define WEAPON_ALLWEAPONS (~(1<skin = ENTINDEX(pEntity);
+ pev->body = attachment;
+ pev->aiment = pEntity;
+ pev->movetype = MOVETYPE_FOLLOW;
+ }
+ }
+
+ float Frames() const { return m_maxFrame; }
+ void SetTransparency(int rendermode, int r, int g, int b, int a, int fx)
+ {
+ pev->rendermode = rendermode;
+ pev->rendercolor.x = r;
+ pev->rendercolor.y = g;
+ pev->rendercolor.z = b;
+ pev->renderamt = a;
+ pev->renderfx = fx;
+ }
+
+ void SetTexture(int spriteIndex) { pev->modelindex = spriteIndex; }
+ void SetScale(float scale) { pev->scale = scale; }
+ void SetColor(int r, int g, int b) { pev->rendercolor.x = r; pev->rendercolor.y = g; pev->rendercolor.z = b; }
+ void SetBrightness(int brightness) { pev->renderamt = brightness; }
+ void AnimateAndDie(float framerate)
+ {
+ SetThink(&CSprite::AnimateUntilDead);
+ pev->framerate = framerate;
+ pev->dmgtime = gpGlobals->time + (m_maxFrame / framerate);
+ pev->nextthink = gpGlobals->time;
+ }
+private:
+ float m_lastTime;
+ float m_maxFrame;
+};
+
+#define SF_BEAM_STARTON BIT(0)
+#define SF_BEAM_TOGGLE BIT(1)
+#define SF_BEAM_RANDOM BIT(2)
+#define SF_BEAM_RING BIT(3)
+#define SF_BEAM_SPARKSTART BIT(4)
+#define SF_BEAM_SPARKEND BIT(5)
+#define SF_BEAM_DECALS BIT(6)
+#define SF_BEAM_SHADEIN BIT(7)
+#define SF_BEAM_SHADEOUT BIT(8)
+#define SF_BEAM_TEMPORARY BIT(15)
+
+class CBeam: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual int ObjectCaps() = 0;
+ virtual Vector Center() = 0;
+public:
+ void SetType(int type) { pev->rendermode = (pev->rendermode & 0xF0) | (type & 0x0F); }
+ void SetFlags(int flags) { pev->rendermode = (pev->rendermode & 0x0F) | (flags & 0xF0); }
+ void SetStartPos(const Vector &pos) { pev->origin = pos; }
+ void SetEndPos(const Vector &pos) { pev->angles = pos; }
+
+ void SetStartEntity(int entityIndex);
+ void SetEndEntity(int entityIndex);
+
+ void SetStartAttachment(int attachment) { pev->sequence = (pev->sequence & 0x0FFF) | ((attachment & 0xF) << 12); }
+ void SetEndAttachment(int attachment) { pev->skin = (pev->skin & 0x0FFF) | ((attachment & 0xF) << 12); }
+ void SetTexture(int spriteIndex) { pev->modelindex = spriteIndex; }
+ void SetWidth(int width) { pev->scale = width; }
+ void SetNoise(int amplitude) { pev->body = amplitude; }
+ void SetColor(int r, int g, int b) { pev->rendercolor.x = r; pev->rendercolor.y = g; pev->rendercolor.z = b; }
+ void SetBrightness(int brightness) { pev->renderamt = brightness; }
+ void SetFrame(float frame) { pev->frame = frame; }
+ void SetScrollRate(int speed) { pev->animtime = speed; }
+ int GetType() const { return pev->rendermode & 0x0F; }
+ int GetFlags() const { return pev->rendermode & 0xF0; }
+ int GetStartEntity() const { return pev->sequence & 0xFFF; }
+ int GetEndEntity() const { return pev->skin & 0xFFF; }
+
+ const Vector &GetStartPos();
+ const Vector &GetEndPos();
+
+ int GetTexture() const { return pev->modelindex; }
+ int GetWidth() const { return pev->scale; }
+ int GetNoise() const { return pev->body; }
+ int GetBrightness() const { return pev->renderamt; }
+ int GetFrame() const { return pev->frame; }
+ int GetScrollRate() const { return pev->animtime; }
+
+ void LiveForTime(float time)
+ {
+ SetThink(&CBeam::SUB_Remove);
+ pev->nextthink = gpGlobals->time + time;
+ }
+ void BeamDamageInstant(TraceResult *ptr, float damage)
+ {
+ pev->dmg = damage;
+ pev->dmgtime = gpGlobals->time - 1;
+ BeamDamage(ptr);
+ }
+};
+
+class CLaser: public CBeam {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ CSprite *m_pSprite;
+ int m_iszSpriteName;
+ Vector m_firePosition;
+};
+
+#define SF_BUBBLES_STARTOFF BIT(0)
+
+class CBubbling: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ int m_density;
+ int m_frequency;
+ int m_bubbleModel;
+ int m_state;
+};
+
+class CLightning: public CBeam {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Activate() = 0;
+public:
+ inline BOOL ServerSide() const
+ {
+ if (!m_life && !(pev->spawnflags & SF_BEAM_RING))
+ return TRUE;
+
+ return FALSE;
+ }
+public:
+ int m_active;
+ int m_iszStartEntity;
+ int m_iszEndEntity;
+ float m_life;
+ int m_boltWidth;
+ int m_noiseAmplitude;
+ int m_brightness;
+ int m_speed;
+ float m_restrike;
+ int m_spriteTexture;
+ int m_iszSpriteName;
+ int m_frameStart;
+ float m_radius;
+};
+
+class CGlow: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Think() = 0;
+public:
+ float m_lastTime;
+ float m_maxFrame;
+};
+
+class CBombGlow: public CSprite {
+public:
+ virtual void Spawn() = 0;
+ virtual void Think() = 0;
+public:
+ float m_lastTime;
+ float m_tmBeepPeriod;
+ bool m_bSetModel;
+};
+
+#define SF_GIBSHOOTER_REPEATABLE BIT(0) // Allows a gibshooter to be refired
+
+class CGibShooter: public CBaseDelay {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+ virtual CGib *CreateGib() = 0;
+public:
+ int m_iGibs;
+ int m_iGibCapacity;
+ int m_iGibMaterial;
+ int m_iGibModelIndex;
+
+ float m_flGibVelocity;
+ float m_flVariance;
+ float m_flGibLife;
+};
+
+class CEnvShooter: public CGibShooter {
+public:
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual CGib *CreateGib() = 0;
+};
+
+const int MAX_BEAM = 24;
+
+class CTestEffect: public CBaseDelay {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ int m_iLoop;
+ int m_iBeam;
+
+ CBeam *m_pBeam[MAX_BEAM];
+
+ float m_flBeamTime[MAX_BEAM];
+ float m_flStartTime;
+};
+
+#define SF_BLOOD_RANDOM BIT(0)
+#define SF_BLOOD_STREAM BIT(1)
+#define SF_BLOOD_PLAYER BIT(2)
+#define SF_BLOOD_DECAL BIT(3)
+
+class CBlood: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+
+public:
+ int Color() const { return pev->impulse; }
+ float BloodAmount() const { return pev->dmg; }
+
+ void SetColor(int color) { pev->impulse = color; }
+ void SetBloodAmount(float amount) { pev->dmg = amount; }
+};
+
+#define SF_SHAKE_EVERYONE BIT(0) // Don't check radius
+#define SF_SHAKE_DISRUPT BIT(1) // Disrupt controls
+#define SF_SHAKE_INAIR BIT(2) // Shake players in air
+
+class CShake: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ float Amplitude() const { return pev->scale; }
+ float Frequency() const { return pev->dmg_save; }
+ float Duration() const { return pev->dmg_take; }
+ float Radius() const { return pev->dmg; }
+
+ void SetAmplitude(float amplitude) { pev->scale = amplitude; }
+ void SetFrequency(float frequency) { pev->dmg_save = frequency; }
+ void SetDuration(float duration) { pev->dmg_take = duration; }
+ void SetRadius(float radius) { pev->dmg = radius; }
+};
+
+#define SF_FADE_IN BIT(0) // Fade in, not out
+#define SF_FADE_MODULATE BIT(1) // Modulate, don't blend
+#define SF_FADE_ONLYONE BIT(2)
+
+class CFade: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ float Duration() const { return pev->dmg_take; }
+ float HoldTime() const { return pev->dmg_save; }
+
+ void SetDuration(float duration) { pev->dmg_take = duration; }
+ void SetHoldTime(float hold) { pev->dmg_save = hold; }
+};
+
+#define SF_MESSAGE_ONCE BIT(0) // Fade in, not out
+#define SF_MESSAGE_ALL BIT(1) // Send to all clients
+
+class CMessage: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+};
+
+#define SF_FUNNEL_REVERSE BIT(0) // Funnel effect repels particles instead of attracting them
+
+class CEnvFunnel: public CBaseDelay {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ int m_iSprite;
+};
+
+class CEnvBeverage: public CBaseDelay {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+};
+
+class CItemSoda: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+};
+
+// Inlines
+inline void CBeam::SetStartEntity(int entityIndex)
+{
+ pev->sequence = (entityIndex & 0x0FFF) | ((pev->sequence & 0xF000) << 12);
+ pev->owner = INDEXENT(entityIndex);
+}
+
+inline void CBeam::SetEndEntity(int entityIndex)
+{
+ pev->skin = (entityIndex & 0x0FFF) | ((pev->skin & 0xF000) << 12);
+ pev->aiment = INDEXENT(entityIndex);
+}
+
+inline const Vector &CBeam::GetStartPos()
+{
+ if (GetType() == BEAM_ENTS)
+ {
+ edict_t *pent = INDEXENT(GetStartEntity());
+ return pent->v.origin;
+ }
+
+ return pev->origin;
+}
+
+inline const Vector &CBeam::GetEndPos()
+{
+ int type = GetType();
+ if (type == BEAM_POINTS || type == BEAM_HOSE)
+ {
+ return pev->angles;
+ }
+
+ edict_t *pent = INDEXENT(GetEndEntity());
+ if (pent)
+ {
+ return pent->v.origin;
+ }
+
+ return pev->angles;
+}
diff --git a/dep/hlsdk/dlls/ehandle.h b/dep/hlsdk/dlls/ehandle.h
new file mode 100644
index 0000000..66897fb
--- /dev/null
+++ b/dep/hlsdk/dlls/ehandle.h
@@ -0,0 +1,239 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+// Safe way to point to CBaseEntities who may die between frames.
+template
+class EntityHandle
+{
+public:
+ EntityHandle() : m_edict(nullptr), m_serialnumber(0) {}
+ EntityHandle(const EntityHandle &other);
+ EntityHandle(const T *pEntity);
+ EntityHandle(const edict_t *pEdict);
+
+ // cast to base class
+ // NOTE: this is a unsafe method
+ template
+ R *Get() const;
+
+ edict_t *Get() const;
+ edict_t *Set(edict_t *pEdict);
+
+ void Remove();
+ bool IsValid() const;
+ int GetSerialNumber() const;
+
+ bool operator==(T *pEntity) const;
+ operator bool() const;
+ operator T *() const;
+
+ T *operator=(T *pEntity);
+ T *operator->();
+
+ // Copy the ehandle.
+ EntityHandle& operator=(const EntityHandle &other);
+
+private:
+ edict_t *m_edict;
+ int m_serialnumber;
+};
+
+// Short alias
+using EHandle = EntityHandle<>;
+using EHANDLE = EHandle;
+
+// Inlines
+template
+inline bool FNullEnt(EntityHandle &hent)
+{
+ return (!hent || FNullEnt(OFFSET(hent.Get())));
+}
+
+// Copy constructor
+template
+EntityHandle::EntityHandle(const EntityHandle &other)
+{
+ m_edict = other.m_edict;
+ m_serialnumber = other.m_serialnumber;
+}
+
+template
+EntityHandle::EntityHandle(const T *pEntity)
+{
+ if (pEntity)
+ {
+ Set(ENT(pEntity->pev));
+ }
+ else
+ {
+ m_edict = nullptr;
+ m_serialnumber = 0;
+ }
+}
+
+template
+EntityHandle::EntityHandle(const edict_t *pEdict)
+{
+ Set(const_cast(pEdict));
+}
+
+template
+template
+inline R *EntityHandle::Get() const
+{
+ return GET_PRIVATE(Get());
+}
+
+template
+inline edict_t *EntityHandle::Get() const
+{
+ if (!m_edict || m_edict->serialnumber != m_serialnumber || m_edict->free)
+ {
+ return nullptr;
+ }
+
+ return m_edict;
+}
+
+template
+inline edict_t *EntityHandle::Set(edict_t *pEdict)
+{
+ m_edict = pEdict;
+ if (pEdict)
+ {
+ m_serialnumber = pEdict->serialnumber;
+ }
+
+ return pEdict;
+}
+
+template
+void EntityHandle::Remove()
+{
+ if (IsValid())
+ {
+ UTIL_Remove(*this);
+ }
+
+ m_edict = nullptr;
+ m_serialnumber = 0;
+}
+
+// Returns whether this handle is valid.
+template
+inline bool EntityHandle::IsValid() const
+{
+ edict_t *pEdict = Get();
+ if (!pEdict)
+ {
+ return false;
+ }
+
+ CBaseEntity *pEntity = GET_PRIVATE(pEdict);
+ if (!pEntity)
+ {
+ return false;
+ }
+
+ return true;
+}
+
+// CBaseEntity serial number.
+// Used to determine if the entity is still valid.
+template
+inline int EntityHandle::GetSerialNumber() const
+{
+ return m_serialnumber;
+}
+
+template
+inline bool EntityHandle::operator==(T *pEntity) const
+{
+ assert(("EntityHandle::operator==: got a nullptr pointer!", pEntity != nullptr));
+
+ if (m_serialnumber != pEntity->edict()->serialnumber)
+ {
+ return false;
+ }
+
+ return m_edict == pEntity->edict();
+}
+
+template
+inline EntityHandle::operator bool() const
+{
+ return IsValid();
+}
+
+// Gets the Entity this handle refers to.
+// Returns null if invalid.
+template
+inline EntityHandle::operator T *() const
+{
+ return GET_PRIVATE(Get());
+}
+
+// Assigns the given entity to this handle.
+template
+inline T *EntityHandle::operator=(T *pEntity)
+{
+ if (pEntity)
+ {
+ Set(ENT(pEntity->pev));
+ }
+ else
+ {
+ m_edict = nullptr;
+ m_serialnumber = 0;
+ }
+
+ return static_cast(pEntity);
+}
+
+template
+inline T *EntityHandle::operator->()
+{
+ edict_t *pEdict = Get();
+ assert(("EntityHandle::operator->: pointer is nullptr!", pEdict != nullptr));
+
+ T *pEntity = GET_PRIVATE(pEdict);
+ assert(("EntityHandle::operator->: pvPrivateData is nullptr!", pEntity != nullptr));
+ return pEntity;
+}
+
+// Makes this handle refer to the same entity as the given handle.
+template
+inline EntityHandle& EntityHandle::operator=(const EntityHandle &other)
+{
+ m_edict = other.m_edict;
+ m_serialnumber = other.m_serialnumber;
+
+ return (*this);
+}
diff --git a/dep/hlsdk/dlls/enginecallback.h b/dep/hlsdk/dlls/enginecallback.h
new file mode 100644
index 0000000..93150ac
--- /dev/null
+++ b/dep/hlsdk/dlls/enginecallback.h
@@ -0,0 +1,186 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "event_flags.h"
+
+// Must be provided by user of this code
+extern enginefuncs_t g_engfuncs;
+
+// The actual engine callbacks
+#define GETPLAYERUSERID (*g_engfuncs.pfnGetPlayerUserId)
+#define PRECACHE_MODEL (*g_engfuncs.pfnPrecacheModel)
+#define PRECACHE_SOUND (*g_engfuncs.pfnPrecacheSound)
+#define PRECACHE_GENERIC (*g_engfuncs.pfnPrecacheGeneric)
+#define SET_MODEL (*g_engfuncs.pfnSetModel)
+#define MODEL_INDEX (*g_engfuncs.pfnModelIndex)
+#define MODEL_FRAMES (*g_engfuncs.pfnModelFrames)
+#define SET_SIZE (*g_engfuncs.pfnSetSize)
+#define CHANGE_LEVEL (*g_engfuncs.pfnChangeLevel)
+#define GET_SPAWN_PARMS (*g_engfuncs.pfnGetSpawnParms)
+#define SAVE_SPAWN_PARMS (*g_engfuncs.pfnSaveSpawnParms)
+#define VEC_TO_YAW (*g_engfuncs.pfnVecToYaw)
+#define VEC_TO_ANGLES (*g_engfuncs.pfnVecToAngles)
+#define MOVE_TO_ORIGIN (*g_engfuncs.pfnMoveToOrigin)
+#define oldCHANGE_YAW (*g_engfuncs.pfnChangeYaw)
+#define CHANGE_PITCH (*g_engfuncs.pfnChangePitch)
+#define MAKE_VECTORS (*g_engfuncs.pfnMakeVectors)
+#define CREATE_ENTITY (*g_engfuncs.pfnCreateEntity)
+#define REMOVE_ENTITY (*g_engfuncs.pfnRemoveEntity)
+#define CREATE_NAMED_ENTITY (*g_engfuncs.pfnCreateNamedEntity)
+#define MAKE_STATIC (*g_engfuncs.pfnMakeStatic)
+#define ENT_IS_ON_FLOOR (*g_engfuncs.pfnEntIsOnFloor)
+#define DROP_TO_FLOOR (*g_engfuncs.pfnDropToFloor)
+#define WALK_MOVE (*g_engfuncs.pfnWalkMove)
+#define SET_ORIGIN (*g_engfuncs.pfnSetOrigin)
+#define EMIT_SOUND_DYN2 (*g_engfuncs.pfnEmitSound)
+#define BUILD_SOUND_MSG (*g_engfuncs.pfnBuildSoundMsg)
+#define TRACE_LINE (*g_engfuncs.pfnTraceLine)
+#define TRACE_TOSS (*g_engfuncs.pfnTraceToss)
+#define TRACE_MONSTER_HULL (*g_engfuncs.pfnTraceMonsterHull)
+#define TRACE_HULL (*g_engfuncs.pfnTraceHull)
+#define TRACE_MODEL (*g_engfuncs.pfnTraceModel)
+#define GET_AIM_VECTOR (*g_engfuncs.pfnGetAimVector)
+#define SERVER_COMMAND (*g_engfuncs.pfnServerCommand)
+#define SERVER_EXECUTE (*g_engfuncs.pfnServerExecute)
+#define CLIENT_COMMAND (*g_engfuncs.pfnClientCommand)
+#define PARTICLE_EFFECT (*g_engfuncs.pfnParticleEffect)
+#define LIGHT_STYLE (*g_engfuncs.pfnLightStyle)
+#define DECAL_INDEX (*g_engfuncs.pfnDecalIndex)
+#define POINT_CONTENTS (*g_engfuncs.pfnPointContents)
+#define CRC32_INIT (*g_engfuncs.pfnCRC32_Init)
+#define CRC32_PROCESS_BUFFER (*g_engfuncs.pfnCRC32_ProcessBuffer)
+#define CRC32_PROCESS_BYTE (*g_engfuncs.pfnCRC32_ProcessByte)
+#define CRC32_FINAL (*g_engfuncs.pfnCRC32_Final)
+#define RANDOM_LONG (*g_engfuncs.pfnRandomLong)
+#define RANDOM_FLOAT (*g_engfuncs.pfnRandomFloat)
+#define ADD_SERVER_COMMAND (*g_engfuncs.pfnAddServerCommand)
+#define SET_CLIENT_LISTENING (*g_engfuncs.pfnVoice_SetClientListening)
+#define GETPLAYERAUTHID (*g_engfuncs.pfnGetPlayerAuthId)
+#define GET_FILE_SIZE (*g_engfuncs.pfnGetFileSize)
+#define GET_APPROX_WAVE_PLAY_LEN (*g_engfuncs.pfnGetApproxWavePlayLen)
+#define IS_CAREER_MATCH (*g_engfuncs.pfnIsCareerMatch)
+#define GET_LOCALIZED_STRING_LENGTH (*g_engfuncs.pfnGetLocalizedStringLength)
+#define REGISTER_TUTOR_MESSAGE_SHOWN (*g_engfuncs.pfnRegisterTutorMessageShown)
+#define GET_TIMES_TUTOR_MESSAGE_SHOWN (*g_engfuncs.pfnGetTimesTutorMessageShown)
+#define ENG_CHECK_PARM (*g_engfuncs.pfnEngCheckParm)
+
+inline void MESSAGE_BEGIN(int msg_dest, int msg_type, const float *pOrigin = nullptr, edict_t *ed = nullptr)
+{
+ (*g_engfuncs.pfnMessageBegin)(msg_dest, msg_type, pOrigin, ed);
+}
+
+template
+inline T *GET_PRIVATE(edict_t *pEdict)
+{
+ if (pEdict)
+ {
+ return static_cast(pEdict->pvPrivateData);
+ }
+
+ return nullptr;
+}
+
+#define MESSAGE_END (*g_engfuncs.pfnMessageEnd)
+#define WRITE_BYTE (*g_engfuncs.pfnWriteByte)
+#define WRITE_CHAR (*g_engfuncs.pfnWriteChar)
+#define WRITE_SHORT (*g_engfuncs.pfnWriteShort)
+#define WRITE_LONG (*g_engfuncs.pfnWriteLong)
+#define WRITE_ANGLE (*g_engfuncs.pfnWriteAngle)
+#define WRITE_COORD (*g_engfuncs.pfnWriteCoord)
+#define WRITE_STRING (*g_engfuncs.pfnWriteString)
+#define WRITE_ENTITY (*g_engfuncs.pfnWriteEntity)
+#define CVAR_REGISTER (*g_engfuncs.pfnCVarRegister)
+#define CVAR_GET_FLOAT (*g_engfuncs.pfnCVarGetFloat)
+#define CVAR_GET_STRING (*g_engfuncs.pfnCVarGetString)
+#define CVAR_SET_FLOAT (*g_engfuncs.pfnCVarSetFloat)
+#define CVAR_SET_STRING (*g_engfuncs.pfnCVarSetString)
+#define CVAR_GET_POINTER (*g_engfuncs.pfnCVarGetPointer)
+#define ALERT (*g_engfuncs.pfnAlertMessage)
+#define ENGINE_FPRINTF (*g_engfuncs.pfnEngineFprintf)
+#define ALLOC_PRIVATE (*g_engfuncs.pfnPvAllocEntPrivateData)
+#define FREE_PRIVATE (*g_engfuncs.pfnFreeEntPrivateData)
+//#define STRING (*g_engfuncs.pfnSzFromIndex)
+#define ALLOC_STRING (*g_engfuncs.pfnAllocString)
+#define FIND_ENTITY_BY_STRING (*g_engfuncs.pfnFindEntityByString)
+#define GETENTITYILLUM (*g_engfuncs.pfnGetEntityIllum)
+#define FIND_ENTITY_IN_SPHERE (*g_engfuncs.pfnFindEntityInSphere)
+#define FIND_CLIENT_IN_PVS (*g_engfuncs.pfnFindClientInPVS)
+#define FIND_ENTITY_IN_PVS (*g_engfuncs.pfnEntitiesInPVS)
+#define EMIT_AMBIENT_SOUND (*g_engfuncs.pfnEmitAmbientSound)
+#define GET_MODEL_PTR (*g_engfuncs.pfnGetModelPtr)
+#define REG_USER_MSG (*g_engfuncs.pfnRegUserMsg)
+#define GET_BONE_POSITION (*g_engfuncs.pfnGetBonePosition)
+#define FUNCTION_FROM_NAME (*g_engfuncs.pfnFunctionFromName)
+#define NAME_FOR_FUNCTION (*g_engfuncs.pfnNameForFunction)
+#define TRACE_TEXTURE (*g_engfuncs.pfnTraceTexture)
+#define CLIENT_PRINTF (*g_engfuncs.pfnClientPrintf)
+#define SERVER_PRINT (*g_engfuncs.pfnServerPrint)
+#define CMD_ARGS (*g_engfuncs.pfnCmd_Args)
+#define CMD_ARGC (*g_engfuncs.pfnCmd_Argc)
+#define CMD_ARGV (*g_engfuncs.pfnCmd_Argv)
+#define GET_ATTACHMENT (*g_engfuncs.pfnGetAttachment)
+#define SET_VIEW (*g_engfuncs.pfnSetView)
+#define SET_CROSSHAIRANGLE (*g_engfuncs.pfnCrosshairAngle)
+#define LOAD_FILE_FOR_ME (*g_engfuncs.pfnLoadFileForMe)
+#define FREE_FILE (*g_engfuncs.pfnFreeFile)
+#define END_SECTION (*g_engfuncs.pfnEndSection)
+#define COMPARE_FILE_TIME (*g_engfuncs.pfnCompareFileTime)
+#define GET_GAME_DIR (*g_engfuncs.pfnGetGameDir)
+#define SET_CLIENT_MAXSPEED (*g_engfuncs.pfnSetClientMaxspeed)
+#define CREATE_FAKE_CLIENT (*g_engfuncs.pfnCreateFakeClient)
+#define PLAYER_RUN_MOVE (*g_engfuncs.pfnRunPlayerMove)
+#define NUMBER_OF_ENTITIES (*g_engfuncs.pfnNumberOfEntities)
+#define GET_INFO_BUFFER (*g_engfuncs.pfnGetInfoKeyBuffer)
+#define GET_KEY_VALUE (*g_engfuncs.pfnInfoKeyValue)
+#define SET_KEY_VALUE (*g_engfuncs.pfnSetKeyValue)
+#define SET_CLIENT_KEY_VALUE (*g_engfuncs.pfnSetClientKeyValue)
+#define IS_MAP_VALID (*g_engfuncs.pfnIsMapValid)
+#define STATIC_DECAL (*g_engfuncs.pfnStaticDecal)
+#define IS_DEDICATED_SERVER (*g_engfuncs.pfnIsDedicatedServer)
+#define PRECACHE_EVENT (*g_engfuncs.pfnPrecacheEvent)
+#define PLAYBACK_EVENT_FULL (*g_engfuncs.pfnPlaybackEvent)
+#define ENGINE_SET_PVS (*g_engfuncs.pfnSetFatPVS)
+#define ENGINE_SET_PAS (*g_engfuncs.pfnSetFatPAS)
+#define ENGINE_CHECK_VISIBILITY (*g_engfuncs.pfnCheckVisibility)
+#define DELTA_SET (*g_engfuncs.pfnDeltaSetField)
+#define DELTA_UNSET (*g_engfuncs.pfnDeltaUnsetField)
+#define DELTA_ADDENCODER (*g_engfuncs.pfnDeltaAddEncoder)
+#define ENGINE_CURRENT_PLAYER (*g_engfuncs.pfnGetCurrentPlayer)
+#define ENGINE_CANSKIP (*g_engfuncs.pfnCanSkipPlayer)
+#define DELTA_FINDFIELD (*g_engfuncs.pfnDeltaFindField)
+#define DELTA_SETBYINDEX (*g_engfuncs.pfnDeltaSetFieldByIndex)
+#define DELTA_UNSETBYINDEX (*g_engfuncs.pfnDeltaUnsetFieldByIndex)
+#define REMOVE_KEY_VALUE (*g_engfuncs.pfnInfo_RemoveKey)
+#define SET_PHYSICS_KEY_VALUE (*g_engfuncs.pfnSetPhysicsKeyValue)
+#define ENGINE_GETPHYSINFO (*g_engfuncs.pfnGetPhysicsInfoString)
+#define ENGINE_SETGROUPMASK (*g_engfuncs.pfnSetGroupMask)
+#define ENGINE_INSTANCE_BASELINE (*g_engfuncs.pfnCreateInstancedBaseline)
+#define ENGINE_FORCE_UNMODIFIED (*g_engfuncs.pfnForceUnmodified)
+#define PLAYER_CNX_STATS (*g_engfuncs.pfnGetPlayerStats)
diff --git a/dep/hlsdk/dlls/explode.h b/dep/hlsdk/dlls/explode.h
new file mode 100644
index 0000000..67a73f7
--- /dev/null
+++ b/dep/hlsdk/dlls/explode.h
@@ -0,0 +1,56 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class CShower: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Think() = 0;
+ virtual void Touch(CBaseEntity *pOther) = 0;
+};
+
+#define SF_ENVEXPLOSION_NODAMAGE BIT(0) // when set, ENV_EXPLOSION will not actually inflict damage
+#define SF_ENVEXPLOSION_REPEATABLE BIT(1) // can this entity be refired?
+#define SF_ENVEXPLOSION_NOFIREBALL BIT(2) // don't draw the fireball
+#define SF_ENVEXPLOSION_NOSMOKE BIT(3) // don't draw the smoke
+#define SF_ENVEXPLOSION_NODECAL BIT(4) // don't make a scorch mark
+#define SF_ENVEXPLOSION_NOSPARKS BIT(5) // don't make a scorch mark
+
+class CEnvExplosion: public CBaseMonster {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ int m_iMagnitude;
+ int m_spriteScale;
+};
diff --git a/dep/hlsdk/dlls/extdef.h b/dep/hlsdk/dlls/extdef.h
new file mode 100644
index 0000000..ed204fe
--- /dev/null
+++ b/dep/hlsdk/dlls/extdef.h
@@ -0,0 +1,115 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "regamedll_const.h"
+
+#undef DLLEXPORT
+#ifdef _WIN32
+ // Attributes to specify an "exported" function, visible from outside the
+ // DLL.
+ #define DLLEXPORT __declspec(dllexport)
+ // WINAPI should be provided in the windows compiler headers.
+ // It's usually defined to something like "__stdcall".
+
+ #define NOINLINE __declspec(noinline)
+#else
+ #define DLLEXPORT __attribute__((visibility("default")))
+ #define WINAPI /* */
+ #define NOINLINE __attribute__((noinline))
+#endif // _WIN32
+
+// Manual branch optimization for GCC 3.0.0 and newer
+#if !defined(__GNUC__) || __GNUC__ < 3
+ #define likely(x) (x)
+ #define unlikely(x) (x)
+#else
+ #define likely(x) __builtin_expect(!!(x), 1)
+ #define unlikely(x) __builtin_expect(!!(x), 0)
+#endif
+
+const int MAX_MAPNAME_LENGHT = 32;
+
+// Simplified macro for declaring/defining exported DLL functions. They
+// need to be 'extern "C"' so that the C++ compiler enforces parameter
+// type-matching, rather than considering routines with mis-matched
+// arguments/types to be overloaded functions...
+//
+// AFAIK, this is os-independent, but it's included here in osdep.h where
+// DLLEXPORT is defined, for convenience.
+#define C_DLLEXPORT extern "C" DLLEXPORT
+
+enum hash_types_e { CLASSNAME };
+
+// Things that toggle (buttons/triggers/doors) need this
+enum TOGGLE_STATE { TS_AT_TOP, TS_AT_BOTTOM, TS_GOING_UP, TS_GOING_DOWN };
+
+typedef struct hash_item_s
+{
+ entvars_t *pev;
+ struct hash_item_s *next;
+ struct hash_item_s *lastHash;
+ int pevIndex;
+
+} hash_item_t;
+
+typedef struct locksounds
+{
+ string_t sLockedSound;
+ string_t sLockedSentence;
+ string_t sUnlockedSound;
+ string_t sUnlockedSentence;
+ int iLockedSentence;
+ int iUnlockedSentence;
+ float flwaitSound;
+ float flwaitSentence;
+ byte bEOFLocked;
+ byte bEOFUnlocked;
+
+} locksound_t;
+
+typedef struct hudtextparms_s
+{
+ float x;
+ float y;
+ int effect;
+ byte r1,g1,b1,a1;
+ byte r2,g2,b2,a2;
+ float fadeinTime;
+ float fadeoutTime;
+ float holdTime;
+ float fxTime;
+ int channel;
+
+} hudtextparms_t;
+
+enum USE_TYPE { USE_OFF, USE_ON, USE_SET, USE_TOGGLE };
+enum IGNORE_MONSTERS { ignore_monsters = 1, dont_ignore_monsters = 0, missile = 2 };
+enum IGNORE_GLASS { ignore_glass = 1, dont_ignore_glass = 0 };
+enum { point_hull = 0, human_hull = 1, large_hull = 2, head_hull = 3 };
diff --git a/dep/hlsdk/dlls/extdll.h b/dep/hlsdk/dlls/extdll.h
new file mode 100644
index 0000000..1c242c3
--- /dev/null
+++ b/dep/hlsdk/dlls/extdll.h
@@ -0,0 +1,82 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#pragma warning(disable:4244) // int or float down-conversion
+#pragma warning(disable:4305) // int or float data truncation
+#pragma warning(disable:4201) // nameless struct/union
+#pragma warning(disable:4514) // unreferenced inline function removed
+#pragma warning(disable:4100) // unreferenced formal parameter
+
+#include "basetypes.h"
+#include "maintypes.h"
+#include "strtools.h"
+
+#ifdef _WIN32
+ #define WIN32_LEAN_AND_MEAN
+ #define NOWINRES
+ #define NOSERVICE
+ #define NOMCX
+ #define NOIME
+ #include "winsani_in.h"
+ #include "windows.h"
+ #include "winsani_out.h"
+ #undef PlaySound
+#else
+ #include
+ #include
+ #include
+#endif // _WIN32
+
+// Misc C-runtime library headers
+#include "stdio.h"
+#include "stdlib.h"
+#include "math.h"
+
+// Header file containing definition of globalvars_t and entvars_t
+typedef int EOFFSET; // More explicit than "int"
+typedef unsigned int func_t;
+typedef unsigned int string_t; // from engine's pr_comp.h;
+typedef float vec_t; // needed before including progdefs.h
+
+// Vector class
+#include "vector.h"
+//#include "vector.h"
+// Defining it as a (bogus) struct helps enforce type-checking
+#define vec3_t Vector
+// Shared engine/DLL constants
+
+#include "const.h"
+#include "edict.h"
+
+// Shared header describing protocol between engine and DLLs
+#include "eiface.h"
+// Shared header between the client DLL and the game DLLs
+#include "cdll_dll.h"
+#include "extdef.h"
diff --git a/dep/hlsdk/dlls/func_break.h b/dep/hlsdk/dlls/func_break.h
new file mode 100644
index 0000000..c618a31
--- /dev/null
+++ b/dep/hlsdk/dlls/func_break.h
@@ -0,0 +1,118 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+enum Explosions
+{
+ expRandom = 0,
+ expDirected,
+};
+
+enum Materials
+{
+ matGlass = 0,
+ matWood,
+ matMetal,
+ matFlesh,
+ matCinderBlock,
+ matCeilingTile,
+ matComputer,
+ matUnbreakableGlass,
+ matRocks,
+ matNone,
+ matLastMaterial,
+};
+
+// this many shards spawned when breakable objects break
+#define NUM_SHARDS 6 // this many shards spawned when breakable objects break
+
+// func breakable
+#define SF_BREAK_TRIGGER_ONLY BIT(0) // may only be broken by trigger
+#define SF_BREAK_TOUCH BIT(1) // can be 'crashed through' by running player (plate glass)
+#define SF_BREAK_PRESSURE BIT(2) // can be broken by a player standing on it
+#define SF_BREAK_CROWBAR BIT(8) // instant break if hit with crowbar
+
+class CBreakable: public CBaseDelay {
+public:
+ // basic functions
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Restart() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+
+ // To spark when hit
+ virtual void TraceAttack(entvars_t *pevAttacker, float flDamage, Vector vecDir, TraceResult *ptr, int bitsDamageType) = 0;
+
+ // breakables use an overridden takedamage
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+
+ virtual int DamageDecal(int bitsDamageType) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+
+public:
+ BOOL Explodable() const { return ExplosionMagnitude() > 0; }
+ int ExplosionMagnitude() const { return pev->impulse; }
+ void ExplosionSetMagnitude(int magnitude) { pev->impulse = magnitude; }
+
+public:
+ Materials m_Material;
+ Explosions m_Explosion;
+ int m_idShard;
+ float m_angle;
+ int m_iszGibModel;
+ int m_iszSpawnObject;
+ float m_flHealth;
+};
+
+#define SF_PUSH_BREAKABLE BIT(7) // func_pushable (it's also func_breakable, so don't collide with those flags)
+
+class CPushable: public CBreakable {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Restart() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+ virtual void Touch(CBaseEntity *pOther) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+
+public:
+ float MaxSpeed() const { return m_maxSpeed; }
+
+public:
+ int m_lastSound;
+ float m_maxSpeed;
+ float m_soundTime;
+};
diff --git a/dep/hlsdk/dlls/func_tank.h b/dep/hlsdk/dlls/func_tank.h
new file mode 100644
index 0000000..63c3ff8
--- /dev/null
+++ b/dep/hlsdk/dlls/func_tank.h
@@ -0,0 +1,161 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+enum TANKBULLET
+{
+ TANK_BULLET_NONE = 0, // Custom damage
+ TANK_BULLET_9MM, // env_laser (duration is 0.5 rate of fire)
+ TANK_BULLET_MP5, // rockets
+ TANK_BULLET_12MM, // explosion?
+};
+
+#define SF_TANK_ACTIVE BIT(0)
+#define SF_TANK_PLAYER BIT(1)
+#define SF_TANK_HUMANS BIT(2)
+#define SF_TANK_ALIENS BIT(3)
+#define SF_TANK_LINEOFSIGHT BIT(4)
+#define SF_TANK_CANCONTROL BIT(5)
+
+#define SF_TANK_SOUNDON BIT(15)
+
+class CFuncTank: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+
+ // Bmodels don't go across transitions
+ virtual int ObjectCaps() = 0;
+ virtual BOOL OnControls(entvars_t *pevTest) = 0;
+ virtual void Think() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+ virtual void Fire(const Vector &barrelEnd, const Vector &forward, entvars_t *pevAttacker) = 0;
+ virtual Vector UpdateTargetPosition(CBaseEntity *pTarget) = 0;
+public:
+ BOOL IsActive() const { return (pev->spawnflags & SF_TANK_ACTIVE) == SF_TANK_ACTIVE; }
+ void TankActivate()
+ {
+ pev->spawnflags |= SF_TANK_ACTIVE;
+ pev->nextthink = pev->ltime + 0.1f;
+ m_fireLast = 0.0f;
+ }
+ void TankDeactivate()
+ {
+ pev->spawnflags &= ~SF_TANK_ACTIVE;
+ m_fireLast = 0.0f;
+ StopRotSound();
+ }
+
+ BOOL CanFire() const { return (gpGlobals->time - m_lastSightTime) < m_persist; }
+ Vector BarrelPosition()
+ {
+ Vector forward, right, up;
+ UTIL_MakeVectorsPrivate(pev->angles, forward, right, up);
+ return pev->origin + (forward * m_barrelPos.x) + (right * m_barrelPos.y) + (up * m_barrelPos.z);
+ }
+protected:
+ CBasePlayer *m_pController;
+ float m_flNextAttack;
+ Vector m_vecControllerUsePos;
+
+ float m_yawCenter; // "Center" yaw
+ float m_yawRate; // Max turn rate to track targets
+ float m_yawRange; // Range of turning motion (one-sided: 30 is +/- 30 degress from center)
+ // Zero is full rotation
+
+ float m_yawTolerance; // Tolerance angle
+
+ float m_pitchCenter; // "Center" pitch
+ float m_pitchRate; // Max turn rate on pitch
+ float m_pitchRange; // Range of pitch motion as above
+ float m_pitchTolerance; // Tolerance angle
+
+ float m_fireLast; // Last time I fired
+ float m_fireRate; // How many rounds/second
+ float m_lastSightTime; // Last time I saw target
+ float m_persist; // Persistence of firing (how long do I shoot when I can't see)
+ float m_minRange; // Minimum range to aim/track
+ float m_maxRange; // Max range to aim/track
+
+ Vector m_barrelPos; // Length of the freakin barrel
+ float m_spriteScale; // Scale of any sprites we shoot
+ int m_iszSpriteSmoke;
+ int m_iszSpriteFlash;
+ TANKBULLET m_bulletType;// Bullet type
+ int m_iBulletDamage; // 0 means use Bullet type's default damage
+
+ Vector m_sightOrigin; // Last sight of target
+ int m_spread; // firing spread
+ int m_iszMaster; // Master entity (game_team_master or multisource)
+};
+
+class CFuncTankGun: public CFuncTank {
+public:
+ virtual void Fire(const Vector &barrelEnd, const Vector &forward, entvars_t *pevAttacker) = 0;
+};
+
+class CFuncTankLaser: public CFuncTank {
+public:
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Activate() = 0;
+ virtual void Think() = 0;
+ virtual void Fire(const Vector &barrelEnd, const Vector &forward, entvars_t *pevAttacker) = 0;
+public:
+ CLaser *m_pLaser;
+ float m_laserTime;
+};
+
+class CFuncTankRocket: public CFuncTank {
+public:
+ virtual void Precache() = 0;
+ virtual void Fire(const Vector &barrelEnd, const Vector &forward, entvars_t *pevAttacker) = 0;
+};
+
+class CFuncTankMortar: public CFuncTank {
+public:
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual void Fire(const Vector &barrelEnd, const Vector &forward, entvars_t *pevAttacker) = 0;
+};
+
+class CFuncTankControls: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Think() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ CFuncTank *m_pTank;
+};
diff --git a/dep/hlsdk/dlls/gamerules.h b/dep/hlsdk/dlls/gamerules.h
new file mode 100644
index 0000000..aa9ee6e
--- /dev/null
+++ b/dep/hlsdk/dlls/gamerules.h
@@ -0,0 +1,690 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+
+#include "voice_gamemgr.h"
+
+const int MAX_RULE_BUFFER = 1024;
+const int MAX_VOTE_MAPS = 100;
+const int MAX_VIP_QUEUES = 5;
+
+const int MAX_MOTD_CHUNK = 60;
+const int MAX_MOTD_LENGTH = 1536; // (MAX_MOTD_CHUNK * 4)
+
+const float ITEM_RESPAWN_TIME = 30;
+const float WEAPON_RESPAWN_TIME = 20;
+const float AMMO_RESPAWN_TIME = 20;
+const float ROUND_RESPAWN_TIME = 20;
+const float ROUND_BEGIN_DELAY = 5; // delay before beginning new round
+
+// longest the intermission can last, in seconds
+const int MAX_INTERMISSION_TIME = 120;
+
+// when we are within this close to running out of entities, items
+// marked with the ITEM_FLAG_LIMITINWORLD will delay their respawn
+const int ENTITY_INTOLERANCE = 100;
+
+// custom enum
+#define WINNER_NONE 0
+#define WINNER_DRAW 1
+
+enum
+{
+ WINSTATUS_NONE = 0,
+ WINSTATUS_CTS,
+ WINSTATUS_TERRORISTS,
+ WINSTATUS_DRAW,
+};
+
+// Custom enum
+// Used for EndRoundMessage() logged messages
+enum ScenarioEventEndRound
+{
+ ROUND_NONE,
+ ROUND_TARGET_BOMB,
+ ROUND_VIP_ESCAPED,
+ ROUND_VIP_ASSASSINATED,
+ ROUND_TERRORISTS_ESCAPED,
+ ROUND_CTS_PREVENT_ESCAPE,
+ ROUND_ESCAPING_TERRORISTS_NEUTRALIZED,
+ ROUND_BOMB_DEFUSED,
+ ROUND_CTS_WIN,
+ ROUND_TERRORISTS_WIN,
+ ROUND_END_DRAW,
+ ROUND_ALL_HOSTAGES_RESCUED,
+ ROUND_TARGET_SAVED,
+ ROUND_HOSTAGE_NOT_RESCUED,
+ ROUND_TERRORISTS_NOT_ESCAPED,
+ ROUND_VIP_NOT_ESCAPED,
+ ROUND_GAME_COMMENCE,
+ ROUND_GAME_RESTART,
+ ROUND_GAME_OVER
+};
+
+enum RewardRules
+{
+ RR_CTS_WIN,
+ RR_TERRORISTS_WIN,
+ RR_TARGET_BOMB,
+ RR_VIP_ESCAPED,
+ RR_VIP_ASSASSINATED,
+ RR_TERRORISTS_ESCAPED,
+ RR_CTS_PREVENT_ESCAPE,
+ RR_ESCAPING_TERRORISTS_NEUTRALIZED,
+ RR_BOMB_DEFUSED,
+ RR_BOMB_PLANTED,
+ RR_BOMB_EXPLODED,
+ RR_ALL_HOSTAGES_RESCUED,
+ RR_TARGET_BOMB_SAVED,
+ RR_HOSTAGE_NOT_RESCUED,
+ RR_VIP_NOT_ESCAPED,
+ RR_LOSER_BONUS_DEFAULT,
+ RR_LOSER_BONUS_MIN,
+ RR_LOSER_BONUS_MAX,
+ RR_LOSER_BONUS_ADD,
+ RR_RESCUED_HOSTAGE,
+ RR_TOOK_HOSTAGE_ACC,
+ RR_TOOK_HOSTAGE,
+ RR_END
+};
+
+// custom enum
+enum RewardAccount
+{
+ REWARD_TARGET_BOMB = 3500,
+ REWARD_VIP_ESCAPED = 3500,
+ REWARD_VIP_ASSASSINATED = 3250,
+ REWARD_TERRORISTS_ESCAPED = 3150,
+ REWARD_CTS_PREVENT_ESCAPE = 3500,
+ REWARD_ESCAPING_TERRORISTS_NEUTRALIZED = 3250,
+ REWARD_BOMB_DEFUSED = 3250,
+ REWARD_BOMB_PLANTED = 800,
+ REWARD_BOMB_EXPLODED = 3250,
+ REWARD_CTS_WIN = 3000,
+ REWARD_TERRORISTS_WIN = 3000,
+ REWARD_ALL_HOSTAGES_RESCUED = 2500,
+
+ // the end round was by the expiration time
+ REWARD_TARGET_BOMB_SAVED = 3250,
+ REWARD_HOSTAGE_NOT_RESCUED = 3250,
+ REWARD_VIP_NOT_ESCAPED = 3250,
+
+ // loser bonus
+ REWARD_LOSER_BONUS_DEFAULT = 1400,
+ REWARD_LOSER_BONUS_MIN = 1500,
+ REWARD_LOSER_BONUS_MAX = 3000,
+ REWARD_LOSER_BONUS_ADD = 500,
+
+ REWARD_RESCUED_HOSTAGE = 750,
+ REWARD_KILLED_ENEMY = 300,
+ REWARD_KILLED_VIP = 2500,
+ REWARD_VIP_HAVE_SELF_RESCUED = 2500,
+
+ REWARD_TAKEN_HOSTAGE = 1000,
+ REWARD_TOOK_HOSTAGE_ACC = 100,
+ REWARD_TOOK_HOSTAGE = 150,
+};
+
+// custom enum
+enum PaybackForBadThing
+{
+ PAYBACK_FOR_KILLED_TEAMMATES = -3300,
+};
+
+// custom enum
+enum InfoMapBuyParam
+{
+ BUYING_EVERYONE = 0,
+ BUYING_ONLY_CTS,
+ BUYING_ONLY_TERRORISTS,
+ BUYING_NO_ONE,
+};
+
+// weapon respawning return codes
+enum
+{
+ GR_NONE = 0,
+
+ GR_WEAPON_RESPAWN_YES,
+ GR_WEAPON_RESPAWN_NO,
+
+ GR_AMMO_RESPAWN_YES,
+ GR_AMMO_RESPAWN_NO,
+
+ GR_ITEM_RESPAWN_YES,
+ GR_ITEM_RESPAWN_NO,
+
+ GR_PLR_DROP_GUN_ALL,
+ GR_PLR_DROP_GUN_ACTIVE,
+ GR_PLR_DROP_GUN_NO,
+
+ GR_PLR_DROP_AMMO_ALL,
+ GR_PLR_DROP_AMMO_ACTIVE,
+ GR_PLR_DROP_AMMO_NO,
+};
+
+// custom enum
+enum
+{
+ SCENARIO_BLOCK_TIME_EXPRIRED = BIT(0), // flag "a"
+ SCENARIO_BLOCK_NEED_PLAYERS = BIT(1), // flag "b"
+ SCENARIO_BLOCK_VIP_ESCAPE = BIT(2), // flag "c"
+ SCENARIO_BLOCK_PRISON_ESCAPE = BIT(3), // flag "d"
+ SCENARIO_BLOCK_BOMB = BIT(4), // flag "e"
+ SCENARIO_BLOCK_TEAM_EXTERMINATION = BIT(5), // flag "f"
+ SCENARIO_BLOCK_HOSTAGE_RESCUE = BIT(6), // flag "g"
+};
+
+// Player relationship return codes
+enum
+{
+ GR_NOTTEAMMATE = 0,
+ GR_TEAMMATE,
+ GR_ENEMY,
+ GR_ALLY,
+ GR_NEUTRAL,
+};
+
+class CItem;
+
+class CGameRules {
+protected:
+ virtual ~CGameRules() {};
+public:
+ virtual void RefreshSkillData() = 0; // fill skill data struct with proper values
+ virtual void Think() = 0; // runs every server frame, should handle any timer tasks, periodic events, etc.
+ virtual BOOL IsAllowedToSpawn(CBaseEntity *pEntity) = 0; // Can this item spawn (eg monsters don't spawn in deathmatch).
+
+ virtual BOOL FAllowFlashlight() = 0; // Are players allowed to switch on their flashlight?
+ virtual BOOL FShouldSwitchWeapon(CBasePlayer *pPlayer, CBasePlayerItem *pWeapon) = 0; // should the player switch to this weapon?
+ virtual BOOL GetNextBestWeapon(CBasePlayer *pPlayer, CBasePlayerItem *pCurrentWeapon) = 0; // I can't use this weapon anymore, get me the next best one.
+
+ // Functions to verify the single/multiplayer status of a game
+ virtual BOOL IsMultiplayer() = 0; // is this a multiplayer game? (either coop or deathmatch)
+ virtual BOOL IsDeathmatch() = 0; // is this a deathmatch game?
+ virtual BOOL IsTeamplay() = 0; // is this deathmatch game being played with team rules?
+ virtual BOOL IsCoOp() = 0; // is this a coop game?
+ virtual const char *GetGameDescription() = 0; // this is the game name that gets seen in the server browser
+
+ // Client connection/disconnection
+ virtual BOOL ClientConnected(edict_t *pEntity, const char *pszName, const char *pszAddress, char *szRejectReason) = 0; // a client just connected to the server (player hasn't spawned yet)
+ virtual void InitHUD(CBasePlayer *pl) = 0; // the client dll is ready for updating
+ virtual void ClientDisconnected(edict_t *pClient) = 0; // a client just disconnected from the server
+ virtual void UpdateGameMode(CBasePlayer *pPlayer) = 0; // the client needs to be informed of the current game mode
+
+ // Client damage rules
+ virtual float FlPlayerFallDamage(CBasePlayer *pPlayer) = 0;
+ virtual BOOL FPlayerCanTakeDamage(CBasePlayer *pPlayer, CBaseEntity *pAttacker) = 0; // can this player take damage from this attacker?
+ virtual BOOL ShouldAutoAim(CBasePlayer *pPlayer, edict_t *target) = 0;
+
+ // Client spawn/respawn control
+ virtual void PlayerSpawn(CBasePlayer *pPlayer) = 0; // called by CBasePlayer::Spawn just before releasing player into the game
+ virtual void PlayerThink(CBasePlayer *pPlayer) = 0; // called by CBasePlayer::PreThink every frame, before physics are run and after keys are accepted
+ virtual BOOL FPlayerCanRespawn(CBasePlayer *pPlayer) = 0; // is this player allowed to respawn now?
+ virtual float FlPlayerSpawnTime(CBasePlayer *pPlayer) = 0; // When in the future will this player be able to spawn?
+ virtual edict_t *GetPlayerSpawnSpot(CBasePlayer *pPlayer) = 0; // Place this player on their spawnspot and face them the proper direction.
+
+ virtual BOOL AllowAutoTargetCrosshair() = 0;
+ virtual BOOL ClientCommand_DeadOrAlive(CBasePlayer *pPlayer, const char *pcmd) = 0;
+ virtual BOOL ClientCommand(CBasePlayer *pPlayer, const char *pcmd) = 0; // handles the user commands; returns TRUE if command handled properly
+ virtual void ClientUserInfoChanged(CBasePlayer *pPlayer, char *infobuffer) = 0; // the player has changed userinfo; can change it now
+
+ // Client kills/scoring
+ virtual int IPointsForKill(CBasePlayer *pAttacker, CBasePlayer *pKilled) = 0; // how many points do I award whoever kills this player?
+ virtual void PlayerKilled(CBasePlayer *pVictim, entvars_t *pKiller, entvars_t *pInflictor) = 0; // Called each time a player dies
+ virtual void DeathNotice(CBasePlayer *pVictim, entvars_t *pKiller, entvars_t *pevInflictor) = 0; // Call this from within a GameRules class to report an obituary.
+
+ // Weapon retrieval
+ virtual BOOL CanHavePlayerItem(CBasePlayer *pPlayer, CBasePlayerItem *pItem) = 0; // The player is touching an CBasePlayerItem, do I give it to him?
+ virtual void PlayerGotWeapon(CBasePlayer *pPlayer, CBasePlayerItem *pWeapon) = 0; // Called each time a player picks up a weapon from the ground
+
+ // Weapon spawn/respawn control
+ virtual int WeaponShouldRespawn(CBasePlayerItem *pWeapon) = 0; // should this weapon respawn?
+ virtual float FlWeaponRespawnTime(CBasePlayerItem *pWeapon) = 0; // when may this weapon respawn?
+ virtual float FlWeaponTryRespawn(CBasePlayerItem *pWeapon) = 0; // can i respawn now, and if not, when should i try again?
+ virtual Vector VecWeaponRespawnSpot(CBasePlayerItem *pWeapon) = 0; // where in the world should this weapon respawn?
+
+ // Item retrieval
+ virtual BOOL CanHaveItem(CBasePlayer *pPlayer, CItem *pItem) = 0; // is this player allowed to take this item?
+ virtual void PlayerGotItem(CBasePlayer *pPlayer, CItem *pItem) = 0; // call each time a player picks up an item (battery, healthkit, longjump)
+
+ // Item spawn/respawn control
+ virtual int ItemShouldRespawn(CItem *pItem) = 0; // Should this item respawn?
+ virtual float FlItemRespawnTime(CItem *pItem) = 0; // when may this item respawn?
+ virtual Vector VecItemRespawnSpot(CItem *pItem) = 0; // where in the world should this item respawn?
+
+ // Ammo retrieval
+ virtual BOOL CanHaveAmmo(CBasePlayer *pPlayer, const char *pszAmmoName, int iMaxCarry) = 0; // can this player take more of this ammo?
+ virtual void PlayerGotAmmo(CBasePlayer *pPlayer, char *szName, int iCount) = 0; // called each time a player picks up some ammo in the world
+
+ // Ammo spawn/respawn control
+ virtual int AmmoShouldRespawn(CBasePlayerAmmo *pAmmo) = 0; // should this ammo item respawn?
+ virtual float FlAmmoRespawnTime(CBasePlayerAmmo *pAmmo) = 0; // when should this ammo item respawn?
+ virtual Vector VecAmmoRespawnSpot(CBasePlayerAmmo *pAmmo) = 0; // where in the world should this ammo item respawn?
+
+ // Healthcharger respawn control
+ virtual float FlHealthChargerRechargeTime() = 0; // how long until a depleted HealthCharger recharges itself?
+ virtual float FlHEVChargerRechargeTime() = 0; // how long until a depleted HealthCharger recharges itself?
+
+ // What happens to a dead player's weapons
+ virtual int DeadPlayerWeapons(CBasePlayer *pPlayer) = 0; // what do I do with a player's weapons when he's killed?
+
+ // What happens to a dead player's ammo
+ virtual int DeadPlayerAmmo(CBasePlayer *pPlayer) = 0; // Do I drop ammo when the player dies? How much?
+
+ // Teamplay stuff
+ virtual const char *GetTeamID(CBaseEntity *pEntity) = 0; // what team is this entity on?
+ virtual int PlayerRelationship(CBasePlayer *pPlayer, CBaseEntity *pTarget) = 0; // What is the player's relationship with this entity?
+ virtual int GetTeamIndex(const char *pTeamName) = 0;
+ virtual const char *GetIndexedTeamName(int teamIndex) = 0;
+ virtual BOOL IsValidTeam(const char *pTeamName) = 0;
+ virtual void ChangePlayerTeam(CBasePlayer *pPlayer, const char *pTeamName, BOOL bKill, BOOL bGib) = 0;
+ virtual const char *SetDefaultPlayerTeam(CBasePlayer *pPlayer) = 0;
+
+ // Sounds
+ virtual BOOL PlayTextureSounds() = 0;
+
+ // Monsters
+ virtual BOOL FAllowMonsters() = 0; // are monsters allowed
+
+ // Immediately end a multiplayer game
+ virtual void EndMultiplayerGame() = 0;
+
+ // Stuff that is shared between client and server.
+ virtual BOOL IsFreezePeriod() = 0;
+ virtual void ServerDeactivate() = 0;
+ virtual void CheckMapConditions() = 0;
+
+ // inline function's
+ inline bool IsGameOver() const { return m_bGameOver; }
+ inline void SetGameOver() { m_bGameOver = true; }
+
+public:
+ BOOL m_bFreezePeriod; // TRUE at beginning of round, set to FALSE when the period expires
+ BOOL m_bBombDropped;
+
+ // custom
+ char *m_GameDesc;
+ bool m_bGameOver; // intermission or finale (deprecated name g_fGameOver)
+};
+
+// CHalfLifeRules - rules for the single player Half-Life game.
+class CHalfLifeRules: public CGameRules {
+protected:
+ virtual ~CHalfLifeRules() {};
+public:
+ virtual void Think() = 0;
+ virtual BOOL IsAllowedToSpawn(CBaseEntity *pEntity) = 0;
+ virtual BOOL FAllowFlashlight() = 0;
+
+ virtual BOOL FShouldSwitchWeapon(CBasePlayer *pPlayer, CBasePlayerItem *pWeapon) = 0;
+ virtual BOOL GetNextBestWeapon(CBasePlayer *pPlayer, CBasePlayerItem *pCurrentWeapon) = 0;
+
+ // Functions to verify the single/multiplayer status of a game
+ virtual BOOL IsMultiplayer() = 0;
+ virtual BOOL IsDeathmatch() = 0;
+ virtual BOOL IsCoOp() = 0;
+
+ // Client connection/disconnection
+ virtual BOOL ClientConnected(edict_t *pEntity, const char *pszName, const char *pszAddress, char szRejectReason[128]) = 0;
+ virtual void InitHUD(CBasePlayer *pl) = 0; // the client dll is ready for updating
+ virtual void ClientDisconnected(edict_t *pClient) = 0;
+
+ // Client damage rules
+ virtual float FlPlayerFallDamage(CBasePlayer *pPlayer) = 0;
+
+ // Client spawn/respawn control
+ virtual void PlayerSpawn(CBasePlayer *pPlayer) = 0;
+ virtual void PlayerThink(CBasePlayer *pPlayer) = 0;
+ virtual BOOL FPlayerCanRespawn(CBasePlayer *pPlayer) = 0;
+ virtual float FlPlayerSpawnTime(CBasePlayer *pPlayer) = 0;
+ virtual edict_t *GetPlayerSpawnSpot(CBasePlayer *pPlayer) = 0;
+
+ virtual BOOL AllowAutoTargetCrosshair() = 0;
+
+ // Client kills/scoring
+ virtual int IPointsForKill(CBasePlayer *pAttacker, CBasePlayer *pKilled) = 0;
+ virtual void PlayerKilled(CBasePlayer *pVictim, entvars_t *pKiller, entvars_t *pInflictor) = 0;
+ virtual void DeathNotice(CBasePlayer *pVictim, entvars_t *pKiller, entvars_t *pInflictor) = 0;
+
+ // Weapon retrieval
+ virtual void PlayerGotWeapon(CBasePlayer *pPlayer, CBasePlayerItem *pWeapon) = 0;
+
+ // Weapon spawn/respawn control
+ virtual int WeaponShouldRespawn(CBasePlayerItem *pWeapon) = 0;
+ virtual float FlWeaponRespawnTime(CBasePlayerItem *pWeapon) = 0;
+ virtual float FlWeaponTryRespawn(CBasePlayerItem *pWeapon) = 0;
+ virtual Vector VecWeaponRespawnSpot(CBasePlayerItem *pWeapon) = 0;
+
+ // Item retrieval
+ virtual BOOL CanHaveItem(CBasePlayer *pPlayer, CItem *pItem) = 0;
+ virtual void PlayerGotItem(CBasePlayer *pPlayer, CItem *pItem) = 0;
+
+ // Item spawn/respawn control
+ virtual int ItemShouldRespawn(CItem *pItem) = 0;
+ virtual float FlItemRespawnTime(CItem *pItem) = 0;
+ virtual Vector VecItemRespawnSpot(CItem *pItem) = 0;
+
+ // Ammo retrieval
+ virtual void PlayerGotAmmo(CBasePlayer *pPlayer, char *szName, int iCount) = 0;
+
+ // Ammo spawn/respawn control
+ virtual int AmmoShouldRespawn(CBasePlayerAmmo *pAmmo) = 0;
+ virtual float FlAmmoRespawnTime(CBasePlayerAmmo *pAmmo) = 0;
+ virtual Vector VecAmmoRespawnSpot(CBasePlayerAmmo *pAmmo) = 0;
+
+ // Healthcharger respawn control
+ virtual float FlHealthChargerRechargeTime() = 0;
+
+ // What happens to a dead player's weapons
+ virtual int DeadPlayerWeapons(CBasePlayer *pPlayer) = 0;
+
+ // What happens to a dead player's ammo
+ virtual int DeadPlayerAmmo(CBasePlayer *pPlayer) = 0;
+
+ // Teamplay stuff
+ virtual const char *GetTeamID(CBaseEntity *pEntity) = 0;
+ virtual int PlayerRelationship(CBasePlayer *pPlayer, CBaseEntity *pTarget) = 0;
+
+ // Monsters
+ virtual BOOL FAllowMonsters() = 0;
+};
+
+// CHalfLifeMultiplay - rules for the basic half life multiplayer competition
+class CHalfLifeMultiplay: public CGameRules {
+protected:
+ virtual ~CHalfLifeMultiplay() {};
+public:
+ virtual void RefreshSkillData() = 0;
+ virtual void Think() = 0;
+ virtual BOOL IsAllowedToSpawn(CBaseEntity *pEntity) = 0;
+ virtual BOOL FAllowFlashlight() = 0;
+
+ virtual BOOL FShouldSwitchWeapon(CBasePlayer *pPlayer, CBasePlayerItem *pWeapon) = 0;
+ virtual BOOL GetNextBestWeapon(CBasePlayer *pPlayer, CBasePlayerItem *pCurrentWeapon) = 0;
+
+ virtual BOOL IsMultiplayer() = 0;
+ virtual BOOL IsDeathmatch() = 0;
+ virtual BOOL IsCoOp() = 0;
+
+ // Client connection/disconnection
+ // If ClientConnected returns FALSE, the connection is rejected and the user is provided the reason specified in szRejectReason
+ // Only the client's name and remote address are provided to the dll for verification.
+ virtual BOOL ClientConnected(edict_t *pEntity, const char *pszName, const char *pszAddress, char szRejectReason[128]) = 0;
+ virtual void InitHUD(CBasePlayer *pl) = 0;
+ virtual void ClientDisconnected(edict_t *pClient) = 0;
+ virtual void UpdateGameMode(CBasePlayer *pPlayer) = 0;
+
+ // Client damage rules
+ virtual float FlPlayerFallDamage(CBasePlayer *pPlayer) = 0;
+ virtual BOOL FPlayerCanTakeDamage(CBasePlayer *pPlayer, CBaseEntity *pAttacker) = 0;
+
+ // Client spawn/respawn control
+ virtual void PlayerSpawn(CBasePlayer *pPlayer) = 0;
+ virtual void PlayerThink(CBasePlayer *pPlayer) = 0;
+ virtual BOOL FPlayerCanRespawn(CBasePlayer *pPlayer) = 0;
+ virtual float FlPlayerSpawnTime(CBasePlayer *pPlayer) = 0;
+ virtual edict_t *GetPlayerSpawnSpot(CBasePlayer *pPlayer) = 0;
+
+ virtual BOOL AllowAutoTargetCrosshair() = 0;
+
+ virtual BOOL ClientCommand_DeadOrAlive(CBasePlayer *pPlayer, const char *pcmd) = 0;
+ virtual BOOL ClientCommand(CBasePlayer *pPlayer, const char *pcmd) = 0;
+ virtual void ClientUserInfoChanged(CBasePlayer *pPlayer, char *infobuffer) = 0;
+
+ // Client kills/scoring
+ virtual int IPointsForKill(CBasePlayer *pAttacker, CBasePlayer *pKilled) = 0;
+ virtual void PlayerKilled(CBasePlayer *pVictim, entvars_t *pKiller, entvars_t *pInflictor) = 0;
+ virtual void DeathNotice(CBasePlayer *pVictim, entvars_t *pKiller, entvars_t *pInflictor) = 0;
+
+ // Weapon retrieval
+ virtual BOOL CanHavePlayerItem(CBasePlayer *pPlayer, CBasePlayerItem *pWeapon) = 0;
+ virtual void PlayerGotWeapon(CBasePlayer *pPlayer, CBasePlayerItem *pWeapon) = 0;
+
+ // Weapon spawn/respawn control
+ virtual int WeaponShouldRespawn(CBasePlayerItem *pWeapon) = 0;
+ virtual float FlWeaponRespawnTime(CBasePlayerItem *pWeapon) = 0;
+ virtual float FlWeaponTryRespawn(CBasePlayerItem *pWeapon) = 0;
+ virtual Vector VecWeaponRespawnSpot(CBasePlayerItem *pWeapon) = 0;
+
+ // Item retrieval
+ virtual BOOL CanHaveItem(CBasePlayer *pPlayer, CItem *pItem) = 0;
+ virtual void PlayerGotItem(CBasePlayer *pPlayer, CItem *pItem) = 0;
+
+ // Item spawn/respawn control
+ virtual int ItemShouldRespawn(CItem *pItem) = 0;
+ virtual float FlItemRespawnTime(CItem *pItem) = 0;
+ virtual Vector VecItemRespawnSpot(CItem *pItem) = 0;
+
+ // Ammo retrieval
+ virtual void PlayerGotAmmo(CBasePlayer *pPlayer, char *szName, int iCount) = 0;
+
+ // Ammo spawn/respawn control
+ virtual int AmmoShouldRespawn(CBasePlayerAmmo *pAmmo) = 0;
+ virtual float FlAmmoRespawnTime(CBasePlayerAmmo *pAmmo) = 0;
+ virtual Vector VecAmmoRespawnSpot(CBasePlayerAmmo *pAmmo) = 0;
+
+ // Healthcharger respawn control
+ virtual float FlHealthChargerRechargeTime() = 0;
+ virtual float FlHEVChargerRechargeTime() = 0;
+
+ // What happens to a dead player's weapons
+ virtual int DeadPlayerWeapons(CBasePlayer *pPlayer) = 0;
+
+ // What happens to a dead player's ammo
+ virtual int DeadPlayerAmmo(CBasePlayer *pPlayer) = 0;
+
+ // Teamplay stuff
+ virtual const char *GetTeamID(CBaseEntity *pEntity) = 0;
+ virtual int PlayerRelationship(CBasePlayer *pPlayer, CBaseEntity *pTarget) = 0;
+ virtual void ChangePlayerTeam(CBasePlayer *pPlayer, const char *pTeamName, BOOL bKill, BOOL bGib) = 0;
+
+ virtual BOOL PlayTextureSounds() = 0;
+
+ // Monsters
+ virtual BOOL FAllowMonsters() = 0;
+
+ // Immediately end a multiplayer game
+ virtual void EndMultiplayerGame() = 0;
+ virtual void ServerDeactivate() = 0;
+ virtual void CheckMapConditions() = 0;
+
+ // Recreate all the map entities from the map data (preserving their indices),
+ // then remove everything else except the players.
+ // Also get rid of all world decals.
+ virtual void CleanUpMap() = 0;
+
+ virtual void RestartRound() = 0;
+
+ // check if the scenario has been won/lost
+ virtual void CheckWinConditions() = 0;
+ virtual void RemoveGuns() = 0;
+ virtual void GiveC4() = 0;
+ virtual void ChangeLevel() = 0;
+ virtual void GoToIntermission() = 0;
+
+ // Setup counts for m_iNumTerrorist, m_iNumCT, m_iNumSpawnableTerrorist, m_iNumSpawnableCT, etc.
+ virtual void InitializePlayerCounts(int &NumAliveTerrorist, int &NumAliveCT, int &NumDeadTerrorist, int &NumDeadCT) = 0;
+
+ virtual void BalanceTeams() = 0;
+ virtual void SwapAllPlayers() = 0;
+ virtual void UpdateTeamScores() = 0;
+ virtual void EndRoundMessage(const char *sentence, int event) = 0;
+ virtual void SetAccountRules(RewardRules rules, int amount) = 0;
+ virtual RewardAccount GetAccountRules(RewardRules rules) const = 0;
+
+ // BOMB MAP FUNCTIONS
+ virtual BOOL IsThereABomber() = 0;
+ virtual BOOL IsThereABomb() = 0;
+ virtual TeamName SelectDefaultTeam() = 0;
+
+ virtual bool HasRoundTimeExpired() = 0;
+ virtual bool IsBombPlanted() = 0;
+
+public:
+ bool ShouldSkipShowMenu() const { return m_bSkipShowMenu; }
+ void MarkShowMenuSkipped() { m_bSkipShowMenu = false; }
+
+ bool ShouldSkipSpawn() const { return m_bSkipSpawn; }
+ void MarkSpawnSkipped() { m_bSkipSpawn = false; }
+
+ float GetRoundRemainingTime() const { return m_iRoundTimeSecs - gpGlobals->time + m_fRoundStartTime; }
+ float GetRoundRemainingTimeReal() const { return m_iRoundTimeSecs - gpGlobals->time + m_fRoundStartTimeReal; }
+ float GetTimeLeft() const { return m_flTimeLimit - gpGlobals->time; }
+ bool IsMatchStarted() { return (m_flRestartRoundTime != 0.0f || m_fCareerRoundMenuTime != 0.0f || m_fCareerMatchMenuTime != 0.0f); }
+
+ void TerminateRound(float tmDelay, int iWinStatus);
+
+public:
+ CVoiceGameMgr m_VoiceGameMgr;
+ float m_flRestartRoundTime; // The global time when the round is supposed to end, if this is not 0 (deprecated name m_fTeamCount)
+ float m_flCheckWinConditions;
+ float m_fRoundStartTime; // Time round has started (deprecated name m_fRoundCount)
+ int m_iRoundTime; // (From mp_roundtime) - How many seconds long this round is.
+ int m_iRoundTimeSecs;
+ int m_iIntroRoundTime; // (From mp_freezetime) - How many seconds long the intro round (when players are frozen) is.
+ float m_fRoundStartTimeReal; // The global time when the intro round ends and the real one starts
+ // wrote the original "m_flRoundTime" comment for this variable).
+ int m_iAccountTerrorist;
+ int m_iAccountCT;
+ int m_iNumTerrorist; // The number of terrorists on the team (this is generated at the end of a round)
+ int m_iNumCT; // The number of CTs on the team (this is generated at the end of a round)
+ int m_iNumSpawnableTerrorist;
+ int m_iNumSpawnableCT;
+ int m_iSpawnPointCount_Terrorist; // Number of Terrorist spawn points
+ int m_iSpawnPointCount_CT; // Number of CT spawn points
+ int m_iHostagesRescued;
+ int m_iHostagesTouched;
+ int m_iRoundWinStatus; // 1 == CT's won last round, 2 == Terrorists did, 3 == Draw, no winner
+
+ short m_iNumCTWins;
+ short m_iNumTerroristWins;
+
+ bool m_bTargetBombed; // whether or not the bomb has been bombed
+ bool m_bBombDefused; // whether or not the bomb has been defused
+
+ bool m_bMapHasBombTarget;
+ bool m_bMapHasBombZone;
+ bool m_bMapHasBuyZone;
+ bool m_bMapHasRescueZone;
+ bool m_bMapHasEscapeZone;
+
+ BOOL m_bMapHasVIPSafetyZone; // TRUE = has VIP safety zone, FALSE = does not have VIP safetyzone
+ BOOL m_bMapHasCameras;
+ int m_iC4Timer;
+ int m_iC4Guy; // The current Terrorist who has the C4.
+ int m_iLoserBonus; // the amount of money the losing team gets. This scales up as they lose more rounds in a row
+ int m_iNumConsecutiveCTLoses; // the number of rounds the CTs have lost in a row.
+ int m_iNumConsecutiveTerroristLoses; // the number of rounds the Terrorists have lost in a row.
+
+ float m_fMaxIdlePeriod; // For the idle kick functionality. This is tha max amount of time that the player has to be idle before being kicked
+
+ int m_iLimitTeams;
+ bool m_bLevelInitialized;
+ bool m_bRoundTerminating;
+ bool m_bCompleteReset; // Set to TRUE to have the scores reset next time round restarts
+ float m_flRequiredEscapeRatio;
+ int m_iNumEscapers;
+ int m_iHaveEscaped;
+ bool m_bCTCantBuy;
+ bool m_bTCantBuy; // Who can and can't buy.
+ float m_flBombRadius;
+ int m_iConsecutiveVIP;
+ int m_iTotalGunCount;
+ int m_iTotalGrenadeCount;
+ int m_iTotalArmourCount;
+ int m_iUnBalancedRounds; // keeps track of the # of consecutive rounds that have gone by where one team outnumbers the other team by more than 2
+ int m_iNumEscapeRounds; // keeps track of the # of consecutive rounds of escape played.. Teams will be swapped after 8 rounds
+ int m_iMapVotes[MAX_VOTE_MAPS];
+ int m_iLastPick;
+ int m_iMaxMapTime;
+ int m_iMaxRounds;
+ int m_iTotalRoundsPlayed;
+ int m_iMaxRoundsWon;
+ int m_iStoredSpectValue;
+ float m_flForceCameraValue;
+ float m_flForceChaseCamValue;
+ float m_flFadeToBlackValue;
+ CBasePlayer *m_pVIP;
+ CBasePlayer *m_pVIPQueue[MAX_VIP_QUEUES];
+ float m_flIntermissionEndTime;
+ float m_flIntermissionStartTime;
+ BOOL m_iEndIntermissionButtonHit;
+ float m_tmNextPeriodicThink;
+ bool m_bGameStarted; // TRUE = the game commencing when there is at least one CT and T, FALSE = scoring will not start until both teams have players (deprecated name m_bFirstConnected)
+ bool m_bInCareerGame;
+ float m_fCareerRoundMenuTime;
+ int m_iCareerMatchWins;
+ int m_iRoundWinDifference;
+ float m_fCareerMatchMenuTime;
+ bool m_bSkipSpawn;
+
+ // custom
+ bool m_bSkipShowMenu;
+ bool m_bNeededPlayers;
+ float m_flEscapeRatio;
+ float m_flTimeLimit;
+ float m_flGameStartTime;
+};
+
+typedef struct mapcycle_item_s
+{
+ struct mapcycle_item_s *next;
+ char mapname[32];
+ int minplayers;
+ int maxplayers;
+ char rulebuffer[MAX_RULE_BUFFER];
+
+} mapcycle_item_t;
+
+typedef struct mapcycle_s
+{
+ struct mapcycle_item_s *items;
+ struct mapcycle_item_s *next_item;
+
+} mapcycle_t;
+
+class CCStrikeGameMgrHelper: public IVoiceGameMgrHelper {
+public:
+ virtual bool CanPlayerHearPlayer(CBasePlayer *pListener, CBasePlayer *pSender) = 0;
+};
+
+extern CGameRules *g_pGameRules;
+
+// Gets us at the CS game rules
+inline CHalfLifeMultiplay *CSGameRules()
+{
+ return static_cast(g_pGameRules);
+}
+
+inline void CHalfLifeMultiplay::TerminateRound(float tmDelay, int iWinStatus)
+{
+ m_iRoundWinStatus = iWinStatus;
+ m_flRestartRoundTime = gpGlobals->time + tmDelay;
+ m_bRoundTerminating = true;
+}
diff --git a/dep/hlsdk/dlls/gib.h b/dep/hlsdk/dlls/gib.h
new file mode 100644
index 0000000..dc5be9a
--- /dev/null
+++ b/dep/hlsdk/dlls/gib.h
@@ -0,0 +1,39 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class CGib: public CBaseEntity {
+public:
+ virtual int ObjectCaps() = 0;
+public:
+ int m_bloodColor;
+ int m_cBloodDecals;
+ int m_material;
+ float m_lifeTime;
+};
diff --git a/dep/hlsdk/dlls/h_battery.h b/dep/hlsdk/dlls/h_battery.h
new file mode 100644
index 0000000..987ffcb
--- /dev/null
+++ b/dep/hlsdk/dlls/h_battery.h
@@ -0,0 +1,46 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class CRecharge: public CBaseToggle {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ float m_flNextCharge;
+ int m_iReactivate;
+ int m_iJuice;
+ int m_iOn;
+ float m_flSoundTime;
+};
diff --git a/dep/hlsdk/dlls/h_cycler.h b/dep/hlsdk/dlls/h_cycler.h
new file mode 100644
index 0000000..dc917f0
--- /dev/null
+++ b/dep/hlsdk/dlls/h_cycler.h
@@ -0,0 +1,105 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class CCycler: public CBaseMonster {
+public:
+ virtual void Spawn() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+
+ // Don't treat as a live target
+ virtual BOOL IsAlive() = 0;
+ virtual void Think() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ int m_animate;
+};
+
+// We should get rid of all the other cyclers and replace them with this.
+class CGenericCycler: public CCycler {
+public:
+ virtual void Spawn() = 0;
+};
+
+// Probe droid imported for tech demo compatibility
+class CCyclerProbe: public CCycler {
+public:
+ virtual void Spawn() = 0;
+};
+
+class CCyclerSprite: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Restart() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+ virtual void Think() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ inline int ShouldAnimate() { return (m_animate && m_maxFrame > 1.0f); }
+public:
+ int m_animate;
+ float m_lastTime;
+ float m_maxFrame;
+ int m_renderfx;
+ int m_rendermode;
+ float m_renderamt;
+ vec3_t m_rendercolor;
+};
+
+class CWeaponCycler: public CBasePlayerWeapon {
+public:
+ virtual void Spawn() = 0;
+ virtual int GetItemInfo(ItemInfo *p) = 0;
+ virtual BOOL Deploy() = 0;
+ virtual void Holster(int skiplocal = 0) = 0;
+ virtual int iItemSlot() = 0;
+ virtual void PrimaryAttack() = 0;
+ virtual void SecondaryAttack() = 0;
+public:
+ int m_iszModel;
+ int m_iModel;
+};
+
+// Flaming Wreakage
+class CWreckage: public CBaseMonster {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Think() = 0;
+public:
+ int m_flStartTime;
+};
diff --git a/dep/hlsdk/dlls/healthkit.h b/dep/hlsdk/dlls/healthkit.h
new file mode 100644
index 0000000..643ec6f
--- /dev/null
+++ b/dep/hlsdk/dlls/healthkit.h
@@ -0,0 +1,53 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class CHealthKit: public CItem {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
+
+class CWallHealth: public CBaseToggle {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ float m_flNextCharge;
+ int m_iReactivate;
+ int m_iJuice;
+ int m_iOn;
+ float m_flSoundTime;
+};
diff --git a/dep/hlsdk/dlls/hintmessage.h b/dep/hlsdk/dlls/hintmessage.h
new file mode 100644
index 0000000..c166517
--- /dev/null
+++ b/dep/hlsdk/dlls/hintmessage.h
@@ -0,0 +1,82 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "utlvector.h"
+
+#define DHF_ROUND_STARTED BIT(1)
+#define DHF_HOSTAGE_SEEN_FAR BIT(2)
+#define DHF_HOSTAGE_SEEN_NEAR BIT(3)
+#define DHF_HOSTAGE_USED BIT(4)
+#define DHF_HOSTAGE_INJURED BIT(5)
+#define DHF_HOSTAGE_KILLED BIT(6)
+#define DHF_FRIEND_SEEN BIT(7)
+#define DHF_ENEMY_SEEN BIT(8)
+#define DHF_FRIEND_INJURED BIT(9)
+#define DHF_FRIEND_KILLED BIT(10)
+#define DHF_ENEMY_KILLED BIT(11)
+#define DHF_BOMB_RETRIEVED BIT(12)
+#define DHF_AMMO_EXHAUSTED BIT(15)
+#define DHF_IN_TARGET_ZONE BIT(16)
+#define DHF_IN_RESCUE_ZONE BIT(17)
+#define DHF_IN_ESCAPE_ZONE BIT(18)
+#define DHF_IN_VIPSAFETY_ZONE BIT(19)
+#define DHF_NIGHTVISION BIT(20)
+#define DHF_HOSTAGE_CTMOVE BIT(21)
+#define DHF_SPEC_DUCK BIT(22)
+
+#define DHM_ROUND_CLEAR (DHF_ROUND_STARTED | DHF_HOSTAGE_KILLED | DHF_FRIEND_KILLED | DHF_BOMB_RETRIEVED)
+#define DHM_CONNECT_CLEAR (DHF_HOSTAGE_SEEN_FAR | DHF_HOSTAGE_SEEN_NEAR | DHF_HOSTAGE_USED | DHF_HOSTAGE_INJURED | DHF_FRIEND_SEEN | DHF_ENEMY_SEEN | DHF_FRIEND_INJURED | DHF_ENEMY_KILLED | DHF_AMMO_EXHAUSTED | DHF_IN_TARGET_ZONE | DHF_IN_RESCUE_ZONE | DHF_IN_ESCAPE_ZONE | DHF_IN_VIPSAFETY_ZONE | DHF_HOSTAGE_CTMOVE | DHF_SPEC_DUCK)
+
+class CHintMessage {
+public:
+ CHintMessage(const char *hintString, bool isHint, CUtlVector *args, float duration);
+ ~CHintMessage();
+public:
+ float GetDuration() const { return m_duration; }
+ void Send(CBaseEntity *client);
+
+private:
+ const char *m_hintString;
+ bool m_isHint;
+ CUtlVector m_args;
+ float m_duration;
+};
+
+class CHintMessageQueue {
+public:
+ void Reset();
+ void Update(CBaseEntity *client);
+ bool AddMessage(const char *message, float duration, bool isHint, CUtlVector *args);
+ bool IsEmpty() const { return m_messages.Count() == 0; }
+
+private:
+ float m_tmMessageEnd;
+ CUtlVector m_messages;
+};
diff --git a/dep/hlsdk/dlls/hookchains.h b/dep/hlsdk/dlls/hookchains.h
new file mode 100644
index 0000000..7ea472a
--- /dev/null
+++ b/dep/hlsdk/dlls/hookchains.h
@@ -0,0 +1,121 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+
+template
+class IHookChain {
+protected:
+ virtual ~IHookChain() {}
+
+public:
+ virtual t_ret callNext(t_args... args) = 0;
+ virtual t_ret callOriginal(t_args... args) = 0;
+};
+
+template
+class IHookChainClass {
+protected:
+ virtual ~IHookChainClass() {}
+
+public:
+ virtual t_ret callNext(t_class *, t_args... args) = 0;
+ virtual t_ret callOriginal(t_class *, t_args... args) = 0;
+};
+
+template
+class IVoidHookChain
+{
+protected:
+ virtual ~IVoidHookChain() {}
+
+public:
+ virtual void callNext(t_args... args) = 0;
+ virtual void callOriginal(t_args... args) = 0;
+};
+
+template
+class IVoidHookChainClass
+{
+protected:
+ virtual ~IVoidHookChainClass() {}
+
+public:
+ virtual void callNext(t_class *, t_args... args) = 0;
+ virtual void callOriginal(t_class *, t_args... args) = 0;
+};
+
+// Specifies priorities for hooks call order in the chain.
+// For equal priorities first registered hook will be called first.
+enum HookChainPriority
+{
+ HC_PRIORITY_UNINTERRUPTABLE = 255, // Hook will be called before other hooks.
+ HC_PRIORITY_HIGH = 192, // Hook will be called before hooks with default priority.
+ HC_PRIORITY_DEFAULT = 128, // Default hook call priority.
+ HC_PRIORITY_MEDIUM = 64, // Hook will be called after hooks with default priority.
+ HC_PRIORITY_LOW = 0, // Hook will be called after all other hooks.
+};
+
+// Hook chain registry(for hooks [un]registration)
+template
+class IHookChainRegistry {
+public:
+ typedef t_ret(*hookfunc_t)(IHookChain*, t_args...);
+
+ virtual void registerHook(hookfunc_t hook, int priority = HC_PRIORITY_DEFAULT) = 0;
+ virtual void unregisterHook(hookfunc_t hook) = 0;
+};
+
+// Hook chain registry(for hooks [un]registration)
+template
+class IHookChainRegistryClass {
+public:
+ typedef t_ret(*hookfunc_t)(IHookChainClass*, t_class *, t_args...);
+
+ virtual void registerHook(hookfunc_t hook, int priority = HC_PRIORITY_DEFAULT) = 0;
+ virtual void unregisterHook(hookfunc_t hook) = 0;
+};
+
+// Hook chain registry(for hooks [un]registration)
+template
+class IVoidHookChainRegistry {
+public:
+ typedef void(*hookfunc_t)(IVoidHookChain*, t_args...);
+
+ virtual void registerHook(hookfunc_t hook, int priority = HC_PRIORITY_DEFAULT) = 0;
+ virtual void unregisterHook(hookfunc_t hook) = 0;
+};
+
+// Hook chain registry(for hooks [un]registration)
+template
+class IVoidHookChainRegistryClass {
+public:
+ typedef void(*hookfunc_t)(IVoidHookChainClass*, t_class *, t_args...);
+
+ virtual void registerHook(hookfunc_t hook, int priority = HC_PRIORITY_DEFAULT) = 0;
+ virtual void unregisterHook(hookfunc_t hook) = 0;
+};
diff --git a/dep/hlsdk/dlls/hostage/hostage.h b/dep/hlsdk/dlls/hostage/hostage.h
new file mode 100644
index 0000000..a0be24c
--- /dev/null
+++ b/dep/hlsdk/dlls/hostage/hostage.h
@@ -0,0 +1,233 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+
+#define MAX_NODES 100
+#define MAX_HOSTAGES 12
+#define MAX_HOSTAGES_NAV 20
+
+#define HOSTAGE_STEPSIZE 26.0f
+#define HOSTAGE_STEPSIZE_DEFAULT 18.0f
+
+#define VEC_HOSTAGE_VIEW Vector(0, 0, 12)
+#define VEC_HOSTAGE_HULL_MIN Vector(-10, -10, 0)
+#define VEC_HOSTAGE_HULL_MAX Vector(10, 10, 62)
+
+#define VEC_HOSTAGE_CROUCH Vector(10, 10, 30)
+#define RESCUE_HOSTAGES_RADIUS 256.0f // rescue zones from legacy info_*
+
+class CHostage;
+class CLocalNav;
+class CHostageImprov;
+class CHostageManager;
+
+enum HostageChatterType
+{
+ HOSTAGE_CHATTER_START_FOLLOW = 0,
+ HOSTAGE_CHATTER_STOP_FOLLOW,
+ HOSTAGE_CHATTER_INTIMIDATED,
+ HOSTAGE_CHATTER_PAIN,
+ HOSTAGE_CHATTER_SCARED_OF_GUNFIRE,
+ HOSTAGE_CHATTER_SCARED_OF_MURDER,
+ HOSTAGE_CHATTER_LOOK_OUT,
+ HOSTAGE_CHATTER_PLEASE_RESCUE_ME,
+ HOSTAGE_CHATTER_SEE_RESCUE_ZONE,
+ HOSTAGE_CHATTER_IMPATIENT_FOR_RESCUE,
+ HOSTAGE_CHATTER_CTS_WIN ,
+ HOSTAGE_CHATTER_TERRORISTS_WIN,
+ HOSTAGE_CHATTER_RESCUED,
+ HOSTAGE_CHATTER_WARN_NEARBY,
+ HOSTAGE_CHATTER_WARN_SPOTTED,
+ HOSTAGE_CHATTER_CALL_TO_RESCUER,
+ HOSTAGE_CHATTER_RETREAT,
+ HOSTAGE_CHATTER_COUGH,
+ HOSTAGE_CHATTER_BLINDED,
+ HOSTAGE_CHATTER_SAW_HE_GRENADE,
+ HOSTAGE_CHATTER_DEATH_CRY,
+ NUM_HOSTAGE_CHATTER_TYPES,
+};
+
+// Improved the hostages from CZero
+#include "hostage/hostage_improv.h"
+
+extern CHostageManager *g_pHostages;
+extern int g_iHostageNumber;
+
+// A Counter-Strike Hostage Simple
+class CHostage: public CBaseMonster {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual int ObjectCaps() = 0; // make hostage "useable"
+ virtual int Classify() = 0;
+ virtual void TraceAttack(entvars_t *pevAttacker, float flDamage, Vector vecDir, TraceResult *ptr, int bitsDamageType) = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+ virtual int BloodColor() = 0;
+ virtual void Touch(CBaseEntity *pOther) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ int GetActivity() { return m_Activity; }
+
+ // queries
+ bool IsFollowingSomeone() { return IsFollowing(); }
+ CBaseEntity *GetLeader() // return our leader, or NULL
+ {
+ if (m_improv != NULL)
+ {
+ return m_improv->GetFollowLeader();
+ }
+
+ return m_hTargetEnt;
+ }
+ bool IsFollowing(const CBaseEntity *entity = NULL)
+ {
+ if (m_improv != NULL)
+ {
+ return m_improv->IsFollowing();
+ }
+
+ if (entity == NULL && m_hTargetEnt == NULL || (entity != NULL && m_hTargetEnt != entity))
+ return false;
+
+ if (m_State != FOLLOW)
+ return false;
+
+ return true;
+ }
+ bool IsValid() const { return (pev->takedamage == DAMAGE_YES); }
+ bool IsDead() const { return (pev->deadflag == DEAD_DEAD); }
+ bool IsAtHome() const { return (pev->origin - m_vStart).IsLengthGreaterThan(20) != true; }
+ const Vector *GetHomePosition() const { return &m_vStart; }
+public:
+ int m_Activity;
+ BOOL m_bTouched;
+ BOOL m_bRescueMe;
+ float m_flFlinchTime;
+ float m_flNextChange;
+ float m_flMarkPosition;
+ int m_iModel;
+ int m_iSkin;
+ float m_flNextRadarTime;
+ enum state { FOLLOW, STAND, DUCK, SCARED, IDLE, FOLLOWPATH }
+ m_State;
+ Vector m_vStart;
+ Vector m_vStartAngles;
+ Vector m_vPathToFollow[20];
+ int m_iWaypoint;
+ CBasePlayer *m_target;
+ CLocalNav *m_LocalNav;
+ int nTargetNode;
+ Vector vecNodes[MAX_NODES];
+ EHANDLE m_hStoppedTargetEnt;
+ float m_flNextFullThink;
+ float m_flPathCheckInterval;
+ float m_flLastPathCheck;
+ int m_nPathNodes;
+ BOOL m_fHasPath;
+ float m_flPathAcquired;
+ Vector m_vOldPos;
+ int m_iHostageIndex;
+ BOOL m_bStuck;
+ float m_flStuckTime;
+ CHostageImprov *m_improv;
+
+ enum ModelType { REGULAR_GUY, OLD_GUY, BLACK_GUY, GOOFY_GUY }
+ m_whichModel;
+};
+
+class SimpleChatter {
+public:
+ struct SoundFile
+ {
+ char *filename;
+ float duration;
+ };
+
+ struct ChatterSet
+ {
+ SoundFile file[32];
+ int count;
+ int index;
+ bool needsShuffle;
+ };
+private:
+ ChatterSet m_chatter[21];
+};
+
+class CHostageManager {
+public:
+ SimpleChatter *GetChatter()
+ {
+ return &m_chatter;
+ }
+ // Iterate over all active hostages in the game, invoking functor on each.
+ // If functor returns false, stop iteration and return false.
+ template
+ inline bool ForEachHostage(Functor &func) const
+ {
+ for (int i = 0; i < m_hostageCount; i++)
+ {
+ CHostage *hostage = m_hostage[i];
+
+ if (hostage == NULL || hostage->pev->deadflag == DEAD_DEAD)
+ continue;
+
+ if (func(hostage) == false)
+ return false;
+ }
+
+ return true;
+ }
+ inline CHostage *GetClosestHostage(const Vector &pos, float *resultRange = NULL)
+ {
+ float range;
+ float closeRange = 1e8f;
+ CHostage *close = NULL;
+
+ for (int i = 0; i < m_hostageCount; i++)
+ {
+ range = (m_hostage[i]->pev->origin - pos).Length();
+
+ if (range < closeRange)
+ {
+ closeRange = range;
+ close = m_hostage[i];
+ }
+ }
+
+ if (resultRange)
+ *resultRange = closeRange;
+
+ return close;
+ }
+
+private:
+ CHostage *m_hostage[MAX_HOSTAGES];
+ int m_hostageCount;
+ SimpleChatter m_chatter;
+};
diff --git a/dep/hlsdk/dlls/hostage/hostage_improv.h b/dep/hlsdk/dlls/hostage/hostage_improv.h
new file mode 100644
index 0000000..9c1784d
--- /dev/null
+++ b/dep/hlsdk/dlls/hostage/hostage_improv.h
@@ -0,0 +1,334 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "hostage/hostage.h"
+#include "hostage/hostage_states.h"
+
+class CHostage;
+enum HostageChatterType;
+
+// A Counter-Strike Hostage improved
+class CHostageImprov: public CImprov {
+public:
+ virtual ~CHostageImprov() {};
+
+ // invoked when an improv reaches its MoveTo goal
+ virtual void OnMoveToSuccess(const Vector &goal) = 0;
+
+ // invoked when an improv fails to reach a MoveTo goal
+ virtual void OnMoveToFailure(const Vector &goal, MoveToFailureType reason) = 0;
+ virtual void OnInjury(float amount) = 0;
+ virtual bool IsAlive() const = 0;
+ virtual void MoveTo(const Vector &goal) = 0;
+ virtual void LookAt(const Vector &target) = 0;
+ virtual void ClearLookAt() = 0;
+ virtual void FaceTo(const Vector &goal) = 0;
+ virtual void ClearFaceTo() = 0;
+ virtual bool IsAtMoveGoal(float error = 20.0f) const = 0;
+ virtual bool HasLookAt() const = 0;
+ virtual bool HasFaceTo() const = 0;
+ virtual bool IsAtFaceGoal() const = 0;
+ virtual bool IsFriendInTheWay(const Vector &goalPos) const = 0;
+ virtual bool IsFriendInTheWay(CBaseEntity *myFriend, const Vector &goalPos) const = 0;
+ virtual void MoveForward() = 0;
+ virtual void MoveBackward() = 0;
+ virtual void StrafeLeft() = 0;
+ virtual void StrafeRight() = 0;
+
+ #define HOSTAGE_MUST_JUMP true
+ virtual bool Jump() = 0;
+
+ virtual void Crouch() = 0;
+ virtual void StandUp() = 0;
+ virtual void TrackPath(const Vector &pathGoal, float deltaT) = 0; // move along path by following "pathGoal"
+ virtual void StartLadder(const CNavLadder *ladder, NavTraverseType how, const Vector *approachPos, const Vector *departPos) = 0;
+ virtual bool TraverseLadder(const CNavLadder *ladder, NavTraverseType how, const Vector *approachPos, const Vector *departPos, float deltaT) = 0;
+ virtual bool GetSimpleGroundHeightWithFloor(const Vector *pos, float *height, Vector *normal = NULL) = 0;
+ virtual void Run() = 0;
+ virtual void Walk() = 0;
+ virtual void Stop() = 0;
+ virtual float GetMoveAngle() const = 0;
+ virtual float GetFaceAngle() const = 0;
+ virtual const Vector &GetFeet() const = 0;
+ virtual const Vector &GetCentroid() const = 0;
+ virtual const Vector &GetEyes() const = 0;
+ virtual bool IsRunning() const = 0;
+ virtual bool IsWalking() const = 0;
+ virtual bool IsStopped() const = 0;
+ virtual bool IsCrouching() const = 0;
+ virtual bool IsJumping() const = 0;
+ virtual bool IsUsingLadder() const = 0;
+ virtual bool IsOnGround() const = 0;
+ virtual bool IsMoving() const = 0;
+ virtual bool CanRun() const = 0;
+ virtual bool CanCrouch() const = 0;
+ virtual bool CanJump() const = 0;
+ virtual bool IsVisible(const Vector &pos, bool testFOV = false) const = 0; // return true if hostage can see position
+ virtual bool IsPlayerLookingAtMe(CBasePlayer *other, float cosTolerance = 0.95f) const = 0;
+ virtual CBasePlayer *IsAnyPlayerLookingAtMe(int team = 0, float cosTolerance = 0.95f) const = 0;
+ virtual CBasePlayer *GetClosestPlayerByTravelDistance(int team = 0, float *range = NULL) const = 0;
+ virtual CNavArea *GetLastKnownArea() const = 0;
+ virtual void OnUpdate(float deltaT) = 0;
+ virtual void OnUpkeep(float deltaT) = 0;
+ virtual void OnReset() = 0;
+ virtual void OnGameEvent(GameEventType event, CBaseEntity *entity = NULL, CBaseEntity *other = NULL) = 0;
+ virtual void OnTouch(CBaseEntity *other) = 0; // in contact with "other"
+public:
+ enum MoveType { Stopped, Walking, Running };
+ enum ScareType { NERVOUS, SCARED, TERRIFIED };
+
+ const Vector &GetKnownGoodPosition() const { return m_knownGoodPos; }
+ void ApplyForce(Vector force) { m_vel.x += force.x; m_vel.y += force.y; } // apply a force to the hostage
+ const Vector GetActualVelocity() const { return m_actualVel; }
+ void SetMoveLimit(MoveType limit) { m_moveLimit = limit; }
+ MoveType GetMoveLimit() const { return m_moveLimit; }
+ CNavPath *GetPath() { return &m_path; }
+
+ // hostage states
+ // stand idle
+ void Idle() { m_behavior.SetState(&m_idleState); }
+ bool IsIdle() const { return m_behavior.IsState(&m_idleState); }
+
+ // begin following "leader"
+ void Follow(CBasePlayer *leader) { m_followState.SetLeader(leader); m_behavior.SetState(&m_followState); }
+ bool IsFollowing(const CBaseEntity *leader = NULL) const { return m_behavior.IsState(&m_followState); }
+
+ // Escape
+ void Escape() { m_behavior.SetState(&m_escapeState); }
+ bool IsEscaping() const { return m_behavior.IsState(&m_escapeState); }
+
+ // Retreat
+ void Retreat() { m_behavior.SetState(&m_retreatState); }
+ bool IsRetreating() const { return m_behavior.IsState(&m_retreatState); }
+
+ CBaseEntity *GetFollowLeader() const { return m_followState.GetLeader(); }
+ ScareType GetScareIntensity() const { return m_scareIntensity; }
+ bool IsIgnoringTerrorists() const { return m_ignoreTerroristTimer.IsElapsed(); }
+ float GetAggression() const { return m_aggression; }
+ bool IsTalking() const { return m_talkingTimer.IsElapsed(); }
+ CHostage *GetEntity() const { return m_hostage; }
+ void SetMoveAngle(float angle) { m_moveAngle = angle; }
+public:
+ CountdownTimer m_coughTimer;
+ CountdownTimer m_grenadeTimer;
+private:
+ CHostage *m_hostage;
+ CNavArea *m_lastKnownArea; // last area we were in
+ mutable Vector m_centroid;
+ mutable Vector m_eye;
+ HostageStateMachine m_behavior;
+ HostageIdleState m_idleState;
+ HostageEscapeState m_escapeState;
+ HostageRetreatState m_retreatState;
+ HostageFollowState m_followState;
+ HostageAnimateState m_animateState;
+ bool m_didFidget;
+ float m_aggression;
+ IntervalTimer m_lastSawCT;
+ IntervalTimer m_lastSawT;
+ CountdownTimer m_checkNearbyTerroristTimer;
+ bool m_isTerroristNearby;
+ CountdownTimer m_nearbyTerroristTimer;
+ CountdownTimer m_scaredTimer;
+ ScareType m_scareIntensity;
+ CountdownTimer m_ignoreTerroristTimer;
+ CountdownTimer m_blinkTimer;
+ char m_blinkCounter;
+ IntervalTimer m_lastInjuryTimer;
+ IntervalTimer m_lastNoiseTimer;
+ mutable CountdownTimer m_avoidFriendTimer;
+ mutable bool m_isFriendInTheWay;
+ CountdownTimer m_chatterTimer;
+ bool m_isDelayedChatterPending;
+ CountdownTimer m_delayedChatterTimer;
+ HostageChatterType m_delayedChatterType;
+ bool m_delayedChatterMustSpeak;
+ CountdownTimer m_talkingTimer;
+ unsigned int m_moveFlags;
+ Vector2D m_vel;
+ Vector m_actualVel;
+ Vector m_moveGoal;
+ Vector m_knownGoodPos;
+ bool m_hasKnownGoodPos;
+ Vector m_priorKnownGoodPos;
+ bool m_hasPriorKnownGoodPos;
+ CountdownTimer m_priorKnownGoodPosTimer;
+ IntervalTimer m_collisionTimer;
+ Vector m_viewGoal;
+ bool m_isLookingAt;
+ Vector m_faceGoal;
+ bool m_isFacingTo;
+ CNavPath m_path; // current path to follow
+ CNavPathFollower m_follower;
+ Vector m_lastPosition;
+ MoveType m_moveType;
+ MoveType m_moveLimit;
+ bool m_isCrouching; // true if hostage is crouching
+ CountdownTimer m_minCrouchTimer;
+ float m_moveAngle;
+ NavRelativeDirType m_wiggleDirection;
+
+ CountdownTimer m_wiggleTimer; // for wiggling
+ CountdownTimer m_wiggleJumpTimer;
+ CountdownTimer m_inhibitObstacleAvoidance;
+ CountdownTimer m_jumpTimer; // if zero, we can jump
+
+ bool m_hasJumped;
+ bool m_hasJumpedIntoAir;
+ Vector m_jumpTarget;
+ CountdownTimer m_clearPathTimer;
+ bool m_traversingLadder;
+ EntityHandle m_visiblePlayer[MAX_CLIENTS];
+ int m_visiblePlayerCount;
+ CountdownTimer m_visionTimer;
+};
+
+class CheckWayFunctor {
+public:
+ CheckWayFunctor(const CHostageImprov *me, const Vector &goalPos)
+ {
+ m_me = me;
+ m_goalPos = goalPos;
+ m_blocker = NULL;
+ }
+ bool operator()(CHostage *them)
+ {
+ if (((CBaseMonster *)them)->IsAlive() && m_me->IsFriendInTheWay((CBaseEntity *)them, m_goalPos))
+ {
+ m_blocker = them;
+ return false;
+ }
+
+ return true;
+ }
+
+ const CHostageImprov *m_me;
+ Vector m_goalPos;
+ CHostage *m_blocker;
+};
+
+// Functor used with NavAreaBuildPath() for building Hostage paths.
+// Once we hook up crouching and ladders, this can be removed and ShortestPathCost() can be used instead.
+class HostagePathCost {
+public:
+ float operator()(CNavArea *area, CNavArea *fromArea, const CNavLadder *ladder)
+ {
+ if (fromArea == NULL)
+ {
+ // first area in path, no cost
+ return 0.0f;
+ }
+ else
+ {
+ // compute distance travelled along path so far
+ float dist;
+
+ if (ladder != NULL)
+ {
+ const float ladderCost = 10.0f;
+ return ladder->m_length * ladderCost + fromArea->GetCostSoFar();
+ }
+ else
+ {
+ dist = (*area->GetCenter() - *fromArea->GetCenter()).Length();
+ }
+
+ float cost = dist + fromArea->GetCostSoFar();
+
+ // if this is a "crouch" area, add penalty
+ if (area->GetAttributes() & NAV_CROUCH)
+ {
+ const float crouchPenalty = 10.0f;
+ cost += crouchPenalty * dist;
+ }
+
+ // if this is a "jump" area, add penalty
+ if (area->GetAttributes() & NAV_JUMP)
+ {
+ const float jumpPenalty = 10.0f;
+ cost += jumpPenalty * dist;
+ }
+
+ return cost;
+ }
+ }
+};
+
+class KeepPersonalSpace {
+public:
+ KeepPersonalSpace(CHostageImprov *improv)
+ {
+ m_improv = improv;
+ m_velDir = improv->GetActualVelocity();
+ m_speed = m_velDir.NormalizeInPlace();
+ }
+ bool operator()(CBaseEntity *entity)
+ {
+ const float space = 1.0f;
+ Vector to;
+ float range;
+
+ if (entity == reinterpret_cast(m_improv->GetEntity()))
+ return true;
+
+ if (entity->IsPlayer() && !entity->IsAlive())
+ return true;
+
+ to = entity->pev->origin - m_improv->GetCentroid();
+ range = to.NormalizeInPlace();
+
+ CBasePlayer *player = static_cast(entity);
+
+ const float spring = 50.0f;
+ const float damper = 1.0f;
+
+ if (range >= spring)
+ return true;
+
+ const float cosTolerance = 0.8f;
+ if (entity->IsPlayer() && player->m_iTeam == CT && !m_improv->IsFollowing() && m_improv->IsPlayerLookingAtMe(player, cosTolerance))
+ return true;
+
+ const float minSpace = (spring - range);
+ float ds = -minSpace;
+
+ m_improv->ApplyForce(to * ds);
+
+ const float force = 0.1f;
+ m_improv->ApplyForce(m_speed * -force * m_velDir);
+
+ return true;
+ }
+
+private:
+ CHostageImprov *m_improv;
+ Vector m_velDir;
+ float m_speed;
+};
diff --git a/dep/hlsdk/dlls/hostage/hostage_localnav.h b/dep/hlsdk/dlls/hostage/hostage_localnav.h
new file mode 100644
index 0000000..5a40e6f
--- /dev/null
+++ b/dep/hlsdk/dlls/hostage/hostage_localnav.h
@@ -0,0 +1,58 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+
+#define NODE_INVALID_EMPTY -1
+
+#define PATH_TRAVERSABLE_EMPTY 0
+#define PATH_TRAVERSABLE_SLOPE 1
+#define PATH_TRAVERSABLE_STEP 2
+#define PATH_TRAVERSABLE_STEPJUMPABLE 3
+
+typedef int node_index_t;
+
+typedef struct localnode_s
+{
+ Vector vecLoc;
+ int offsetX;
+ int offsetY;
+ byte bDepth;
+ BOOL fSearched;
+ node_index_t nindexParent;
+
+} localnode_t;
+
+class CLocalNav {
+private:
+ CHostage *m_pOwner;
+ edict_t *m_pTargetEnt;
+ BOOL m_fTargetEntHit;
+ localnode_t *m_nodeArr;
+ node_index_t m_nindexAvailableNode;
+ Vector m_vecStartingLoc;
+};
diff --git a/dep/hlsdk/dlls/hostage/hostage_states.h b/dep/hlsdk/dlls/hostage/hostage_states.h
new file mode 100644
index 0000000..95c5854
--- /dev/null
+++ b/dep/hlsdk/dlls/hostage/hostage_states.h
@@ -0,0 +1,204 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class CHostageImprov;
+
+class HostageState: public SimpleState, public IImprovEvent {
+public:
+ virtual ~HostageState() {}
+ virtual void UpdateStationaryAnimation(CHostageImprov *improv) {}
+};
+
+class HostageStateMachine: public SimpleStateMachine, public IImprovEvent {
+public:
+ virtual void OnMoveToSuccess(const Vector &goal) {}
+ virtual void OnMoveToFailure(const Vector &goal, MoveToFailureType reason) {}
+ virtual void OnInjury(float amount) {}
+};
+
+class HostageIdleState: public HostageState {
+public:
+ virtual ~HostageIdleState() {}
+ virtual void OnEnter(CHostageImprov *improv) {}
+ virtual void OnUpdate(CHostageImprov *improv) {}
+ virtual void OnExit(CHostageImprov *improv) {}
+ virtual const char *GetName() const { return "Idle"; }
+ virtual void UpdateStationaryAnimation(CHostageImprov *improv) {}
+ virtual void OnMoveToSuccess(const Vector &goal) {}
+ virtual void OnMoveToFailure(const Vector &goal, MoveToFailureType reason) {}
+ virtual void OnInjury(float amount = -1.0f) {}
+private:
+ CountdownTimer m_waveTimer;
+ CountdownTimer m_fleeTimer;
+ CountdownTimer m_disagreeTimer;
+ CountdownTimer m_escapeTimer;
+ CountdownTimer m_askTimer;
+ IntervalTimer m_intimidatedTimer;
+ CountdownTimer m_pleadTimer;
+
+ enum
+ {
+ NotMoving = 0,
+ Moving,
+ MoveDone,
+ MoveFailed,
+ } m_moveState;
+
+ bool m_mustFlee;
+};
+
+class HostageEscapeToCoverState: public HostageState {
+public:
+ virtual ~HostageEscapeToCoverState() {}
+ virtual void OnEnter(CHostageImprov *improv) {}
+ virtual void OnUpdate(CHostageImprov *improv) {}
+ virtual void OnExit(CHostageImprov *improv) {}
+ virtual const char *GetName() const { return "Escape:ToCover"; }
+ virtual void OnMoveToFailure(const Vector &goal, MoveToFailureType reason) {}
+public:
+ void SetRescueGoal(const Vector &rescueGoal) { m_rescueGoal = rescueGoal; }
+
+private:
+ Vector m_rescueGoal;
+ Vector m_spot;
+ bool m_canEscape;
+};
+
+class HostageEscapeLookAroundState: public HostageState {
+public:
+ virtual ~HostageEscapeLookAroundState() {}
+ virtual void OnEnter(CHostageImprov *improv) {}
+ virtual void OnUpdate(CHostageImprov *improv) {}
+ virtual void OnExit(CHostageImprov *improv) {}
+ virtual const char *GetName() const { return "Escape:LookAround"; }
+
+private:
+ CountdownTimer m_timer;
+};
+
+class HostageEscapeState: public HostageState {
+public:
+ virtual ~HostageEscapeState() {}
+ virtual void OnEnter(CHostageImprov *improv) {}
+ virtual void OnUpdate(CHostageImprov *improv) {}
+ virtual void OnExit(CHostageImprov *improv) {}
+ virtual const char *GetName() const { return "Escape"; }
+ virtual void OnMoveToFailure(const Vector &goal, MoveToFailureType reason) {}
+public:
+ void ToCover() { m_behavior.SetState(&m_toCoverState); }
+ void LookAround() { m_behavior.SetState(&m_lookAroundState); }
+private:
+ HostageEscapeToCoverState m_toCoverState;
+ HostageEscapeLookAroundState m_lookAroundState;
+ HostageStateMachine m_behavior;
+ bool m_canEscape;
+ CountdownTimer m_runTimer;
+};
+
+class HostageRetreatState: public HostageState {
+public:
+ virtual ~HostageRetreatState() {}
+ virtual void OnEnter(CHostageImprov *improv) {}
+ virtual void OnUpdate(CHostageImprov *improv) {}
+ virtual void OnExit(CHostageImprov *improv) {}
+ virtual const char *GetName() const { return "Retreat"; }
+};
+
+class HostageFollowState: public HostageState {
+public:
+ virtual ~HostageFollowState() {}
+ virtual void OnEnter(CHostageImprov *improv) {}
+ virtual void OnUpdate(CHostageImprov *improv) {}
+ virtual void OnExit(CHostageImprov *improv) {}
+ virtual const char *GetName() const { return "Follow"; }
+ virtual void UpdateStationaryAnimation(CHostageImprov *improv) {}
+public:
+ void SetLeader(CBasePlayer *leader) { m_leader = leader; }
+ CBasePlayer *GetLeader() const { return m_leader; }
+private:
+ mutable EntityHandle m_leader;
+ Vector m_lastLeaderPos;
+ bool m_isWaiting;
+ float m_stopRange;
+ CountdownTimer m_makeWayTimer;
+ CountdownTimer m_impatientTimer;
+ CountdownTimer m_repathTimer;
+ bool m_isWaitingForFriend;
+ CountdownTimer m_waitForFriendTimer;
+};
+
+class HostageAnimateState: public HostageState {
+public:
+ virtual ~HostageAnimateState() {}
+ virtual void OnEnter(CHostageImprov *improv) {}
+ virtual void OnUpdate(CHostageImprov *improv) {}
+ virtual void OnExit(CHostageImprov *improv) {}
+ virtual const char *GetName() const { return "Animate"; }
+public:
+ struct SeqInfo
+ {
+ int seqID;
+ float holdTime;
+ float rate;
+ };
+
+ enum PerformanceType
+ {
+ None = 0,
+ Walk,
+ Run,
+ Jump,
+ Fall,
+ Crouch,
+ CrouchWalk,
+ Calm,
+ Anxious,
+ Afraid,
+ Sitting,
+ GettingUp,
+ Waving,
+ LookingAround,
+ Disagreeing,
+ Flinching,
+ };
+
+ bool IsBusy() const { return (m_sequenceCount > 0); }
+ int GetCurrentSequenceID() { return m_currentSequence; }
+ PerformanceType GetPerformance() const { return m_performance; }
+ void SetPerformance(PerformanceType performance) { m_performance = performance; }
+private:
+ enum { MAX_SEQUENCES = 8 };
+ struct SeqInfo m_sequence[MAX_SEQUENCES];
+ int m_sequenceCount;
+ int m_currentSequence;
+ enum PerformanceType m_performance;
+ bool m_isHolding;
+ CountdownTimer m_holdTimer;
+};
diff --git a/dep/hlsdk/dlls/items.h b/dep/hlsdk/dlls/items.h
new file mode 100644
index 0000000..614ce05
--- /dev/null
+++ b/dep/hlsdk/dlls/items.h
@@ -0,0 +1,156 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+enum ItemRestType
+{
+ ITEM_TYPE_BUYING, // When a player is buying items
+ ITEM_TYPE_TOUCHED, // When the player touches with a weaponbox or armoury_entity
+ ITEM_TYPE_EQUIPPED // When an entity game_player_equip gives item to player or default items on player spawn
+};
+
+// Constant items
+#define ITEM_ID_ANTIDOTE 2
+#define ITEM_ID_SECURITY 3
+
+enum ItemID
+{
+ ITEM_NONE = -1,
+ ITEM_SHIELDGUN,
+ ITEM_P228,
+ ITEM_GLOCK,
+ ITEM_SCOUT,
+ ITEM_HEGRENADE,
+ ITEM_XM1014,
+ ITEM_C4,
+ ITEM_MAC10,
+ ITEM_AUG,
+ ITEM_SMOKEGRENADE,
+ ITEM_ELITE,
+ ITEM_FIVESEVEN,
+ ITEM_UMP45,
+ ITEM_SG550,
+ ITEM_GALIL,
+ ITEM_FAMAS,
+ ITEM_USP,
+ ITEM_GLOCK18,
+ ITEM_AWP,
+ ITEM_MP5N,
+ ITEM_M249,
+ ITEM_M3,
+ ITEM_M4A1,
+ ITEM_TMP,
+ ITEM_G3SG1,
+ ITEM_FLASHBANG,
+ ITEM_DEAGLE,
+ ITEM_SG552,
+ ITEM_AK47,
+ ITEM_KNIFE,
+ ITEM_P90,
+ ITEM_NVG,
+ ITEM_DEFUSEKIT,
+ ITEM_KEVLAR,
+ ITEM_ASSAULT,
+ ITEM_LONGJUMP,
+ ITEM_SODACAN,
+ ITEM_HEALTHKIT,
+ ITEM_ANTIDOTE,
+ ITEM_BATTERY
+};
+
+class CItem: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual CBaseEntity *Respawn() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
+
+class CWorldItem: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+public:
+ int m_iType;
+};
+
+class CItemSuit: public CItem {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
+
+class CItemBattery: public CItem {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
+
+class CItemAntidote: public CItem {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
+
+class CItemSecurity: public CItem {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
+
+class CItemLongJump: public CItem {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
+
+class CItemKevlar: public CItem {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
+
+class CItemAssaultSuit: public CItem {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
+
+class CItemThighPack: public CItem {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual BOOL MyTouch(CBasePlayer *pPlayer) = 0;
+};
diff --git a/dep/hlsdk/dlls/lights.h b/dep/hlsdk/dlls/lights.h
new file mode 100644
index 0000000..b35eed1
--- /dev/null
+++ b/dep/hlsdk/dlls/lights.h
@@ -0,0 +1,51 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#define SF_LIGHT_START_OFF BIT(0)
+
+class CLight: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Restart() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+private:
+ int m_iStyle;
+ int m_iszPattern;
+ BOOL m_iStartedOff;
+};
+
+class CEnvLight: public CLight {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+};
diff --git a/dep/hlsdk/dlls/mapinfo.h b/dep/hlsdk/dlls/mapinfo.h
new file mode 100644
index 0000000..acefce0
--- /dev/null
+++ b/dep/hlsdk/dlls/mapinfo.h
@@ -0,0 +1,43 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+const float MAX_BOMB_RADIUS = 2048.0f;
+
+class CMapInfo: public CPointEntity
+{
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual void UpdateOnRemove() = 0;
+
+public:
+ InfoMapBuyParam m_iBuyingStatus;
+ float m_flBombRadius;
+};
diff --git a/dep/hlsdk/dlls/maprules.h b/dep/hlsdk/dlls/maprules.h
new file mode 100644
index 0000000..fd663fe
--- /dev/null
+++ b/dep/hlsdk/dlls/maprules.h
@@ -0,0 +1,224 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class CRuleEntity: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+public:
+ void SetMaster(int iszMaster) { m_iszMaster = iszMaster; }
+
+private:
+ string_t m_iszMaster;
+};
+
+// Base class for all rule "point" entities (not brushes)
+class CRulePointEntity: public CRuleEntity {
+public:
+ virtual void Spawn() = 0;
+};
+
+// Base class for all rule "brush" entities (not brushes)
+// Default behavior is to set up like a trigger, invisible, but keep the model for volume testing
+class CRuleBrushEntity: public CRuleEntity {
+public:
+ virtual void Spawn() = 0;
+};
+
+#define SF_SCORE_NEGATIVE BIT(0) // Allow negative scores
+#define SF_SCORE_TEAM BIT(1) // Award points to team in teamplay
+
+// Award points to player / team
+// Points +/- total
+class CGameScore: public CRulePointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ int Points() const { return int(pev->frags); }
+ bool AllowNegativeScore() { return (pev->spawnflags & SF_SCORE_NEGATIVE) == SF_SCORE_NEGATIVE; }
+ bool AwardToTeam() const { return (pev->spawnflags & SF_SCORE_TEAM) == SF_SCORE_TEAM; }
+ void SetPoints(int points) { pev->frags = points; }
+};
+
+// Ends the game in Multiplayer
+class CGameEnd: public CRulePointEntity {
+public:
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+};
+
+#define SF_ENVTEXT_ALLPLAYERS BIT(0) // Message will be displayed to all players instead of just the activator.
+
+// NON-Localized HUD Message (use env_message to display a titles.txt message)
+class CGameText: public CRulePointEntity {
+public:
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+
+public:
+ bool MessageToAll() const { return (pev->spawnflags & SF_ENVTEXT_ALLPLAYERS) == SF_ENVTEXT_ALLPLAYERS; }
+ void MessageSet(const char *pMessage) { pev->message = ALLOC_STRING(pMessage); }
+ const char *MessageGet() const { return STRING(pev->message); }
+
+private:
+ hudtextparms_t m_textParms;
+};
+
+#define SF_TEAMMASTER_FIREONCE BIT(0) // Remove on Fire
+#define SF_TEAMMASTER_ANYTEAM BIT(1) // Any team until set? -- Any team can use this until the team is set (otherwise no teams can use it)
+
+// "Masters" like multisource, but based on the team of the activator
+// Only allows mastered entity to fire if the team matches my team
+//
+// team index (pulled from server team list "mp_teamlist"
+class CGameTeamMaster: public CRulePointEntity {
+public:
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual BOOL IsTriggered(CBaseEntity *pActivator) = 0;
+ virtual const char *TeamID() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ bool RemoveOnFire() const { return (pev->spawnflags & SF_TEAMMASTER_FIREONCE) == SF_TEAMMASTER_FIREONCE; }
+ bool AnyTeam() const { return (pev->spawnflags & SF_TEAMMASTER_ANYTEAM) == SF_TEAMMASTER_ANYTEAM; }
+
+public:
+ int m_teamIndex;
+ USE_TYPE m_triggerType;
+};
+
+#define SF_TEAMSET_FIREONCE BIT(0) // Remove entity after firing.
+#define SF_TEAMSET_CLEARTEAM BIT(1) // Clear team -- Sets the team to "NONE" instead of activator
+
+// Changes the team of the entity it targets to the activator's team
+class CGameTeamSet: public CRulePointEntity {
+public:
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ bool RemoveOnFire() const { return (pev->spawnflags & SF_TEAMSET_FIREONCE) == SF_TEAMSET_FIREONCE; }
+ bool ShouldClearTeam() const { return (pev->spawnflags & SF_TEAMSET_CLEARTEAM) == SF_TEAMSET_CLEARTEAM; }
+};
+
+// Players in the zone fire my target when I'm fired
+// Needs master?
+class CGamePlayerZone: public CRuleBrushEntity {
+public:
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+private:
+ string_t m_iszInTarget;
+ string_t m_iszOutTarget;
+ string_t m_iszInCount;
+ string_t m_iszOutCount;
+};
+
+#define SF_PKILL_FIREONCE BIT(0) // Remove entity after firing.
+
+// Damages the player who fires it
+class CGamePlayerHurt: public CRulePointEntity {
+public:
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ bool RemoveOnFire() const { return (pev->spawnflags & SF_PKILL_FIREONCE) == SF_PKILL_FIREONCE; }
+};
+
+#define SF_GAMECOUNT_FIREONCE BIT(0) // Remove entity after firing.
+#define SF_GAMECOUNT_RESET BIT(1) // Reset entity Initial value after fired.
+#define SF_GAMECOUNT_OVER_LIMIT BIT(2) // Fire a target when initial value is higher than limit value.
+
+// Counts events and fires target
+class CGameCounter: public CRulePointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ bool RemoveOnFire() const { return (pev->spawnflags & SF_GAMECOUNT_FIREONCE) == SF_GAMECOUNT_FIREONCE; }
+ bool ResetOnFire() const { return (pev->spawnflags & SF_GAMECOUNT_RESET) == SF_GAMECOUNT_RESET; }
+
+ void CountUp() { pev->frags++; }
+ void CountDown() { pev->frags--; }
+ void ResetCount() { pev->frags = pev->dmg; }
+
+ int CountValue() const { return int(pev->frags); }
+ int LimitValue() const { return int(pev->health); }
+ bool HitLimit() const { return ((pev->spawnflags & SF_GAMECOUNT_OVER_LIMIT) == SF_GAMECOUNT_OVER_LIMIT) ? (CountValue() >= LimitValue()) : (CountValue() == LimitValue()); }
+
+private:
+ void SetCountValue(int value) { pev->frags = value; }
+ void SetInitialValue(int value) { pev->dmg = value; }
+};
+
+#define SF_GAMECOUNTSET_FIREONCE BIT(0) // Remove entity after firing.
+
+// Sets the counter's value
+class CGameCounterSet: public CRulePointEntity {
+public:
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ bool RemoveOnFire() const { return (pev->spawnflags & SF_GAMECOUNTSET_FIREONCE) == SF_GAMECOUNTSET_FIREONCE; }
+};
+
+#define MAX_EQUIP 32
+#define SF_PLAYEREQUIP_USEONLY BIT(0) // If set, the game_player_equip entity will not equip respawning players,
+ // but only react to direct triggering, equipping its activator. This makes its master obsolete.
+
+// Sets the default player equipment
+class CGamePlayerEquip: public CRulePointEntity {
+public:
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual void Touch(CBaseEntity *pOther) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+public:
+ bool UseOnly() const { return (pev->spawnflags & SF_PLAYEREQUIP_USEONLY) == SF_PLAYEREQUIP_USEONLY; }
+public:
+ string_t m_weaponNames[MAX_EQUIP];
+ int m_weaponCount[MAX_EQUIP];
+};
+
+#define SF_PTEAM_FIREONCE BIT(0) // Remove entity after firing.
+#define SF_PTEAM_KILL BIT(1) // Kill Player.
+#define SF_PTEAM_GIB BIT(2) // Gib Player.
+
+// Changes the team of the player who fired it
+class CGamePlayerTeam: public CRulePointEntity {
+public:
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+private:
+ bool RemoveOnFire() const { return (pev->spawnflags & SF_PTEAM_FIREONCE) == SF_PTEAM_FIREONCE; }
+ bool ShouldKillPlayer() const { return (pev->spawnflags & SF_PTEAM_KILL) == SF_PTEAM_KILL; }
+ bool ShouldGibPlayer() const { return (pev->spawnflags & SF_PTEAM_GIB) == SF_PTEAM_GIB; }
+};
diff --git a/dep/hlsdk/dlls/monsterevent.h b/dep/hlsdk/dlls/monsterevent.h
new file mode 100644
index 0000000..2c7b1b0
--- /dev/null
+++ b/dep/hlsdk/dlls/monsterevent.h
@@ -0,0 +1,45 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+typedef struct MonsterEvent_s
+{
+ int event;
+ char *options;
+
+} MonsterEvent_t;
+
+#define EVENT_SPECIFIC 0
+#define EVENT_SCRIPTED 1000
+#define EVENT_SHARED 2000
+#define EVENT_CLIENT 5000
+
+#define MONSTER_EVENT_BODYDROP_LIGHT 2001
+#define MONSTER_EVENT_BODYDROP_HEAVY 2002
+#define MONSTER_EVENT_SWISHSOUND 2010
diff --git a/dep/hlsdk/dlls/monsters.h b/dep/hlsdk/dlls/monsters.h
new file mode 100644
index 0000000..b4495e2
--- /dev/null
+++ b/dep/hlsdk/dlls/monsters.h
@@ -0,0 +1,84 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "skill.h"
+
+#define R_AL -2 // (ALLY) pals. Good alternative to R_NO when applicable.
+#define R_FR -1 // (FEAR) will run
+#define R_NO 0 // (NO RELATIONSHIP) disregard
+#define R_DL 1 // (DISLIKE) will attack
+#define R_HT 2 // (HATE) will attack this character instead of any visible DISLIKEd characters
+#define R_NM 3 // (NEMESIS) A monster Will ALWAYS attack its nemsis, no matter what
+
+#define SF_MONSTER_WAIT_TILL_SEEN BIT(0) // spawnflag that makes monsters wait until player can see them before attacking.
+#define SF_MONSTER_GAG BIT(1) // no idle noises from this monster
+#define SF_MONSTER_HITMONSTERCLIP BIT(2)
+#define SF_MONSTER_PRISONER BIT(4) // monster won't attack anyone, no one will attacks him.
+
+#define SF_MONSTER_WAIT_FOR_SCRIPT BIT(7) //spawnflag that makes monsters wait to check for attacking until the script is done or they've been attacked
+#define SF_MONSTER_PREDISASTER BIT(8) //this is a predisaster scientist or barney. Influences how they speak.
+#define SF_MONSTER_FADECORPSE BIT(9) // Fade out corpse after death
+#define SF_MONSTER_FALL_TO_GROUND BIT(31)
+
+// These bits represent the monster's memory
+#define MEMORY_CLEAR 0
+#define bits_MEMORY_PROVOKED BIT(0) // right now only used for houndeyes.
+#define bits_MEMORY_INCOVER BIT(1) // monster knows it is in a covered position.
+#define bits_MEMORY_SUSPICIOUS BIT(2) // Ally is suspicious of the player, and will move to provoked more easily
+#define bits_MEMORY_PATH_FINISHED BIT(3) // Finished monster path (just used by big momma for now)
+#define bits_MEMORY_ON_PATH BIT(4) // Moving on a path
+#define bits_MEMORY_MOVE_FAILED BIT(5) // Movement has already failed
+#define bits_MEMORY_FLINCHED BIT(6) // Has already flinched
+#define bits_MEMORY_KILLED BIT(7) // HACKHACK -- remember that I've already called my Killed()
+#define bits_MEMORY_CUSTOM4 BIT(28) // Monster-specific memory
+#define bits_MEMORY_CUSTOM3 BIT(29) // Monster-specific memory
+#define bits_MEMORY_CUSTOM2 BIT(30) // Monster-specific memory
+#define bits_MEMORY_CUSTOM1 BIT(31) // Monster-specific memory
+
+// MoveToOrigin stuff
+#define MOVE_START_TURN_DIST 64 // when this far away from moveGoal, start turning to face next goal
+#define MOVE_STUCK_DIST 32 // if a monster can't step this far, it is stuck.
+
+#define MOVE_NORMAL 0 // normal move in the direction monster is facing
+#define MOVE_STRAFE 1 // moves in direction specified, no matter which way monster is facing
+
+enum HitBoxGroup
+{
+ HITGROUP_GENERIC = 0,
+ HITGROUP_HEAD,
+ HITGROUP_CHEST,
+ HITGROUP_STOMACH,
+ HITGROUP_LEFTARM,
+ HITGROUP_RIGHTARM,
+ HITGROUP_LEFTLEG,
+ HITGROUP_RIGHTLEG,
+ HITGROUP_SHIELD,
+ NUM_HITGROUPS,
+};
diff --git a/dep/hlsdk/dlls/mortar.h b/dep/hlsdk/dlls/mortar.h
new file mode 100644
index 0000000..85e4d60
--- /dev/null
+++ b/dep/hlsdk/dlls/mortar.h
@@ -0,0 +1,56 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+class CFuncMortarField: public CBaseToggle {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+
+ // Bmodels don't go across transitions
+ virtual int ObjectCaps() = 0;
+public:
+ int m_iszXController;
+ int m_iszYController;
+ float m_flSpread;
+ float m_flDelay;
+ int m_iCount;
+ int m_fControl;
+};
+
+class CMortar: public CGrenade {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+public:
+ int m_spriteTexture;
+};
diff --git a/dep/hlsdk/dlls/observer.h b/dep/hlsdk/dlls/observer.h
new file mode 100644
index 0000000..937c86d
--- /dev/null
+++ b/dep/hlsdk/dlls/observer.h
@@ -0,0 +1,32 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+
+#define CAMERA_MODE_SPEC_ANYONE 0
+#define CAMERA_MODE_SPEC_ONLY_TEAM 1
+#define CAMERA_MODE_SPEC_ONLY_FRIST_PERSON 2
diff --git a/dep/hlsdk/dlls/pathcorner.h b/dep/hlsdk/dlls/pathcorner.h
new file mode 100644
index 0000000..4499bfd
--- /dev/null
+++ b/dep/hlsdk/dlls/pathcorner.h
@@ -0,0 +1,44 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#define SF_CORNER_WAITFORTRIG BIT(0)
+#define SF_CORNER_TELEPORT BIT(1)
+#define SF_CORNER_FIREONCE BIT(2)
+
+class CPathCorner: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual float GetDelay() = 0;
+private:
+ float m_flWait;
+};
diff --git a/dep/hlsdk/dlls/plats.h b/dep/hlsdk/dlls/plats.h
new file mode 100644
index 0000000..3d318b7
--- /dev/null
+++ b/dep/hlsdk/dlls/plats.h
@@ -0,0 +1,190 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#define SF_PLAT_TOGGLE BIT(0) // The lift is no more automatically called from top and activated by stepping on it.
+ // It required trigger to do so.
+
+class CBasePlatTrain: public CBaseToggle {
+public:
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+
+ // This is done to fix spawn flag collisions between this class and a derived class
+ virtual BOOL IsTogglePlat() = 0;
+public:
+ byte m_bMoveSnd;
+ byte m_bStopSnd;
+ float m_volume;
+};
+
+class CFuncPlat: public CBasePlatTrain {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Blocked(CBaseEntity *pOther) = 0;
+ virtual void GoUp() = 0;
+ virtual void GoDown() = 0;
+ virtual void HitTop() = 0;
+ virtual void HitBottom() = 0;
+};
+
+class CPlatTrigger: public CBaseEntity {
+public:
+ virtual int ObjectCaps() = 0;
+ virtual void Touch(CBaseEntity *pOther) = 0;
+public:
+ CFuncPlat *m_pPlatform;
+};
+
+class CFuncPlatRot: public CFuncPlat {
+public:
+ virtual void Spawn() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void GoUp() = 0;
+ virtual void GoDown() = 0;
+ virtual void HitTop() = 0;
+ virtual void HitBottom() = 0;
+public:
+ Vector m_end;
+ Vector m_start;
+};
+
+#define SF_TRAIN_WAIT_RETRIGGER BIT(0)
+#define SF_TRAIN_START_ON BIT(2) // Train is initially moving
+#define SF_TRAIN_PASSABLE BIT(3) // Train is not solid -- used to make water trains
+
+class CFuncTrain: public CBasePlatTrain {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void Restart() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void Activate() = 0;
+ virtual void OverrideReset() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+ virtual void Blocked(CBaseEntity *pOther) = 0;
+public:
+ Vector m_vStartPosition;
+ entvars_t *m_pevFirstTarget;
+ entvars_t *m_pevCurrentTarget;
+ int m_sounds;
+ BOOL m_activated;
+};
+
+// This class defines the volume of space that the player must stand in to control the train
+class CFuncTrainControls: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual int ObjectCaps() = 0;
+};
+
+#define SF_TRACK_ACTIVATETRAIN BIT(0)
+#define SF_TRACK_RELINK BIT(1)
+#define SF_TRACK_ROTMOVE BIT(2)
+#define SF_TRACK_STARTBOTTOM BIT(3)
+#define SF_TRACK_DONT_MOVE BIT(4)
+
+enum TRAIN_CODE { TRAIN_SAFE, TRAIN_BLOCKING, TRAIN_FOLLOWING };
+
+// This entity is a rotating/moving platform that will carry a train to a new track.
+// It must be larger in X-Y planar area than the train, since it must contain the
+// train within these dimensions in order to operate when the train is near it.
+class CFuncTrackChange: public CFuncPlatRot {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual void OverrideReset() = 0;
+ virtual void Touch(CBaseEntity *pOther) = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+ virtual BOOL IsTogglePlat() = 0;
+ virtual void GoUp() = 0;
+ virtual void GoDown() = 0;
+ virtual void HitTop() = 0;
+ virtual void HitBottom() = 0;
+ virtual void UpdateAutoTargets(int toggleState) = 0;
+
+public:
+ void DisableUse() { m_use = 0; }
+ void EnableUse() { m_use = 1; }
+
+ int UseEnabled() const { return m_use; }
+
+public:
+ static TYPEDESCRIPTION IMPL(m_SaveData)[9];
+
+ CPathTrack *m_trackTop;
+ CPathTrack *m_trackBottom;
+ CFuncTrackTrain *m_train;
+
+ int m_trackTopName;
+ int m_trackBottomName;
+ int m_trainName;
+
+ TRAIN_CODE m_code;
+ int m_targetState;
+ int m_use;
+};
+
+class CFuncTrackAuto: public CFuncTrackChange {
+public:
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+ virtual void UpdateAutoTargets(int toggleState) = 0;
+};
+
+// pev->speed is the travel speed
+// pev->health is current health
+// pev->max_health is the amount to reset to each time it starts
+
+#define SF_GUNTARGET_START_ON BIT(0)
+
+class CGunTarget: public CBaseMonster {
+public:
+ virtual void Spawn() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual void Activate() = 0;
+ virtual int Classify() = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+ virtual int BloodColor() = 0;
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+ virtual Vector BodyTarget(const Vector &posSrc) = 0;
+private:
+ BOOL m_on;
+};
diff --git a/dep/hlsdk/dlls/player.h b/dep/hlsdk/dlls/player.h
new file mode 100644
index 0000000..042a191
--- /dev/null
+++ b/dep/hlsdk/dlls/player.h
@@ -0,0 +1,668 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#include "weapons.h"
+#include "pm_materials.h"
+#include "hintmessage.h"
+#include "unisignals.h"
+
+#define SOUND_FLASHLIGHT_ON "items/flashlight1.wav"
+#define SOUND_FLASHLIGHT_OFF "items/flashlight1.wav"
+
+const int MAX_PLAYER_NAME_LENGTH = 32;
+const int MAX_AUTOBUY_LENGTH = 256;
+const int MAX_REBUY_LENGTH = 256;
+
+const int MAX_RECENT_PATH = 20;
+const int MAX_HOSTAGE_ICON = 4; // the maximum number of icons of the hostages in the HUD
+
+const int MAX_SUIT_NOREPEAT = 32;
+const int MAX_SUIT_PLAYLIST = 4; // max of 4 suit sentences queued up at any time
+
+const int MAX_BUFFER_MENU = 175;
+const int MAX_BUFFER_MENU_BRIEFING = 50;
+
+const float SUIT_UPDATE_TIME = 3.5f;
+const float SUIT_FIRST_UPDATE_TIME = 0.1f;
+
+const float MAX_PLAYER_FATAL_FALL_SPEED = 1100.0f;
+const float MAX_PLAYER_SAFE_FALL_SPEED = 500.0f;
+const float MAX_PLAYER_USE_RADIUS = 64.0f;
+
+const float ARMOR_RATIO = 0.5f; // Armor Takes 50% of the damage
+const float ARMOR_BONUS = 0.5f; // Each Point of Armor is work 1/x points of health
+
+const float FLASH_DRAIN_TIME = 1.2f; // 100 units/3 minutes
+const float FLASH_CHARGE_TIME = 0.2f; // 100 units/20 seconds (seconds per unit)
+
+// damage per unit per second.
+const float DAMAGE_FOR_FALL_SPEED = 100.0f / (MAX_PLAYER_FATAL_FALL_SPEED - MAX_PLAYER_SAFE_FALL_SPEED);
+const float PLAYER_MIN_BOUNCE_SPEED = 350.0f;
+
+// won't punch player's screen/make scrape noise unless player falling at least this fast.
+const float PLAYER_FALL_PUNCH_THRESHHOLD = 250.0f;
+
+// Money blinks few of times on the freeze period
+// NOTE: It works for CZ
+const int MONEY_BLINK_AMOUNT = 30;
+
+// Player time based damage
+#define AIRTIME 12 // lung full of air lasts this many seconds
+#define PARALYZE_DURATION 2 // number of 2 second intervals to take damage
+#define PARALYZE_DAMAGE 1.0f // damage to take each 2 second interval
+
+#define NERVEGAS_DURATION 2
+#define NERVEGAS_DAMAGE 5.0f
+
+#define POISON_DURATION 5
+#define POISON_DAMAGE 2.0f
+
+#define RADIATION_DURATION 2
+#define RADIATION_DAMAGE 1.0f
+
+#define ACID_DURATION 2
+#define ACID_DAMAGE 5.0f
+
+#define SLOWBURN_DURATION 2
+#define SLOWBURN_DAMAGE 1.0f
+
+#define SLOWFREEZE_DURATION 2
+#define SLOWFREEZE_DAMAGE 1.0f
+
+// Player physics flags bits
+// CBasePlayer::m_afPhysicsFlags
+#define PFLAG_ONLADDER BIT(0)
+#define PFLAG_ONSWING BIT(0)
+#define PFLAG_ONTRAIN BIT(1)
+#define PFLAG_ONBARNACLE BIT(2)
+#define PFLAG_DUCKING BIT(3) // In the process of ducking, but not totally squatted yet
+#define PFLAG_USING BIT(4) // Using a continuous entity
+#define PFLAG_OBSERVER BIT(5) // Player is locked in stationary cam mode. Spectators can move, observers can't.
+
+#define TRAIN_OFF 0x00
+#define TRAIN_NEUTRAL 0x01
+#define TRAIN_SLOW 0x02
+#define TRAIN_MEDIUM 0x03
+#define TRAIN_FAST 0x04
+#define TRAIN_BACK 0x05
+
+#define TRAIN_ACTIVE 0x80
+#define TRAIN_NEW 0xc0
+
+const bool SUIT_GROUP = true;
+const bool SUIT_SENTENCE = false;
+
+const int SUIT_REPEAT_OK = 0;
+const int SUIT_NEXT_IN_30SEC = 30;
+const int SUIT_NEXT_IN_1MIN = 60;
+const int SUIT_NEXT_IN_5MIN = 300;
+const int SUIT_NEXT_IN_10MIN = 600;
+const int SUIT_NEXT_IN_30MIN = 1800;
+const int SUIT_NEXT_IN_1HOUR = 3600;
+
+const int MAX_TEAM_NAME_LENGTH = 16;
+
+const auto AUTOAIM_2DEGREES = 0.0348994967025;
+const auto AUTOAIM_5DEGREES = 0.08715574274766;
+const auto AUTOAIM_8DEGREES = 0.1391731009601;
+const auto AUTOAIM_10DEGREES = 0.1736481776669;
+
+// custom enum
+enum RewardType
+{
+ RT_NONE,
+ RT_ROUND_BONUS,
+ RT_PLAYER_RESET,
+ RT_PLAYER_JOIN,
+ RT_PLAYER_SPEC_JOIN,
+ RT_PLAYER_BOUGHT_SOMETHING,
+ RT_HOSTAGE_TOOK,
+ RT_HOSTAGE_RESCUED,
+ RT_HOSTAGE_DAMAGED,
+ RT_HOSTAGE_KILLED,
+ RT_TEAMMATES_KILLED,
+ RT_ENEMY_KILLED,
+ RT_INTO_GAME,
+ RT_VIP_KILLED,
+ RT_VIP_RESCUED_MYSELF
+};
+
+enum PLAYER_ANIM
+{
+ PLAYER_IDLE,
+ PLAYER_WALK,
+ PLAYER_JUMP,
+ PLAYER_SUPERJUMP,
+ PLAYER_DIE,
+ PLAYER_ATTACK1,
+ PLAYER_ATTACK2,
+ PLAYER_FLINCH,
+ PLAYER_LARGE_FLINCH,
+ PLAYER_RELOAD,
+ PLAYER_HOLDBOMB
+};
+
+enum _Menu
+{
+ Menu_OFF,
+ Menu_ChooseTeam,
+ Menu_IGChooseTeam,
+ Menu_ChooseAppearance,
+ Menu_Buy,
+ Menu_BuyPistol,
+ Menu_BuyRifle,
+ Menu_BuyMachineGun,
+ Menu_BuyShotgun,
+ Menu_BuySubMachineGun,
+ Menu_BuyItem,
+ Menu_Radio1,
+ Menu_Radio2,
+ Menu_Radio3,
+ Menu_ClientBuy
+};
+
+enum TeamName
+{
+ UNASSIGNED,
+ TERRORIST,
+ CT,
+ SPECTATOR,
+};
+
+enum ModelName
+{
+ MODEL_UNASSIGNED,
+ MODEL_URBAN,
+ MODEL_TERROR,
+ MODEL_LEET,
+ MODEL_ARCTIC,
+ MODEL_GSG9,
+ MODEL_GIGN,
+ MODEL_SAS,
+ MODEL_GUERILLA,
+ MODEL_VIP,
+ MODEL_MILITIA,
+ MODEL_SPETSNAZ,
+ MODEL_AUTO
+};
+
+enum JoinState
+{
+ JOINED,
+ SHOWLTEXT,
+ READINGLTEXT,
+ SHOWTEAMSELECT,
+ PICKINGTEAM,
+ GETINTOGAME
+};
+
+enum TrackCommands
+{
+ CMD_SAY = 0,
+ CMD_SAYTEAM,
+ CMD_FULLUPDATE,
+ CMD_VOTE,
+ CMD_VOTEMAP,
+ CMD_LISTMAPS,
+ CMD_LISTPLAYERS,
+ CMD_NIGHTVISION,
+ COMMANDS_TO_TRACK,
+};
+
+enum IgnoreChatMsg : int
+{
+ IGNOREMSG_NONE, // Nothing to do
+ IGNOREMSG_ENEMY, // To ignore any chat messages from the enemy
+ IGNOREMSG_TEAM // Same as IGNOREMSG_ENEMY but ignore teammates
+};
+
+struct RebuyStruct
+{
+ int m_primaryWeapon;
+ int m_primaryAmmo;
+ int m_secondaryWeapon;
+ int m_secondaryAmmo;
+ int m_heGrenade;
+ int m_flashbang;
+ int m_smokeGrenade;
+ int m_defuser;
+ int m_nightVision;
+ ArmorType m_armor;
+};
+
+enum ThrowDirection
+{
+ THROW_NONE,
+ THROW_FORWARD,
+ THROW_BACKWARD,
+ THROW_HITVEL,
+ THROW_BOMB,
+ THROW_GRENADE,
+ THROW_HITVEL_MINUS_AIRVEL
+};
+
+const float MAX_ID_RANGE = 2048.0f;
+const float MAX_SPEC_ID_RANGE = 8192.0f;
+const int MAX_SBAR_STRING = 128;
+
+const int SBAR_TARGETTYPE_TEAMMATE = 1;
+const int SBAR_TARGETTYPE_ENEMY = 2;
+const int SBAR_TARGETTYPE_HOSTAGE = 3;
+
+enum sbar_data
+{
+ SBAR_ID_TARGETTYPE = 1,
+ SBAR_ID_TARGETNAME,
+ SBAR_ID_TARGETHEALTH,
+ SBAR_END
+};
+
+enum MusicState { SILENT, CALM, INTENSE };
+
+class CCSPlayer;
+
+class CStripWeapons: public CPointEntity {
+public:
+ virtual void Use(CBaseEntity *pActivator, CBaseEntity *pCaller, USE_TYPE useType, float value) = 0;
+};
+
+// Multiplayer intermission spots.
+class CInfoIntermission: public CPointEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Think() = 0;
+};
+
+// Dead HEV suit prop
+class CDeadHEV: public CBaseMonster {
+public:
+ virtual void Spawn() = 0;
+ virtual void KeyValue(KeyValueData *pkvd) = 0;
+ virtual int Classify() = 0;
+public:
+ int m_iPose; // which sequence to display -- temporary, don't need to save
+ static char *m_szPoses[4];
+};
+
+class CSprayCan: public CBaseEntity {
+public:
+ virtual void Think() = 0;
+ virtual int ObjectCaps() = 0;
+};
+
+class CBloodSplat: public CBaseEntity {
+public:
+};
+
+class CBasePlayer: public CBaseMonster {
+public:
+ virtual void Spawn() = 0;
+ virtual void Precache() = 0;
+ virtual int Save(CSave &save) = 0;
+ virtual int Restore(CRestore &restore) = 0;
+ virtual int ObjectCaps() = 0;
+ virtual int Classify() = 0;
+ virtual void TraceAttack(entvars_t *pevAttacker, float flDamage, Vector vecDir, TraceResult *ptr, int bitsDamageType) = 0;
+ virtual BOOL TakeDamage(entvars_t *pevInflictor, entvars_t *pevAttacker, float flDamage, int bitsDamageType) = 0;
+ virtual BOOL TakeHealth(float flHealth, int bitsDamageType) = 0;
+ virtual void Killed(entvars_t *pevAttacker, int iGib) = 0;
+ virtual void AddPoints(int score, BOOL bAllowNegativeScore) = 0;
+ virtual void AddPointsToTeam(int score, BOOL bAllowNegativeScore) = 0;
+ virtual BOOL AddPlayerItem(CBasePlayerItem *pItem) = 0;
+ virtual BOOL RemovePlayerItem(CBasePlayerItem *pItem) = 0;
+ virtual int GiveAmmo(int iAmount, const char *szName, int iMax = -1) = 0;
+ virtual void StartSneaking() = 0;
+ virtual void UpdateOnRemove() = 0;
+ virtual BOOL IsSneaking() = 0;
+ virtual BOOL IsAlive() = 0;
+ virtual BOOL IsPlayer() = 0;
+ virtual BOOL IsNetClient() = 0;
+ virtual const char *TeamID() = 0;
+ virtual BOOL FBecomeProne() = 0;
+ virtual Vector BodyTarget(const Vector &posSrc) = 0;
+ virtual int Illumination() = 0;
+ virtual BOOL ShouldFadeOnDeath() = 0;
+ virtual void ResetMaxSpeed() = 0;
+ virtual void Jump() = 0;
+ virtual void Duck() = 0;
+ virtual void PreThink() = 0;
+ virtual void PostThink() = 0;
+ virtual Vector GetGunPosition() = 0;
+ virtual BOOL IsBot() = 0;
+ virtual void UpdateClientData() = 0;
+ virtual void ImpulseCommands() = 0;
+ virtual void RoundRespawn() = 0;
+ virtual Vector GetAutoaimVector(float flDelta) = 0;
+ virtual void Blind(float flUntilTime, float flHoldTime, float flFadeTime, int iAlpha) = 0;
+ virtual void OnTouchingWeapon(CWeaponBox *pWeapon) = 0;
+public:
+ static CBasePlayer *Instance(edict_t *pent) { return (CBasePlayer *)GET_PRIVATE(pent ? pent : ENT(0)); }
+ static CBasePlayer *Instance(entvars_t *pev) { return Instance(ENT(pev)); }
+ static CBasePlayer *Instance(int offset) { return Instance(ENT(offset)); }
+
+ int IsObserver() { return pev->iuser1; }
+ void SetWeaponAnimType(const char *szExtention) { strcpy(m_szAnimExtention, szExtention); }
+ bool IsProtectedByShield() { return m_bOwnsShield && m_bShieldDrawn; }
+ bool IsReloading() const;
+ bool IsBlind() const { return (m_blindUntilTime > gpGlobals->time); }
+ bool IsAutoFollowAllowed() const { return (gpGlobals->time > m_allowAutoFollowTime); }
+ void InhibitAutoFollow(float duration) { m_allowAutoFollowTime = gpGlobals->time + duration; }
+ void AllowAutoFollow() { m_allowAutoFollowTime = 0; }
+ void SetObserverAutoDirector(bool val) { m_bObserverAutoDirector = val; }
+ bool CanSwitchObserverModes() const { return m_canSwitchObserverModes; }
+ CCSPlayer *CSPlayer() const;
+
+ // templates
+ template
+ T *ForEachItem(int slot, const Functor &func)
+ {
+ auto item = m_rgpPlayerItems[ slot ];
+ while (item)
+ {
+ if (func(static_cast(item)))
+ return static_cast(item);
+
+ item = item->m_pNext;
+ }
+
+ return nullptr;
+ }
+
+ template
+ T *ForEachItem(const Functor &func)
+ {
+ for (auto item : m_rgpPlayerItems)
+ {
+ while (item)
+ {
+ if (func(static_cast(item)))
+ return static_cast(item);
+
+ item = item->m_pNext;
+ }
+ }
+
+ return nullptr;
+ }
+
+ template
+ T *ForEachItem(const char *pszItemName, const Functor &func)
+ {
+ if (!pszItemName) {
+ return nullptr;
+ }
+
+ for (auto item : m_rgpPlayerItems)
+ {
+ while (item)
+ {
+ if (FClassnameIs(pszItemName, STRING(item->pev->classname)) && func(static_cast(item))) {
+ return static_cast(item);
+ }
+
+ item = item->m_pNext;
+ }
+ }
+
+ return nullptr;
+ }
+
+public:
+ enum { MaxLocationLen = 32 };
+
+ int random_seed;
+ unsigned short m_usPlayerBleed;
+ EHANDLE m_hObserverTarget;
+ float m_flNextObserverInput;
+ int m_iObserverWeapon;
+ int m_iObserverC4State;
+ bool m_bObserverHasDefuser;
+ int m_iObserverLastMode;
+ float m_flFlinchTime;
+ float m_flAnimTime;
+ bool m_bHighDamage;
+ float m_flVelocityModifier;
+ int m_iLastZoom;
+ bool m_bResumeZoom;
+ float m_flEjectBrass;
+ ArmorType m_iKevlar;
+ bool m_bNotKilled;
+ TeamName m_iTeam;
+ int m_iAccount;
+ bool m_bHasPrimary;
+ float m_flDeathThrowTime;
+ int m_iThrowDirection;
+ float m_flLastTalk;
+ bool m_bJustConnected;
+ bool m_bContextHelp;
+ JoinState m_iJoiningState;
+ CBaseEntity *m_pIntroCamera;
+ float m_fIntroCamTime;
+ float m_fLastMovement;
+ bool m_bMissionBriefing;
+ bool m_bTeamChanged;
+ ModelName m_iModelName;
+ int m_iTeamKills;
+ IgnoreChatMsg m_iIgnoreGlobalChat;
+ bool m_bHasNightVision;
+ bool m_bNightVisionOn;
+ Vector m_vRecentPath[MAX_RECENT_PATH];
+ float m_flIdleCheckTime;
+ float m_flRadioTime;
+ int m_iRadioMessages;
+ bool m_bIgnoreRadio;
+ bool m_bHasC4;
+ bool m_bHasDefuser;
+ bool m_bKilledByBomb;
+ Vector m_vBlastVector;
+ bool m_bKilledByGrenade;
+ CHintMessageQueue m_hintMessageQueue;
+ int m_flDisplayHistory;
+ _Menu m_iMenu;
+ int m_iChaseTarget;
+ CBaseEntity *m_pChaseTarget;
+ float m_fCamSwitch;
+ bool m_bEscaped;
+ bool m_bIsVIP;
+ float m_tmNextRadarUpdate;
+ Vector m_vLastOrigin;
+ int m_iCurrentKickVote;
+ float m_flNextVoteTime;
+ bool m_bJustKilledTeammate;
+ int m_iHostagesKilled;
+ int m_iMapVote;
+ bool m_bCanShoot;
+ float m_flLastFired;
+ float m_flLastAttackedTeammate;
+ bool m_bHeadshotKilled;
+ bool m_bPunishedForTK;
+ bool m_bReceivesNoMoneyNextRound;
+ int m_iTimeCheckAllowed;
+ bool m_bHasChangedName;
+ char m_szNewName[MAX_PLAYER_NAME_LENGTH];
+ bool m_bIsDefusing;
+ float m_tmHandleSignals;
+ CUnifiedSignals m_signals;
+ edict_t *m_pentCurBombTarget;
+ int m_iPlayerSound;
+ int m_iTargetVolume;
+ int m_iWeaponVolume;
+ int m_iExtraSoundTypes;
+ int m_iWeaponFlash;
+ float m_flStopExtraSoundTime;
+ float m_flFlashLightTime;
+ int m_iFlashBattery;
+ int m_afButtonLast;
+ int m_afButtonPressed;
+ int m_afButtonReleased;
+ edict_t *m_pentSndLast;
+ float m_flSndRoomtype;
+ float m_flSndRange;
+ float m_flFallVelocity;
+ int m_rgItems[MAX_ITEMS];
+ int m_fNewAmmo;
+ unsigned int m_afPhysicsFlags;
+ float m_fNextSuicideTime;
+ float m_flTimeStepSound;
+ float m_flTimeWeaponIdle;
+ float m_flSwimTime;
+ float m_flDuckTime;
+ float m_flWallJumpTime;
+ float m_flSuitUpdate;
+ int m_rgSuitPlayList[MAX_SUIT_PLAYLIST];
+ int m_iSuitPlayNext;
+ int m_rgiSuitNoRepeat[MAX_SUIT_NOREPEAT];
+ float m_rgflSuitNoRepeatTime[MAX_SUIT_NOREPEAT];
+ int m_lastDamageAmount;
+ float m_tbdPrev;
+ float m_flgeigerRange;
+ float m_flgeigerDelay;
+ int m_igeigerRangePrev;
+ int m_iStepLeft;
+ char m_szTextureName[CBTEXTURENAMEMAX];
+ char m_chTextureType;
+ int m_idrowndmg;
+ int m_idrownrestored;
+ int m_bitsHUDDamage;
+ BOOL m_fInitHUD;
+ BOOL m_fGameHUDInitialized;
+ int m_iTrain;
+ BOOL m_fWeapon;
+ EHANDLE m_pTank;
+ float m_fDeadTime;
+ BOOL m_fNoPlayerSound;
+ BOOL m_fLongJump;
+ float m_tSneaking;
+ int m_iUpdateTime;
+ int m_iClientHealth;
+ int m_iClientBattery;
+ int m_iHideHUD;
+ int m_iClientHideHUD;
+ int m_iFOV;
+ int m_iClientFOV;
+ int m_iNumSpawns;
+ CBaseEntity *m_pObserver;
+ CBasePlayerItem *m_rgpPlayerItems[MAX_ITEM_TYPES];
+ CBasePlayerItem *m_pActiveItem;
+ CBasePlayerItem *m_pClientActiveItem;
+ CBasePlayerItem *m_pLastItem;
+ int m_rgAmmo[MAX_AMMO_SLOTS];
+ int m_rgAmmoLast[MAX_AMMO_SLOTS];
+ Vector m_vecAutoAim;
+ BOOL m_fOnTarget;
+ int m_iDeaths;
+ int m_izSBarState[SBAR_END];
+ float m_flNextSBarUpdateTime;
+ float m_flStatusBarDisappearDelay;
+ char m_SbarString0[MAX_SBAR_STRING];
+ int m_lastx;
+ int m_lasty;
+ int m_nCustomSprayFrames;
+ float m_flNextDecalTime;
+ char m_szTeamName[MAX_TEAM_NAME_LENGTH];
+ int m_modelIndexPlayer;
+ char m_szAnimExtention[32];
+ int m_iGaitsequence;
+ float m_flGaitframe;
+ float m_flGaityaw;
+ Vector m_prevgaitorigin;
+ float m_flPitch;
+ float m_flYaw;
+ float m_flGaitMovement;
+ int m_iAutoWepSwitch;
+ bool m_bVGUIMenus;
+ bool m_bShowHints;
+ bool m_bShieldDrawn;
+ bool m_bOwnsShield;
+ bool m_bWasFollowing;
+ float m_flNextFollowTime;
+ float m_flYawModifier;
+ float m_blindUntilTime;
+ float m_blindStartTime;
+ float m_blindHoldTime;
+ float m_blindFadeTime;
+ int m_blindAlpha;
+ float m_allowAutoFollowTime;
+ char m_autoBuyString[MAX_AUTOBUY_LENGTH];
+ char *m_rebuyString;
+ RebuyStruct m_rebuyStruct;
+ bool m_bIsInRebuy;
+ float m_flLastUpdateTime;
+ char m_lastLocation[MaxLocationLen];
+ float m_progressStart;
+ float m_progressEnd;
+ bool m_bObserverAutoDirector;
+ bool m_canSwitchObserverModes;
+ float m_heartBeatTime;
+ float m_intenseTimestamp;
+ float m_silentTimestamp;
+ MusicState m_musicState;
+ float m_flLastCommandTime[COMMANDS_TO_TRACK];
+};
+
+class CWShield: public CBaseEntity {
+public:
+ virtual void Spawn() = 0;
+ virtual void Touch(CBaseEntity *pOther) = 0;
+public:
+ void SetCantBePickedUpByUser(CBasePlayer *pPlayer, float time) { m_hEntToIgnoreTouchesFrom = pPlayer; m_flTimeToIgnoreTouches = gpGlobals->time + time; }
+public:
+ EntityHandle m_hEntToIgnoreTouchesFrom;
+ float m_flTimeToIgnoreTouches;
+};
+
+inline bool CBasePlayer::IsReloading() const
+{
+ CBasePlayerWeapon *weapon = static_cast(m_pActiveItem);
+ if (weapon && weapon->m_fInReload)
+ return true;
+
+ return false;
+}
+
+inline CCSPlayer *CBasePlayer::CSPlayer() const {
+ return reinterpret_cast(this->m_pEntity);
+}
+
+// returns a CBaseEntity pointer to a player by index. Only returns if the player is spawned and connected otherwise returns NULL
+// Index is 1 based
+inline CBasePlayer *UTIL_PlayerByIndex(int playerIndex)
+{
+ return (CBasePlayer *)GET_PRIVATE(INDEXENT(playerIndex));
+}
+
+inline CBasePlayer *UTIL_PlayerByIndexSafe(int playerIndex)
+{
+ CBasePlayer *player = nullptr;
+ if (likely(playerIndex > 0 && playerIndex <= gpGlobals->maxClients))
+ player = UTIL_PlayerByIndex(playerIndex);
+
+ return player;
+}
diff --git a/dep/hlsdk/dlls/qstring.h b/dep/hlsdk/dlls/qstring.h
new file mode 100644
index 0000000..d6e1799
--- /dev/null
+++ b/dep/hlsdk/dlls/qstring.h
@@ -0,0 +1,121 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+
+#pragma once
+
+#define QSTRING_DEFINE
+
+constexpr unsigned int iStringNull = {0};
+
+// Quake string (helper class)
+class QString final
+{
+public:
+ using qstring_t = unsigned int;
+
+ QString(): m_string(iStringNull) {};
+ QString(qstring_t string): m_string(string) {};
+
+ bool IsNull() const;
+ bool IsNullOrEmpty() const;
+
+ // Copy the array
+ QString &operator=(const QString &other);
+
+ bool operator==(qstring_t string) const;
+ bool operator==(const QString &s) const;
+ bool operator==(const char *pszString) const;
+
+ operator const char *() const;
+ operator unsigned int() const;
+ const char *str() const;
+
+private:
+ qstring_t m_string;
+};
+
+#ifdef USE_QSTRING
+#define string_t QString
+#endif
+
+#include "const.h"
+#include "edict.h"
+#include "eiface.h"
+#include "enginecallback.h"
+
+extern globalvars_t *gpGlobals;
+
+#define STRING(offset) ((const char *)(gpGlobals->pStringBase + (unsigned int)(offset)))
+#define MAKE_STRING(str) ((unsigned int)(str) - (unsigned int)(STRING(0)))
+
+// Inlines
+inline bool QString::IsNull() const
+{
+ return m_string == iStringNull;
+}
+
+inline bool QString::IsNullOrEmpty() const
+{
+ return IsNull() || (&gpGlobals->pStringBase[m_string])[0] == '\0';
+}
+
+inline QString &QString::operator=(const QString &other)
+{
+ m_string = other.m_string;
+ return (*this);
+}
+
+inline bool QString::operator==(qstring_t string) const
+{
+ return m_string == string;
+}
+
+inline bool QString::operator==(const QString &s) const
+{
+ return m_string == s.m_string;
+}
+
+inline bool QString::operator==(const char *pszString) const
+{
+ return strcmp(&gpGlobals->pStringBase[m_string], pszString) == 0;
+}
+
+inline const char *QString::str() const
+{
+ return &gpGlobals->pStringBase[m_string];
+}
+
+inline QString::operator const char *() const
+{
+ return str();
+}
+
+inline QString::operator unsigned int() const
+{
+ return m_string;
+}
diff --git a/dep/hlsdk/dlls/regamedll_api.h b/dep/hlsdk/dlls/regamedll_api.h
new file mode 100644
index 0000000..47bdb10
--- /dev/null
+++ b/dep/hlsdk/dlls/regamedll_api.h
@@ -0,0 +1,490 @@
+/*
+*
+* This program is free software; you can redistribute it and/or modify it
+* under the terms of the GNU General Public License as published by the
+* Free Software Foundation; either version 2 of the License, or (at
+* your option) any later version.
+*
+* This program is distributed in the hope that it will be useful, but
+* WITHOUT ANY WARRANTY; without even the implied warranty of
+* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
+* General Public License for more details.
+*
+* You should have received a copy of the GNU General Public License
+* along with this program; if not, write to the Free Software Foundation,
+* Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
+*
+* In addition, as a special exception, the author gives permission to
+* link the code of this program with the Half-Life Game Engine ("HL
+* Engine") and Modified Game Libraries ("MODs") developed by Valve,
+* L.L.C ("Valve"). You must obey the GNU General Public License in all
+* respects for all of the code used other than the HL Engine and MODs
+* from Valve. If you modify this file, you may extend this exception
+* to your version of the file, but you are not obligated to do so. If
+* you do not wish to do so, delete this exception statement from your
+* version.
+*
+*/
+#pragma once
+#include "archtypes.h"
+#include "regamedll_interfaces.h"
+#include "hookchains.h"
+#include "interface.h"
+#include "player.h"
+#include "gamerules.h"
+#include "cdll_dll.h"
+#include "items.h"
+
+#define REGAMEDLL_API_VERSION_MAJOR 5
+#define REGAMEDLL_API_VERSION_MINOR 3
+
+// CBasePlayer::Spawn hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_Spawn;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Spawn;
+
+// CBasePlayer::Precache hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_Precache;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Precache;
+
+// CBasePlayer::ObjectCaps hook
+typedef IHookChainClass IReGameHook_CBasePlayer_ObjectCaps;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_ObjectCaps;
+
+// CBasePlayer::Classify hook
+typedef IHookChainClass IReGameHook_CBasePlayer_Classify;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Classify;
+
+// CBasePlayer::TraceAttack hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_TraceAttack;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_TraceAttack;
+
+// CBasePlayer::TakeDamage hook
+typedef IHookChainClass IReGameHook_CBasePlayer_TakeDamage;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_TakeDamage;
+
+// CBasePlayer::TakeHealth hook
+typedef IHookChainClass IReGameHook_CBasePlayer_TakeHealth;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_TakeHealth;
+
+// CBasePlayer::Killed hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_Killed;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Killed;
+
+// CBasePlayer::AddPoints hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_AddPoints;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_AddPoints;
+
+// CBasePlayer::AddPointsToTeam hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_AddPointsToTeam;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_AddPointsToTeam;
+
+// CBasePlayer::AddPlayerItem hook
+typedef IHookChainClass IReGameHook_CBasePlayer_AddPlayerItem;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_AddPlayerItem;
+
+// CBasePlayer::RemovePlayerItem hook
+typedef IHookChainClass IReGameHook_CBasePlayer_RemovePlayerItem;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_RemovePlayerItem;
+
+// CBasePlayer::GiveAmmo hook
+typedef IHookChainClass IReGameHook_CBasePlayer_GiveAmmo;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_GiveAmmo;
+
+// CBasePlayer::ResetMaxSpeed hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_ResetMaxSpeed;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_ResetMaxSpeed;
+
+// CBasePlayer::Jump hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_Jump;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Jump;
+
+// CBasePlayer::Duck hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_Duck;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Duck;
+
+// CBasePlayer::PreThink hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_PreThink;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_PreThink;
+
+// CBasePlayer::PostThink hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_PostThink;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_PostThink;
+
+// CBasePlayer::UpdateClientData hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_UpdateClientData;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_UpdateClientData;
+
+// CBasePlayer::ImpulseCommands hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_ImpulseCommands;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_ImpulseCommands;
+
+// CBasePlayer::RoundRespawn hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_RoundRespawn;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_RoundRespawn;
+
+// CBasePlayer::Blind hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_Blind;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Blind;
+
+// CBasePlayer::Observer_IsValidTarget hook
+typedef IHookChainClass IReGameHook_CBasePlayer_Observer_IsValidTarget;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Observer_IsValidTarget;
+
+// CBasePlayer::SetAnimation hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_SetAnimation;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_SetAnimation;
+
+// CBasePlayer::GiveDefaultItems hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_GiveDefaultItems;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_GiveDefaultItems;
+
+// CBasePlayer::GiveNamedItem hook
+typedef IHookChainClass IReGameHook_CBasePlayer_GiveNamedItem;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_GiveNamedItem;
+
+// CBasePlayer::AddAccount hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_AddAccount;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_AddAccount;
+
+// CBasePlayer::GiveShield hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_GiveShield;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_GiveShield;
+
+// CBasePlayer:SetClientUserInfoModel hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_SetClientUserInfoModel;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_SetClientUserInfoModel;
+
+// CBasePlayer:SetClientUserInfoName hook
+typedef IHookChainClass IReGameHook_CBasePlayer_SetClientUserInfoName;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_SetClientUserInfoName;
+
+// CBasePlayer::HasRestrictItem hook
+typedef IHookChainClass IReGameHook_CBasePlayer_HasRestrictItem;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_HasRestrictItem;
+
+// CBasePlayer::DropPlayerItem hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_DropPlayerItem;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_DropPlayerItem;
+
+// CBasePlayer::DropShield hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_DropShield;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_DropShield;
+
+// CBasePlayer::OnSpawnEquip hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_OnSpawnEquip;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_OnSpawnEquip;
+
+// CBasePlayer::Radio hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_Radio;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Radio;
+
+// CBasePlayer::Disappear hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_Disappear;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_Disappear;
+
+// CBasePlayer::MakeVIP hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_MakeVIP;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_MakeVIP;
+
+// CBasePlayer::MakeBomber hook
+typedef IHookChainClass IReGameHook_CBasePlayer_MakeBomber;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_MakeBomber;
+
+// CBasePlayer::StartObserver hook
+typedef IVoidHookChainClass IReGameHook_CBasePlayer_StartObserver;
+typedef IVoidHookChainRegistryClass IReGameHookRegistry_CBasePlayer_StartObserver;
+
+// CBasePlayer::GetIntoGame hook
+typedef IHookChainClass IReGameHook_CBasePlayer_GetIntoGame;
+typedef IHookChainRegistryClass IReGameHookRegistry_CBasePlayer_GetIntoGame;
+
+// CBaseAnimating::ResetSequenceInfo hook
+typedef IVoidHookChainClass IReGameHook_CBaseAnimating_ResetSequenceInfo;
+typedef IVoidHookChainRegistryClass